PHP: File Handling
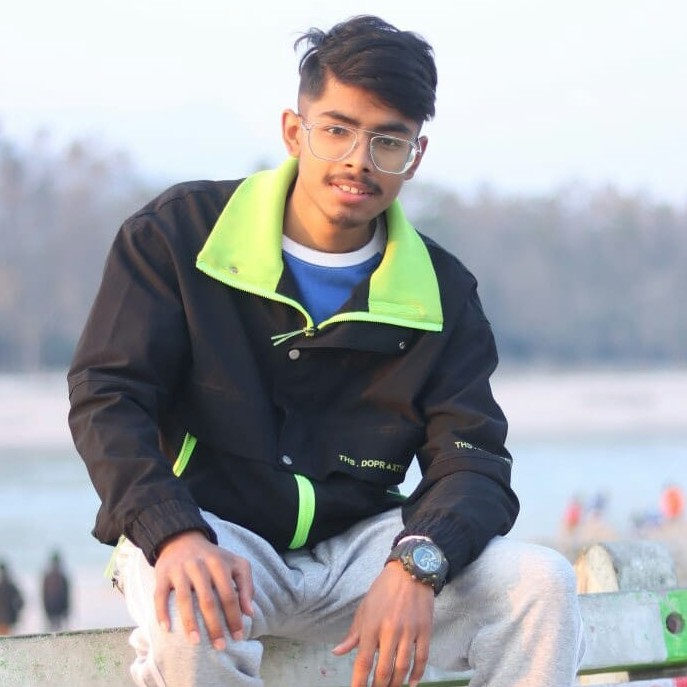
Table of contents
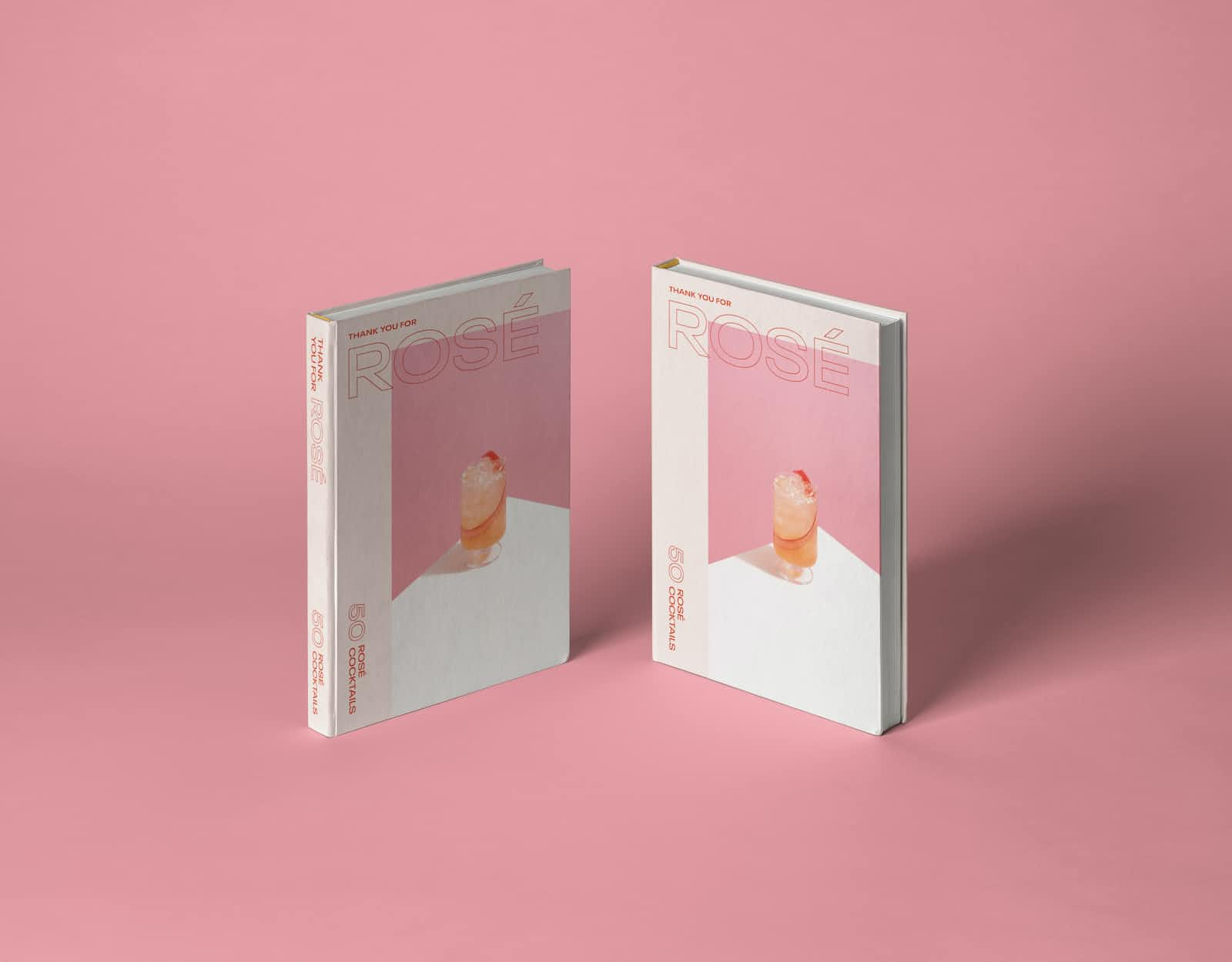
File Opening
We can perform CRUD operations in files using PHP which is similar to file handling in C-Program. To perform any read or write operation, we have to open the file first:
$fileName = "myFile.txt";
$handler = fopen($fileName, "r");
Here, the $handler now becomes the pointer to the file we want to work with. The argument "r" in the fopen() means read mode which only allows us to read the file and blocks us from writing into the file. We will discuss other modes in this article later on.
File Read
To read the file, we use fread():
// 'fread(file_pointer, size_of_file)'
$data = fread($handler, filesize($fileName));
// Prints file content as string
echo $data;
To read a single line of the file, we use fgets():
// 'fgets(file_pointer)'
echo fgets($handler);
To read a single character, we use fgetc():
echo fgetc($handler);
File Write
Now, to perform the write operation in the file we have to use fwrite() function while opening the file in write mode.
$fileName = "myFile.txt";
$handler = fopen($fileName, "w"); // Write Mode
fwrite($handler, "This is new content");
After we are done with all the file-handling tasks, we need to release the file pointer ($header) to free up CPU resources. For that we use fclose() method:
fclose($handler);
Modes
Till now we only discussed read and write modes but there are many other modes which are listed in the table below:
Modes | Functionality |
r | Allow read-only |
w | Allow write-only |
a | Allow append-only |
r+ | Allow read and write |
w+ | Allow read and write |
a+ | Allow read and append |
Subscribe to my newsletter
Read articles from Giver Kdk directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
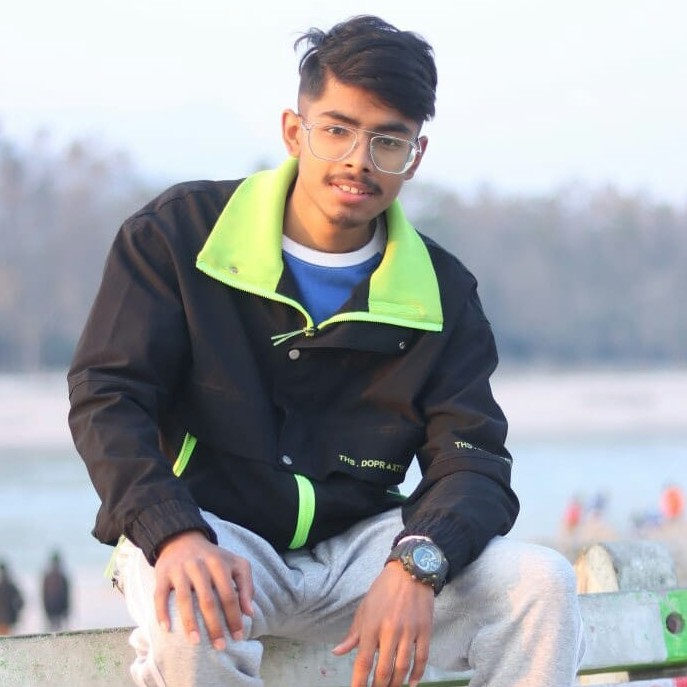
Giver Kdk
Giver Kdk
I am a guy who loves building logic.