PHP: MySQL Database
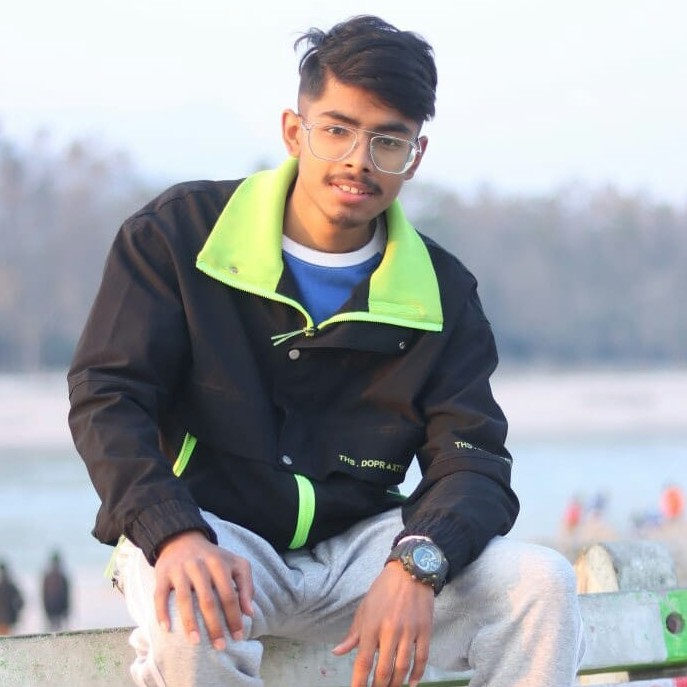
Table of contents
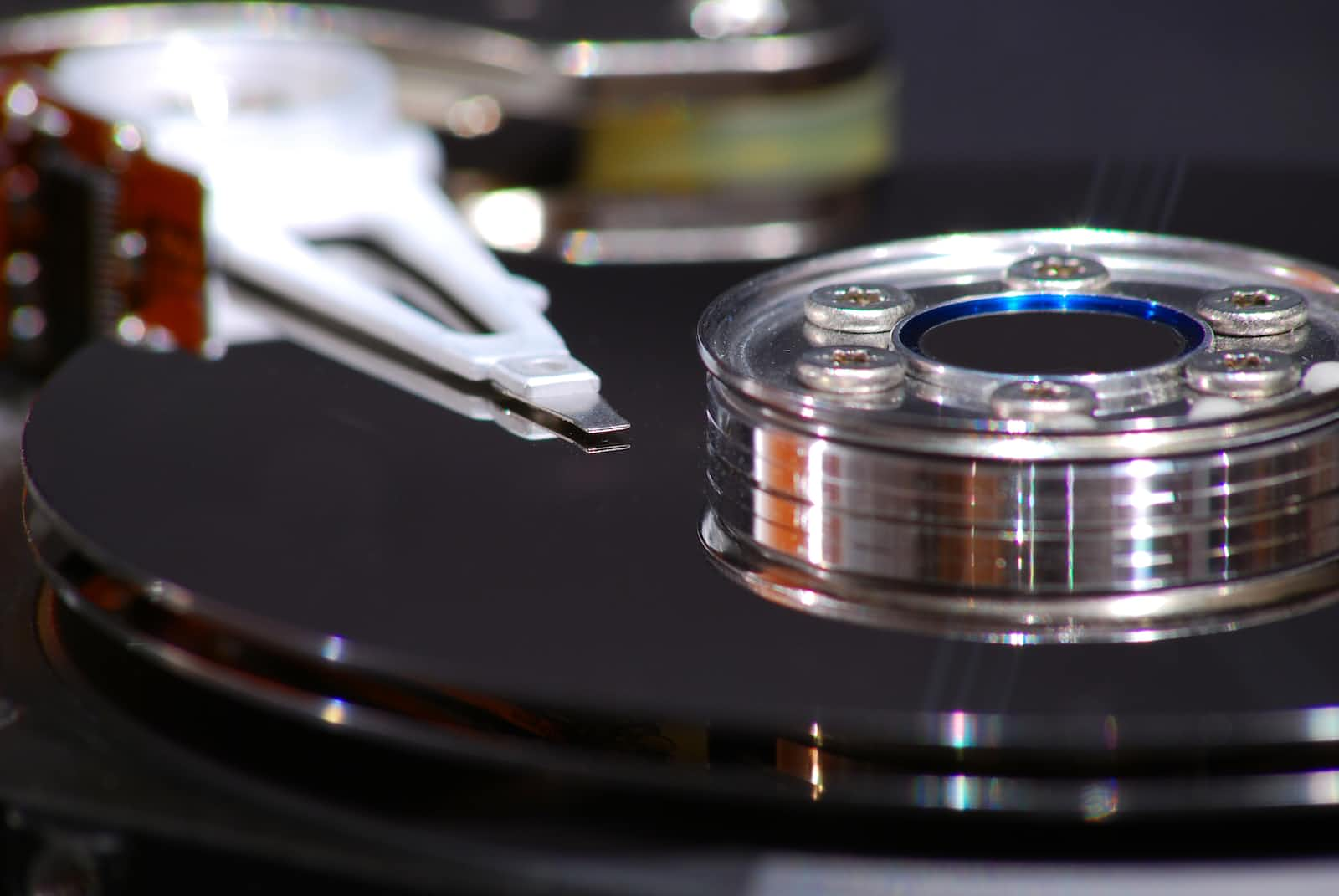
MySQL
It is an open-source relational database system where we can store data in the form of tables. To use this database with PHP, we need to install MySQL but if you have XAMPP installed, MySQL already comes with it. To work with MySQL in PHP:
We need to get connected with the MySQL server.
Execute our SQL queries to interact with the database.
The result can then be fetched and displayed in the browser.
Connection
To connect with the server using Object Oriented method, we use:
mysqli(host, username, password, database, port, socket)
$conn = new mysqli("localhost", "root", "", "form_db");
// Error Handling
if($conn->connect_error) echo "Connection Failed";
else echo "Connection Success. ";
Execute Query
Once the connection is established, the SQL query can be executed and the result can be stored in a variable.
// Write a SQL query
$sql = "INSERT INTO pizza(email, title, ingredient) VALUES('youremail@gmail.com', 'Pizza', 'Cheese')";
// Execute SQL query using above '$conn' object
$result = $conn->query($sql);
// Error Handling
if($result) "Insertion Successful";
else echo "Insetion Failed!";
Display Result
To display the query result in the browser, we use a 'fetch_assoc()' method which returns the next row of the table as an associative array every time it is called. It returns 'FALSE' if no rows are left to be fetched. The 'pizza' table is shown below:
// Display attributes of each row
while($row = $result->fetch_assoc())
{
echo "<br>";
echo $row['email'];
echo $row['title'];
echo $row['ingredient'];
echo "<br>";
}
Likewise, we can use other SQL queries to perform CRUD(Create, Read, Update and Delete) operations and get that result shown to the user.
Subscribe to my newsletter
Read articles from Giver Kdk directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
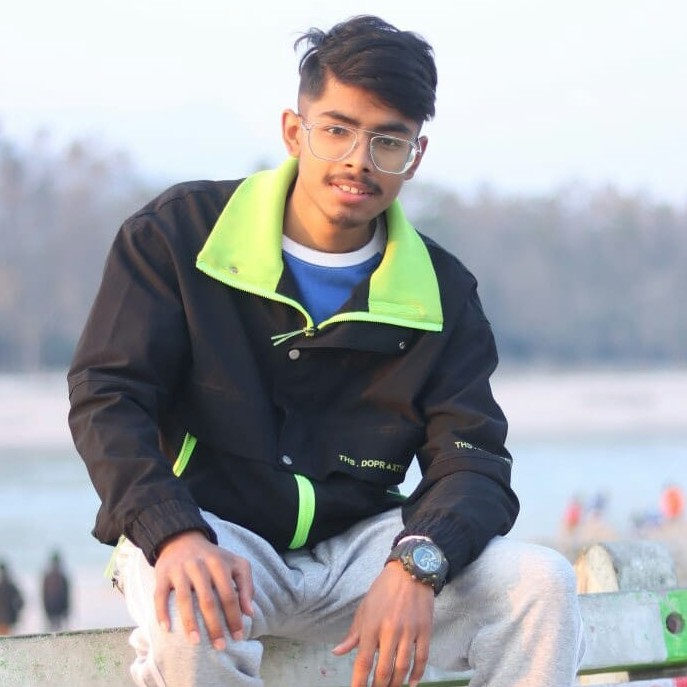
Giver Kdk
Giver Kdk
I am a guy who loves building logic.