Enum in JAVA for beginners

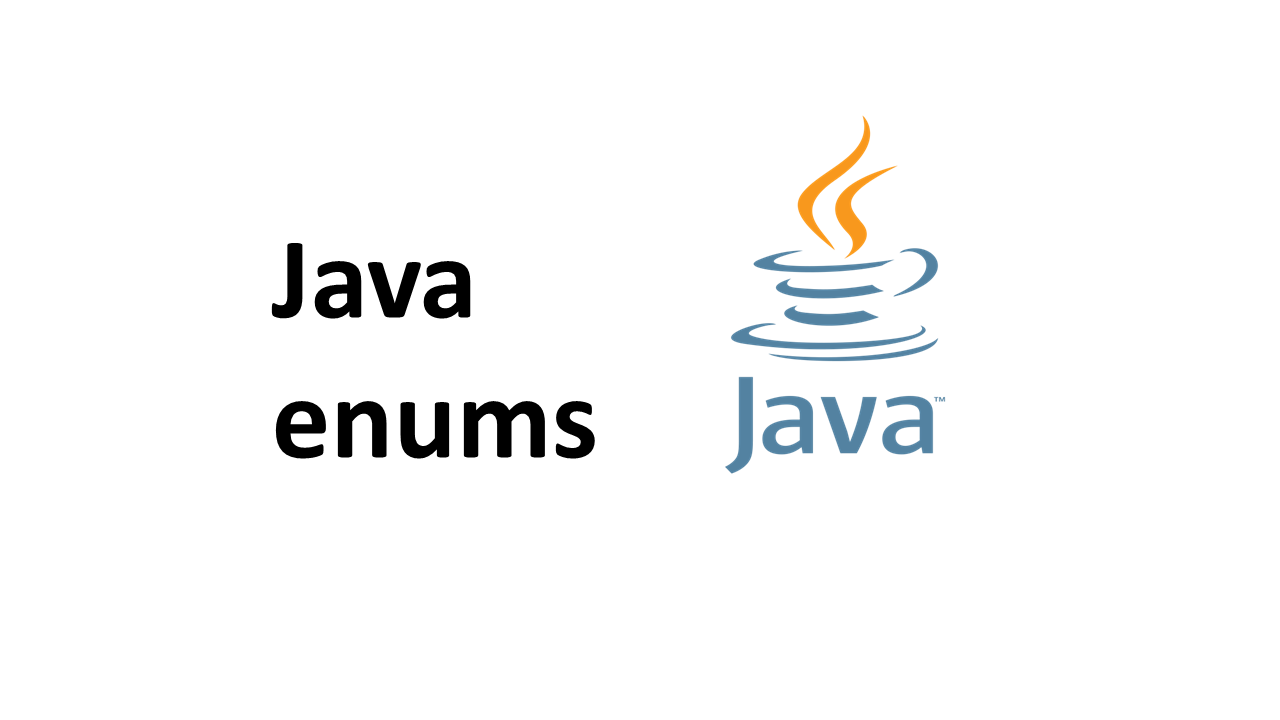
Introduction
Java, a versatile and powerful programming language, offers a multitude of features to simplify and enhance the coding experience. If you're new to programming or just starting your journey with Java, you might have heard about "enums." These are an essential part of Java and are used to represent a fixed set of constants or enumerated values.
In this beginner's guide, we'll dive headfirst into the world of enums in Java, breaking down this concept into digestible pieces. Whether you're just getting started or looking to solidify your understanding of enums, you're in the right place.
In the upcoming sections, we'll explore what enums are, why they're valuable, and how to work with them effectively in Java.
What is an enum?
In Java, an enum is a data type used to define custom data types, often referred to as enumerated data types. Enums are employed to represent a group of named constants. They are more robust and versatile. You can define an enum either within a class or outside a class. Every enum is implemented internally using the class concept. Each enum constant represents an object of the enum type.
enum declaration
Every enum constant is inherently public, static, and final. Consequently, we can access enum constants by using the enum's name.
public class enum_example {
enum Month
{
Jan, Feb, March, April,
May, June;
}
public static void main(String args[])
{
Month m = Month.Jan;
System.out.println(m);
}
}
Enums can be declared either within a class or outside of a class, but they cannot be declared inside a method. Enum declarations are not allowed within a method.
enum declaration outside the class. An enum can be declared outside of a class, and when declaring an enum in this manner, modifiers like public, strictfp and default can be utilized.
public enum Color {
RED, GREEN, BLUE
}
public class Main {
public static void main(String[] args) {
Color favoriteColor = Color.BLUE;
System.out.println("My favorite color is " + favoriteColor);
}
}
When declaring an enum within a class, the applicable modifiers include public, default, private, protected, strictfp and static.
public class enum_example1 {
// Enum declared inside a class
public enum Days {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY
}
public static void main(String[] args) {
Days today = Days.THURSDAY;
System.out.println("Today is " + today);
}
}
enum with switch case in Java
In Java, an enum
with a switch
statement is a powerful combination that allows you to easily and cleanly handle different cases or options represented by the enum constants. we can pass an enum type as an argument to switch statements.
using enums with a switch statement enhances code readability, maintainability, and type safety, which is particularly useful when dealing with a fixed set of options or states in your Java programs.
enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY
}
public class EnumSwitchExample {
public static void main(String[] args) {
Day today = Day.FRIDAY;
switch (today) {
case MONDAY:
System.out.println("It's Monday. The weekend is over.");
break;
case TUESDAY:
System.out.println("It's tuesday.");
case WEDNESDAY:
System.out.println("It's wednesday.");
case THURSDAY:
System.out.println("It's a workday.");
break;
case FRIDAY:
System.out.println("It's Friday. The weekend is almost here!");
break;
case SATURDAY:
System.out.println("It's saturday.");
case SUNDAY:
System.out.println("It's the weekend. Enjoy your time off.");
break;
default:
System.out.println("Invalid day.");
}
}
}
enum with Constructor in Java
An enum can indeed contain constructors, and these constructors are executed individually for each enum constant at the time of the enum class loading.
We cannot create enum objects directly using the new operator, and as a result, we cannot invoke an enum constructor directly.
Inside an enum, you can declare methods, but they must be concrete methods; you cannot declare abstract methods.
// Enum declared outside a class
enum Color{
RED, GREEN, BLUE;
// Constructor for Color enum
Color()
{
System.out.println("enum example with constructor");
}
}
class enum_example3 {
public static void main(String[] args) {
Color c= Color.GREEN;
System.out.println("hello constructor enum");
}
}
Speciality of Java enum
We can declare the main method inside an enum.
Java enums are more powerful.
An empty enum is a valid syntax.
Every enum in Java is a direct child class of Java.lang.Enum
makes this class the base class for all Java enums. It implements the serialization and comparable interfaces.
Every enum implicitly contains a values()
method to return all the values present inside the enum.
Within the enum, the order of constants is important, and you can represent this order using the ordinal value. You can find the ordinal value of an enum constant by using the ordinal()
method.
If we write only a method inside the enum block, it is necessary to use a semicolon before the method. Otherwise, if we write the method without the semicolon, the code is considered invalid.
You cannot first write the method inside the enum block and then declare the enum data type; if you do that, it is considered invalid.
In an enum block, writing only a method is not valid.
Conclusion
In our journey through the fascinating world of enums in Java, we've unraveled the essence of this essential concept. We began with the basics, understanding what enums are and why they are a vital part of Java programming.
We then explored how to declare enums, use them effectively in switch cases, and even enhance their capabilities by incorporating constructors. Along the way, we covered key points to ensure your proficiency in working with enums
Enums, with their ability to represent a fixed set of constants, offer clarity and structure to your code, making it more robust and understandable. As you continue your journey in the world of Java programming, remember that enums are your allies in creating organized, readable, and maintainable code.
So, keep exploring, keep coding, and keep utilizing enums to simplify your Java programming adventures. The world of Java development is at your fingertips.
Subscribe to my newsletter
Read articles from Ranju Kumari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
