Exploring Dependency Injection in Android with Dagger 2
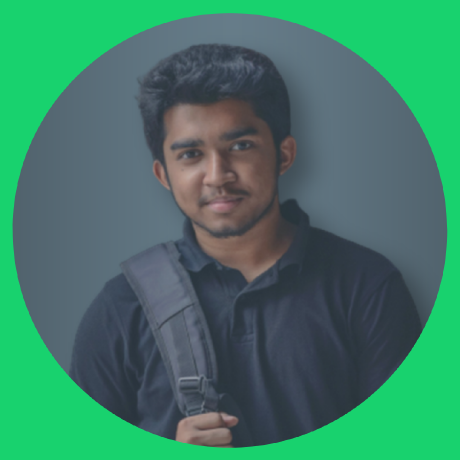
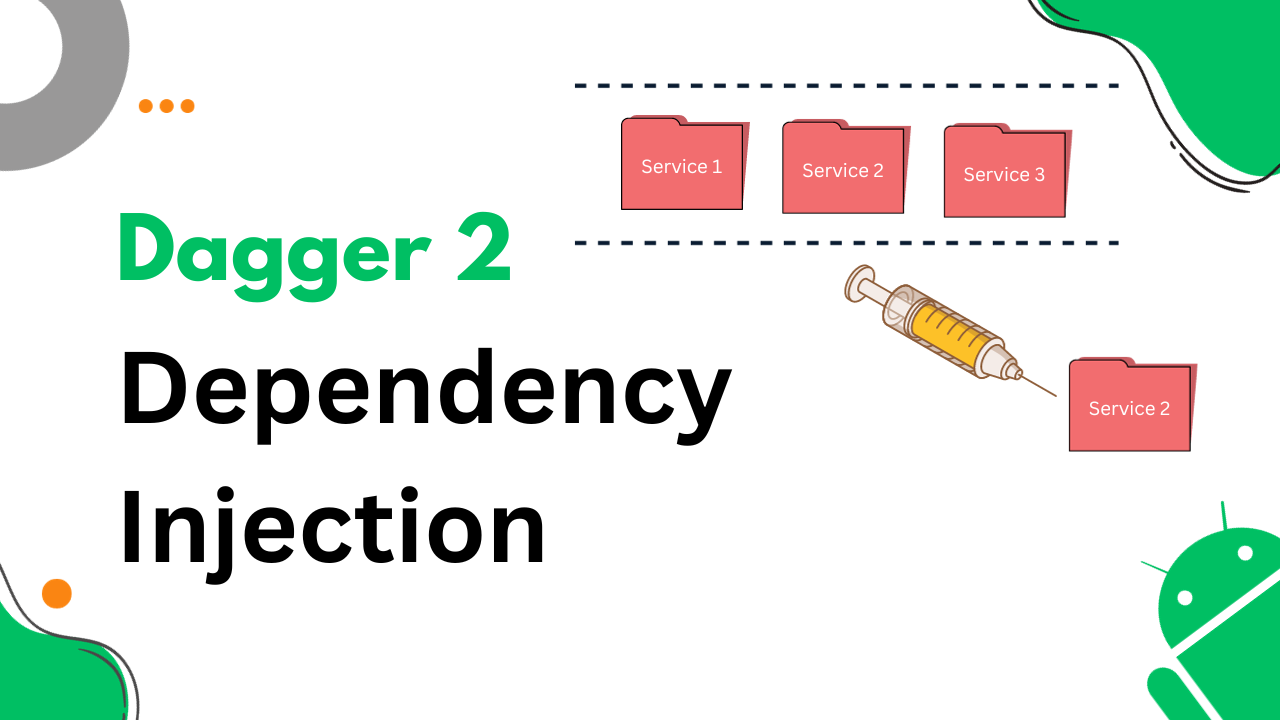
Dependency Injection (DI) is a crucial concept in software development that aids in achieving better code maintainability, testability, and scalability. When it comes to Android app development, Dagger 2 is a popular framework used to implement DI effectively. In this blog post, we'll walk through the process of integrating Dagger 2 into an Android application.
Step 1: Adding Dagger 2 to the Project
To begin, open your app-level build.gradle
file and add the Dagger 2 dependencies:
dependencies {
// Other dependencies...
implementation 'com.google.dagger:dagger:2.38.1'
annotationProcessor 'com.google.dagger:dagger-compiler:2.38.1'
}
Step 2: Creating a Module
A Dagger module is responsible for providing instances of the dependencies we want to inject. Let's create an AppModule
:
@Module
class AppModule {
@Provides
fun provideMyDependency(): MyDependency {
return MyDependency()
}
}
In this module, we define a method provideMyDependency()
annotated with @Provides
. This method provides an instance of MyDependency
, which we want to inject.
Step 3: Creating a Component
Next, let's create a Dagger component that ties the module and the injection target (our MainActivity
) together:
@Component(modules = [AppModule::class])
interface AppComponent {
fun inject(activity: MainActivity)
}
The AppComponent
interface is annotated with @Component
and specifies the modules it will use (AppModule
in this case). The inject()
method injects the dependencies into the specified target.
Step 4: Defining the Dependency
Now, let's define the MyDependency
class:
class MyDependency {
fun doSomething(): String {
return "Dependency is doing something!"
}
}
This class represents the dependency we'll be injecting into our MainActivity
.
Step 5: Using Dependency Injection in an Activity
In the MainActivity
, we'll use Dagger to inject the MyDependency
:
class MainActivity : AppCompatActivity() {
@Inject
lateinit var myDependency: MyDependency
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
DaggerAppComponent.builder()
.appModule(AppModule())
.build()
.inject(this)
val result = myDependency.doSomething()
// Use the result as needed
}
}
Here, we annotate myDependency
with @Inject
, indicating that Dagger should provide this dependency. In the onCreate()
method, we build the Dagger component and perform the injection.
Conclusion
Dagger 2 simplifies the implementation of Dependency Injection in Android apps. By following the steps outlined in this blog post, you can effectively use Dagger 2 to manage dependencies and write more maintainable, testable, and scalable Android applications. Happy coding! ๐
Subscribe to my newsletter
Read articles from Emran Khandaker Evan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
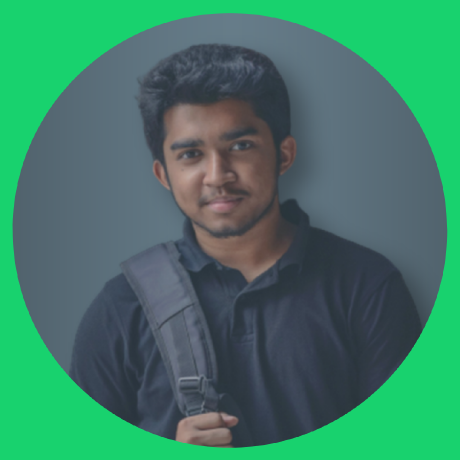
Emran Khandaker Evan
Emran Khandaker Evan
Software Engineer | Content Creator