Day 15: Python Libraries for DevOps
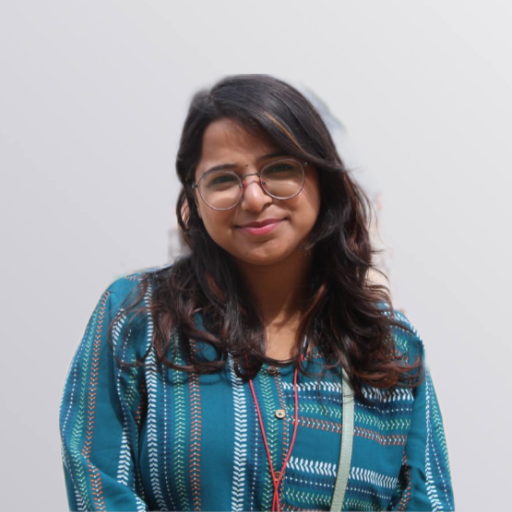

What is JSON?
JSON stands for "JavaScript Object Notation." It is a widely used, language-agnostic data interchange format that is easy to read and write. JSON represents structured data as key-value pairs and can be used in various programming languages for data storage and exchange.
Here's a simple example of JSON data:
{
"name": "John Doe",
"age": 30,
"isStudent": false,
"hobbies": ["reading", "swimming", "hiking"],
"address": { "street": "123 Main St", "city": "Exampleville", "state": "CA" }
}
To convert Python data to JSON string:
import json as js
data = {"name": "Tom", "age": 20}
json_str= js.dumps(data)
print(json_str)
To convert JSON string to Python data structure,
import json as js
json_str = '{"name": "Tom", "age":20}'
data=js.loads(json_str)
print(data["name"])
What is YAML?
YAML, or "YAML Ain't Markup Language," is a human-readable data serialization format used for configuration files and data exchange. Its clean syntax and simplicity make it popular in software development and system administration.
Python converts into yaml:
import yaml
data = {"name": "Tom", "age":20, "city": "NewYork"}
yaml_data = yaml.dump(data,default_flow_style = False)
YAML convert into Python:
python_data =
yaml.safe
.load(yaml_data)
print(f"name: {python_data["name"]}")
Tasks:
Create a Dictionary in Python and write it to a JSON File.
json.dumps(): used to convert Python dictionary to JSON string.
2. Read a JSON file services.json
kept in this folder and print the service names of every cloud service provider.
json.load()
takes a file object and returns the json object. A JSON object contains data in the form of key/value pair. The keys are strings and the values are the JSON types. Keys and values are separated by a colon. Each entry (key/value pair) is separated by a comma.
Read the YAML file using Python, file
services. yaml
and read the contents to convert yaml to jsonIf you need to convert YAML to JSON, you can parse the YAML as we did above. In the next step, you can use the JSON module to convert the object to JSON.
Thank you for reading :-)
Priyanka Varshney
Subscribe to my newsletter
Read articles from priyanka varshney directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
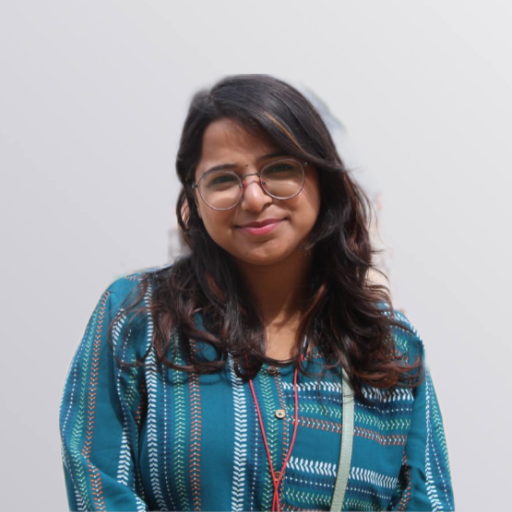
priyanka varshney
priyanka varshney
๐ Hello, and welcome to my DevOps journey! ๐ I am Priyanka Varshney,๐ ๏ธ As an aspiring DevOps engineer, I'm all about bridging the gap between development and operations, making software delivery seamless and efficient. ๐ป๐ง On this Hashnode blog, I'll be sharing my learnings, experiences and adventures as I dive deep into the world of continuous integration, automation, and cloud technologies. โ๏ธโ๏ธ Let's connect, learn, and grow as a vibrant DevOps community. Follow my Hashnode blog, and let's embrace the DevOps adventure together! ๐ค๐