Real-World Examples of Functional Programming Patterns
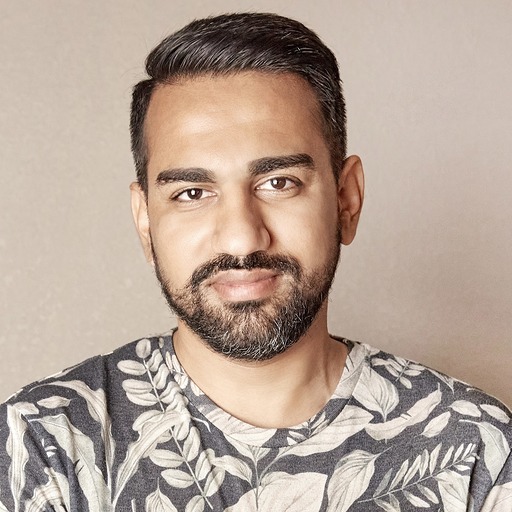
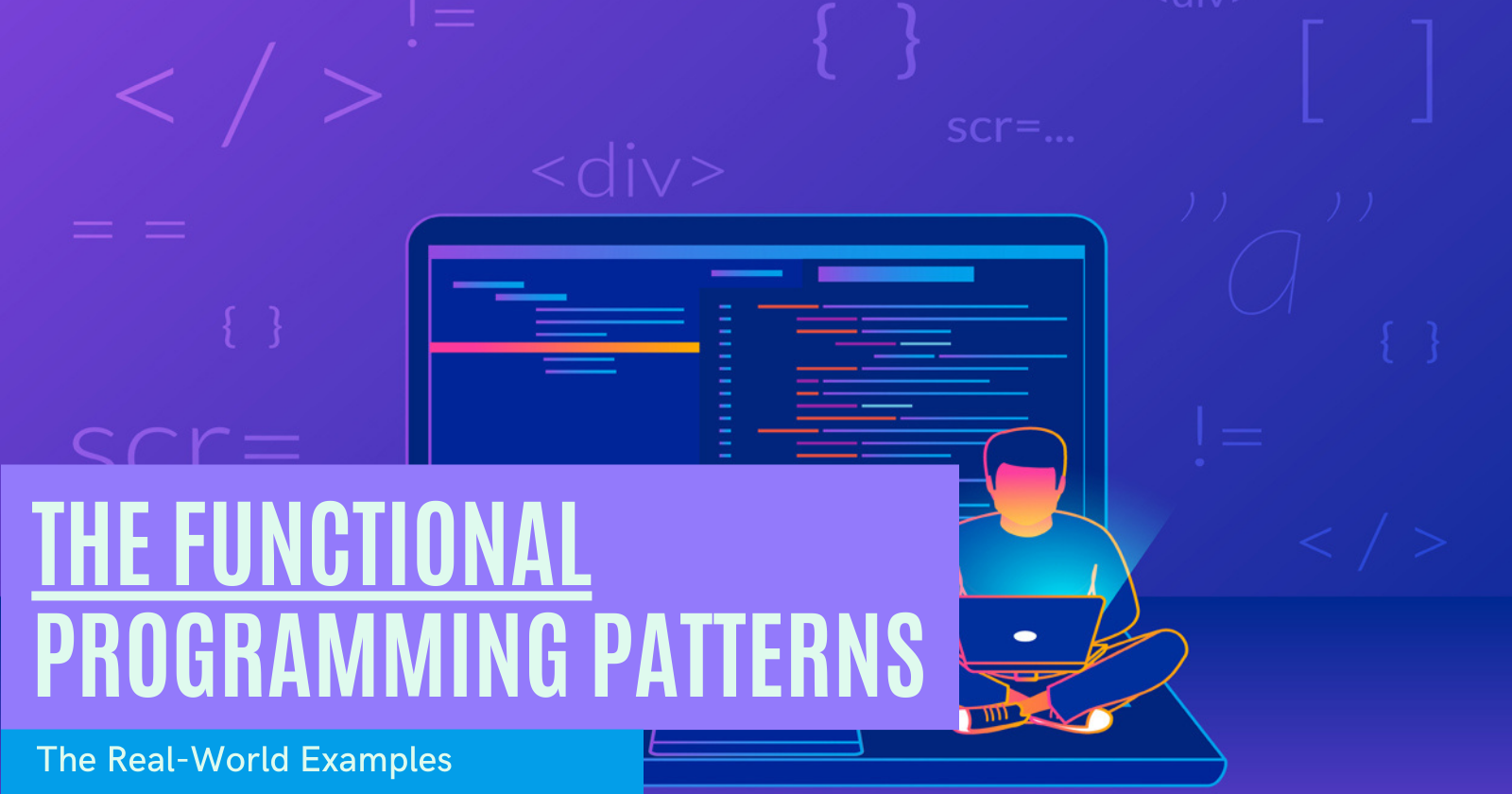
Functional programming is a programming paradigm that emphasizes the use of pure functions, immutability, and higher-order functions to build software. It's a paradigm that has gained popularity in recent years due to its advantages in terms of code maintainability, scalability, and testability. In this article, we will explore real-world examples of functional programming patterns and how they can be applied to solve practical problems.
At Coding Crafts, a leading name in Custom Software Development Company in USA, we've harnessed the power of functional programming to deliver top-notch solutions to our clients. We'll show you how real-world examples of functional programming patterns can make a difference.
Map, Filter, and Reduce
One of the fundamental concepts in functional programming is the use of higher-order functions like map, filter, and reduce. These functions are powerful tools for processing collections of data. Let's consider an example of using these functions to manipulate an array of numbers:
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map((num) => num * num);
const evenNumbers = numbers.filter((num) => num % 2 === 0);
const sum = numbers.reduce((acc, num) => acc + num, 0);
These functions make the code more readable and declarative, and they are widely used in real-world applications to transform and manipulate data.
Immutability
Immutability is a key concept in functional programming. Instead of modifying data in place, functional programming encourages creating new data structures with the desired changes. This approach leads to safer and more predictable code. Consider a simple example of working with an immutable data structure in JavaScript:
const originalArray = [1, 2, 3, 4, 5];
const newArray = [...originalArray, 6];
By creating a new array, we maintain the integrity of the original data, making it easier to reason about and test our code.
First-Class and Higher-Order Functions
Functional programming treats functions as first-class citizens, allowing them to be assigned to variables, passed as arguments, and returned as values. This concept is particularly useful in real-world scenarios, such as event handling or asynchronous operations in JavaScript.
const multiply = (x) => (y) => x * y;
const double = multiply(2);
const triple = multiply(3);
const result1 = double(5); // 10
const result2 = triple(5); // 15
This pattern enables code reuse and flexibility in designing software.
Recursion
Recursion is a common technique in functional programming, and it involves solving problems by breaking them down into smaller, similar sub-problems. A classic example is calculating the factorial of a number using recursion in JavaScript:
const factorial = (n) => (n <= 1 ? 1 : n * factorial(n - 1));
While recursion can sometimes be less efficient than iterative solutions, it showcases the elegance and simplicity of functional programming.
Monads
Monads are a more advanced concept in functional programming, often used to manage side effects in a pure and composable way. One real-world example of a monad is the Maybe
monad, which handles optional values, avoiding null or undefined errors.
const safeDivide = (a, b) => (b === 0 ? Maybe.Nothing() : Maybe.Just(a / b));
By using the Maybe
monad, we ensure that the division operation is safe, even if b
is zero, without introducing null checks.
Conclusion
Functional programming patterns provide powerful tools for writing clean, maintainable, and scalable code. In this article, we explored real-world examples of functional programming patterns, including map, filter, and reduce, immutability, first-class and higher-order functions, recursion, and monads. These patterns are widely applied in modern software development, enhancing code quality and promoting better software design.
At Coding Crafts, we leverage these functional programming patterns to deliver cutting-edge solutions. As one of the Best IT Companies in USA, we take pride in our commitment to delivering exceptional software solutions.
If you're interested in learning more about functional programming and its applications, here are some valuable resources:
Mozilla Developer Network: Provides comprehensive documentation and tutorials on JavaScript, which includes functional programming concepts.
Eloquent JavaScript: An online book by Marijn Haverbeke that covers JavaScript and includes a section on functional programming.
Functional Programming Principles in Scala (Coursera): A Coursera specialization that covers functional programming principles in Scala.
Haskell Programming from First Principles: A resource for learning Haskell, a functional programming language, from scratch.
Functional Programming in C# (Pluralsight): A Pluralsight course that explores functional programming concepts in C#.
Functional Programming for the Web (edX): An edX professional certificate program that introduces functional programming for web development.
Coding Crafts: Go there for more insights and services related to technologies.
Please note that the availability and content of these resources may change over time, so I recommend conducting an online search to find the most up-to-date and relevant materials for your specific interests and needs.
Subscribe to my newsletter
Read articles from Hakeem Abbas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
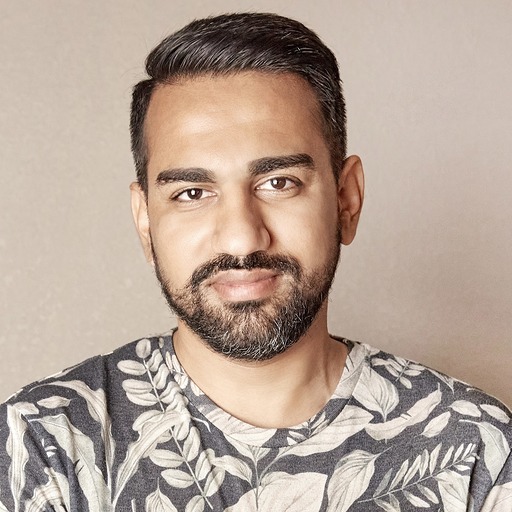
Hakeem Abbas
Hakeem Abbas
Hakeem Abbas is a Software Engineer based in San Francisco, Bay Area. Besides building beautiful web and mobile applications that scale well, Hakeem likes to spend his time travelling, hiking and trying new restaurants.