Java Calendar Date and Time tips
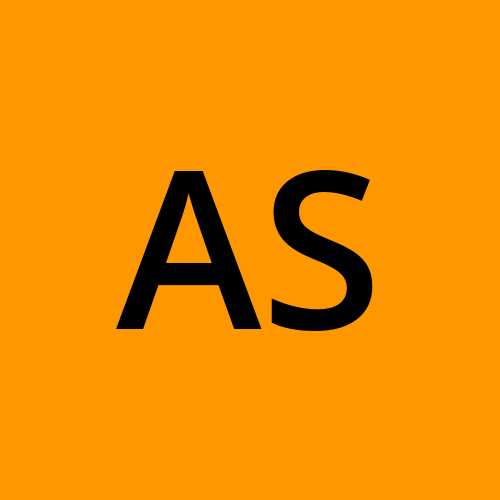
1 min read
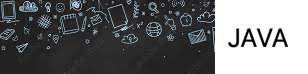
Adding some utilities concerning Java date-related objects
Finding the number of months between two dates
import java.util.GregorianCalendar;
import java.util.Calendar;
public static int numberOfMonthsBetween(Date start, Date end){
Calendar startCal = new GregorianCalendar();
startCal.setTime(start);
Calendar endCal = new GregorianCalendar();
endCal.setTime(end);
return (endCal.get(Calendar.YEAR) - startCal.get(Calendar.YEAR)) * 12
+ endCal.get(Calendar.MONTH) - startCal.get(Calendar.MONTH);
}
Date start = simpleDateFormat.parse("10-10-2021");
Date end = simpleDateFormat.parse("10-10-2022");
System.out.println("Number of months between "
+ start + " and "
+ end + " is "
+ numberOfMonthsBetween(start, end));
Output
Number of months between Sun Oct 10 00:00:00 IST 2021 and Mon Oct 10 00:00:00 IST 2022 is 12
Find if a date is a weekday
public static boolean isWeekDay(Date date){
GregorianCalendar gc = new GregorianCalendar();
gc.setTime(date);
if(Arrays.asList(Calendar.SATURDAY, Calendar.SUNDAY)
.contains(gc.get(Calendar.DAY_OF_WEEK)))
return false;
else return true;
}
System.out.println(isWeekDay(simpleDateFormat.parse("09-10-2021")));
Output
true
Get the next business day
public static Date getNextBusinessDate(Date start) {
GregorianCalendar gc = new GregorianCalendar();
gc.setTime(start);
switch (gc.get(Calendar.DAY_OF_WEEK)) {
case Calendar.FRIDAY:
gc.add(Calendar.DAY_OF_MONTH, 3);
break;
case Calendar.SATURDAY:
gc.add(Calendar.DAY_OF_MONTH, 2);
break;
default:
gc.add(Calendar.DAY_OF_MONTH, 1);
}
return gc.getTime();
}
System.out.println(getNextBusinessDate(simpleDateFormat.parse("09-10-2021")));
Output
Mon Sep 13 00:00:00 IST 2021
0
Subscribe to my newsletter
Read articles from prettyprint directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
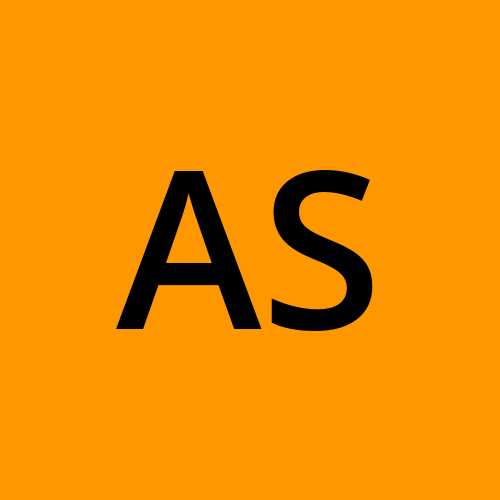
prettyprint
prettyprint
I am an application developer from bangalore who have been coding in java for around 6 years , python for around 1year and scala for around two years. Along the lines got into a little love triangle with javascript /extjs/css as well and after the break up I am missing them :) .