Day 9 | GIT - Version Controller (aka Source Code Management Tool-SCM) & GitHub
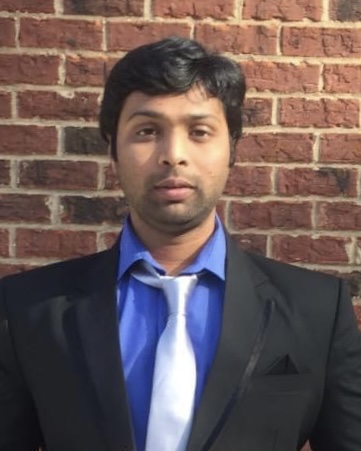
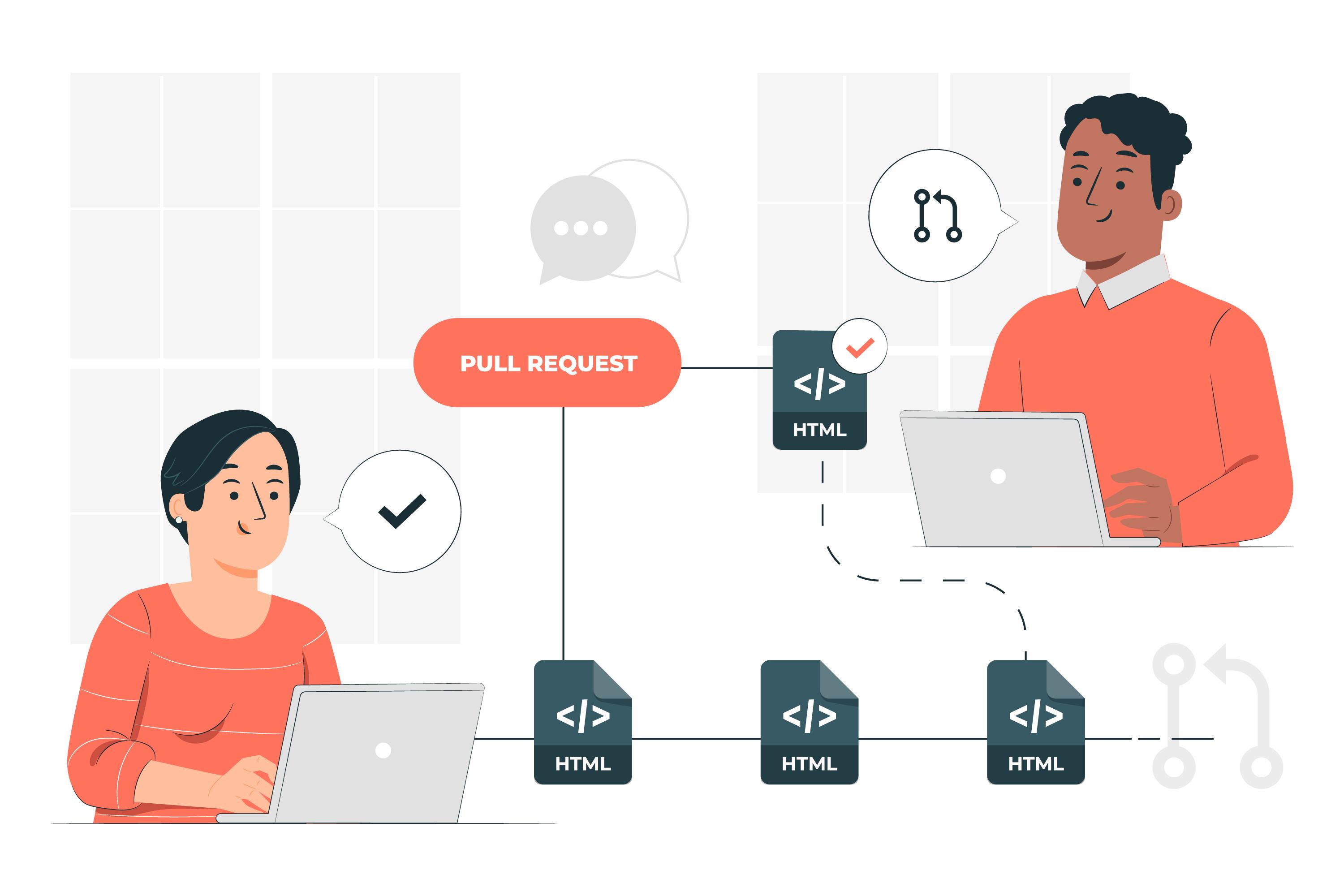
Git is a distributed version control system (DVCS) that is widely used in software development to manage and track changes in source code. It was created by Linus Torvalds in 2005 and has become the de facto standard for version control in the software industry.
What is the tool? What problem does it solve?
Git is a tool for tracking changes in source code during software development.
It allows multiple developers to work on the same project simultaneously without conflicts.
Git helps in maintaining a history of changes, which is crucial for collaboration, code review, and software releases.
It's especially useful for managing distributed teams and open-source projects.
Sample examples and Basic implementations.
These are some of the basic Git commands:
git init
: Initializes a new Git repository.git clone <repository>
: Copies a Git repository from a remote source (GITHUB).git add <file>
: Stages changes for commit.git commit -m "Message"
: Records changes in the repository with a descriptive message.git pull
: Fetches changes from a remote repository (GITHUB).git push
: Pushes local changes to a remote repository (GITHUB).
Understanding the Script of the Tool:
Git Commands and Snapshots:
Git Operating Modes: Git can be operated through the command line (CLI) or graphical user interfaces (GUI). For this example, we'll use the CLI.
Creating a Repository: You can create a new Git repository using the
git init
command. For instance, if you have a project folder called "MyProject," you can initialize it as a Git repository:cd MyProject git init
This command initializes a Git repository in the "MyProject" directory.
Staging Changes: To work with Git, you need to stage your changes. You can do this using the
git add
command. Let's say you have a file named "index.html" that you've modified:git add index.html
In this stage the changes made to "index.html."
Committing Changes: Once changes are staged, you can commit them to the repository using
git commit
:git commit -m "Added new feature to index.html"
This creates a snapshot of the changes and adds a descriptive commit message.
Creating Branches: Git enables you to create branches using the
git branch
command. For instance, to create a new branch called "feature-branch," you can run:git branch feature-branch
Switching Between Branches: You can switch between branches with the
git checkout
command:git checkout feature-branch
This allows you to work on separate features or bug fixes in isolation.
Real-Time Example:
Let's say you're working on a website project, and your goal is to add a new "Contact Us" page to the site. You start by creating a new branch for this feature:
git branch contact-us-feature
git checkout contact-us-feature
Now, you make changes to your project, add new HTML and CSS files for the "Contact Us" page, and use Git to track these changes:
git add contactus.html
git add styles/contactus.css
git commit -m "Added Contact Us page"
After making these changes and committing, your project has a snapshot with the "Contact Us" page.
Meanwhile, your colleague is working on a different feature in another branch. Git allows both of you to work independently without interfering with each other's code. You can later merge your changes with the main branch when the features are complete and tested.
The snapshot-based system that Git uses efficiently tracks changes and allows for branching and merging. This approach helps manage different features or bug fixes in a collaborative environment while maintaining a clear history of changes and who made them.
How Git Is Used in Companies (Real Corporate Examples):
Company: Let's consider a fictitious company named "TechSolutions Inc."
Scenario: TechSolutions Inc. is a software development company with a team of developers working on a web application called "TechApp." They use Git to manage their source code.
Usage of Git in TechSolutions Inc.:
Version Control: Git allows TechSolutions Inc. to maintain a versioned codebase for the "TechApp" project. Each developer can work on their features or bug fixes in isolation, making changes without affecting the main codebase.
Branching: Developers at TechSolutions Inc. use branches in Git to create isolated environments for their work. For example:
When a developer, Alice, is tasked with adding a new feature, she creates a feature branch from the main codebase.
Alice's colleague, Bob, is working on a critical bug fix, so he also creates a separate branch.
This branching strategy allows both Alice and Bob to work independently without interfering with each other.
Collaboration: Git is integrated with a platform like GitHub in this scenario. TechSolutions Inc. hosts its Git repositories on GitHub. This provides a central platform for collaboration:
Developers push their branches to the shared GitHub repository.
They can create pull requests for code review and discuss changes with their colleagues.
Code reviews help maintain code quality and catch potential issues.
Continuous Integration (CI) and Continuous Deployment (CD): TechSolutions Inc. integrates Git with CI/CD tools like Jenkins. Whenever changes are pushed to the main branch (e.g., after a successful code review), Jenkins automatically builds and deploys the application to a test environment for quality assurance.
Release Coordination: When it's time for a new release, the team uses Git tags to mark specific commits as release points. This makes it easy to track which codebase was used for each version of the "TechApp."
Error and Bug Tracking: In addition to Git, the team might use integrated issue-tracking systems to monitor and manage errors and bugs in the application. Developers can link issues to specific branches or commits in Git for traceability.
Example: Imagine Alice working on a new "User Profile" feature and Bob fixing a critical security bug. They use Git to manage their code separately. When their work is complete and reviewed, it's merged into the main codebase, and Jenkins deploys it for further testing. Git plays a vital role in keeping their work organized, tracking changes, and enabling collaboration.
- In real corporate scenarios, the use of Git, along with platforms like GitHub or GitLab, simplifies teamwork, version control, and code quality management, which are crucial for software development companies like TechSolutions Inc.
Corporate Errors in Git and Troubleshooting:
Let's go through each of these common errors and how to troubleshoot them with simple examples:
Merge Conflicts:
Error: Merge conflicts occur when two or more developers make changes to the same part of a file, and Git can't automatically determine which changes to keep.
Troubleshooting: To resolve a merge conflict, follow these steps:
Git will mark the conflicted area in the file with special markers
(
<<<<<<<
,=======
,>>>>>>>
).Manually edit the file to keep the changes you want.
Remove the conflict markers and save the file.
Add the resolved file with
git add
.Commit the changes with
git commit
.
Example: Imagine two developers, Alice and Bob, both edit the same line in a file. A merge conflict occurs when Git tries to combine their changes.
Accidental Data Loss:
Error: Accidental data loss can happen when you use commands like
git reset
orgit clean
without being careful.Troubleshooting: To recover accidentally deleted data, consider these steps:
Use
git reflog
to find the commit reference you want to restore.Use
git cherry-pick
orgit branch
to create a new branch pointing to the lost commit.
Example: If mistakenly run git reset --hard
and lose the last few commits, git reflog
can help find the commit hash to recover them.
Improper Branching:
Error: Creating too many branches or not following a clear branching strategy can lead to confusion.
Troubleshooting: Maintaining a clear branching strategy, such as:
Master branch for stable code.
Feature branches for new features.
Hotfix branches for critical bug fixes.
Delete branches once their purpose is served.
Example: A common mistake is creating too many feature branches without proper organization, making it hard to track progress.
Best Practices to Minimize Errors:
Regular Commits: Commit frequently with descriptive messages. Small, focused commits are easier to manage.
Branching Strategies: Use a clear branching strategy, like Git Flow or GitHub Flow, to streamline development.
Code Reviews: Conduct code reviews to catch errors before they reach the repository.
Let's say you're working on a team project. A common example might involve you and a colleague working on different parts of the same file. If you both make changes and then attempt to merge your work, a merge conflict could arise. You would then need to resolve the conflict by manually choosing which changes to keep and which to discard. This is a merge conflict scenario that highlights the importance of clear communication and coordination in a team to minimize such errors.
GitHub
GitHub is a web-based platform for version control and collaborative software development.
It serves as a central hub where individuals like Alice & Bob as said earlier and other team members can manage, share, and collaborate on coding projects.
With features like version tracking, issue management, and pull requests, GitHub streamlines the development process and fosters community-driven innovation.
It's the go-to platform for hosting and sharing open-source code and has become an essential tool for developers worldwide.
A sample diagram that represents GitHub:
[ GitHub ]
/ | \
[ Repositories ]
/ | \
[ Commits ] [ Issues ] [ Collaborators ]
In this diagram:
"GitHub" is the central platform.
"Repositories" are where your code projects are hosted.
"Commits" represent code changes and version history.
"Issues" are used for tracking bugs and feature requests.
"Collaborators" are team members working on the project.
This illustrates how GitHub acts as a central hub for managing code, tracking changes, and facilitating collaboration.
What does the Git Push command do:
A repository is created in GitHub, once it is created and when the code is ready to push after git init, git add index.html, git commit -m "Initial Commit", we need to push the code using the git push "repository name" from the local machine to the GitHub repo where other collaborators/peers view the code.
- Pushing to GitHub:
Local Machine -> GitHub Repository
"Git clone" is used to clone the repo from GitHub to the local machine.
Cloning from GitHub:
GitHub Repository -> Local Machine
In the world of version control, these are the fundamental building blocks โ the ABCs of Git. ๐
Subscribe to my newsletter
Read articles from Sarat Motamarri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
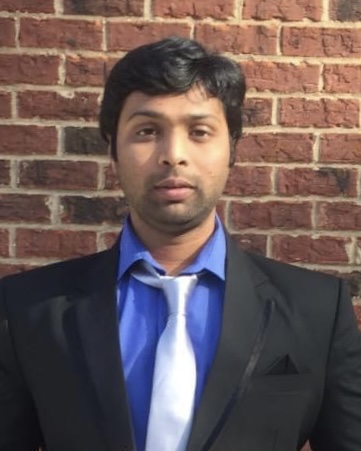
Sarat Motamarri
Sarat Motamarri
Hey there, I'm Sarat Motamarri, an aspiring engineer mastering the art of DevOps & AWS. If you're as passionate about the cloud and open-source wizardry as I am, you're in the right place! Let's explore the skies together ๐๐ฎ #CloudEnthusiast #DevOpsDreamer"