Flutter Riverpod 2.0 Explained: The Complete Guide
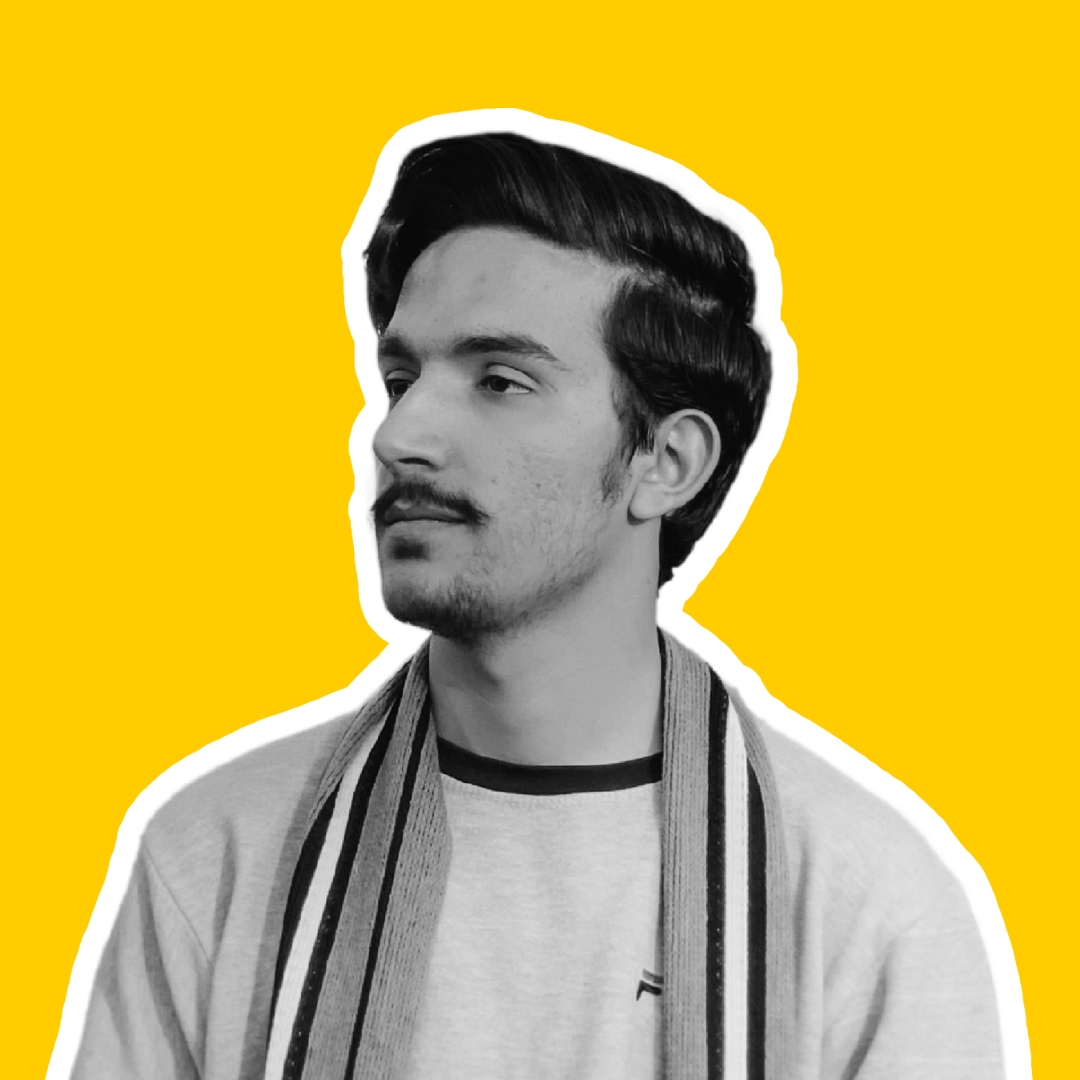
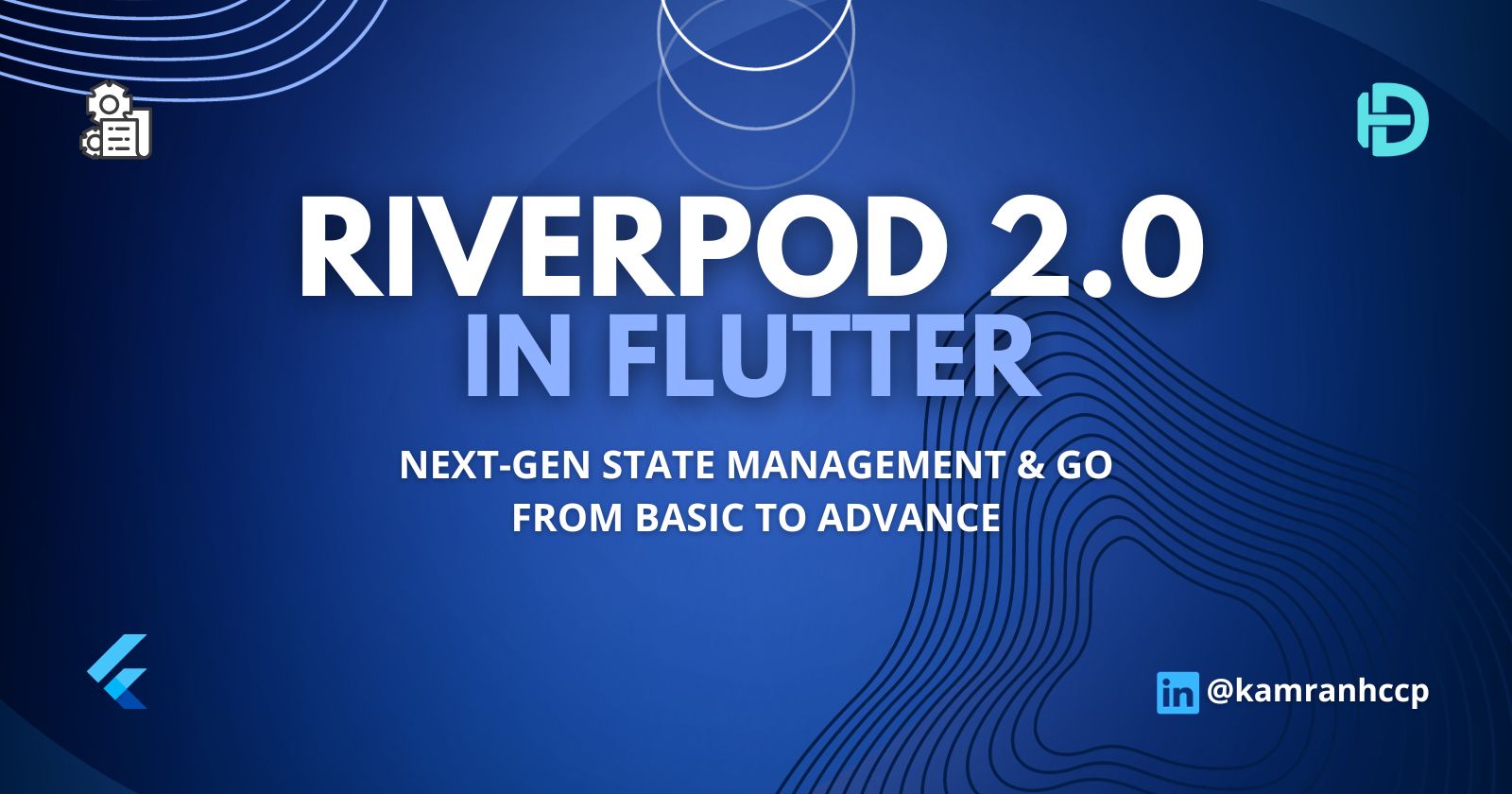
Flutter Riverpod is a powerful state management library that simplifies and enhances the way you manage your application's state in Flutter. With the release of Riverpod 2.0, many new features and improvements make it an even more compelling choice for state management in your Flutter apps. In this ultimate guide, we'll explore Riverpod 2.0 in-depth, covering everything from its core concepts to advanced features and best practices.
Table of Contents
Introduction to Riverpod
What is Riverpod?
Why Choose Riverpod?
Riverpod vs. Other State Management Solutions
Core Concepts
Providers
Reading and Watching Providers
Mutating State
Consumer Widgets
Scoped Providers
Combining Providers
Riverpod 2.0 Features
NotifierProvider and AsyncNotifierProvider
Dependency Injection
Overriding Dependencies for Testing
Provider Observers
Using Riverpod with Flutter 2.0
Testing with Riverpod
Writing Widget Tests with ProviderScope
Mocking Dependencies for Testing
Testing Async Notifiers
Logging with ProviderObserver
Advanced Topics
Using StateNotifierProvider
Family Modifiers
Using AutoDispose and Caching
Scoping Providers
Filtering Widget Rebuilds with "select"
Best Practices
Structuring Your Flutter Project with Riverpod
Performance Optimization
Error Handling and Debugging
Migrating to Riverpod 2.0
Case Studies
- Real-world examples of using Riverpod in Flutter apps
Resources and Further Learning
Official Documentation
Example Apps
Online Courses and Tutorials
1. Introduction to Riverpod
What is Riverpod?
Riverpod is a state management library for Flutter that simplifies the way you manage application state. It's designed to be easy to use, testable, and to help you avoid common state management pitfalls. With Riverpod, you can create and manage your state effortlessly, making your Flutter app more efficient and maintainable.
Why Choose Riverpod?
Predictable: Riverpod's architecture promotes predictable state management, reducing the risk of bugs and unexpected behaviour.
Testable: Riverpod simplifies testing with its ability to override dependencies, allowing you to isolate and test specific parts of your app.
Flexible: It offers a wide range of providers, from simple state providers to more advanced notifiers. This flexibility allows you to choose the right tool for your specific use case.
Performance: Riverpod is optimized for performance and efficiently rebuilds widgets when state changes, minimizing unnecessary UI updates.
Community Support: Riverpod has an active community of developers and contributors who share their knowledge and create helpful resources.
Riverpod vs. Other State Management Solutions
Comparing Riverpod to other state management solutions like Provider and BloC, you'll find that Riverpod combines the best features of both. It offers the simplicity of Provider and the predictability of BloC, making it a strong contender for state management in Flutter.
2. Core Concepts
Providers
Providers in Riverpod are the building blocks of your application's state. They can be simple state providers or more complex notifiers, and they serve as the source of truth for your app's data.
Example:
dartCopy codefinal counterProvider = StateProvider((ref) => 0);
In this example, counterProvider
is a state provider that initializes the counter with 0.
Reading and Watching Providers
You can access the state of a provider by either reading or watching it. Using ref.watch
()
allows you to observe changes and rebuild widgets when the state changes, while ref.read
()
gives you a one-time read of the state.
Example:
dartCopy codefinal counter = ref.watch(counterProvider);
In this case, counter
is now watching changes in the counterProvider
.
Mutating State
Riverpod provides notifiers like StateProvider
and StateNotifierProvider
for mutating state. You can use these notifiers to change the state of your providers, and any dependent widgets will automatically rebuild.
Example:
dartCopy coderef.read(counterProvider).state++; // Increment the counter
Consumer Widgets
Consumer widgets, such as ConsumerWidget
and Consumer
, allows you to access providers within your UI code. They help you separate the UI from the business logic and make your code more organized and testable.
Example:
dartCopy codeConsumer(
builder: (context, ref, child) {
final counter = ref.watch(counterProvider);
return Text('Count: ${counter.state}');
},
)
In this example, the Consumer
widget rebuilds when counterProvider
changes, ensuring that the UI always displays the correct count.
Scoped Providers
Riverpod supports scoped providers that allow you to create different scopes for providers. This can be useful for managing the state in different parts of your app.
Example:
dartCopy codefinal localCounterProvider = Provider<int>((ref) => 0);
Here, localCounterProvider
is a provider that exists within a certain scope, separate from the global state.
Combining Providers
You can combine providers to build more complex providers. For instance, you can create a provider that depends on other providers to fetch data.
Example:
dartCopy codefinal userProvider = FutureProvider<User>((ref) {
final authService = ref.watch(authServiceProvider);
return authService.getUser();
});
In this example, userProvider
combines data from authServiceProvider
to provide user information.
3. Riverpod 2.0 Features
NotifierProvider and AsyncNotifierProvider
Riverpod 2.0 introduces the new NotifierProvider
and AsyncNotifierProvider
. These providers simplify working with notifiers, making it easier to manage mutable states in your app.
Example:
dartCopy codefinal counterProvider = NotifierProvider<int, StateController<int>>((ref) {
return StateController(0);
});
Here, counterProvider
is a NotifierProvider
that manages an integer counter.
Dependency Injection
Dependency injection is a crucial aspect of Riverpod. You can override dependencies for testing or to change the behaviour of a provider. This is especially useful for isolating parts of your app for testing purposes.
Example:
dartCopy codefinal mockRepositoryProvider = Provider((ref) => MockRepository());
final realRepositoryProvider = Provider((ref) => RealRepository());
final repositoryProvider = Provider((ref) {
if (isTesting) {
return ref.read(mockRepositoryProvider);
} else {
return ref.read(realRepositoryProvider);
}
});
In this example, repositoryProvider
dynamically selects a repository implementation based on whether you are testing or in a production environment.
Provider Observers
Riverpod comes with a built-in observer system called ProviderObserver
. This allows you to log, monitor, and react to changes in your providers. It's an invaluable tool for debugging and optimizing your app.
Example:
dartCopy codeclass Logger extends ProviderObserver {
@override
void didUpdateProvider(
ProviderBase provider,
Object? previousValue,
Object? newValue,
ProviderContainer container,
) {
print('[${provider.name ?? provider.runtimeType}] value: $newValue');
}
}
In this example, we create a Logger
that logs changes to providers in your app.
Using Riverpod with Flutter 2.0
Riverpod is fully compatible with Flutter 2.0, taking advantage of new features and improvements in the latest Flutter version. You can use Riverpod with the new Navigator 2.0
and other enhanced Flutter features.
4. Testing with Riverpod
Testing is an essential part of app development, and Riverpod makes it easier. You can write widget tests without sharing state between tests, thanks to the implicit creation of ProviderContainer
by ProviderScope
.
Example:
dartCopy codeawait tester.pumpWidget(ProviderScope(child: MyApp()));
In this setup, each test has its own ProviderScope
, ensuring that they don't share any state.
Mocking Dependencies for Testing
Riverpod enables you to override dependencies for testing. You can replace a real implementation with a mock implementation for unit testing, eliminating the need to make network requests or access external services during testing.
Example:
dartCopy codeclass MockMoviesRepository implements MoviesRepository {
@override
Future<List<Movie>> favouriteMovies() {
return Future.value([
Movie(id: 1, title: 'Rick & Morty', posterUrl: 'https://nnbd.me/1.png'),
Movie(id: 2, title: 'Seinfeld', posterUrl: 'https://nnbd.me/2.png'),
]);
}
}
void main() {
testWidgets('Override moviesRepositoryProvider', (tester) async {
await tester.pumpWidget(
ProviderScope(
overrides: [
moviesRepositoryProvider.overrideWithValue(MockMoviesRepository())
],
child: MoviesApp(),
),
);
});
}
In this example, we override moviesRepositoryProvider
with a MockMoviesRepository
for testing.
Logging with ProviderObserver
Riverpod includes a ProviderObserver
allows you to monitor and log changes in your providers. This is beneficial for debugging and understanding how your app's state evolves.
Example:
dartCopy codeclass Logger extends ProviderObserver {
@override
void didUpdateProvider(
ProviderBase provider,
Object? previousValue,
Object? newValue,
ProviderContainer container,
) {
print('[${provider.name ?? provider.runtimeType}] value: $newValue');
}
}
In this example, we create a Logger
that logs changes to providers in your app.
5. Advanced Topics
Using StateNotifierProvider
StateNotifierProvider is a powerful feature that allows you to manage complex states more efficiently. It's especially useful when working with multiple pieces of related state.
Example:
dartCopy codefinal todoListProvider = StateNotifierProvider<TodoList, List<Todo>>(() {
return TodoList();
});
Here, todoListProvider
uses a StateNotifier
to manage a list of todos.
Family Modifiers
Family modifiers are used when you need to provide different instances of the same provider based on a parameter. This is valuable when working with lists, maps, or any situation where providers should vary depending on some input.
Example:
dartCopy codefinal todoProvider = Provider.family<String, int>((ref, id) {
return 'Todo $id';
});
In this example, todoProvider
creates different todo items based on the id
parameter.
Using AutoDispose and Caching
Riverpod offers features like autoDispose
to automatically clean up providers when they are no longer needed. Additionally, you can use caching to store and retrieve expensive resources efficiently.
Example:
dartCopy codefinal cachedDataProvider = Provider.autoDispose((ref) {
return ExpensiveResource();
});
In this case, cachedDataProvider
disposes of the resource when it's no longer in use.
Scoping Providers
Riverpod allows you to create provider scopes, ensuring that providers are only available within a specific widget subtree. This helps manage state in different parts of your app.
Example:
dartCopy codefinal scopedCounterProvider = StateProvider((ref) => 0);
class ScopedCounterWidget extends ConsumerWidget {
@override
Widget build(BuildContext context, ScopedReader watch) {
final count = watch(scopedCounterProvider);
return Text('Count: ${count.state}');
}
}
In this example, scopedCounterProvider
is available only within the ScopedCounterWidget
widget.
Filtering Widget Rebuilds with "select"
The select
method allows you to fine-tune when a widget should rebuild based on a specific provider's state. This is a powerful optimization tool for managing widget rebuilds.
Example:
dartCopy codefinal counterProvider = StateProvider((ref) => 0);
final doubledCounterProvider = Provider<int>((ref) {
final count = ref.watch(counterProvider);
return count * 2;
});
Consumer(
builder: (context, ref, child) {
final doubledCount = ref.select(doubledCounterProvider);
return Text('Double Count: $doubledCount');
},
)
In this example, the Consumer
widget rebuilds only when doubledCounterProvider
changes, not when counterProvider
changes.
6. Best Practices
Structuring Your Flutter Project with Riverpod
Properly structuring your Flutter project with Riverpod is crucial for maintainability. Learn best practices for organizing your code and providers for maximum efficiency.
Performance Optimization
Riverpod is optimized for performance, but there are still ways to further optimize your app. We'll explore techniques and best practices for enhancing your app's speed and responsiveness.
Error Handling and Debugging
We'll cover strategies for handling errors and debugging your Riverpod-based app. Understanding how to deal with error states and unexpected behaviour is essential for robust applications.
Migrating to Riverpod 2.0
If you're already using Riverpod 1.x, we'll guide you through the process of migrating to Riverpod 2.0, ensuring a smooth transition for your existing projects.
7. Case Studies
We'll explore real-world examples of using Riverpod in Flutter apps, demonstrating how to implement state management in different scenarios and applications.
8. Resources and Further Learning
Official Documentation: The official Riverpod documentation is an invaluable resource for in-depth information and examples.
Example Apps: Riverpod's GitHub repository includes example apps that showcase various use cases and implementations.
Online Courses and Tutorials: For comprehensive learning, consider online courses and tutorials that dive deep into Riverpod and Flutter app development.
With Riverpod 2.0, you have a powerful state management solution at your fingertips. By mastering its core concepts and leveraging its advanced features, you can build efficient, maintainable, and robust Flutter applications. Whether you're a beginner or an experienced developer, Riverpod is a valuable addition to your Flutter toolkit.
Start your journey with Riverpod today and discover the difference it can make in your Flutter projects. Happy coding!
Subscribe to my newsletter
Read articles from Kamran Mansoor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
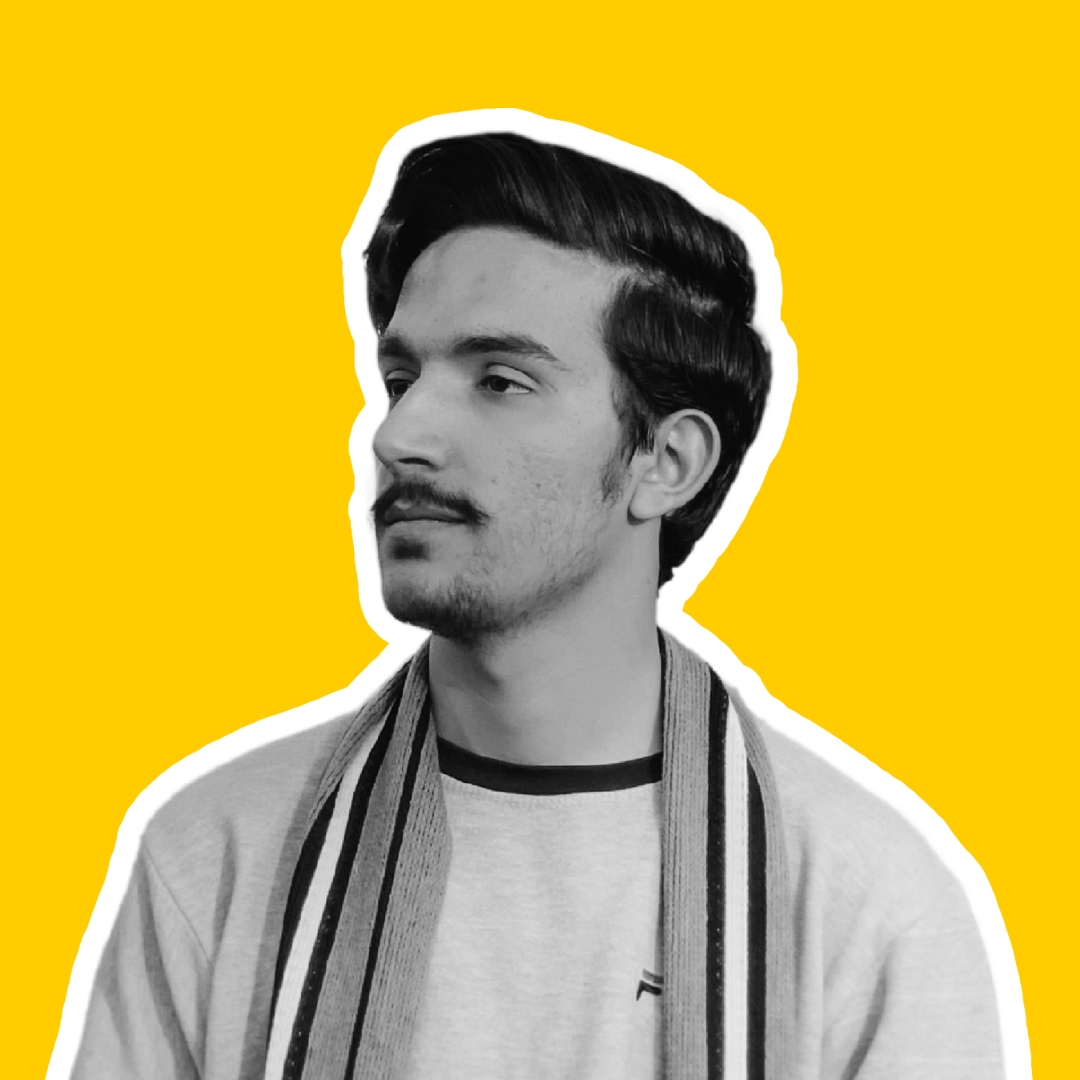
Kamran Mansoor
Kamran Mansoor
Student: Software Engineer. Junior Flutter Developer Data Scientist == Entrepreneur 🤞. COMSATS' 24. @kamran_hccp