Advanced Git and GitHub: Enhancing Collaboration and Version Control
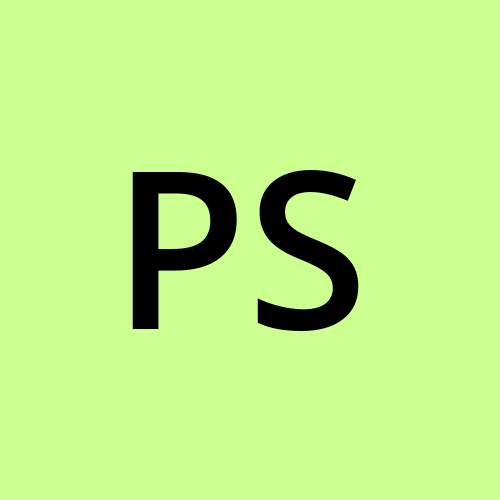
In this blog, we'll dig deep into Git branching, looking at concepts like rebasing and merging, and see how they can make project management better.
Git branching:
Git branching is like having multiple pathways for your code development. Imagine your code as a tree trunk, and each branch is a separate path where you can make changes without affecting the main trunk. Here's how it works:
Creating Branches: You can create these separate branches anytime you need them. The main branch is usually called "main" or "master" and is like the stable version of your code. But you can create new paths to work on different features, fix bugs, or try out new ideas.
Isolating Changes: Each branch keeps your changes separate from the main code until you're ready to bring them together. This way, you can develop new features or fix issues without causing problems in the main code.
Working in Parallel: With multiple branches, you and your team can work on different things at the same time. Each branch is like a different to-do list, making it easier to manage complex projects with many people.
Collaboration: Branches make it easy for your team to work together. Team members can create their own branches, make changes, and then suggest merging their changes into the main code.
Combining Changes: Git has tools to mix changes from one branch into another. Merging combines changes from one branch into another, while rebasing adds changes on top of another branch, keeping things neat.
Naming Your Paths: It's a good idea to give your branches names that show what they're for. For example, "feature/xyz" for working on new features, "bugfix/123" for fixing specific issues, and "hotfix/abc" for urgent fixes.
Git Revert
Git Revert is used to undo the changes introduced by a specific commit in a Git repository. You can use Git revert for the following purposes:
Fixing Mistakes: When a mistake is discovered in a commit (e.g., a bug or unintended change), Git Revert can be used to create a new commit that undoes those specific changes without altering the commit history.
Maintaining Commit History: Git Revert is a safe option for collaborative projects because it preserves the commit history. Other team members can see the mistake, how it was fixed, and when it was fixed.
Git Reset
Git Reset is a powerful Git command used to manipulate the commit history and the position of branch pointers. It serves several purposes:
Moving the Branch Starting Point: You can use Git Reset to change where a branch begins, essentially shifting it to a different commit.
Undoing Changes: Git Reset can be used to "undo" one or more commits by moving the branch back to a previous state. This removes those commits from the branch's history.
Managing Branches: Git Reset helps with branch management. It allows you to reset a branch to an earlier state, essentially starting fresh from that point.
Recovering Deleted Work: If you accidentally delete commits, Git Reset can help recover them by moving the branch back to where they were.
Reordering Commits: In advanced cases, Git Reset is used for interactive reordering or combining commits to make the commit history more organized.
Just remember that Git Reset can have significant effects on your project's history, so use it carefully, especially in shared repositories.
Git Rebase
Git Rebase is a Git command that helps you reorganize and simplify your branch's commit history by incorporating changes from one branch into another. It makes the history cleaner and more linear, which is helpful for better collaboration and understanding of the project's development. Use it to keep your branches up to date and improve the clarity of your Git history.
Git merge
Git Merge is a Git command used to combine changes from one branch into another. It's a way to integrate the work done in a feature branch, for example, back into the main branch.
Task 1:
Add a text file called version01.txt inside the Devops/ with “This is first feature of our application” written inside. This should be in a branch coming from master
, switch to dev
branch.
- version01.txt should reflect at local repo first followed by Remote repo for review.
Add a new commit in dev
branch after adding below mentioned content in Devops/version01.txt: While writing the file make sure you write these lines
1st line>> This is the bug fix in development branch
Commit this with the message “ Added feature2 in development branch”
2nd line>> This is gadbad code
Commit this with a message “ Added feature3 in the development branch
3rd line>> This feature will gadbad everything from now.
Commit with the message “ Added feature4 in the development branch
Restore the file to a previous version where the content should be “This is the bug fix in the development branch”.
Solution:
Create and switch to the
dev
branch frommain
.
git checkout -b dev
echo "This is the first feature of our application" > Devops/version01.txt
Commit this change with the message "Added new feature."
git add Devops/version01.txt
git commit -m "Added new feature"
git push origin dev
Add new commits in the dev
branch with the specified content and commit messages.echo "This is the bug fix in development branch" > Devops/version01.txt
git add Devops/version01.txt
git commit -m "Added feature2 in development branch
echo "This is gadbad code" > Devops/version01.txt
git add Devops/version01.txt
git commit -m "Added feature3 in development branch"
echo "This feature will gadbad everything from now." > Devops/version01.txt
git add Devops/version01.txt
git commit -m "Added feature4 in development branch"
Use git log
to get all the commit ids.
Now, git reset <commit_id>
will reset the changes to your desired commit.
Let's understand the difference between git merge and git rebase.
Task 2:
Demonstrate the concept of branches with 2 or more branches with screenshots.
As a practice try git rebase too, and see what difference you get.
Solution:
Git merge example:
- Add
main1.txt
inmain
branch and commit the change.
- Create a new branch
feature1
and add a file namedfile1.txt
to it. Commit the changes.
- Now,
git log
shows that firstlymain1.txt
added tomain
branch and laterfile1.txt
was added to branchfeature1
.
- Switch back to
main
and add a new filemain2.txt
. Commit the changes.
- Checkout to branch
feature1
and note down the current logs.
- Now, we will merge all the changes of
main
branch to ourfeature1
branch usinggit merge
command.
- Let's take a look at
git log
. Afterfile1.txt
commit, it shows the commit ofmain
branch first and then the log of merge command. This shows thatgit merge
will log commits as per the sequence of commands and in a non-linear format.
Git rebase:
- Switch to
main
branch and a new filemain3.txt
. Commit the change.
- Create a new branch
feature2
and add a new filefile1.txt
to it. Commit the change. Here, ingit log
we can see thatmain3.txt
was committed first and laterfile1.txt
- Let's checkout to
main
again and add a new filemain4.txt
. Commit the change.
- Switch to
feature2
branch and note down the logs.
- Hit
git rebase main
to update the changes frommain
tofeature2
branch. Let's pay some attention to the logs here to understand the difference betweengit merge
andgit rebase
. Unlikegit merge
,git rebase
makes an entry ifmain4.txt
beforefile1.txt
commit. This makes git commit history linear.
It depends on your requirements on how you want to maintain the history of commits on the git repository. Accordingly, choose between these 2 options.
Thank you!
Subscribe to my newsletter
Read articles from Payal Saindane directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
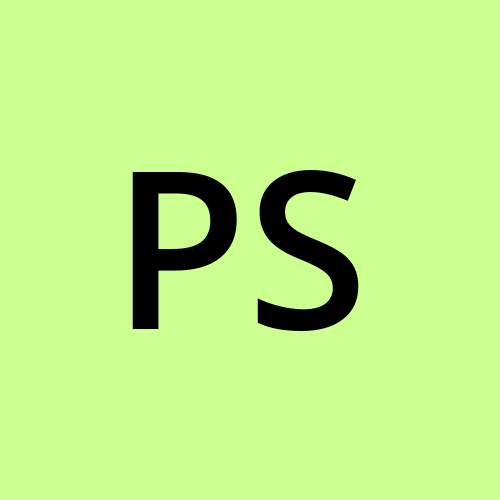
Payal Saindane
Payal Saindane
👨💻 Software Engineer on 🛠️ DevOps Journey | ☁️ AWS Cloud Explorer