React Query and AsyncStorage: How to Persist Data in React Native Apps

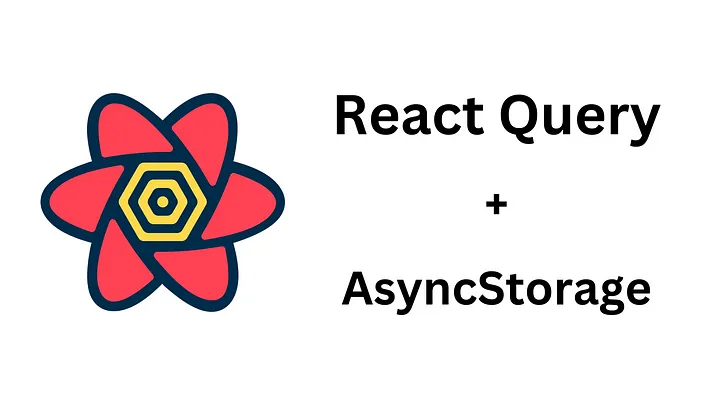
React Query is a state-management library that helps with fetching, caching, synchronizing, and updating the server state in our React/React-Native application. It provides a simple way to cache and manage data in a centralized location. It is simpler to share data across components and reduce the need for complex state management.
However, we can face a challenge with React Query, which is that it does not persist data when the application is restarted. This means that if a user closes the app and then reopens it, the previously fetched data will not be displayed, and the app will need to fetch the data again. This can negatively impact the user experience as users will need to wait for the data to be fetched before being able to view anything on the screen. In cases of network loss, users will not be able to see anything on the screen, which can again result in a poor user experience.
In this blog post, we will discuss how to use AsyncStorage to persist React Query data in a React Native application, and how to utilize this persisted data in offline mode.
What is React Query Persistor?
React Query Persistor is a feature provided by React Query that allows you to save and restore the cache data between application restarts. It also helps to restore stored data when the application is running in offline mode.
Note: React Query v4 supports the persistor, so make sure you have installed React Query v4.
To implement React Query Persistor with AsyncStorage follow the following steps:
Step 1: Install AsyncStorage
We need to install the following library to install AsyncStorage
in our React Native project:
npm install @react-native-async-storage/async-storage
Step 2: Install React Query Async Persistor
We need to install a utility that comes as a separate package and is available for import under the '@tanstack/query-async-storage-persister'
namespace.
npm install @tanstack/query-async-storage-persister @tanstack/react-query-persist-client
Step 3: Create a new asyncStoragePersister
First, we need to import createAsyncStoragePersister
, into the main entry file of our React Native application (usually App.jsx
or App.tsx
). Then we can create a new asyncStoragePersister
by passing the storage object to the createAsyncStoragePersister
function.
const asyncStoragePersister = createAsyncStoragePersister({
storage: AsyncStorage
})
Step 4: Wrap App into the PersistQueryClientProvider
Now, we need to import PersistQueryClientProvider
and wrap the entire App component with it.
import AsyncStorage from '@react-native-async-storage/async-storage'
import { QueryClient } from '@tanstack/react-query'
import { PersistQueryClientProvider } from '@tanstack/react-query-persist-client'
import { createAsyncStoragePersister } from '@tanstack/query-async-storage-persister'
const queryClient = new QueryClient();
const asyncStoragePersister = createAsyncStoragePersister({
storage: AsyncStorage
})
const App = () => (
<PersistQueryClientProvider
client={queryClient}
persistOptions={{ persister: asyncStoragePersister }}
>
// code here
</PersistQueryClientProvider>
);
export default App;
Step 5: Setting up Cache Time
For persist to work properly, we probably want to pass QueryClient
a cacheTime
value to override the default cache time which is 5 minutes.
Here, we are passing a cache time of 24 hours, which means that the data will be stored in AsyncStorage and can be used for up to 24 hours.
import AsyncStorage from '@react-native-async-storage/async-storage'
import { QueryClient } from '@tanstack/react-query'
import { PersistQueryClientProvider } from '@tanstack/react-query-persist-client'
import { createAsyncStoragePersister } from '@tanstack/query-async-storage-persister'
const queryClient = new QueryClient({
defaultOptions: {
cacheTime: 1000 * 60 * 60 * 24 // 24 hours
}
});
const asyncStoragePersister = createAsyncStoragePersister({
storage: AsyncStorage
})
const App = () => (
<PersistQueryClientProvider
client={queryClient}
persistOptions={{ persister: asyncStoragePersister }}
>
// code here
</PersistQueryClientProvider>
);
export default App;
Now, our data will be persisted in AsyncStorage, and we can access it even when the application is offline. When the app restarts, the persisted data will be automatically restored, reducing the number of API requests and improving the user experience.
Conclusion
In this blog post, we have discussed how to use the React Query Persistor to persist data in a React Native application using AsyncStorage. By persisting data and using it in offline mode, we can minimize network traffic and provide a seamless experience for users. With just a few simple steps, we can improve the overall performance and user experience of our React Native applications.
I hope you found this blog post helpful for your project! If you have any suggestions for improving this post, Iโd love to hear feedback in the comments.
Follow me on:
GitHub: https://github.com/tanmaythole
LinkedIn: https://www.linkedin.com/in/tanmay-thole-b82978175/
Thanks for reading, and happy coding!
Subscribe to my newsletter
Read articles from Tanmay Thole directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tanmay Thole
Tanmay Thole
Full stack Developer ๐ป | JavaScript โค๏ธ | Freelancer ๐จ๐พโ๐ป | Competitive Programmer ๐ | More https://tanmaythole.github.io/portfolio