How to Enhance API Requests with Axios Interceptor in a React Application: A Comprehensive Guide
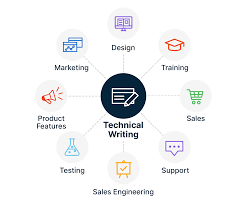
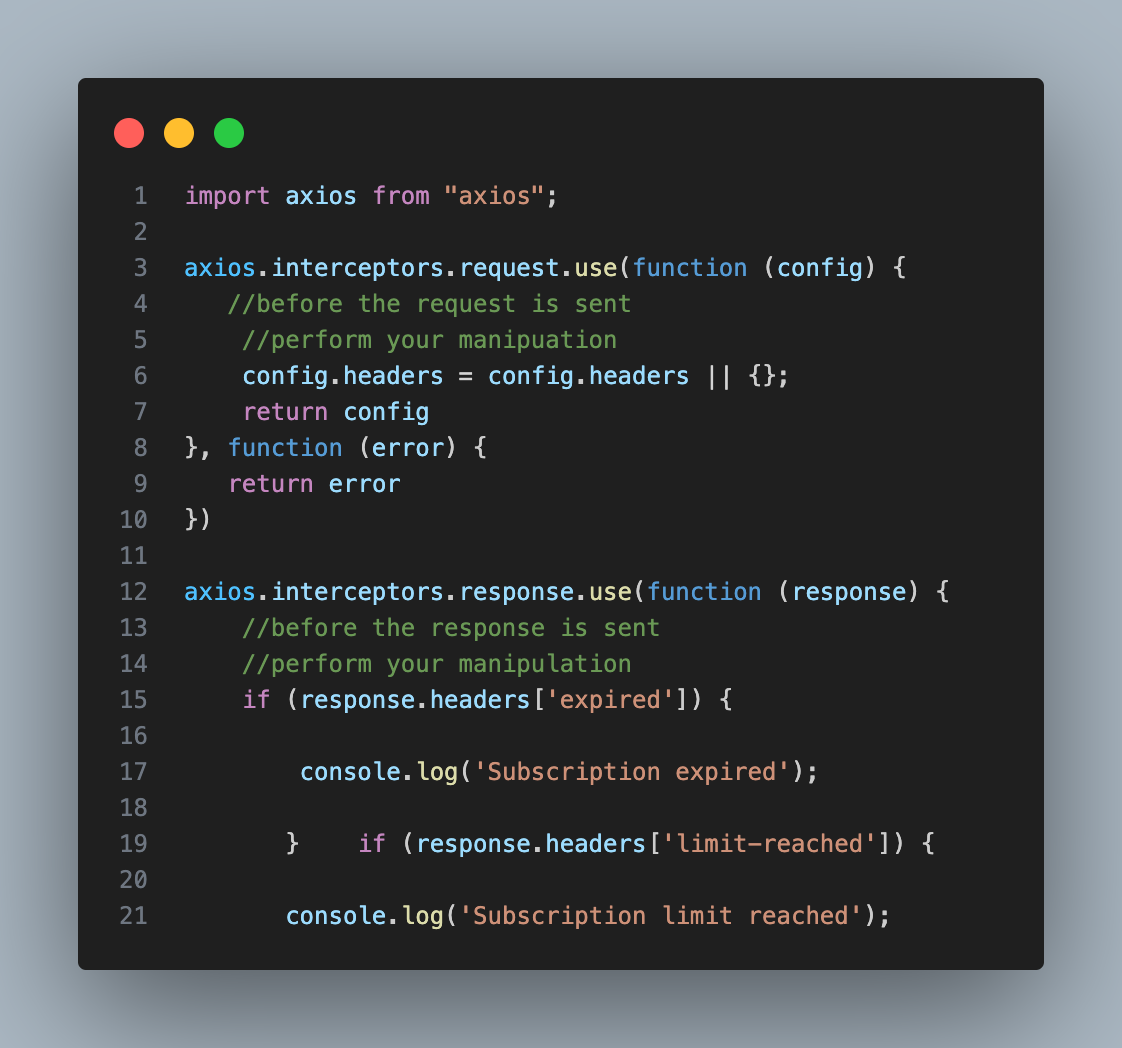
Overview
In an HTTP Protocol, a request is sent to the server and a response is received. But what if I told you there is something that can act as the middleware in an HTTP protocol that intercepts a request and response before they are made? Yes it is possible with the use of axios interceptors. When making HTTP requests and receiving responses, a developer needs to understand how to modify a request and handle a response when performing an HTTP protocol. In the Realm of HTTP Protocol where efficient data exchange is paramount, axios has become a powerful tool for developers to revolutionize how requests and responses are handled. So, let's embark on this journey and unlock the true potential of Axios in the realm of web development.
What is Axios?
Axios is a promise based API that works on web browsers and nodejs to make asynchronous requests. It is also a tool that helps browsers perform cross-origin requests; modern browsers that support Axios can send requests from one domain to a different domain. In Nodejs, Axios makes use of a native Nodejs http module which is used to make HTTP requests to a specified URL. It is also very powerful in creating CRUD operations.
Features
Intercept requests and responses which is possible with the use of Axios interceptors
Customize request headers.
Handle and manipulate a response.
They make use of a browser-based API to make asynchronous HTTP requests on the browser and in nodejs.
They cancel requests.
Posting HTML forms as JSON.
Using Axios in a React Application
Let's quickly delve into a quick and simple way of using axios in a react application.
Installation and setup
Step 1 -
Go to your terminal and type this command to set up a react project with create-react-app.
npx create-react-app axios-react
Step 2 -
change your directories into your axios project
cd axios-react
Step 3 -
Run this command to install axios
npm install axios
Sending requests method with axios in a react application
GET Method:
With Axios, we can handle asynchronous HTTP Get requests on web browsers and nodejs. With the GET Method, we can request data from a specified resource.
Here is the code of how Axios handles GET requests:
import React, { useEffect } from 'react'
import { useState } from 'react';
import axios from 'axios';
const YourComponent = () => {
const [state, setState] = useState(
{
userId: 0,
id: 0,
title: "",
completed: false
}
)
useEffect(() => {
axios.get('https://jsonplaceholder.typicode.com/todos/1'
).then((response) => {
setState(response.data)
})
},[])
return (
<div>
{state.title}
</div>
)
}
export default YourComponent
POST Method
With Axios, you can perform a POST request by creating new data, and then sending it to an API Endpoint. Check the code snippet below to see how to perform a POST Request with axios.
import React, { useEffect } from 'react'
import { useState } from 'react';
import axios from 'axios';
const YourComponent = () => {
const [title, setTitle] = useState("")
const [state, setState] = useState(
{
userId: 0,
id: 0,
title: "",
completed: false
}
)
const createPost = (e: React.FormEvent) => {
e.preventDefault()
axios.post('https://jsonplaceholder.typicode.com/todos', {
title,
userId: 0,
id:0,
completed: false
}).then((response) => {
setState(response.data)
}).catch((error) => {
console.log(error)
})
}
return (
<div>
<form onSubmit={createPost}>
<input name='title' value={title} onChange={(e) => setTitle(e.target.value)}/>
{state.title}
<button>Create Profile</button>
</form>
</div>
)
}
export default YourComponent
PUT Method
This is the request that helps in updating old data or resources in an application. Using the put method, updating data can be made possible with axios.
import React, { useEffect } from 'react'
import { useState } from 'react';
import axios from 'axios';
const YourComponent = () => {
const [title, setTitle] = useState("")
const [state, setState] = useState(
{
userId: 0,
id: 0,
title: "",
completed: false
}
)
const updatePost = (e: React.FormEvent) => {
e.preventDefault()
// perform a put request
axios.put('https://jsonplaceholder.typicode.com/todos/1', {
title,
userId: 789,
id:7,
completed: false
}).then((response) => {
setState(response.data)
}).catch((error) => {
console.log(error)
})
}
return (
<div>
<form onSubmit={updatePost}>
<input name='title' value={title} onChange={(e) => setTitle(e.target.value)}/>
{state.title}
<button>Update Profile</button>
</form>
</div>
)
}
export default YourComponent
DELETE Method
This is the request method used to delete a specified resource. In the code snippet below, you can find the implementation.
import React, { useEffect } from 'react'
import { useState } from 'react';
import axios from 'axios';
const YourComponent = () => {
const [title, setTitle] = useState("")
const [state, setState] = useState(
{
userId: 0,
id: 0,
title: "",
completed: false
}
)
React.useEffect(() => {
//this is where we fetch our data
axios.get("https://jsonplaceholder.typicode.com/todos/1").then((response) => {
setState(response.data);
});
}, []);
const deletePost = (e: React.FormEvent) => {
//this is where we delete our specified resource
e.preventDefault()
axios.delete ('https://jsonplaceholder.typicode.com/todos/1').then((response) => {
setState(response as unknown as any)
})
}
return (
<div>
<form onSubmit={deletePost}>
{state.title}
<button>Delete state</button>
</form>
</div>
)
}
export default YourComponent
Understanding Axios interceptors
Axios Interceptors are basically middleware functions that make your API requests secure and more convenient. With Axios interceptors, HTTP requests and responses are intercepted and transformed before they are handled by then and catch.
Let's delve into the code below and see how they work:
// Here we add a request interceptor
axios.interceptors.request.use(function (config) {
// Do something before request is sent
//maybe we modify the config headers before it is sent to the server
return config;
}, function (error) {
// Do something with request error
return Promise.reject(error);
});
// Add a response interceptor
axios.interceptors.response.use(function (response) {
// Any status code that lie within the range of 2xx cause this function to trigger
// Do something with response data
return response;
}, function (error) {
// Any status codes that falls outside the range of 2xx cause this function to trigger
// Do something with response error
return Promise.reject(error);
});
If you need to remove an interceptor:
const myInterceptor = axios.interceptors.request.use(function () {/*...*/});
axios.interceptors.request.eject(myInterceptor);
You can also clear all interceptors for requests or responses.
const instance = axios.create();
instance.interceptors.request.use(function () {/*...*/});
instance.interceptors.request.clear(); // Removes interceptors from requests
instance.interceptors.response.use(function () {/*...*/});
instance.interceptors.response.clear(); // Removes interceptors from response
Utilizing Axios Interceptors to secure Api Requests in a React application
Let's say, you have your react application setup, ensure you create a file and name the file axios.js.
Inside the file, update it with the following code snippet below that uses axios interceptors to modify a request and response from an API endpoint before they are handled by then and catch:
// axios.js
import axios from 'axios';
const axiosInstance = axios.create({
baseURL: 'https://api.example.com', // Your API base URL
});
// Request interceptor: Modify the request or add headers
axiosInstance.interceptors.request.use(
(config) => {
// You can add headers, authentication tokens, or perform any other task here.
// For example, adding an authorization header with a token:
// config.headers.Authorization = `Bearer ${yourAuthToken}`;
return config;
},
(error) => {
// Handle request error here (e.g., log, show an error message, or redirect)
return Promise.reject(error);
}
);
// Response interceptor: Handle responses or errors globally
axiosInstance.interceptors.response.use(
(response) => {
// Do something with the successful response, like logging, transformation, etc.
return response;
},
(error) => {
// Handle response errors here (e.g., show a toast message or redirect)
return Promise.reject(error);
}
);
export default axiosInstance;
Implementation in a React component
In the previous section, you looked at how to create an axios instance. Here, you will be using the instance to intercept an API response in a react application.
import React, { useEffect } from 'react'
import { useState } from 'react';
import axios from 'axios';
//import our axios instance
import { instance } from './interceptor';
const YourComponent = () => {
const [title, setTitle] = useState("")
const [state, setState] = useState(
{
userId: 0,
id: 0,
title: "",
completed: false
}
)
React.useEffect(() => {
//we use our axios instance which is already a middleware that handles our get request
instance.get("/1").then((response) => {
setState(response.data);
});
}, []);
const deletePost = (e: React.FormEvent) => {
e.preventDefault()
//we use our axios instance which is already a middleware that handles our delete request
instance.delete ('/1').then((response) => {
setState(response as unknown as any)
})
}
return (
<div>
<form onSubmit={deletePost}>
{state.title}
<button>Delete state</button>
</form>
</div>
)
}
export default YourComponent
Conclusion
In this article, we learned about Axios and its features. We also looked at how to send different asynchronous requests that are handled with Axios. Additionally, we explored how Axios interceptors are utilized in a React application. We've navigated through the intricacies of making various asynchronous requests with Axios, understanding the flexibility it offers in handling diverse scenarios. With Axios interceptors, developers can modify a request and handle a response.
Thanks for reading.
Subscribe to my newsletter
Read articles from LordCodeX directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
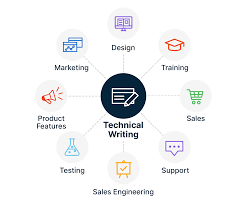
LordCodeX
LordCodeX
Software Developer | Technical Writer | Content Creator |