Mastering JavaScript: A Comprehensive Guide for Beginners
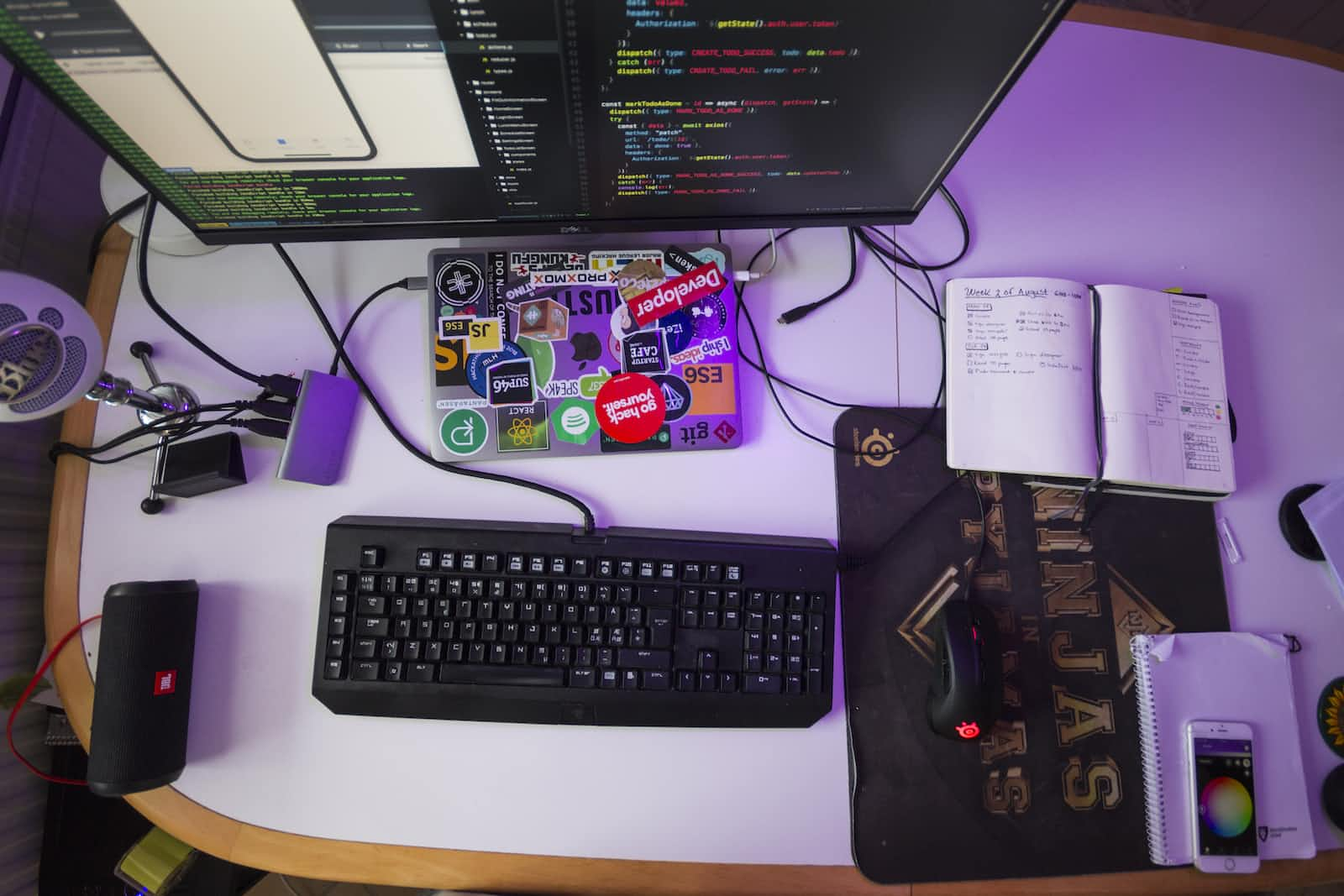
JavaScript, often hailed as the "language of the web," is a programming language that lies at the heart of modern web development. With JavaScript, you can bring your web pages to life, add interactivity, and create dynamic content. But, the world of JavaScript might seem daunting at first glance. A single scroll through a long list of JavaScript concepts can be overwhelming. To overcome this, here's the secret: You don't need to learn it all at once. You can start with the basics and gradually build your knowledge from there.
My journey with JavaScript began through Angela Yu's Complete Web Development Course on Udemy. At first, I found myself losing interest as the self-paced, online learning format lacked the motivation I needed. Well, does it? I was just me being overwhelmed with it being my first approach to tech related topics after switching from Animal production and health. I was a novice Consequently, I abandoned it temporarily. Nevertheless, I realized that the key to mastering any skill lies in dedication and perseverance. So, I decided to take a step back, reassess my approach, and approach my JavaScript learning journey with renewed seriousness and commitment. This shift in mindset allowed me to overcome the initial hurdles and rediscover the excitement of coding with JavaScript.
You can also consider my experience as a valuable lesson in your journey. Learning a new skill, especially something as powerful and versatile as JavaScript, can be challenging. Don't be disheartened by initial struggles or a loss of motivation. Instead, remember that perseverance and dedication are the keys to success. Take inspiration from my story, where I overcame obstacles by recommitting to my learning process. You can do it too. By staying consistent and embracing the learning curve, you'll break down the incredible world of JavaScript, and it will undoubtedly become a valuable tool in your skillset.
Let's break down JavaScript into manageable sections and highlight the fundamental concepts that every aspiring web developer should master.
1. Variables: The Building Blocks
Variables are essential in any programming language. In JavaScript, you have three main ways to declare them: var
, let
, and const
. These keywords allow you to store and manage data.
In short:
Variables include • var • let • const
2. Data Types: Understanding Your Data
JavaScript supports various data types, including numbers, strings, booleans, null, undefined, and symbols. These types help you represent different kinds of information in your programs.
In shortData Types includes • number • string • boolean • null • undefined • symbol
3. Declaring Variables: Storing Information
The way you declare variables in JavaScript significantly affects their behavior. You can use var
, let
, or const
to create and manage variables in your code.
In shortDeclaring variables includes • var • let • const
4. Expressions: The Language of JavaScript
JavaScript expressions are the building blocks of your code. They can be primary or left-hand-side expressions. These include literals, arrays, functions, classes, and more.
Expressions Include 1. Primary expressions • this • Literals • [] • {} • function • class • function* • async function • async function* • /ab+c/i • string • ( ) 2. Left-hand-side expressions • Property accessors
• ?
• new
• new .target
• import.meta
• super
• import()
5. Operators: Making Things Happen
Operators in JavaScript are symbols that perform operations on variables and values. These operations can be arithmetic, comparison, or logical, allowing you to manipulate data as needed.
In shortoperators include • Arithmetic Operators: +, -, *, /, % • Comparison Operators: ==, ===, !=, !==, <, >, <=, >= • Logical Operators: &&, ||, !
6. Control Structures: Directing Your Program
Control structures, such as if
, else
, and switch
, which allows you to control the flow of your program. They help you make decisions and execute different codes based on conditions.
In ShortControl Structures include • if • else if • else • switch • case • default
7. Iterations/Loops: Repeating Tasks
Loops are vital for automating repetitive tasks in your programs. JavaScript provides various types of loops, including for
, while
, and do...while
each with its specific use cases.
In ShortIterations/Loop • do...while • for • for...in • for...of • for await...of • while
8. Functions: Building Blocks of Code
Functions are reusable blocks of code that perform a specific task. JavaScript offers arrow functions, default parameters, and more to help you create modular and efficient code.
In shortFunctions include • Arrow Functions • Default parameters • Rest parameters • arguments • Method definitions • getter • setter
9. Objects and Arrays: Structuring Data
Objects and arrays are essential for organizing and manipulating data. Objects use key-value pairs, while arrays are lists of values. You can apply various methods to both objects and arrays.
In shortObjects and Arrays • Object Literal: { key: value } • Array Literal: [element1, element2, ...] • Object Methods and Properties • Array Methods: push(), pop(), shift(), unshift(), splice(), slice(), forEach(), map(), filter()
10. Classes and Prototypes: Blueprint for Objects
JavaScript supports classes and prototypes for creating objects and managing their inheritance. Understanding these concepts is crucial for building complex applications.
In shortClasses and Prototypes include • Class Declaration • Constructor Functions • Prototypal Inheritance • extends keyword • super keyword • Private class features • Public class fields • static • Static initialization blocks
11. Error Handling: Dealing with the Unexpected
Error handling with try
, catch
, and finally
is a critical skill when writing robust and reliable JavaScript code.
In shortError Handling include • try • catch • finally (exception handling)
Advanced Concepts: The Mastery
As you progress in your JavaScript journey, you'll encounter advanced concepts like closures, asynchronous programming, modules, event handling, DOM manipulation, regular expressions, and browser and web APIs. These topics provide additional depth and enable you to build complex, interactive web applications.
In short, the advance concept is divided into several topics which include:
- Closures
• Lexical Scope
• Function Scope
• Closure Use Cases
Asynchronous JavaScript
• Callback Functions
• Promises
• async/await Syntax
• Fetch API
• XMLHttpRequestModules
• import and export Statements (ES6 Modules)
• CommonJS Modules (require, module.exports)Event Handling
• Event Listeners
• Event Object
• Bubbling and CapturingDOM Manipulation
• Selecting DOM Elements
• Modifying Element Properties
• Creating and Appending ElementsRegular Expressions
• Pattern Matching
• RegExp Methods: test(), exec(), match(), replace()Browser APIs
• localStorage and sessionStorage
• navigator Object
• Geolocation API
• Canvas APIWeb APIs
• setTimeout(), setInterval()
• XMLHttpRequest
• Fetch API
• WebSocketsFunctional Programming
• Higher-Order Functions
• map(), reduce(), filter()
• Pure Functions and ImmutabilityPromises and Asynchronous Patterns
• Promise Chaining
• Error Handling with Promises
• Async/AwaitES6+ Features
• Template Literals
• Destructuring Assignment
• Rest and Spread Operators
• Arrow Functions
• Classes and Inheritance
• Default Parameters
• let, const Block ScopingBrowser Object Model (BOM)
• window Object
• history Object
• location Object
• navigator ObjectNode.js Specific Concepts
• require()
• Node.js Modules (module. exports)
• File System Module (fs)
• npm (Node Package Manager)Testing Frameworks
• Jasmine
• Mocha
• Jest
These advanced concepts will not only enrich your understanding of JavaScript but also empower you to tackle more complex projects and explore various career opportunities.
My final thoughts on it
As you navigate the world of JavaScript, the journey from novice to expert is marked by your willingness to explore these advanced topics and apply them in practical coding scenarios.
Remember, mastering JavaScript is a journey, not a race. Start with the basics, and gradually explore the advanced concepts as you become more comfortable. Don't get overwhelmed—just take it one step at a time. Happy coding! 🚀✨
Disclaimer: The content provided in this blog is intended as a simplified overview of JavaScript concepts for beginners. It's essential to explore each topic in more detail for a complete understanding.
Subscribe to my newsletter
Read articles from The Tech Lover directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
The Tech Lover
The Tech Lover
One thing I love doing is helping people, and I believe one of the key ways I do this is through sharing my knowledge and personal experiences. Prior to this time, I had taught myself several programming languages, libraries, and frameworks for web development, considering myself a full-stack developer with knowledge in HTML, CSS, JavaScript, Node.js, WordPress, React, and SQL. However, I felt I needed more - more learning, more practice, more projects, and more connections with people in the industry. Until May 2023, I wasn't a social person, but joining Twitter changed that. There, I saw an opportunity to learn web3 from learnweb3.io. I began the course but faced challenges when it came to deploying my DApp since I needed Ethereum. My journey took a new direction when I discovered the ALX Software Engineering program for Africans. I immediately applied and got accepted, which marked a new phase in my life as a Software Engineering Student. Despite the challenges of unreliable electricity in my country, I remain committed to the program and plan to document my journey here on this platform. I will share my progress, experiences, and insights on becoming a Software Engineer and learning web3. I invite anyone looking to transition into software engineering or web3 development to follow my journey. Whether you are self-taught or considering teaching yourself, my posts will provide relevant content and valuable resources. Through this platform, I make a commitment to stay accountable by sharing what I learn. Please feel free to share your thoughts and comments on my posts, as your engagement will keep me motivated and focused on pursuing my dream.