Exploring the Power of Python: External and Built-in Modules
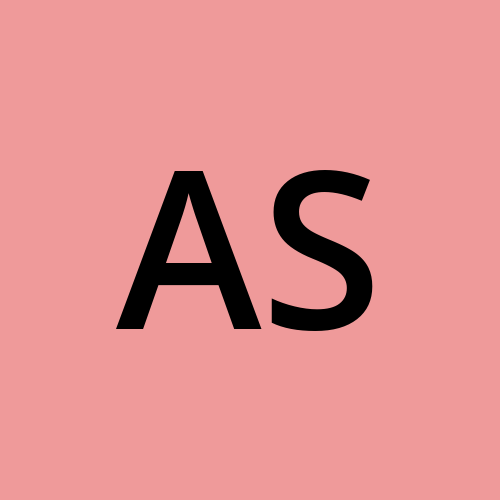
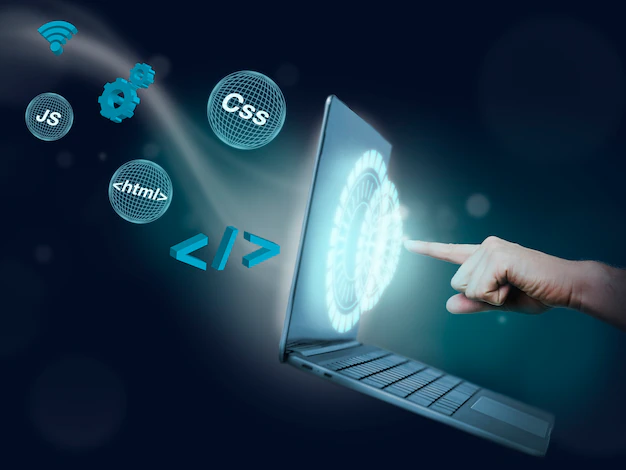
Python is a versatile and powerful programming language known for its simplicity and readability. One of the key reasons behind Python's success is its extensive ecosystem of modules and libraries, which provide a wealth of pre-written code to help you tackle a wide range of tasks. These modules can be categorized into two main types: external modules and built-in modules. In this blog post, we'll dive into both types, exploring their roles and how they can supercharge your Python projects.
Built-in Modules
Python comes with a rich set of built-in modules that are available for use without the need for any additional installation. These modules cover a wide range of functionalities, making them readily accessible and incredibly useful for developers.
1. math
The math
module is a fundamental built-in module that provides mathematical functions. You can use it for everything from basic arithmetic operations to more advanced calculations like trigonometry, logarithms, and complex numbers.
pythonCopy codeimport math
# Example: Calculate the square root of 25
result = math.sqrt(25)
print(result) # Output: 5.0
2. datetime
The datetime
module is indispensable for working with dates and times. It allows you to create, manipulate, and format date and time objects.
pythonCopy codeimport datetime
# Example: Get the current date and time
current_time = datetime.datetime.now()
print(current_time)
3. random
The random
module is your go-to tool for generating random numbers, selecting random elements from a sequence, and simulating randomness in your programs.
pythonCopy codeimport random
# Example: Generate a random number between 1 and 10
random_number = random.randint(1, 10)
print(random_number)
External Modules
While built-in modules provide a solid foundation, Python's true strength lies in its vast collection of external modules. These modules are created and maintained by the Python community and can be easily installed using package managers like pip
. Here are a few notable examples:
1. requests
The requests
library simplifies the process of making HTTP requests. It's widely used for web scraping, interacting with web APIs, and building web applications.
pythonCopy codeimport requests
# Example: Make an HTTP GET request
response = requests.get("https://www.example.com")
print(response.text)
2. Pandas
Pandas is a powerful library for data manipulation and analysis. It provides data structures like DataFrames and Series that make it easy to work with structured data.
pythonCopy codeimport pandas as pd
# Example: Create a DataFrame from a dictionary
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
3. Matplotlib
Matplotlib is a popular library for creating visualizations, including line plots, bar charts, and scatter plots. It's an essential tool for data scientists and analysts.
pythonCopy codeimport matplotlib.pyplot as plt
# Example: Create a simple line plot
x = [1, 2, 3, 4, 5]
y = [10, 15, 13, 18, 20]
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
Conclusion
Python's extensive ecosystem of external and built-in modules empowers developers to tackle a wide array of tasks efficiently. Whether you're working with mathematics, handling dates and times, scraping the web, analyzing data, or creating stunning visualizations, Python has the tools you need. By harnessing the power of these modules, you can streamline your development process and create more robust and feature-rich applications. So, the next time you embark on a Python project, don't forget to explore the vast world of Python modules to make your life as a developer easier and more enjoyable.
Subscribe to my newsletter
Read articles from Anurag Srivastava directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
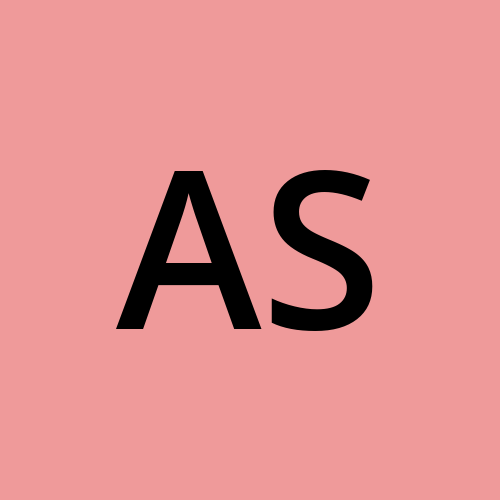