Encrypting and Decrypting Data with Fernet in Python
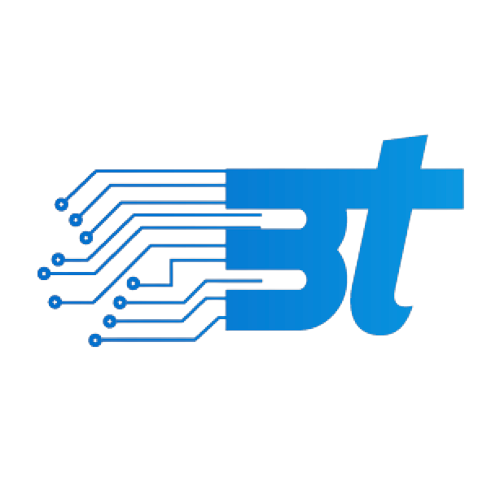
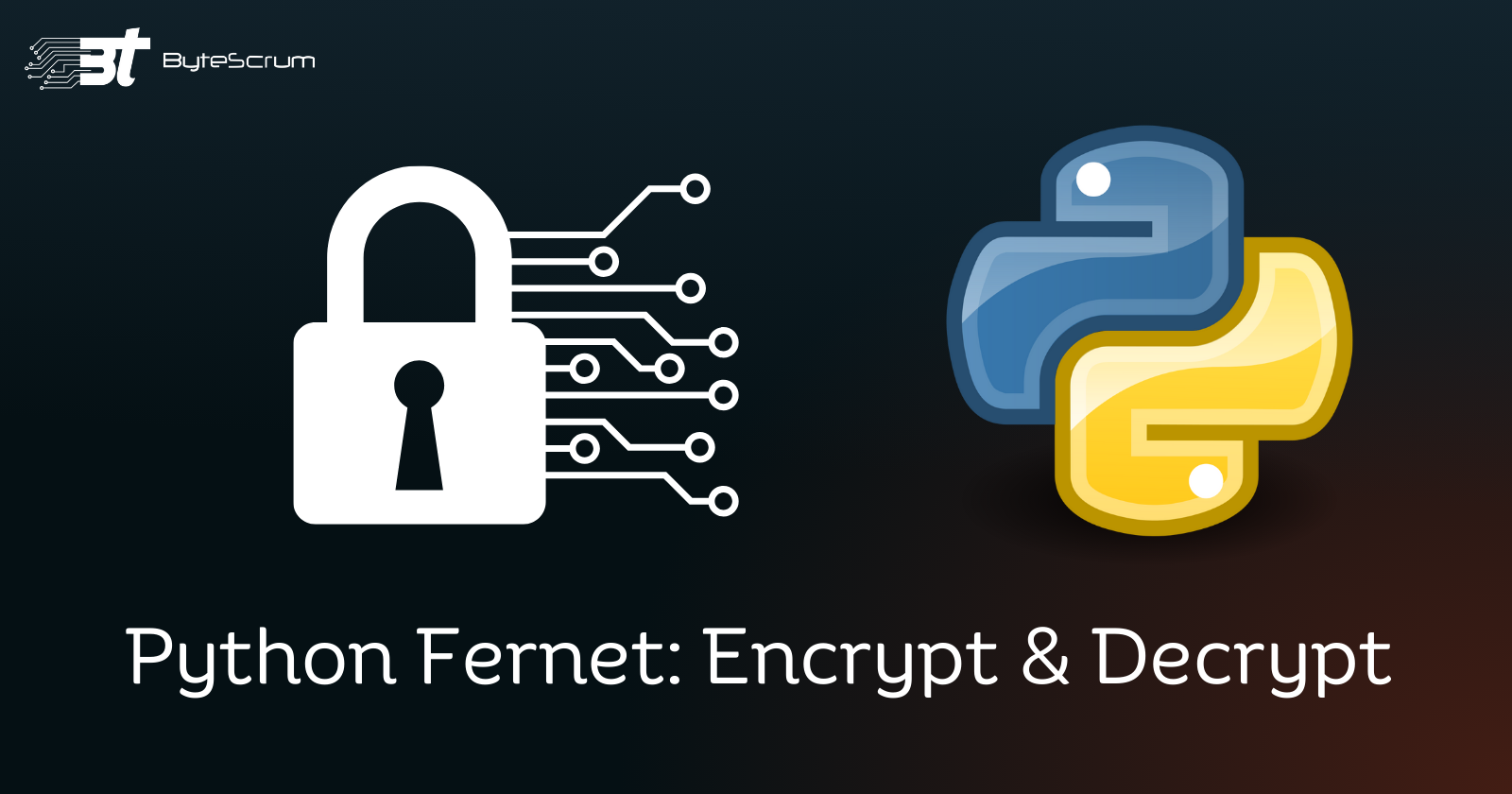
Data security is a critical concern in today's digital age. Whether you're storing sensitive information, sharing data over the internet, or protecting your files, encryption is a fundamental tool for safeguarding your data. In this blog post, we'll explore how to use the Fernet encryption method in Python to encrypt and decrypt data. Fernet provides a simple and secure way to protect your information.
What is Fernet Encryption?
Fernet is a symmetric-key encryption method, which means that the same key is used for both encryption and decryption. It is part of the cryptography library in Python and is designed to be easy to use while providing strong security. Fernet encryption offers the following advantages:
Simplicity: Fernet simplifies encryption and decryption processes, making it accessible to developers with varying levels of expertise.
Security: Fernet uses the Advanced Encryption Standard (AES) in Cipher Block Chaining (CBC) mode and employs a Message Authentication Code (MAC) to ensure data integrity and authenticity.
Speed: Fernet encryption and decryption are fast, which is crucial for real-time or near-real-time applications.
To get started with Fernet encryption in Python, you'll need to install the cryptography
library. You can do this using pip
, the Python package manager:
pip install cryptography
Let's dive into the Python code to see how Fernet can be used to protect your data.
Using Fernet Encryption in Python
Generating a Unique Encryption Key
To get started with Fernet encryption, you need a secret key. This key is crucial, as it's used for both encryption and decryption. Here's how you can generate a unique encryption key:
from cryptography.fernet import Fernet
from getpass import getpass
def generate_key(secret_key):
return Fernet.generate_key() + secret_key.encode()
In the generate_key
function, we use the Fernet.generate_key()
method to create a random encryption key. This key is then combined with a secret key by encoding the secret key as bytes and concatenating it to the generated key.
Encrypting Data
Once you have your encryption key, you can use it to encrypt your data. Here's a function for encrypting data with Fernet:
def encrypt_data(key, data):
f = Fernet(key)
encrypted_data = f.encrypt(data.encode())
return encrypted_data
In this function, we create a Fernet
object that uses the encryption key and then uses the encrypt
method to encrypt the data. The result of this encryption process is in bytes format.
Decrypting Data
When you need to access the original data, you can use the same encryption key to decrypt it. Here's a function for decrypting data:
def decrypt_data(key, encrypted_data):
f = Fernet(key)
decrypted_data = f.decrypt(encrypted_data).decode()
return decrypted_data
The decrypt_data
function takes the encryption key and the encrypted data as inputs creating a Fernet
object using the key, and then decrypts the data using the decrypt
method. The result is converted from bytes to a string.
Storing and Retrieving Encrypted Data
To save your encrypted data, you can use the following functions:
- Storing Encrypted Data:
def store_data(filename, encrypted_data):
with open(filename, "wb") as file:
file.write(encrypted_data)
This function writes the encrypted data to a specified file in binary mode.
- Reading Encrypted Data:
def read_data(filename):
with open(filename, "rb") as file:
encrypted_data = file.read()
return encrypted_data
The read_data
function reads the encrypted data from a specified file in binary mode and returns it as bytes.
Managing the Secret Key
It's crucial to keep your secret key secure. In the code below, we read the secret key from a file named "enc_key.txt":
# Read the secret key from the file
with open("enc_key.txt", "r") as file:
secret_key = file.read().strip()
You should store your secret key in a safe and restricted location, and it should not be shared or exposed to unauthorized individuals.
Summary
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
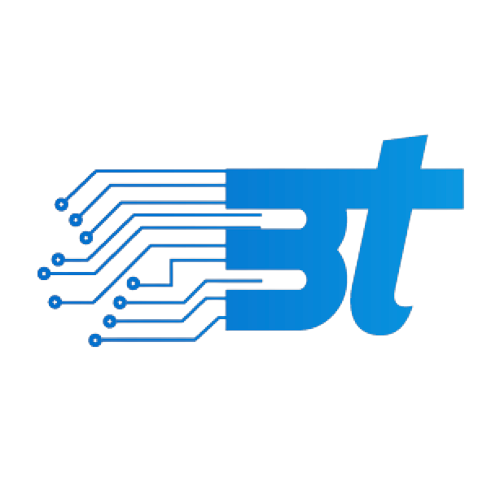
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.