Removing the Last borderBottomWidth in a React Native List
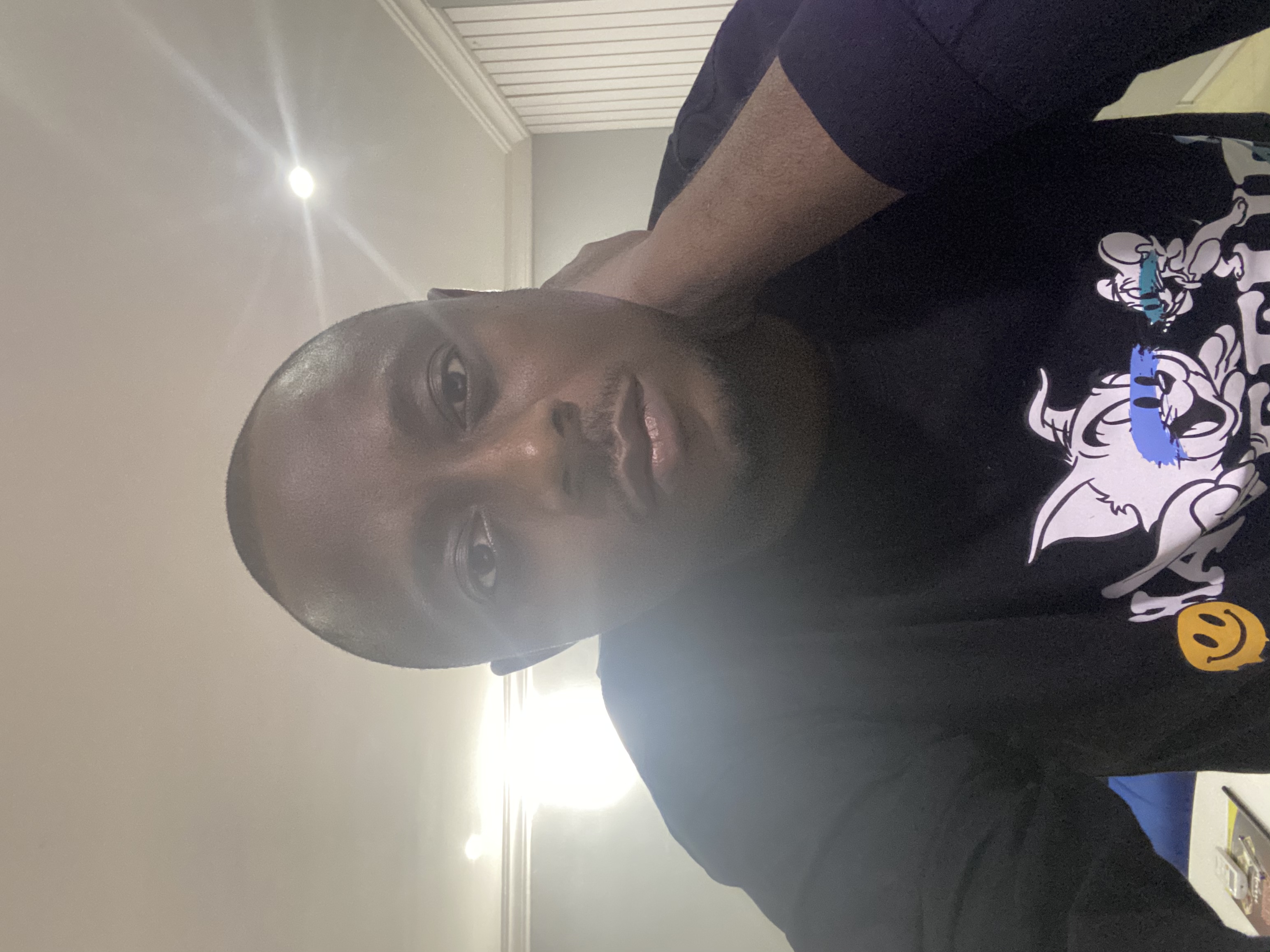
Table of contents
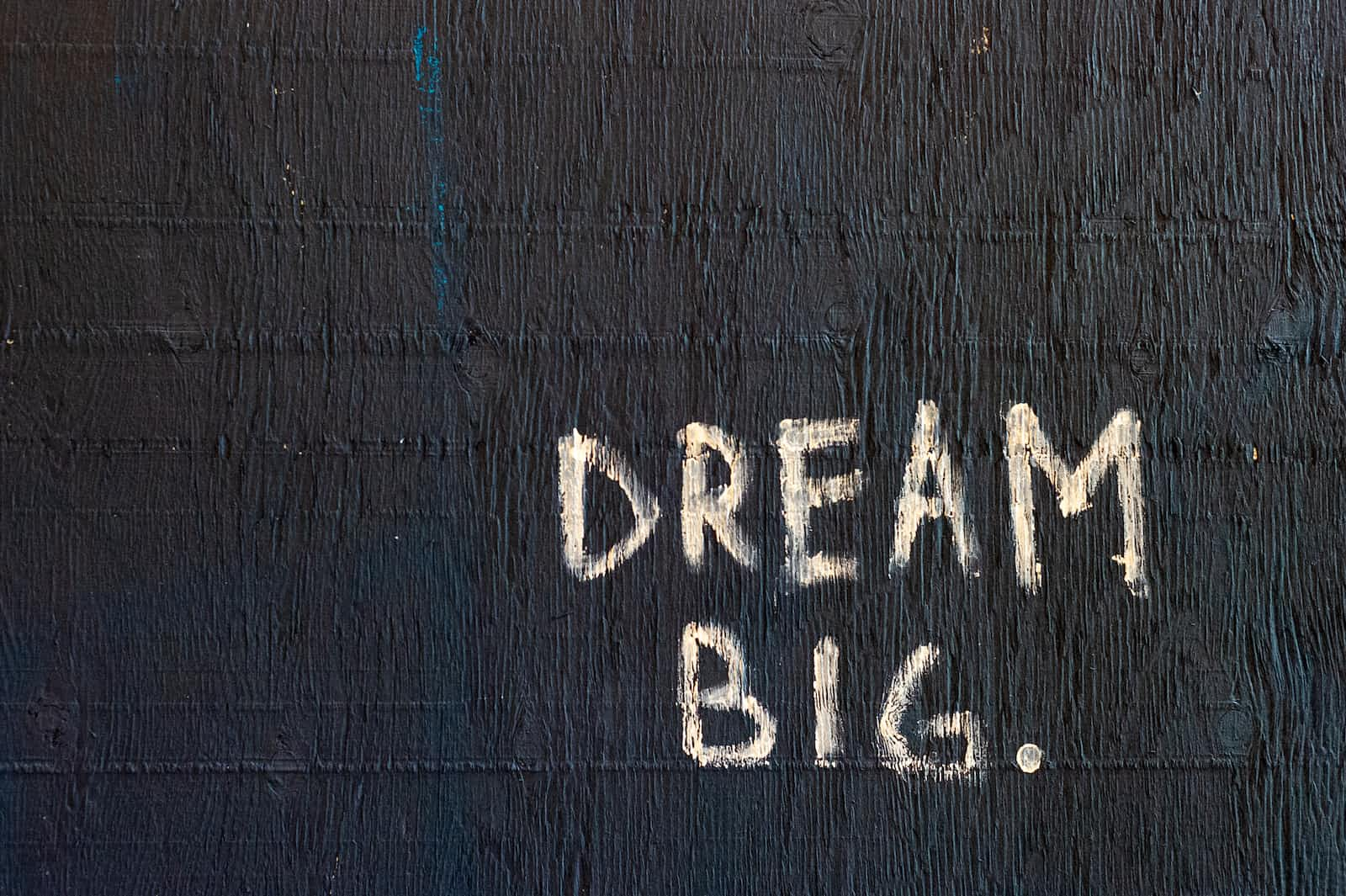
When developing a React Native application, you often need to display lists of items. Styling these lists is essential to create a visually appealing user interface. However, there are cases where you want to remove the last borderBottomWidth
from the last item in the list to achieve a cleaner design. In this blog post, we'll explore how to accomplish this task using React Native.
Styling Lists in React Native
React Native provides a flexible and efficient way to style components using JavaScript. You can use the built-in StyleSheet
API to define and manage styles for your components. Let's consider an example where you have a list of locations, and you want to remove the borderBottomWidth
from the last item in the list.
Here's a simplified React Native component that displays a list of locations with borderBottomWidth
for each item:
import React from 'react';
import { View, Text, StyleSheet, ScrollView } from 'react-native';
import { MaterialIcons } from '@expo/vector-icons';
const LocationList = () => {
const locations = [
{ name: 'Location 1', city: 'City 1' },
{ name: 'Location 2', city: 'City 2' },
{ name: 'Location 3', city: 'City 3' },
{ name: 'Location 4', city: 'City 4' },
];
return (
<ScrollView style={styles.container}>
{locations.map((location, index) => (
<View style={styles.locationItem} key={index}>
<MaterialIcons name="location-on" size={20} color="black" />
<View style={[styles.locationText, index === locations.length - 1 && styles.noBorder]}>
<Text style={styles.locationName}>{location.name}</Text>
<Text style={styles.locationCity}>{location.city}</Text>
</View>
</View>
))}
</ScrollView>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
},
locationItem: {
flexDirection: 'row',
alignItems: 'center',
paddingVertical: 10,
borderBottomWidth: 1,
borderBottomColor: '#d9d9d9',
},
locationText: {
marginLeft: 10,
},
locationName: {
fontSize: 16,
},
locationCity: {
color: '#555',
},
noBorder: {
borderBottomWidth: 0,
borderBottomColor: 'transparent',
},
});
export default LocationList;
In this code, we have a LocationList
component that maps through an array of locations and renders them as a list of items. We use conditional styling to remove the borderBottomWidth
and borderBottomColor
for the last item in the list. This creates a more polished and cleaner appearance, where the last item doesn't have an unnecessary border.
The key to achieving this is the styles.noBorder
style, which sets borderBottomWidth
to 0 and borderBottomColor
to 'transparent' for the last item in the list.
By using this approach, you can easily customize your React Native lists and achieve a more professional and appealing user interface.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
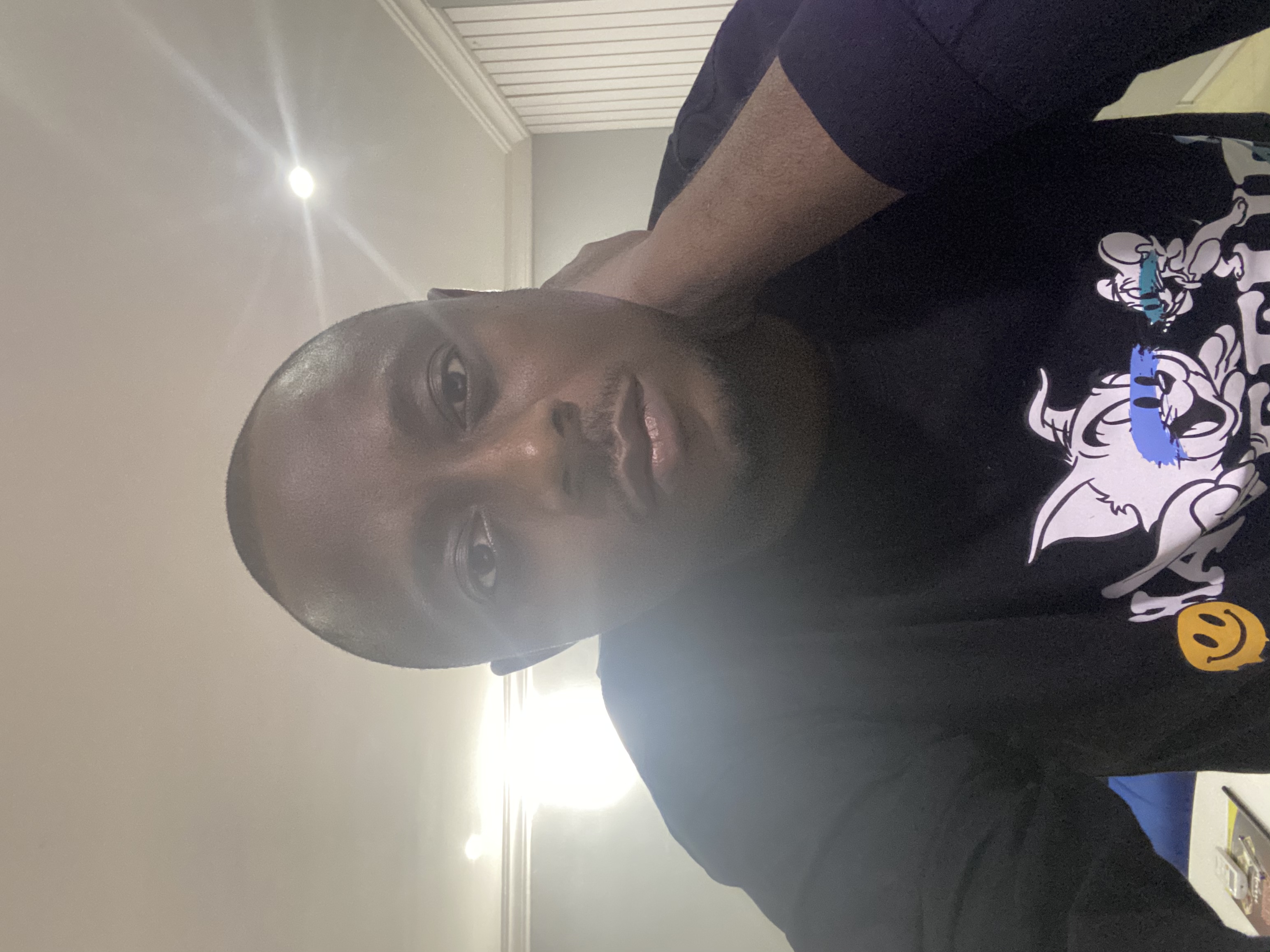
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.