PHP: GET and POST Methods
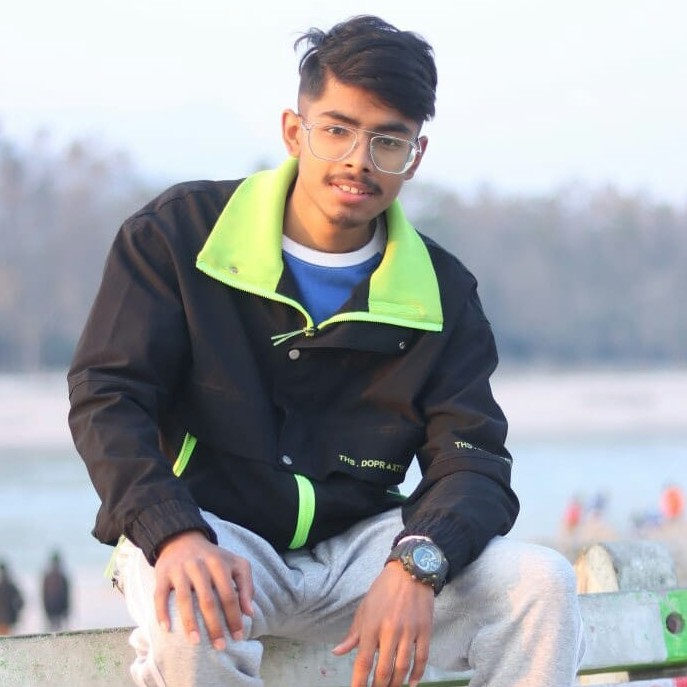
Table of contents
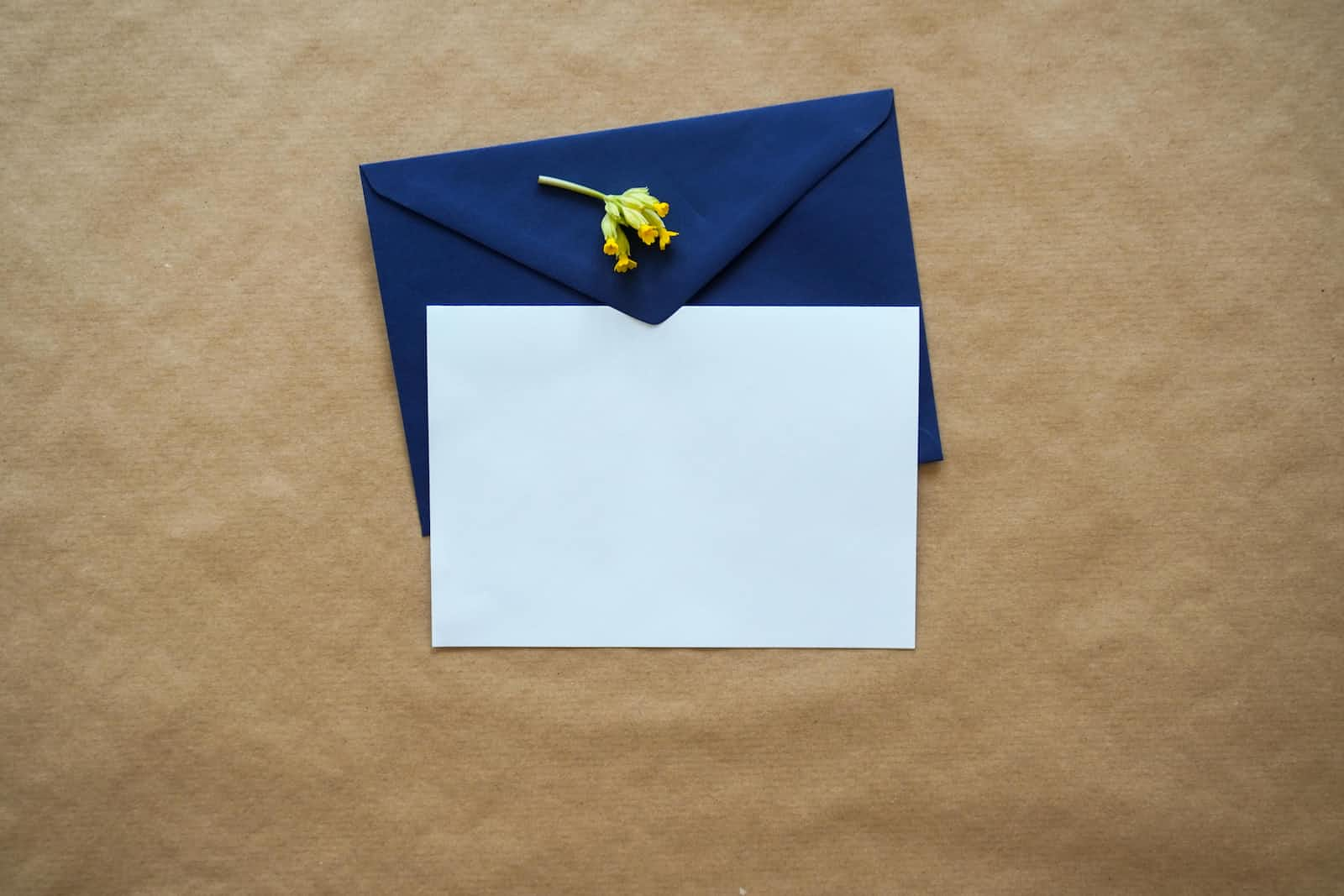
GET Method
It is a method to send data from a browser to a server. It is less secure because the data to be sent is shown in the URL. A form data can be submitted using the 'GET' method as follows:
form.php:
<?php
if(isset($_GET["submit"])){
// This code runs when form is submitted
echo $_GET['email'];
}
?>
<html>
<head><title>Get Method</title></head>
<body>
<form action="form.php" method="get">
<div>
<label for="mail">Email: </label>
<input type="email" name="email" id="mail">
</div>
<button type="submit" name="submit">Submit</button>
</form>
</body>
</html>
In the above code, '$_GET' is a global associative array in PHP that stores the form data submitted using a "GET" method. The "isset()" function checks if the variable has value or not. So, when the submit button is pressed, form data is stored in the '$_GET' array and PHP code is executed to do something with that data.
On form submit, the URL shows the form data as the 'key: value' pair:
http://localhost:3000/GET-and-POST/form.php?email=giver1234%40gmail.com&submit=
Let's break down the URL:
PHP file path starts from 'http' to 'form.php'
The '?' denotes the start of a query string to pass data to the server
KEY: 'email' is the 'name' attribute of the Email input box
VALUE: 'giver1234%40gmail.com' is the actual input given by the user
'&' is the separator of form data
'submit' is the 'name' attribute of the submit button
POST Method
It is also a method to send data from a browser to a server. But it is more secure and large data can be sent at a time. For example:
form.php:
<?php
// '$_POST' is also a global associative array
if(isset($_POST["submit"])){
// This code runs when form is submitted
echo $_POST['email'];
}
?>
<html>
<head><title>Post Method</title></head>
<body>
<form action="form.php" method="post">
<div>
<label for="mail">Email: </label>
<input type="email" name="email" id="mail">
</div>
<button type="submit" name="submit">Submit</button>
</form>
</body>
</html>
When the submit button is pressed, form data is stored in the '$_POST' array and PHP code is executed to handle that data.
NOTE: After submitting the form, the PHP code can be used for the client-side form validation using methods like:
empty(): Checks if a variable is empty or not. Returns 'true' if empty.
preg_match(): Tests string with a regular expression. Returns 'true' if matched.
Subscribe to my newsletter
Read articles from Giver Kdk directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
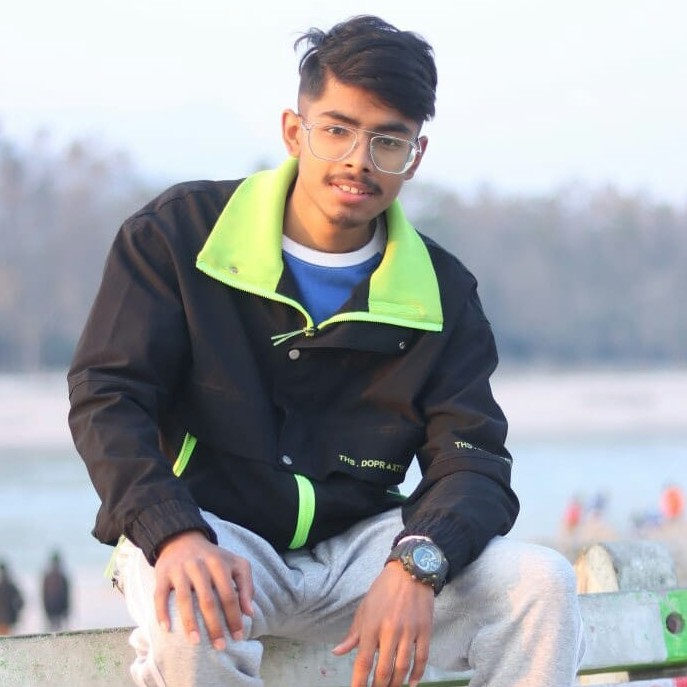
Giver Kdk
Giver Kdk
I am a guy who loves building logic.