Dictionary
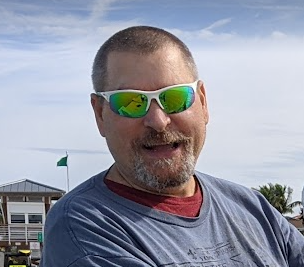
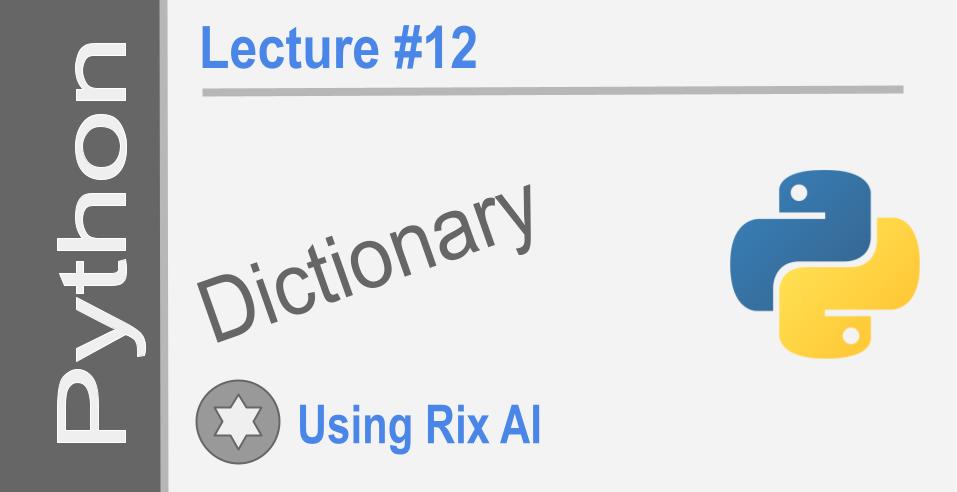
A hashmap, also known as a hash table, is a data structure that maps keys to values. It implements an associative array abstraction, a structure that can map keys to values. A hashmap uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found.
In Python, hashmaps are implemented using the dict object. Here is an example:
phonebook = {}
phonebook["John"] = 938477566
phonebook["Jack"] = 938377264
phonebook["Jill"] = 947662781
print(phonebook["John"])
# 938477566
Here we have created a phonebook dict that maps names to phone numbers. We add entries using square bracket notation, and lookup values using the key in square brackets.
The keys in a Python dict can be of any immutable type - strings, numbers or tuples - while the values can be of any type.
Under the hood, Python uses a hashtable to implement the dict, where the key is hashed to get the bucket index, and the value is stored with that key in that bucket. This allows for fast lookup, insertion and removal of key-value pairs.
Dict Literals
Here is the dictionary literal syntax in Python with examples:
# Empty dictionary
dict1 = {}
# Dictionary with integer keys
dict2 = {1: 'apple', 2: 'ball'}
# Dictionary with mixed keys
dict3 = {'name': 'John', 1: [2, 4, 3]}
# Dictionary with string keys
dict4 = {'key1' : 'value1', 'key2' : 'value2'}
# Preserve insertion order using OrderedDict
from collections import OrderedDict
dict5 = OrderedDict([('a', 1), ('b', 2), ('c', 3)])
As you can see, we use {key1: value1, key2: value2, ...} syntax to define a dictionary.
Keys can be of any immutable type - strings, numbers or tuples. Values can be any type.
We can have dictionaries with:
Integer keys
String keys
Mixed keys (integer and string both)
We can also use OrderedDict from the collections module to preserve the insertion order of keys. This is useful when the order of keys matter.
Some other points:
Keys must be unique within the dictionary, values need not be.
Values can be accessed using the key - dict1[key]
We can check if a key exists using key in dict syntax.
Basics
Here are some basic operations you can perform on a dictionary in Python:
- Access a value using a key:
dict = {'name': 'John', 'age': 30, 'city': 'New York'}
print(dict['name'])
# John
- Update a value:
dict['age'] = 31
- Add a new key-value pair:
dict['gender'] = 'Male'
- Delete a key-value pair:
del dict['city']
- Get all keys:
print(dict.keys())
# dict_keys(['name', 'age', 'gender'])
- Get all values:
print(dict.values())
# dict_values(['John', 31, 'Male'])
- Check if a key exists:
'name' in dict # True
'address' in dict # False
- Get length of the dictionary:
print(len(dict))
# 3
- Copy a dictionary:
dict2 = dict.copy()
- Clear the dictionary:
dict.clear()
So in summary, the basic operations you can perform on a dictionary are: accessing values, updating values, adding new key-value pairs, deleting items, getting keys/values, checking if a key exists, getting the length and copying/clearing the dictionary.
Iteration
There are a couple of ways to iterate over a dictionary in Python:
- Using the .items() method:
dict1 = {'a': 1, 'b': 2, 'c': 3}
for key, value in dict1.items():
print(key, value)
# a 1
# b 2
# c 3
This iterates over each (key, value) pair in the dictionary.
- Using the .keys() method:
for key in dict1.keys():
print(key, dict1[key])
# a 1
# b 2
# c 3
This iterates over the keys, and you lookup the value using the key.
- Using the .values() method:
for value in dict1.values():
print(value)
# 1
# 2
# 3
This iterates over the values alone.
- Using the list() method to iterate over the keys:
for key in list(dict1.keys()):
print(key, dict1[key])
# a 1
# b 2
# c 3
This converts the keys to a list and then iterates.
So in summary, .items() is the most comprehensive as it iterates over the (key, value) pairs directly. .keys() and .values() iterate over just the keys or values respectively.
Comprehension
Dictionary comprehensions were introduced in Python 2.7 as a way to build dictionaries in a concise manner similar to how list comprehensions work.
The general syntax is:
{key:value for (key, value) in iterable}
For example, to create a dictionary that maps each letter to its ASCII code:
ascii_codes = {letter: ord(letter) for letter in 'abcde'}
print(ascii_codes)
# {'a': 97, 'b': 98, 'c': 99, 'd': 100, 'e': 101}
Here we iterate over each letter in 'abcde' and map it to its ASCII code using ord().
We can also apply conditions to the dictionary comprehension:
even_numbers = {num: num*2 for num in range(10) if num % 2 == 0}
print(even_numbers)
# {0: 0, 2: 4, 4: 8, 6: 12, 8: 16}
Here we only include numbers that are even (num % 2 == 0).
The key and value expressions can be any arbitrary Python expression:
squares = {num: num**2 for num in range(10)}
print(squares)
# {0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
So in summary, dictionary comprehensions provide a concise way to build dictionaries programmatically from other sequences or iterables, applying conditions and calculations as needed.
Decomposition
Decomposition is the process of breaking down a complex data structure into simpler parts. This helps make the data structure easier to understand, use and modify.
For dictionaries in Python, decomposition involves breaking the dictionary down into its constituent keys and values. This can be done in a few ways:
- Using the .keys() and .values() methods:
dict = {'a': 1, 'b': 2, 'c': 3}
keys = dict.keys()
values = dict.values()
print(keys) # dict_keys(['a', 'b', 'c'])
print(values) # dict_values([1, 2, 3])
- Unpacking the .items() method into separate variables:
dict = {'a': 1, 'b': 2, 'c': 3}
keys, values = zip(*dict.items())
print(keys) # ('a', 'b', 'c')
print(values) # (1, 2, 3)
- Looping over the .items() and storing in separate lists:
dict = {'a': 1, 'b': 2, 'c': 3}
keys = []
values = []
for k, v in dict.items():
keys.append(k)
values.append(v)
print(keys) # ['a', 'b', 'c']
print(values) # [1, 2, 3]
Decomposing the dictionary into its keys and values allows you to manipulate them independently. For example, you can sort the keys, filter the values, perform set operations on the keys, etc.
So in summary, decomposition involves breaking down a complex data structure (like a dictionary) into simpler parts (keys and values) to make it easier to manipulate and modify programmatically.
Use Cases
Here are some common use cases for dictionaries in Python:
- Mapping unique keys to values: This is the most basic use case. Mapping an ID, name or other identifier to some data. For example:
Mapping student IDs to student records
Mapping employee IDs to employee details
Mapping product codes to product names
- Representing data with attributes: Dictionaries can be used to represent objects or entities with multiple attributes. For example:
student = {
'name': 'John',
'age': 20,
'grades': [80, 90, 95]
}
Storing configuration settings: Dictionaries are useful for storing configuration settings for an application. The keys represent the config options and the values are the settings.
Caching data: Dictionaries can be used as an in-memory cache to store data that needs to be accessed quickly. The keys can be used as the lookup identifiers.
Representing JSON data: Since JSON (JavaScript Object Notation) format is similar to Python dictionaries, dictionaries can be used to represent JSON data in Python.
Implementing simple associative arrays: Dictionaries can be used like associative arrays in other languages, where you can lookup values using keys instead of indices.
Storing data from a database: The rows returned from a database can be mapped to a dictionary where each column is a key and the value is the data from that column.
So in summary, dictionaries are useful in a variety of scenarios where you need to map unique keys to values. Some of the most common use cases include representing objects, storing configurations, caching data, and representing JSON data.
Features
Here are some strengths and weaknesses of dictionaries in Python:
Strengths:
Fast lookup/access time - Since dictionaries are implemented as hash tables, the average time to lookup a value is O(1). This makes dictionaries very fast and efficient.
Flexible keys - Dictionaries can use almost any type as a key, including strings, numbers, and tuples. This makes them very flexible.
Useful for representing objects - Dictionaries can be used to represent objects with attributes, since they allow storing multiple key-value pairs.
Easy to use and understand - Dictionaries have a simple and intuitive syntax that is easy to use and understand.
Weaknesses:
Unordered - Dictionaries do not maintain the insertion order of elements. This can be an issue if you need an ordered map.
Mutable - Since dictionaries are mutable, passing them to functions may result in unexpected behavior if the function modifies the dictionary.
Key collisions - If two keys map to the same hash value, there will be a key collision which can impact performance.
Memory usage - Since dictionaries store keys and values separately, they tend to use more memory compared to lists.
No duplicate keys - Dictionaries cannot have duplicate keys, so if you insert the same key again, the old value will be overwritten.
In summary, the main strengths of dictionaries are the fast lookup time, flexibility of keys, and ability to represent objects. The weaknesses include the unordered and mutable nature, potential for key collisions, higher memory usage and inability to have duplicate keys.
So whether dictionaries are suitable for your use case will depend on factors like performance requirements, mutability, ordering needs and memory constraints. But overall, dictionaries are a very useful and versatile data type in the Python repertoire.
Disclaim: This article is created with Rix AI. I have used about 5 prompts to explain the best I can this data type. If you have more questions comment below.
Subscribe to my newsletter
Read articles from Elucian Moise directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
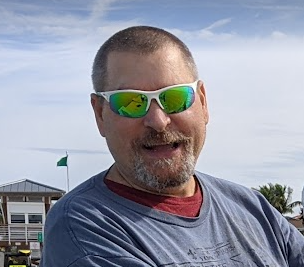
Elucian Moise
Elucian Moise
Software engineer instructor, software developer and community leader. Book author. Computer enthusiast and experienced programmer. Born in Romania, living in US.