Prisma ORM for Database Management

Table of contents
- 1. Introduction to Prisma ORM
- 2. Getting Started with Prisma
- 3. CRUD Operations with Prisma
- 4. Data Modeling and Relationships
- 5. Advanced Prisma Features
- 6. Query Performance and Optimization
- 7. Security and Authentication
- 8. Scaling and Deployment
- 9. Best Practices
- 10. Community and Resources
- 11. Conclusion
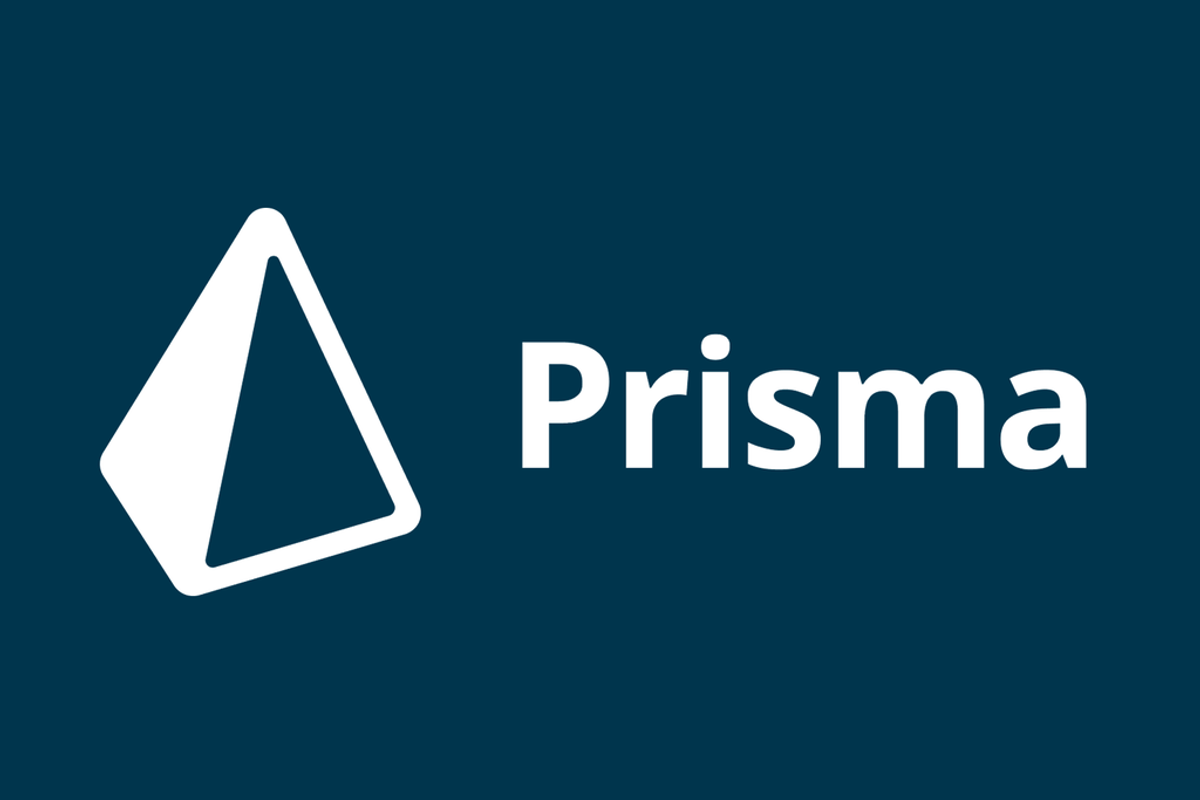
Managing databases is a fundamental aspect of many software applications. Databases store, organize, and retrieve data, making them a critical component of most modern applications. However, interacting with databases can be complex and error-prone, particularly when dealing with relational databases. This is where Object-Relational Mapping (ORM) tools like Prisma come into play.
In this comprehensive guide, we will explore Prisma as an ORM tool and learn how to use it for efficient and effective database management. We will cover everything from the basics of ORM and Prisma's core features to advanced use cases and best practices for developing database-driven applications.
Now, let's dive into the world of Prisma ORM and understand how it can simplify and enhance database management.
1. Introduction to Prisma ORM
What is an ORM?
An Object-Relational Mapping (ORM) tool is a software library or framework that simplifies database operations by mapping database records to objects in code. ORM tools bridge the gap between the object-oriented programming world and relational databases, allowing developers to work with databases using familiar programming concepts.
ORM tools like Prisma handle tasks such as data retrieval, storage, and manipulation, abstracting away the underlying SQL queries. This simplifies the development process, making it more efficient and less error-prone.
Why Use Prisma as an ORM?
Prisma has gained popularity as an ORM tool due to its many advantages, including:
Type Safety: Prisma generates strongly typed query builders based on your database schema. This means you get compile-time checks, autocompletion, and a reduced risk of runtime errors.
Database Agnostic: Prisma supports various databases, including PostgreSQL, MySQL, and SQLite. This allows you to switch databases without rewriting your entire data access layer.
Ease of Use: Prisma's intuitive API makes it straightforward to define data models and perform database operations. It follows a declarative approach, allowing you to focus on what you want to achieve, not how to achieve it.
Performance: Prisma optimizes queries and minimizes the number of database requests, resulting in improved performance.
Real-Time Data: Prisma is designed for real-time applications. It can work seamlessly with GraphQL subscriptions or other real-time technologies.
With these advantages, Prisma has become a valuable tool for modern application development. Let's proceed to the practical aspects of using Prisma.
2. Getting Started with Prisma
Installation and Setup
Getting started with Prisma involves a few key steps:
Installation: Begin by installing Prisma globally using npm or yarn:
npm install -g prisma # or yarn global add prisma
Prisma Client Generation: Use the Prisma CLI to generate a Prisma Client for your project:
prisma generate
Database Configuration: Configure your database connection in the
schema.prisma
file. Prisma supports various database providers, and you can specify the connection details in this file.// schema.prisma datasource db { provider = "postgresql" url = env("DATABASE_URL")
}
### Defining a Data Model <a name="defining-a-data-model"></a>
In Prisma, data models are defined using the Prisma Schema Definition Language (SDL) within the `schema.prisma` file. A data model describes the structure and relationships of your data.
Here's an example of defining a simple data model for a blog application:
```prisma
// schema.prisma
model User {
id Int @id @default(autoincrement())
name String
email String @unique
posts Post[]
}
model Post {
id Int @id @default(autoincrement())
title String
content String
author User @relation(fields: [authorId], references: [id])
authorId Int
published Boolean @default(false)
createdAt DateTime @default(now())
}
This data model defines two entities, User
and Post
, and establishes a one-to-many relationship between them. The @relation
and @default
attributes provide additional information about the fields and relationships.
With Prisma, you can easily define your data models and relationships, and Prisma will generate the necessary database schema and query builders.
3. CRUD Operations with Prisma
Prisma simplifies common database operations, often referred to as CRUD (Create, Read, Update, Delete) operations. Let's explore how to perform these operations using Prisma.
Creating Records
To create a new record in the database using Prisma, you can use the create
method:
const newUser = await prisma.user.create({
data: {
name: 'John Doe',
email: 'john@example.com',
// ...other fields
},
});
The create
method takes an object with the data you want to insert into the database. Prisma will generate the appropriate SQL query to perform the insertion.
Reading Records
Prisma offers various methods for reading records from the database. The simplest way is to use the findUnique
method to retrieve a single record by its unique identifier:
const user = await prisma.user.findUnique({
where: {
id: 1, // User ID
},
});
You can also query multiple records using methods like findMany
. Prisma allows you to filter, sort, and paginate the results to suit your needs.
const activeUsers = await prisma.user.findMany({
where: {
active: true,
},
orderBy: {
name: 'asc',
},
take: 10, // Limit the number of results
skip: 0, // Offset for pagination
});
Updating Records
To update records, use the update
method:
const updatedUser = await prisma.user.update({
where: {
id: 1, // User ID
},
data: {
name: 'Updated Name',
// ...other fields to update
},
});
Prisma will generate the SQL query to update the specified fields in the database.
Deleting Records
To delete records, use the delete
method:
await prisma.user.delete({
where: {
id: 1, // User ID
},
});
Prisma will generate the SQL query to delete the specified record.
These are the fundamental operations you can perform with Prisma. However, Prisma offers much more, including support for complex queries and relationships.
4. Data Modeling and Relationships
Efficiently modeling data and defining relationships between entities is a crucial aspect of database management. Prisma simplifies this process and allows you to create sophisticated data models with ease.
Defining Relationships
In the Prisma data model, you can define relationships between entities using the @relation
attribute. Relationships can be one-to-one, one-to-many, or many-to-many. For example, to establish a one-to-many relationship between User
and Post
, you can define it as follows:
model User {
id Int @id @default(autoincrement())
name String
email String @unique
posts Post[]
}
model Post {
id Int @id @default(autoincrement())
title String
content String
author User @relation(fields: [authorId], references: [id])
authorId Int
published Boolean @default(false)
createdAt DateTime @default(now())
}
Here, the User
model has a one-to-many relationship with the Post
model. Prisma will generate the necessary foreign key relationships in the database schema.
One-to-One Relationships
One-to-one relationships can be defined in a similar manner. For instance, if you want to establish a one-to-one relationship between a User
and a Profile
, you can define it as follows:
model User {
id Int @id @default(autoincrement())
name String
email String @
unique
profile Profile?
}
model Profile {
id Int @id @default(autoincrement())
bio String
user User @relation(fields: [userId], references: [id])
userId Int
}
One-to-Many Relationships
One-to-many relationships are useful when one entity is associated with multiple instances of another entity. The User
and Post
relationship mentioned earlier is an example of a one-to-many relationship.
Many-to-Many Relationships
Many-to-many relationships are common in database design and often require an intermediary table to represent the relationship. Prisma can handle many-to-many relationships seamlessly.
For example, let's say you have a User
entity and a Role
entity, and you want to represent a many-to-many relationship between them. You can define it as follows:
model User {
id Int @id @default(autoincrement())
name String
roles Role[]
@@manyToMany(Role, 'UserRole', 'userId', 'roleId')
}
model Role {
id Int @id @default(autoincrement())
name String
users User[]
@@manyToMany(User, 'UserRole', 'roleId', 'userId')
}
model UserRole {
id Int @id @default(autoincrement())
userId Int
roleId Int
user User @relation(fields: [userId], references: [id])
role Role @relation(fields: [roleId], references: [id])
@@unique([userId, roleId])
}
In this example, the User
and Role
models are related through the UserRole
intermediary table. Prisma will handle the complexity of many-to-many relationships transparently.
5. Advanced Prisma Features
Prisma offers several advanced features that go beyond basic CRUD operations and data modeling. These features enhance your ability to work with databases efficiently and effectively.
Transactions
Transactions are essential for ensuring the integrity and consistency of your database. Prisma supports database transactions, allowing you to group a series of database operations into a single transaction block. If any part of the transaction fails, the entire transaction can be rolled back.
Here's an example of how to use transactions with Prisma:
await prisma.$transaction([
prisma.user.create({
data: {
name: 'User A',
email: 'userA@example.com',
},
}),
prisma.user.create({
data: {
name: 'User B',
email: 'userB@example.com',
},
}),
]);
This code creates two user records within a single transaction. If either creation operation fails, both operations are rolled back.
Raw SQL Queries
While Prisma provides a high-level query builder, there are scenarios where you may need to execute raw SQL queries. Prisma allows you to run raw SQL queries and map the results to your data models.
const users = await prisma.$queryRaw`SELECT * FROM User WHERE age > 25`;
Using raw SQL queries should be done with caution, as it bypasses some of Prisma's type safety and may pose security risks if not properly sanitized.
Database Migrations
Database schema changes and updates are common in application development. Prisma includes a migration system that allows you to evolve your database schema while preserving existing data.
With Prisma migrations, you can:
Add new tables, fields, or relationships to your database schema.
Modify existing fields and constraints.
Handle data migrations when changing data models.
Prisma migrations are an essential tool for maintaining your database schema as your application evolves.
6. Query Performance and Optimization
Query performance is crucial for applications with large and complex datasets. Prisma offers features and best practices to optimize database queries.
Query Performance Tips
To improve query performance with Prisma:
Ensure that your database schema is well-designed, with appropriate indexes and constraints.
Use Prisma's query methods to filter and paginate results efficiently.
Consider caching frequently accessed data to reduce the load on the database.
N+1 Query Problem
The N+1 query problem is a common issue in database-driven applications where a query for a list of items results in an additional query for each item. Prisma provides ways to address this problem, such as using the include
keyword to eagerly load related records:
const usersWithPosts = await prisma.user.findMany({
include: {
posts: true,
},
});
This approach reduces the number of database queries and improves query performance.
Use of Indexes
Indexing is essential for optimizing database queries. Prisma allows you to
define indexes in your data model to speed up queries. For example, you can define an index on an email field to improve the performance of email-based lookups.
model User {
id Int @id @default(autoincrement())
name String
email String @unique @map("idx_email") // Define an index
}
By defining indexes where appropriate, you can significantly enhance the speed of database queries.
7. Security and Authentication
Security is a paramount concern when dealing with databases. Prisma offers features and best practices to enhance the security of your database interactions.
Securing Database Connections
When connecting to databases, it's crucial to secure the connections. Prisma supports secure connections to databases using protocols such as SSL/TLS. Ensure that your database configuration includes the necessary security measures.
User Authentication and Authorization
Prisma integrates with authentication and authorization systems to control user access to data. By implementing user authentication, you can ensure that only authorized users can perform actions on specific data.
Prisma provides fine-grained control over who can access and manipulate data, making it a valuable tool for implementing secure database interactions.
8. Scaling and Deployment
As your application grows, you may need to scale and deploy your database and Prisma servers. Prisma offers considerations and best practices for these scenarios.
Scaling Prisma Servers
To handle increased traffic and data loads, you can scale your Prisma servers horizontally. Load balancing and containerization technologies can help distribute requests across multiple Prisma server instances.
Prisma also provides the ability to configure connection pools to manage database connections efficiently.
Deployment Considerations
When deploying applications that use Prisma, consider factors like the following:
Environment Variables: Use environment variables to store sensitive information, such as database connection strings and authentication keys.
Database Backups: Implement regular database backups and recovery procedures to safeguard your data.
Monitoring and Logging: Set up monitoring and logging to detect and diagnose issues in your application and database.
Distributed Databases: In high-availability scenarios, you may need to consider distributed databases and data replication.
Proper deployment practices are essential for maintaining the reliability and performance of your application.
9. Best Practices
Adhering to best practices is critical for efficient and secure database management with Prisma. Here are some recommendations:
Code Organization
Organize your codebase with a clear structure that separates data access, business logic, and presentation layers. Use models and data access functions to encapsulate database interactions.
Error Handling
Implement error handling and graceful failure mechanisms to handle unexpected issues when interacting with the database. Prisma provides error information that can be used for effective error handling.
Testing with Prisma
Include database testing in your application's test suite. Prisma offers a testing framework that allows you to write tests that interact with a test database. This ensures that your database-related code functions correctly.
10. Community and Resources
Prisma has a vibrant community and offers various resources to help developers on their journey with database management.
Official Prisma Documentation
The official Prisma documentation is a comprehensive resource that covers all aspects of Prisma, from installation and setup to advanced topics. It includes tutorials, guides, and examples.
Prisma GitHub Repository
The Prisma GitHub repository is the place to explore the source code, report issues, and contribute to the development of Prisma.
Community Forums and Tutorials
Prisma has an active community with forums, discussion boards, and tutorials. Engaging with the community is an excellent way to seek help, share experiences, and learn from others.
11. Conclusion
In this guide, we've explored Prisma as an ORM tool for efficient and effective database management. We've covered the basics of ORM, Prisma's core features, data modeling, and advanced use cases. We've also discussed best practices, security, and deployment considerations.
Prisma simplifies database interactions, offers strong type safety, and supports various databases. Whether you're building a small application or a large-scale system, Prisma can enhance your database management and help you build robust, data-driven applications.
As you continue your journey with Prisma, remember that database management is a critical part of application development. It's essential to stay informed about best practices and emerging trends in the database management field.
Keep exploring, learning, and building, and make the most of Prisma's capabilities to create powerful and efficient applications. Good luck on your database management journey with Prisma!
Subscribe to my newsletter
Read articles from Ojoawo Joshua directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ojoawo Joshua
Ojoawo Joshua
As a skilled developer with expertise in a variety of frameworks and libraries, I am passionate about crafting high-quality software solutions. My primary framework of choice is React, and I have extensive experience with React-related tools such as Next.js, React Native, Redux, and Redux Toolkit. I also have a strong foundation in TypeScript and JavaScript, which allows me to build robust and scalable web applications. When it comes to styling, I am well-versed in Tailwind CSS and Chakra UI, both of which help me create visually stunning and responsive user interfaces. I also have experience working with Chart.js, which enables me to create interactive and informative data visualizations. On the backend, I am proficient in Express, Node.js, and popular databases such as MongoDB and PostgreSQL. Additionally, I have experience with containerization tools like Docker, which allows me to create and deploy applications seamlessly. Throughout my career, I have demonstrated a strong commitment to technical excellence, consistently delivering outstanding results on a range of complex projects. With an eye for detail and a focus on clean, maintainable code, I have earned a reputation as a trusted technical expert and an effective problem-solver. In addition to my technical skills, I am also a talented technical writer, able to communicate complex concepts clearly and concisely to a range of audiences. Whether working independently or as part of a team, I am always focused on delivering results that exceed expectations and drive business success. If you're looking for a skilled developer with deep experience in React, Next.js, React Native, TypeScript, JavaScript, Express, MongoDB, PostgreSQL, Git, Node.js, Docker, Tailwind CSS, Chakra UI, Chart.js, Redux, and Redux Toolkit, I invite you to get in touch. I am confident that my expertise and experience make me an ideal candidate for any project.