Optimising React Component Rendering Performance: A Comprehensive Guide

Table of contents
- Understanding React's Rendering Process
- ShouldComponentUpdate Method
- PureComponent
- React.memo
- Identifying Expensive Operations
- Profiling React Components
- Asynchronous State Updates
- The Virtual DOM
- Leveraging the React DevTools
- React.StrictMode for Identifying Issues
- Implementing React.lazy and Suspense for Code Splitting
- Employing Server-Side Rendering (SSR) and Pre-rendering
- End-to-End Example: Optimizing a React Image Gallery
- Conclusion
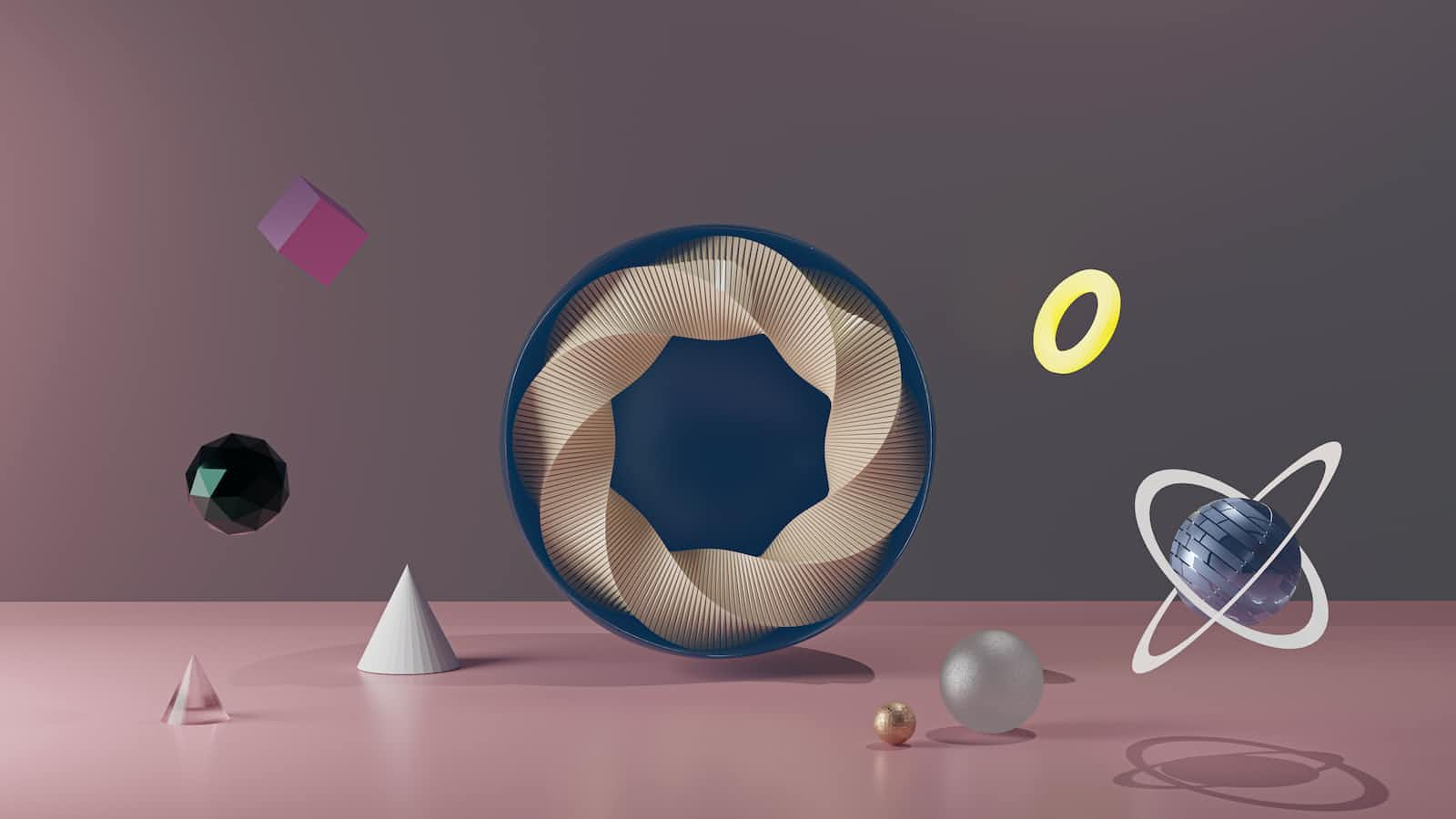
In the fast-paced world of web development, performance is key. Users expect responsive, smooth, and efficient web applications. In the realm of React, a popular JavaScript library for building user interfaces, optimizing component rendering performance is essential to meet these expectations. In this comprehensive guide, we'll explore techniques and best practices to help you fine-tune your React components for optimal performance.
Understanding React's Rendering Process
Before diving into optimization strategies, it's crucial to understand how React renders components. React uses a process called "reconciliation" to determine what changes need to be applied to the DOM. When something in the component's state or props changes, React re-renders the component and reconciles it with the previous version, updating the DOM as needed.
However, sometimes React re-renders components that don't actually need to be updated. This can result in unnecessary work for the browser and negatively impact performance. To address this issue, React offers a few key tools and techniques to optimize rendering.
ShouldComponentUpdate Method
The shouldComponentUpdate
method is a critical component lifecycle method that allows you to control whether or not a component should re-render when its state or props change. By default, this method returns true
, indicating that the component should always re-render. However, you can override it to implement your custom logic.
Example:
class MyComponent extends React.Component {
shouldComponentUpdate(nextProps, nextState) {
// Custom logic to determine if the component should update
return nextProps.someImportantProp !== this.props.someImportantProp;
}
// ... rest of your component
}
In this example, the shouldComponentUpdate
method is customized to re-render only when a specific prop (someImportantProp
) changes. This can significantly reduce unnecessary re-renders.
PureComponent
React's PureComponent
is a convenient alternative to manually implementing shouldComponentUpdate
. It automatically performs a shallow comparison of the new props and state with the previous ones and re-renders the component only if changes are detected.
Example:
class MyPureComponent extends React.PureComponent {
// ... your component
}
By using PureComponent
, you can reduce re-renders and improve performance without writing custom logic for shouldComponentUpdate
.
React.memo
For functional components, React provides the React.memo
higher-order component to achieve similar memoization of components.
Example:
const MemoizedComponent = React.memo((props) => {
// Your component logic here
});
By wrapping a functional component with React.memo
, React will memoize it, ensuring that it re-renders only when its props change. This can be a powerful tool for optimizing functional components.
Identifying Expensive Operations
Optimizing component rendering performance isn't just about preventing unnecessary re-renders; it also involves identifying and mitigating expensive operations within your components. Here are some common scenarios where you might encounter performance bottlenecks:
Excessive State Changes
Excessive state changes, especially within deeply nested components, can trigger unnecessary re-renders. Minimizing state changes by lifting state to higher-level components or using context can help mitigate this issue.
Example:
class PerformanceOptimizedComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
// ... large state object
};
}
// ... component logic
}
In this case, consider breaking down the state into smaller pieces or using context to manage the state more efficiently.
Inefficient Rendering Logic
Complex rendering logic within a component can lead to performance problems. Consider breaking down your component into smaller, more focused components. This not only makes your code more maintainable but can also improve performance.
Example:
class ComplexComponent extends React.Component {
// ... complex rendering logic
}
Splitting the logic into smaller components can lead to a more modular and optimized codebase.
Unnecessary Component Updates
Sometimes, components update even when their data hasn't changed. This can be caused by reference equality rather than value equality comparisons.
Example:
const data = [1, 2, 3];
const updatedData = data; // This creates a reference, not a new array
class UnoptimizedComponent extends React.Component {
state = {
data: data,
};
// ... component logic
}
In this case, even if the data
array remains the same in terms of values, React might trigger re-renders due to reference equality. To avoid this, create new instances when updating the data.
Profiling React Components
React comes with built-in tools to profile and analyze your component's performance. React's Profiler can help you identify bottlenecks and rendering issues within your app. To use the Profiler, you can wrap your application with a Profiler
component and pass a callback function that collects and analyzes performance data.
Example:
import { Profiler } from 'react';
<Profiler id="myComponent" onRender={onRenderCallback}>
<MyComponent />
</Profiler>
In the onRenderCallback
function, you can log and analyze performance data, including component render times and updates.
Asynchronous State Updates
React batches state updates, which can be advantageous for performance. However, sometimes you might need to perform multiple state updates sequentially and ensure that they're processed immediately. You can use the setState
function's callback to accomplish this.
Example:
this.setState({ count: this.state.count + 1 }, () => {
// This code will run after the state update is processed
console.log('State has been updated.');
});
By using the callback, you can be sure that the state has been updated before you proceed with any dependent logic.
The Virtual DOM
React's Virtual DOM is at the core of its efficient rendering process. It represents the virtual representation of the actual DOM. When changes occur in your component's state or props, React creates a new Virtual DOM tree and compares it with the previous one, identifying the differences. It then updates the actual DOM only where changes have occurred.
This mechanism makes React exceptionally efficient at updating the UI, as it minimizes the number of DOM operations. However, understanding how the Virtual DOM works can help you make informed decisions when optimizing your components.
Leveraging the React DevTools
To further aid in your quest for optimal React component rendering performance, you can take advantage of the React DevTools. These browser extensions provide powerful insights into your application's component hierarchy, state, and performance. With React DevTools, you can visualize the component tree, inspect props and state, and even profile rendering performance.
Example: Using the React DevTools, you can inspect component props and state in real-time while your application is running in the browser. This allows you to identify potential areas for optimization by observing how data flows through your component tree. Additionally, the performance profiling feature can help pinpoint bottlenecks and areas that might need attention.
React.StrictMode for Identifying Issues
React.StrictMode is a development mode feature that highlights potential problems in your application during development. It does not affect your production build but can be invaluable for identifying and addressing issues early in the development process.
Example: When wrapped around your application's root component, React.StrictMode will perform additional checks and warnings, helping you spot potential problems. For instance, it can warn about components with unsafe lifecycles, or issues with concurrent mode, encouraging you to address them before they become performance bottlenecks in your production app.
Implementing React.lazy and Suspense for Code Splitting
When building larger applications, it's crucial to consider code splitting to improve initial load times. React offers a built-in solution for this: React.lazy and Suspense. React.lazy allows you to dynamically load components only when they are needed, reducing the initial bundle size.
Example: You can use React.lazy like this:
const LazyComponent = React.lazy(() => import('./LazyComponent'));
And then use Suspense to handle loading:
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
By implementing code splitting with React.lazy and Suspense, you can ensure that your users only download the code they need, which leads to faster load times and better overall performance.
Employing Server-Side Rendering (SSR) and Pre-rendering
Server-side rendering (SSR) and pre-rendering are advanced techniques for optimizing React applications. SSR renders React components on the server and sends the resulting HTML to the client, improving initial load times and SEO.
Example: You can use frameworks like Next.js to implement SSR and pre-rendering. This approach significantly enhances your application's perceived performance by providing a fully rendered page to the user right from the start.
In conclusion, achieving optimal rendering performance for your React components involves a combination of techniques and tools. By understanding React's rendering process, leveraging memoization, identifying expensive operations, and profiling your components, you can significantly improve your application's responsiveness.
Moreover, taking advantage of tools like React DevTools and React.StrictMode, implementing code splitting with React.lazy and Suspense, and considering server-side rendering and pre-rendering can take your application's performance to the next level.
Remember that performance optimization is an ongoing process, and it's essential to keep fine-tuning your React components as your application evolves. By following these best practices and staying vigilant about potential performance bottlenecks, you can ensure that your React application delivers an outstanding user experience.
End-to-End Example: Optimizing a React Image Gallery
To put the optimization techniques we've discussed into practice, let's consider an end-to-end example. Imagine you're building a React image gallery. Users can view a collection of images, and you want to ensure that the gallery is as responsive and efficient as possible.
Initial Implementation
In the initial implementation of the image gallery, you create a Gallery
component that maps through an array of images and renders them using the img
tag. You've also added a feature to toggle between grid and list views.
import React, { useState } from 'react';
const ImageGallery = ({ images }) => {
const [isGrid, setIsGrid] = useState(true);
return (
<div>
<button onClick={() => setIsGrid(!isGrid)}>
Toggle View
</button>
<div className={isGrid ? 'grid-view' : 'list-view'}>
{images.map((image, index) => (
<img key={index} src={image.url} alt={image.title} />
))}
</div>
</div>
);
};
This initial implementation is functional but might not be the most optimized version. Let's apply optimization techniques step by step.
Memoization with React.memo
One of the first things we can do is optimize the rendering of each image within the gallery. We can use React.memo
to memoize the Image
component so that it only re-renders when its props change.
const Image = React.memo(({ url, title }) => (
<img src={url} alt={title} />
));
By wrapping the Image
component with React.memo
, we prevent unnecessary re-renders of individual images.
Code Splitting with React.lazy and Suspense
Next, let's consider code splitting. In a real-world application, the image gallery might be just one part of a larger app. By implementing code splitting with React.lazy
and Suspense
, we can ensure that the image gallery's code is loaded only when the user needs it.
import React, { Suspense, lazy } from 'react';
const LazyImageGallery = lazy(() => import('./ImageGallery'));
function App() {
return (
<div>
<h1>My Awesome App</h1>
<Suspense fallback={<div>Loading...</div>}>
<LazyImageGallery images={imageData} />
</Suspense>
</div>
);
}
By using this approach, the image gallery component is loaded asynchronously, improving the initial load time of the app.
Server-Side Rendering (SSR)
In a more advanced scenario, if SEO and initial load performance are top priorities, you might consider implementing server-side rendering (SSR) for your image gallery. You could use a framework like Next.js to achieve this.
// pages/gallery.js
import React from 'react';
const ImageGallery = ({ images }) => (
<div>
{images.map((image, index) => (
<img key={index} src={image.url} alt={image.title} />
))}
</div>
);
export async function getServerSideProps() {
// Fetch images from an API or database
const images = await fetchImages();
return {
props: {
images,
},
};
}
export default ImageGallery;
In this example, the ImageGallery
component is pre-rendered on the server and sent as HTML to the client. This results in improved SEO and faster initial loading.
By following these optimization techniques, your React image gallery can deliver a responsive user experience with minimized re-renders, code splitting for efficient loading, and even server-side rendering for superior performance.
Remember that the choice of optimization techniques should depend on the specific needs and constraints of your project. Optimization is a continuous process, and as your application evolves, so should your efforts to enhance its performance.
Conclusion
In the world of web development, the quest for optimal performance is ongoing. When working with React, ensuring that your components are finely tuned is a crucial part of this journey. By understanding the rendering process, using memoization techniques, and identifying potential bottlenecks, you can create React applications that are not only feature-rich but also incredibly responsive.
React provides a wealth of tools and optimizations, and with careful consideration, you can harness the power of this library to create fast, efficient, and user-friendly web applications. The journey to React rendering performance optimization may be intricate, but the results are well worth the effort. Keep fine-tuning your React components, and your users will undoubtedly appreciate the enhanced user experience.
Subscribe to my newsletter
Read articles from Shruti Shreyasi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
