Understanding JavaScript Data Types
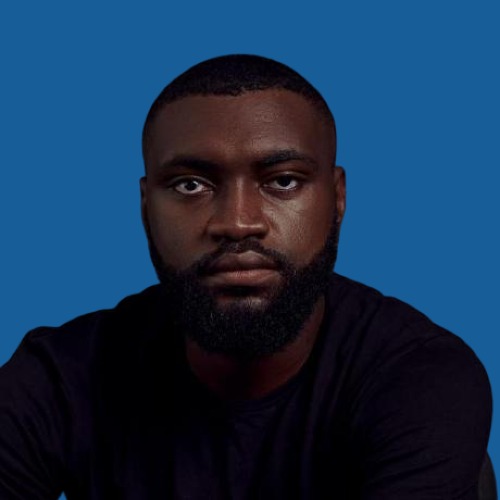
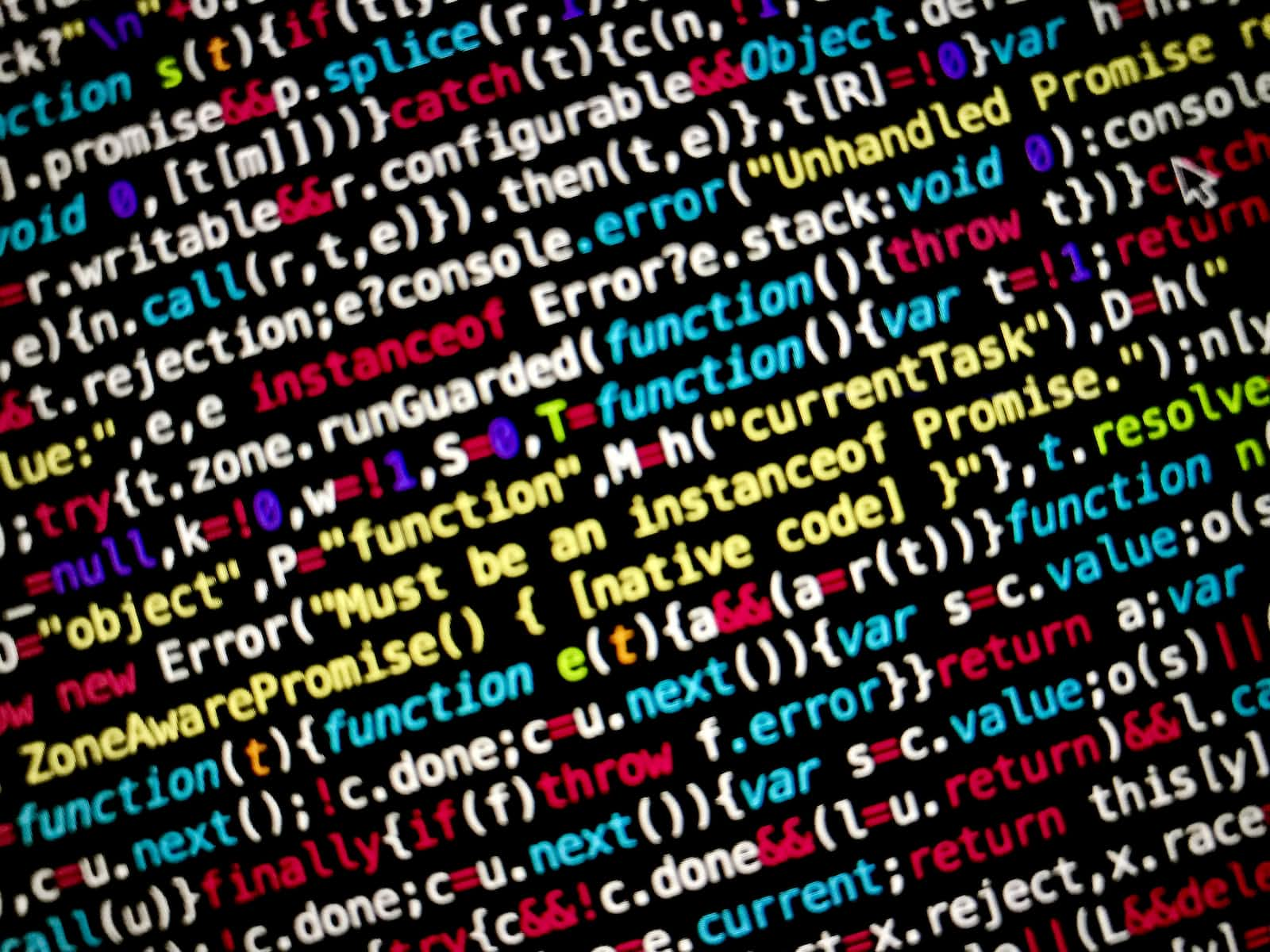
JavaScript is a dynamically typed programming language,
By dynamically typed, it means Javascript does not require variable types to be declared explicitly.
Hence, variable types are determined at runtime based on their values.
This characteristic provides great flexibility when using Javascript.
However, understanding Javascript's data types is key to writing efficient codes with the language.
Two Categories of Data Types in JavaScript
Primitive Data Types | Non-primitive Data Types (Reference) |
Number | Object |
String | Array |
Boolean | Date |
Null | Function |
BigInt | RegExp |
Undefined | Map and WeakMap |
Symbol | Set and WeakMap |
Primitive Data Types
Represent single values
Immutable (cannot be changed)
Stored directly in memory.
Non-Primitive Data Types
Represent collections of values or complex entities
Mutable (can be changed after they have been created)
Stored by reference.
Primitive Data Types
Primitive data types are the simplest data types representation in JavaScript. They are immutable, meaning that their values cannot be changed.
The six primitive data types include:
1. String
A string is a sequence of characters enclosed in single (' ') or double (" ") quotes.
For instance:
let greeting = "Hello, World!"
"Hello world" is a string data type assigned to the variable "greeting".
2. Number
Numbers in JavaScript can be integers (whole numbers) or floating-point numbers (numbers with decimal points).
For instance:
let whole_number = 42
let floating_number = 3.14
42 is an integer, and 3.14 is a floating point number. They both belong to the number data type.
3. BigInt
BigInt allows the representation of very large integers with arbitrary precision, avoiding potential rounding errors.
It is useful when precise calculations with large numbers are required, such as cryptography or scientific computations.
For instance:
//BigInt
const x = BigInt(Number.MAX_SAFE_INTEGER); //// 9007199254740991n
x + 2n === x + 3n; //false
// false because 9007199254740993n and 9007199254740994n are unequal
4. Boolean
A Boolean is a data type with two possible values: true
or false
.
They are fundamental for logical operations and decision-making in programming.
Booleans are pivotal for controlling program flow.
let isAwake = false
let isCold = true
5. undefined
‘undefined' indicates that a variable has been declared but not assigned a value yet.
For instance:
Let students;
console.log(students) //undefined
6. null
null
represents an intentional absence of any value or object.
For instance:
Let principal = null;
console.log(principal) // null
Note on undefined and null
7. Symbol
Symbols are unique, immutable values primarily used as object property keys to avoid unintended name collisions.
For instance:
//The instances create three new Symbols
const sym1 = Symbol();
const sym2 = Symbol("foo");
const sym3 = Symbol("foo");
//unique symbols
Symbol("foo") === Symbol("foo"); // false
Non-primitive Data Types
Non-primitive data types in JavaScript are more complex and can hold multiple values or functions.
Here are some common non-primitive data types:
1. Object:
Objects are collections of key-value pairs. They can hold various data types and even other objects.
Example:
let person = {
name: "John Doe",
age: 30,
isStudent: false,
address: {
street: "123 Main St",
city: "Exampleville"
}
};
2. Array:
Arrays are ordered collections of values accessed by an index. They can hold any data type, including other arrays or objects.
Example:
let items = ["red", "green", "blue", 1, false, {name: "Tony"}];
3. Function:
Functions are blocks of reusable code that perform a specific task. They can be assigned to variables, passed as arguments, and returned from other functions.
Example:
function sum(a, b) {
return a + b;
}
4. Date:
Date objects represent dates and times in JavaScript. They allow manipulation and formatting of dates.
Example:
let today = new Date();
5. RegExp (Regular Expression):
Regular expressions are patterns used for matching character combinations in strings. They enable powerful string manipulation.
Example:
let pattern = /^[a-zA-Z0-9]+$/
6. Map and WeakMap(ES6):
Maps are collections of key-value pairs that maintain the order of insertion. WeakMaps are similar but allow for garbage collection of keys.
Example:
let myMap = new Map();
myMap.set("name", "Alice");
console.log(myMap) //Map(1) {"name" => "Alice"}
7. Set and WeakSet (ES6):
Sets are collections of unique values, which can be of any type. WeakSets are similar but allow for garbage collection of values.
Example:
let mySet = new Set([1, 2, 3, 3, 4, 5]); // Only contains unique values
console.log(mySet) //[1,2,3,4,5]
Conclusion
Understanding data types in JavaScript is foundational to writing efficient and error-free code.
It enables precise manipulation of variables and prevents unexpected behavior.
Concepts such as strings, numbers, booleans, objects and arrays are key to building robust javascript applications.
Subscribe to my newsletter
Read articles from Daniel Ayeni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
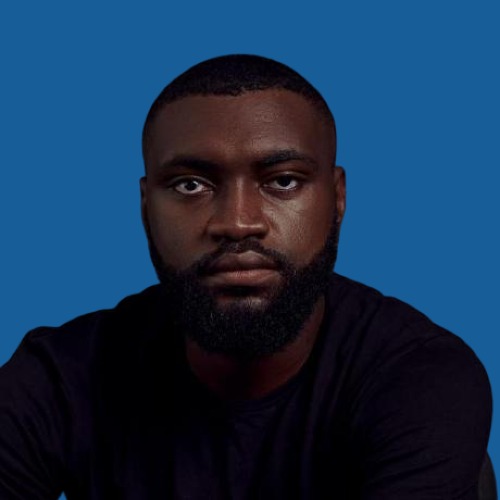
Daniel Ayeni
Daniel Ayeni
I am Daniel Ayeni, also known as Danthesage. A full-stack developer versed in using modern technologies to develop user-friendly web and mobile applications that solve real-life problems.