Improving Angular's Performance by Implementing Virtual Scrolling on MatTable.
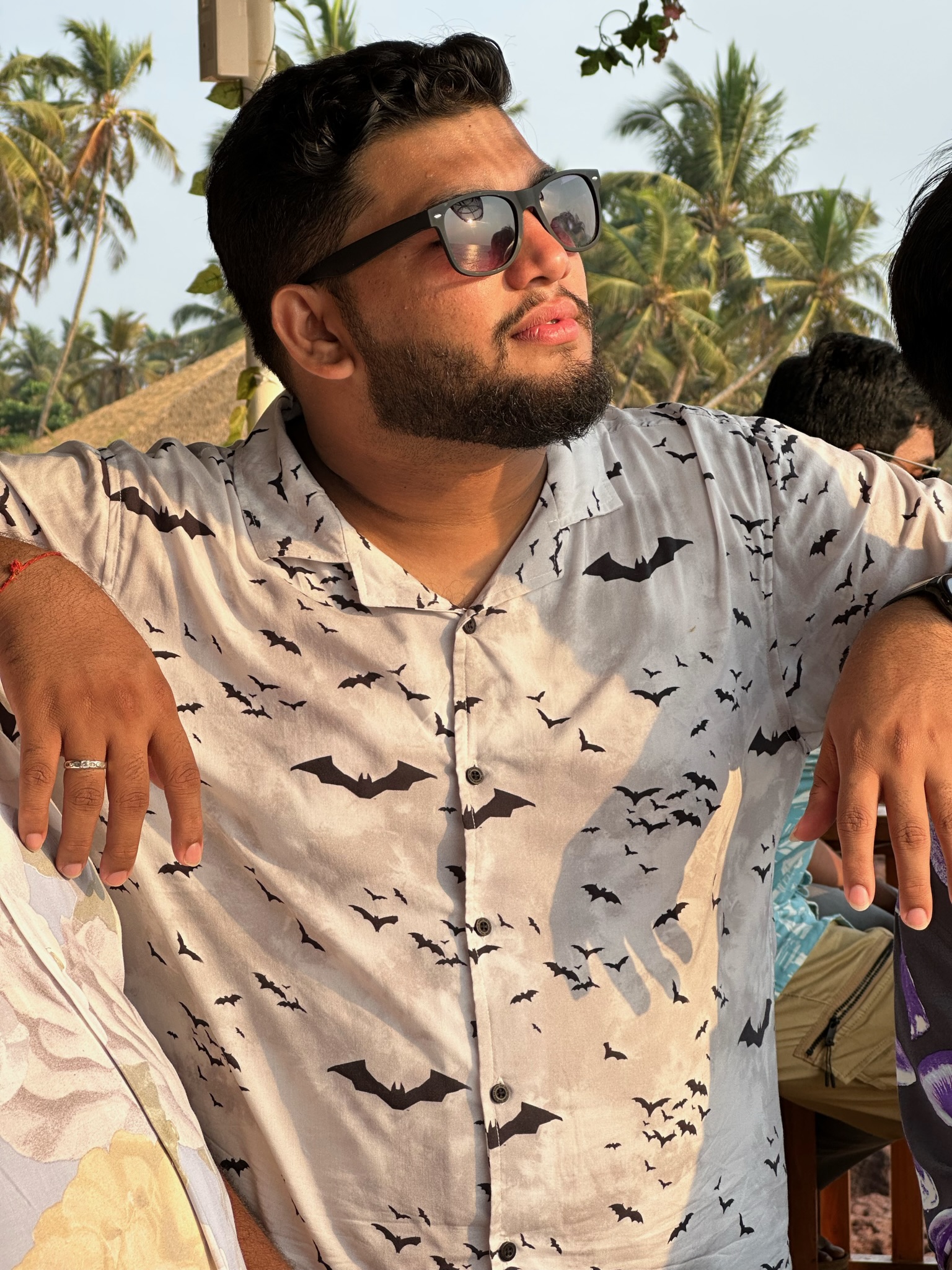
Table of contents
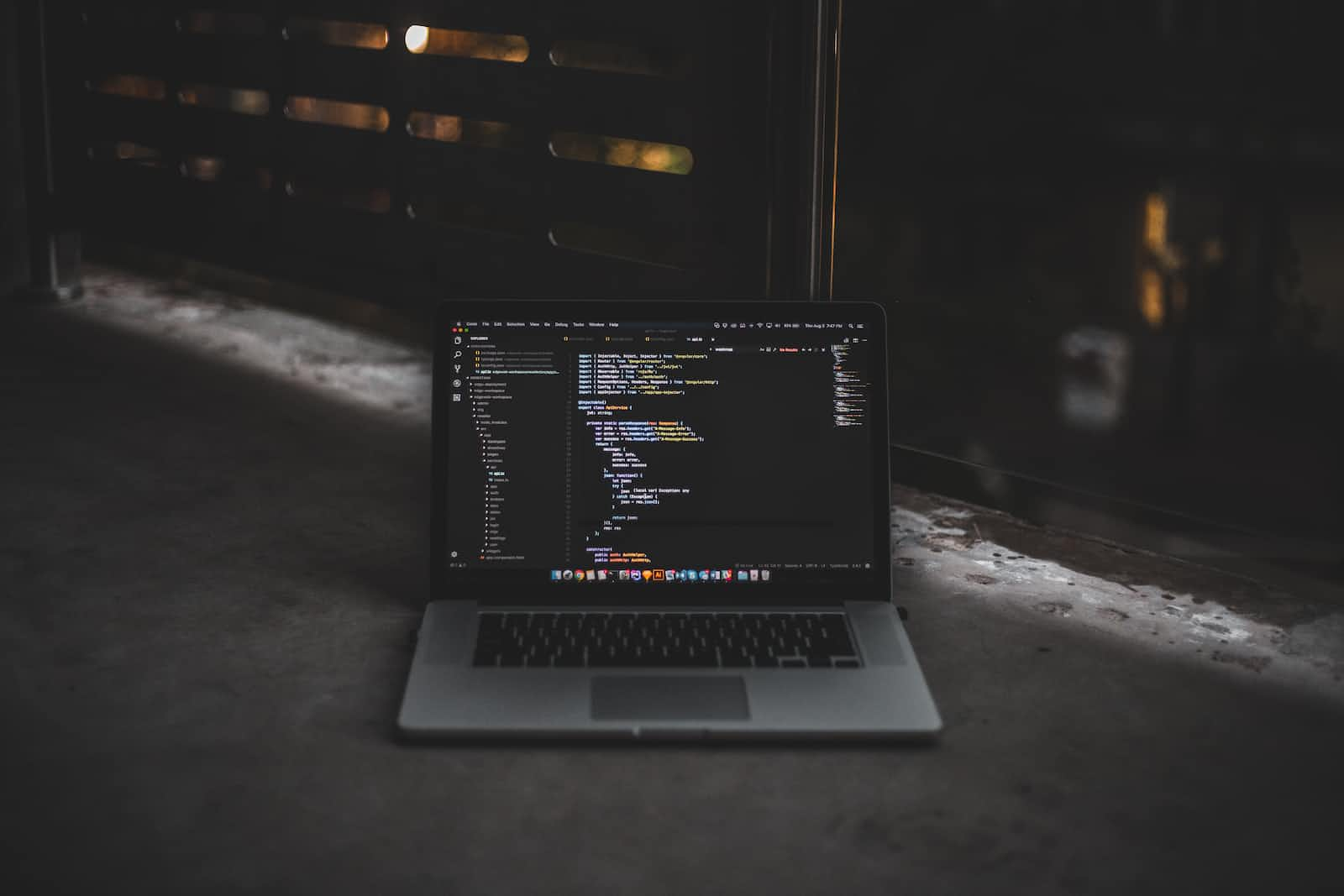
Virtual Scroll is one of the most commonly employed concepts for enhancing Angular performance. Let’s explore how we can harness its power with a MatTable.
Installation:
Make Sure you are running your application on Angular 9.1.13 or Above.
Npm:
npm install mat-table-virtual-scroll
Setup:
Include TableModule in your application’s app.module.ts.
import { TableModule } from "mat-table-virtual-scroll";
@NgModule({
imports: [MaterialModule, CommonModule, RouterModule, TableModule],
declarations: components,
exports: [TableModule, MaterialModule],
})
export class ComponentsModule {}
Create a dataset of over 10,000 records in your app.component.ts.
import { Component } from '@angular/core';
import { ColumnDef } from 'mat-table-virtual-scroll';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
title = 'mat-table-virtual-scroll';
name = 'Angular';
rows = Array(10000)
.fill(0)
.map((x, i) => {
return { name: 'name' + i, id: i, age: 27 };
});
columns: ColumnDef[] = [
{ field: 'name', title: 'Name' },
{ field: 'id', title: 'Id' },
{ field: 'age', title: 'Age' },
];
}
The ‘rows’ will be an array of JSON objects
The ‘columns’ will be an array of JSON objects.
The ‘field’ will be the key extracted from the ‘rows’ JSON array.
The ‘title’ will serve as the header for the columns.
Integrate ‘mat-virtual-table’ into your app.component.html.
<mat-virtual-table [rows]="rows" [columnsDef]="columns"></mat-virtual-table>
Enhancing column customization can be achieved by using ng-templates.
<mat-virtual-table [rows]="rows" [columnsDef]="columns">
<ng-template pCellDef column="name" let-row="row">
<b>{{row.name}}</b>
</ng-template>
</mat-virtual-table>
You can hide rows based on specific conditions.
<mat-virtual-table [rows]="rows" [columnsDef]="columns" [hiddenData]="name" [hiddenValue]="name0"></mat-virtual-table>
StackBlitz Code:
https://stackblitz.com/edit/stackblitz-starters-thc9p2?file=src%2Fapp%2Fapp.component.ts
Thanks for reading. 🚀
Subscribe to my newsletter
Read articles from Dhiraj Shetty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
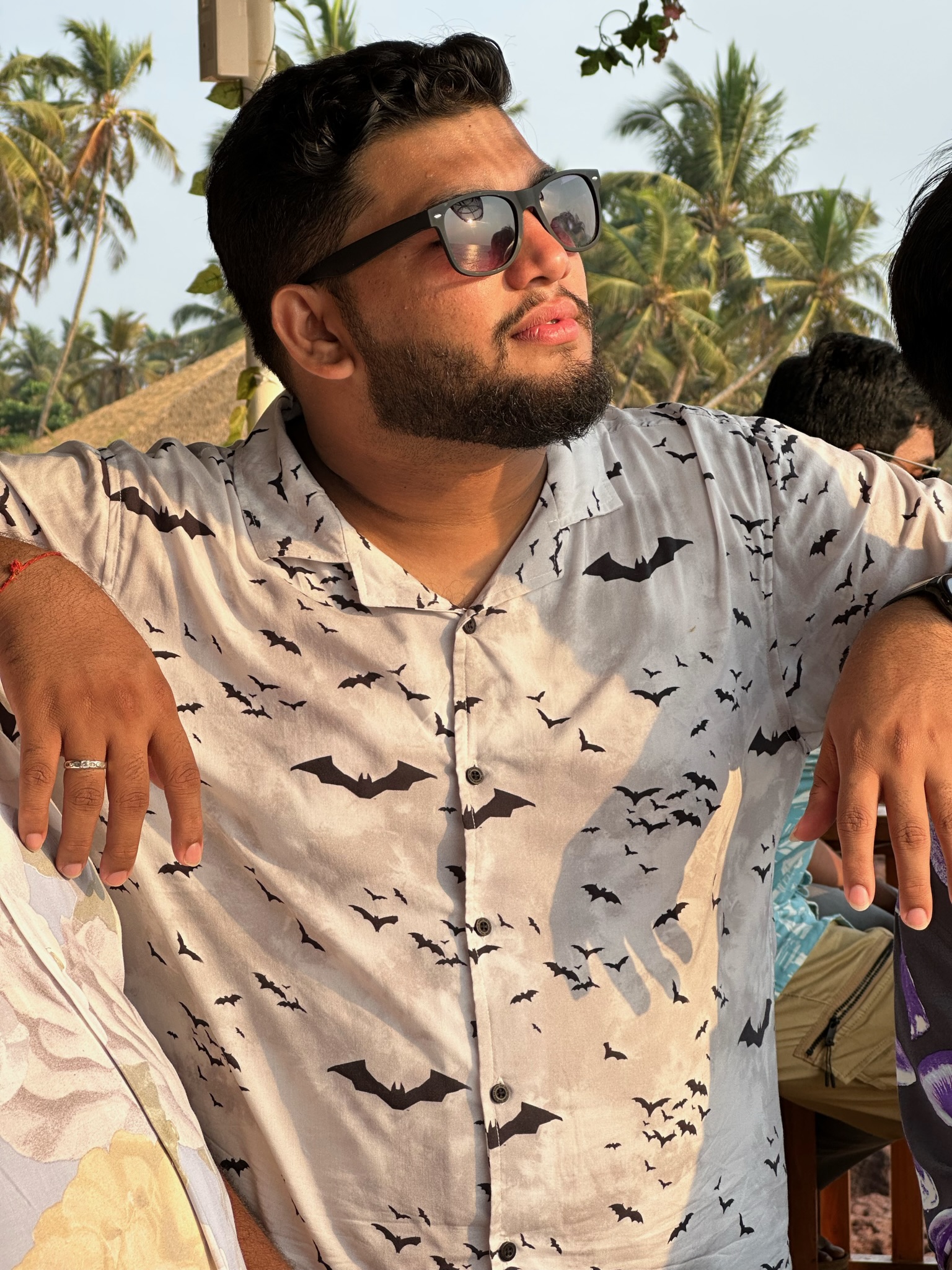
Dhiraj Shetty
Dhiraj Shetty
I am a Senior Product Engineer working on Angular Framework most of my days but love to explore other languages and explore world too.