Maven Interview Questions in 2023

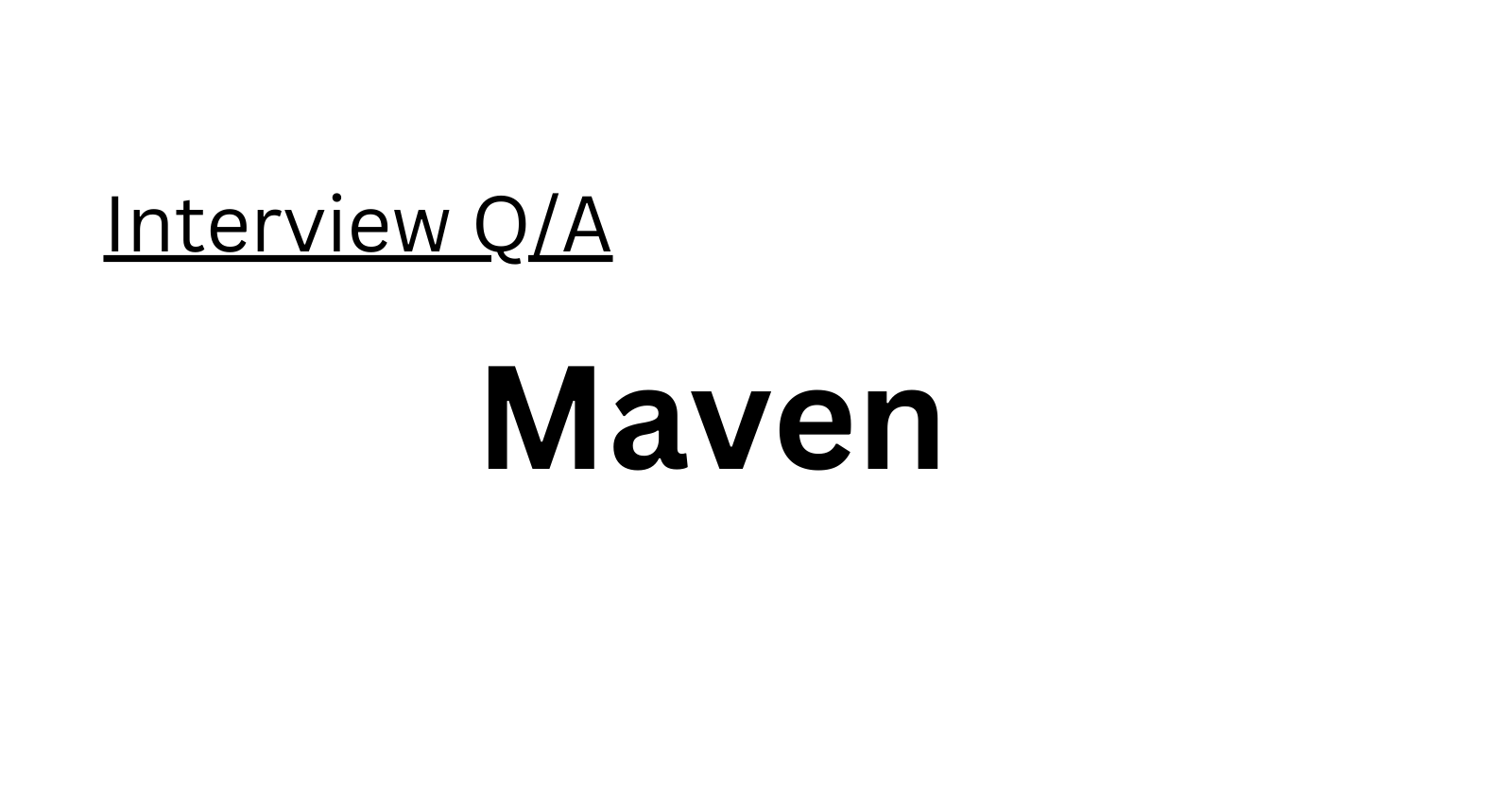
What is Maven?
Maven is a project management and comprehension tool used for automating a project's build infrastructure. It follows a standard directory structure and build lifecycle.
What are the main features of Maven?
Maven is known for its simplicity, speed, easy learning curve, dependency management, support for multiple projects, and a vast library of pre-built artifacts.
What does the build tool do?
A build tool in Maven generates source code, and documentation, compiles source code, packages compiled code into JAR or ZIP files, and installs the packaged code in various repositories.
Why should one use Maven?
Maven simplifies project setup, automates dependency management, and reduces the size of deployment files. It eliminates complex build scripts and uses the pom.xml file to manage project dependencies.
List the differences between ANT and Maven.
Ant is procedural, while Maven is declarative.
Ant lacks a build lifecycle, while Maven has one.
Ant is a toolbox, while Maven is a framework.
Ant scripts are not easily reusable, whereas Maven plugins are reusable.
Maven is generally preferred over Ant for project management.
Mention the steps for installing Maven on Windows.
Download Maven and extract it.
Set JAVA_HOME and MAVEN_HOME environment variables.
Add the environment path in the Maven variable.
Verify the Maven installation using the "mvn -version" command.
What does it mean when you say Maven uses Convention over Configuration?
Maven follows conventions, so developers don't need to specify detailed build configurations manually. It automatically generates the project structure, reducing the need for explicit configuration in the pom.xml file.
What are the aspects Maven manages?
Maven manages builds, documentation, reporting, dependencies, source code management (SCMs), releases, distribution, and mailing lists.
How do you know the version of Maven you are using?
To check the Maven version, use the command "mvn -version."
What is POM (Project Object Model)?
POM stands for Project Object Model, which is an XML file in the project's base directory. It contains information about the project and various configuration details used by Maven for building the project.
What information does POM contain?
POM contains configuration information such as project dependencies, plugins, goals, build profiles, project versions, developers, and mailing lists.
What is Maven's artifact?
An artifact is a file, often a JAR, deployed to a Maven repository. Each artifact has a group ID, artifact ID, and version, which uniquely identify it and specify its dependencies.
What is the Maven Build lifecycle?
The Maven Build lifecycle is a well-defined sequence of phases, each containing a sequence of goals. Execution of one lifecycle triggers all build phases in that lifecycle.
Name the three build lifecycles of Maven.
The three build lifecycles are "clean" (for cleaning artifacts), "default" (for building the application), and "site" (for generating project documentation).
What is the command to build your Maven site?
To build the Maven site, use the command "mvn site."
What would the command "mvn clean" do?
The command "mvn clean" deletes the target directory and all build data before starting the build process.
What are the different phases of a Maven Build Lifecycle?
The phases of a Maven Build Lifecycle include "validate," "compile," "test," "package," "integration-test," "verify," "install," and "deploy."
What is a goal in Maven terminology?
A goal represents a specific task in Maven that contributes to building and managing a project. Goals can be executed directly or bound to build phases.
What would the command "mvn clean dependency:copy-dependencies package" do?
This command will clean the project, copy the dependencies, and package the project, executing all phases up to the "package" phase.
What phases does a Clean Lifecycle consist of?
The Clean Lifecycle consists of the following phases: "pre-clean," "clean," and "post-clean."
What phases does a Site Lifecycle consist of?
The Site Lifecycle includes "pre-site," "site," "post-site," and "site-deploy" phases.
What is a Build Profile?
A Build Profile is used to customize Maven builds for different environments like Production and Development by specifying configuration values.
What are the different types of Build Profiles?
Build profiles can be per project (in the project pom.xml file), per-user (in the user's settings.xml file), or global (in the global settings.xml file).
How can you activate profiles?
Profiles can be activated explicitly using command console input, through Maven settings, based on environment variables, OS settings, or the presence/absence of files.
What is a Maven Repository?
A Maven Repository is a directory where project-specific artifacts like JARs, library JARs, and plugins are stored for use by Maven.
What types of Maven repositories exist?
Maven repositories include Local (on your machine), Central (provided by the Maven community), and Remote (custom repositories).
Can you tell me the default location of your local repository?
The default location of the local repository is "~/.m2/repository."
Tell me the command to install the JAR file in the local repository.
To install a JAR file in the local repository, use the command "mvn install."
Is there a particular sequence in which Maven searches for dependency libraries?
Maven searches for dependencies first in the local repository, then in the central repository, and, if needed, in remote repositories. It stops if a dependency is not found.
What are the uses of Maven Plugins?
Maven Plugins are used for tasks like creating JAR or WAR files, compiling code, unit testing, generating documentation, and creating project reports.
What are the types of Maven Plugins?
Maven provides build plugins that execute during the build and reporting plugins that execute during site generation.
When does Maven use the External Dependency concept?
Maven uses the External Dependency concept when a dependency is not found in remote or central repositories. It allows developers to specify custom dependencies.
What are the things that you must define for each external dependency?
For external dependencies, you must specify the group ID, artifact ID, scope (as "system"), and the system path relative to the project location.
What is Archetype?
An Archetype is a Maven plugin that creates a project structure based on a template.
What is the command to create a new project based on an archetype?
To create a new project based on an archetype, use the command "mvn archetype:generate."
What is SNAPSHOT in Maven?
SNAPSHOT is a special version in Maven indicating a current development copy. Maven checks for SNAPSHOT updates in the remote repository each time you build.
What is the difference between Snapshot and Version?
In the case of Version, Maven downloads the specified version and doesn't update it. For SNAPSHOT, Maven always fetches the latest SNAPSHOT version.
What is a transitive dependency in Maven?
A transitive dependency is a library that your project's dependencies require, and Maven includes it automatically, avoiding the need to specify it explicitly.
What does dependency management mean with respect to transitive dependency?
Dependency management involves specifying versions of artifacts used in transitive dependencies, giving control over which versions are used when referenced.
How does Maven handle multiple versions of an artifact?
If multiple versions of an artifact are found at the same depth in the dependency tree, Maven uses the first declared version, a process known as dependency mediation.
What is the dependency scope, and what are the dependency scopes?
Dependency scope indicates when a dependency is included during the build. The dependency scopes are "compile," "provided," "runtime," "test," "system," and "import."
What is the minimal set of information for matching dependency reference against a dependency management section?
The minimal set includes {groupId, artifactId, type, classifier}.
Is it possible to refer to a property defined in your pom.xml file?
Yes, properties defined in the pom.xml file can be referred to using the syntax ${pom.property_name}.
What are the default values for the packaging element? If there is no packaging element defined? What is the default value for that?
The default packaging values are "jar," "war," "ear," and "pom." If no packaging value is defined, the default is "jar."
What is the use of the execution element in the pom file?
The <execution> element contains information needed to execute a plugin during the build process.
What is a project’s fully qualified artifact name?
A project's fully qualified artifact name is in the format: <groupId>:<artifactId>:<version>.
If you fail to define any information, where does your pom inherit that information from?
All POMs inherit information from a base POM called the Super POM, which contains default values for Maven configurations.
How are profiles specified in Maven?
Profiles are specified in Maven by using elements within the POM file.
What are the elements in POM that a profile can freely modify when specified in the POM?
A profile can freely modify elements such as <repositories>, <pluginRepositories>, <dependencies>, <plugins>, <properties>, <modules>, <reporting>, <dependencyManagement>, and <distributionManagement>.
What are the benefits of storing JARS/external dependencies in the local repository instead of a remote one?
Storing JARS in the local repository saves storage and speeds up project checkout without the need to version JAR files.
What is a system dependency?
A system dependency is always available and is not looked up in repositories. It is used to specify dependencies provided by the JDK or VM.
What is the use of an optional dependency?
An optional dependency allows you to mark a transitive dependency as optional, indicating that it won't be used unless explicitly required.
What is dependency exclusion?
Dependency exclusion allows you to exclude a specific transitive dependency, preventing it from being included in your project.
How to run the clean plugin automatically during the build?
Place the clean plugin configuration within the <execution> tag in the pom.xml file.
What is the meaning of the message "You cannot have two plugin executions with the same or missing elements"?
This message indicates that you've defined multiple plugin executions with the same <id>. To resolve this, each <execution> should have a unique <id>.
Subscribe to my newsletter
Read articles from Amrutha D directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amrutha D
Amrutha D
Gen AI Video Creator | Gen AIOps | AIOps Cloud DevOps Engineer | Let's Connect & share Technical knowledge & grow together in Technologies Everyday..