Become a master of Hashmap by just doing this !!!
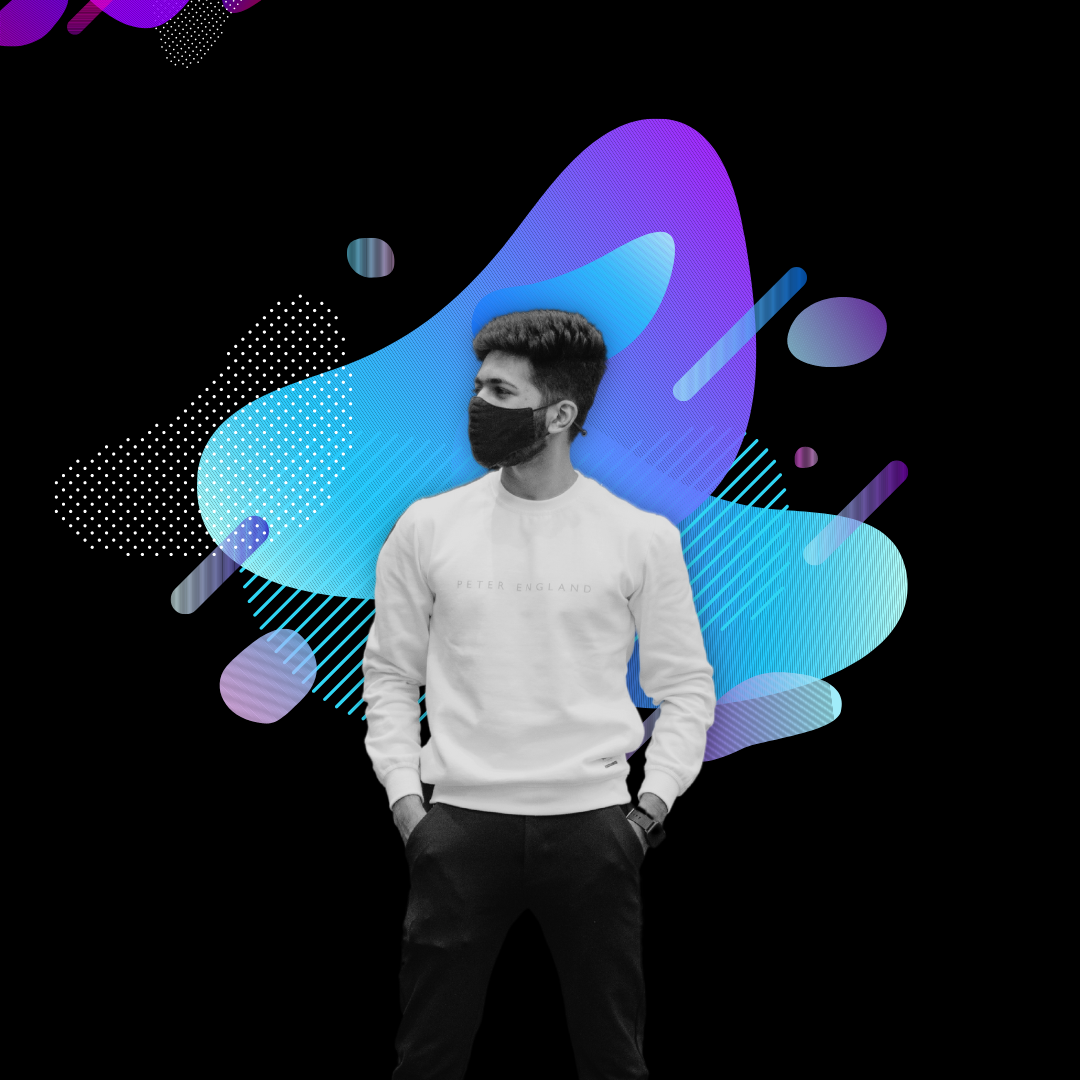
This blog contains everything which you need to study to master Hashmap. Just follow the topics step-by-step and you will be good to go.
Basics of HashMap
Inside Hash data structures we have the following available
Why HashMap ?
Stores data in terms of key(unique), value(variable) pairs (key,value)
insert, remove and update is O(1). In Java Hashmap is unordered
Import
import java.util.HashMap
Syntax
HashMap<data_type,data_type>name = new Hashmap <>();
Operations
put(key, value)
get(key)
- returns the value at the keycontainsKey(key)
- returns a boolean valueremove(key)
Inserting Elements in HashMap
//fill the values in our hashmap
for (int i : arr) {
if(!map.containsKey(i))
{
map.put(i, 1);
}else{
map.put(i, map.get(i)+1);
}
}
Iteration
We make set of all the keys,keyset will contain all the keys of HashMap
//iterate the entire hashmap
Set<Integer> k = map.keySet();
int count = 0;
for(int key : k)
{
System.out.println(key+" "+key.get(k);
}
Linked HashMap
Maintains the order of the insertion
Tree Map
Keys are sorted, and operations are in O(logn)
Important Questions
Now you just need to practice these questions and you'll be good to go.
Majority Elements
Given an integer array of size n, find all elements that appear more than L n/3 J times.
package Hashing;
import java.util.HashMap;
import java.util.Set;
public class majorityElement {
public static void main(String[] args) {
int []arr = {1,3,2,5,1,3,1,5,1};
HashMap<Integer, Integer> map = new HashMap<>();
//fill the values in our hashmap
for (int i : arr) {
if(!map.containsKey(i))
{
map.put(i, 1);
}else{
map.put(i, map.get(i)+1);
}
}
//iterate the entire hashmap
Set<Integer> k = map.keySet();
int count = 0;
for(int key : k)
{
if(map.get(key) > arr.length/3)
count++;
}
System.out.println(count);
}
}
Valid Anagram
Given two strings S and t, return true if t is an anagram of S, and false otherwise. An Anagram is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
package Hashing;
import java.util.HashMap;
public class validAnagram {
public static boolean check (String s, String t)
{
HashMap<Character,Integer> map = new HashMap<>();
for(int i = 0; i < s.length(); i++)
{
if(!map.containsKey(s.charAt(i)))
{
map.put(s.charAt(i), 1);
}else{
map.put(s.charAt(i), map.get(s.charAt(i))+1);
}
}
System.out.println(map);
for(int i =0 ; i< t.length(); i++)
{
if(!map.containsKey(t.charAt(i)))
{
return false;
}else{
if(map.get(t.charAt(i))==1)
map.remove(t.charAt(i));
else
map.put(t.charAt(i), map.get(t.charAt(i))-1);
}
}
return map.isEmpty();
}
public static void main(String[] args) {
String s = "abcd";
String t = "bcad";
System.out.println(check(s, t) );
}
}
Count Distinct Elements
Given an integer array of size n. Find the number of distinct elements in the array.
package Hashing;
import java.util.HashMap;
import java.util.HashSet;
public class countDistinct {
// public static void main(String[] args) {
// int []arr = {1,3,2,5,1,3,1,5,1};
// HashSet<Integer> set = new HashSet<>();
// for(int nums: arr)
// {
// set.add(nums);
// }
// System.out.println(set.size());
public static void main(String[] args) {
int []arr = {1,3,2,5,1,3,1,5,1};
HashMap<Integer,Integer> map = new HashMap<>();
for(int nums: arr){
if(map.containsKey(nums)){
continue;
}else{
map.put(nums, 1);
}
}
System.out.println(map.size());
}
}
Longes Common SubSequence
package Hashing;
import java.util.HashMap;
import java.util.HashSet;
public class longestCommonSequence {
public static void main(String[] args) {
int arr[] = {2,5,3,10,8,11,1,20,6,4};
HashSet<Integer> map = new HashSet<>();
//filling the hashSet
for (int i : arr) {
map.add(i);
}
int length = 0;
for(int i = 0; i <arr.length; i++)
{
int temp = arr[i];
int l = 0;
while(map.contains(temp+1))
{
l++;
temp += 1;
}
length = Math.max(length, l);
}
System.out.println(length+1);
}
}
Zero Sub Array Sum
Given an array of positive and negative numbers, the task is to find if there is a subarray (of size at least one) with 0 sum.
package Hashing;
import java.util.HashMap;
public class zeroSubArraySum {
public static void main(String[] args) {
int[] arr = {15, -2, 2, -8, 1, 7, 10, 23};
int sum = 0;
int length = 0;
HashMap<Integer,Integer> map = new HashMap<>();
for(int j = 0; j < arr.length; j++)
{
sum += arr[j];
if(map.containsKey(sum)){
length = Math.max(length, j-map.get(sum));
}else{
map.put(sum,j);
}
}
System.out.println(length);
}
}
Now, if have solved all the above questions you can go to leetcode or GFG to solve more such problems.
Happy Coding!
Subscribe to my newsletter
Read articles from Vandit Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
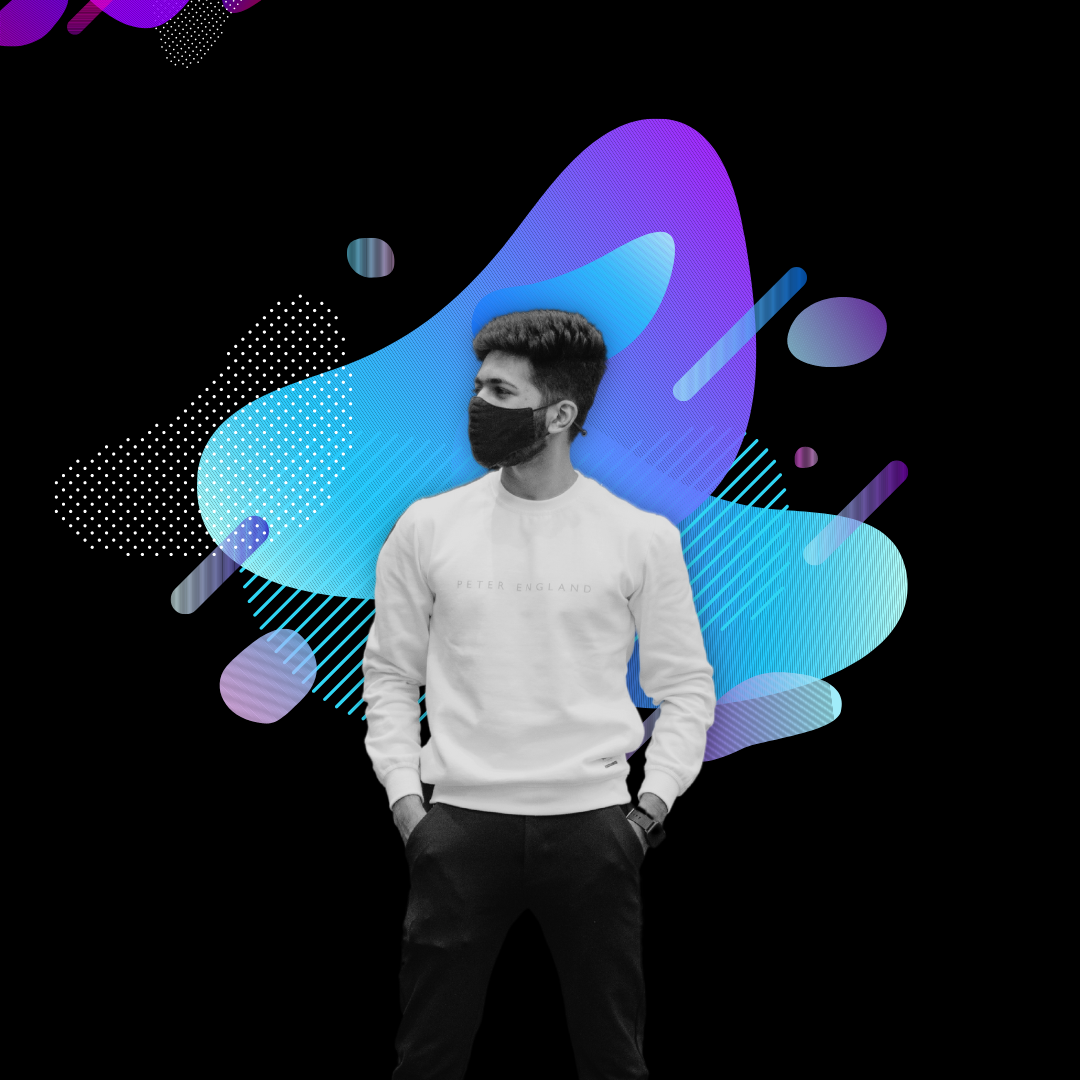
Vandit Dubey
Vandit Dubey
4th year Engineering(CSE) student, passionate about web development, seeking opportunities where I can use my diverse skillset to the fullest and provide room for growth. With a learning attitude and a gauging character and reliability factor thriving to achieve success and also help the business/company grow.