javascript Multidimensional Array
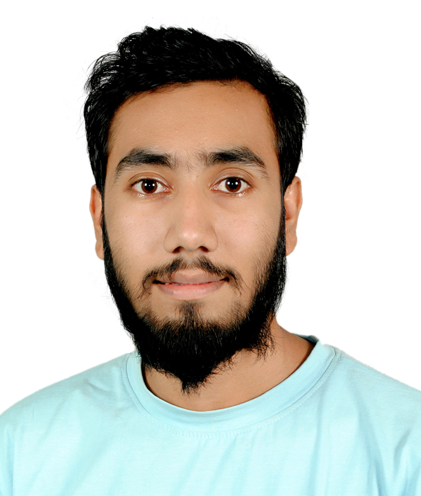
Table of contents
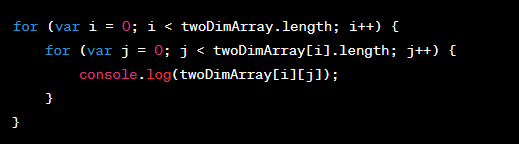
In JavaScript, a multidimensional array is an array of arrays. You can create a two-dimensional or higher-dimensional array to store and manipulate data in a tabular form or a grid-like structure. Here's how you can work with multidimensional arrays in JavaScript:
Creating a Multidimensional Array:
To create a two-dimensional array, you can nest arrays within an outer array. For example:
var twoDimArray = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
This creates a 3x3 two-dimensional array.
Accessing Elements:
To access elements in a multidimensional array, you use multiple square brackets to index each level. For example:
console.log(twoDimArray[0][0]); // Accesses the element at row 0, column 0 (value: 1)
console.log(twoDimArray[1][2]); // Accesses the element at row 1, column 2 (value: 6)
Iterating Through a Multidimensional Array:
You can use nested loops to iterate through all the elements in a multidimensional array:
for (var i = 0; i < twoDimArray.length; i++) {
for (var j = 0; j < twoDimArray[i].length; j++) {
console.log(twoDimArray[i][j]);
}
}
Modifying Elements:
You can modify elements in a multidimensional array just like a regular array:
twoDimArray[1][1] = 99; // Changes the value at row 1, column 1 to 99
Adding Rows or Columns:
To add a new row, you can simply push a new array to the outer array:
twoDimArray.push([10, 11, 12]);
To add a new column, you would need to iterate through the existing rows and add the element at the specified index to each row.
Working with Higher-Dimensional Arrays:
For arrays with more dimensions (e.g., three-dimensional arrays), you can nest more arrays as needed and extend the indexing accordingly.
var threeDimArray = [
[
[1, 2],
[3, 4]
],
[
[5, 6],
[7, 8]
]
];
console.log(threeDimArray[0][1][0]); // Accesses the element at the first level (0), second level (1), and third level (0) (value: 3)
Remember that working with multidimensional arrays can become complex as the dimensions increase, so it's important to keep your code well-structured and organized.
Follow us__
http://securitytalent.net https://t.me/Securi3yTalent
Subscribe to my newsletter
Read articles from Md Mehedi Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
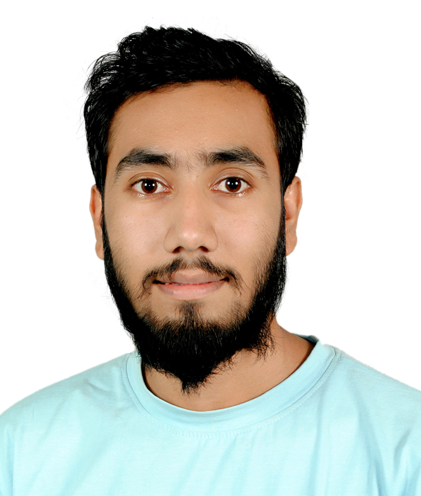