Navigating Go's Core: Syntax, Variables, and Data Types Demystified
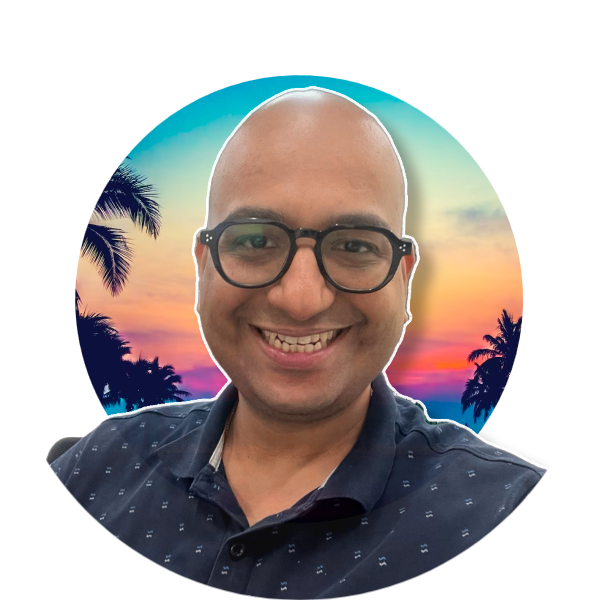
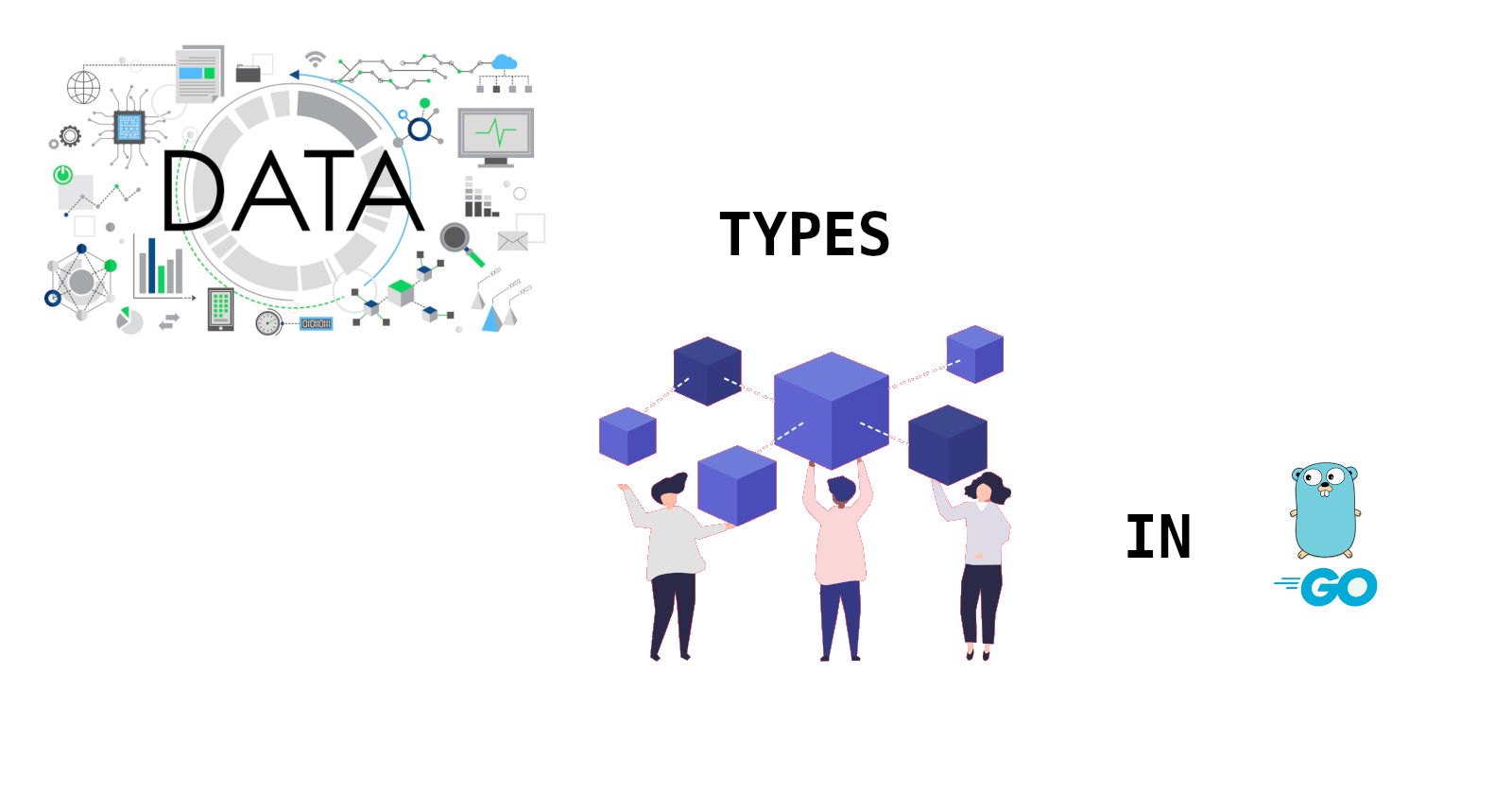
Introduction
Welcome to the next stage of your Go journey! In this guide, we'll unravel the fundamental aspects of Go programming, including its syntax, variables, and data types. Understanding these building blocks is essential as they form the foundation for crafting robust Go applications. Whether you're a Python expert or new to programming, Go's simplicity and power will captivate you.
Simpler explanation
Think of Go as a Swiss Army knife for coding. It provides clear rules for writing code, allows you to store information in labeled boxes (variables), and offers various types of containers (data types) to suit different needs.
Let's look at some example code
Basic Syntax and Variables:
package main
import "fmt"
func main() {
// Declare and initialize a variable
message := "string init in variable with the := walrus operator"
// Print the message
fmt.Println(message)
}
Data Types Exploration:
package main
import "fmt"
func main() {
// Declare variables with different data types
var num1 int = 42
var num2 float64 = 3.14
var isTrue bool = true
var name string = "Gopher"
// Print variables
fmt.Printf("Integer: %d\n", num1)
fmt.Printf("Float: %.2f\n", num2)
fmt.Printf("Boolean: %v\n", isTrue)
fmt.Printf("String: %s\n", name)
}
We declare and initialize a variable called
message
.Then, we print the value of
message
.
In the second example, we explore different data types (int, float64, bool, and string) and print them with formatting.
Reference Links
Conclusion
You've now embarked on a voyage into the heart of Go's syntax, variables, and data types. These essential concepts are the building blocks of any Go program. Armed with this knowledge, you're better equipped to create, manipulate, and organize data in your Go applications. Keep honing your skills, and soon, you'll be crafting efficient and powerful Go code like a true Gopher!
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
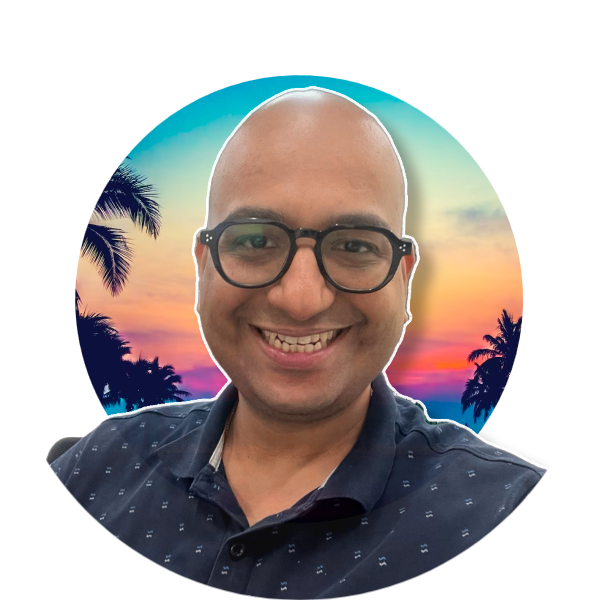
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.