Exploring the Web: Scraping Website Data with Python
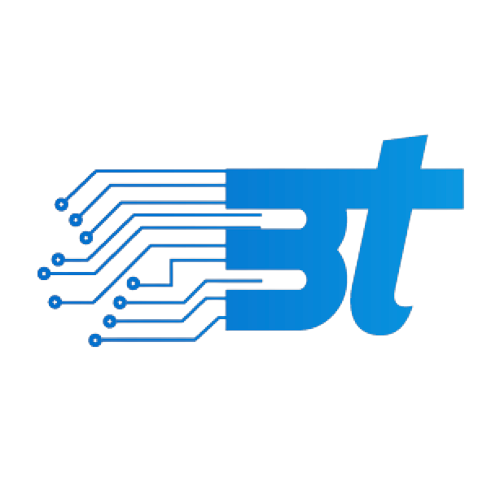
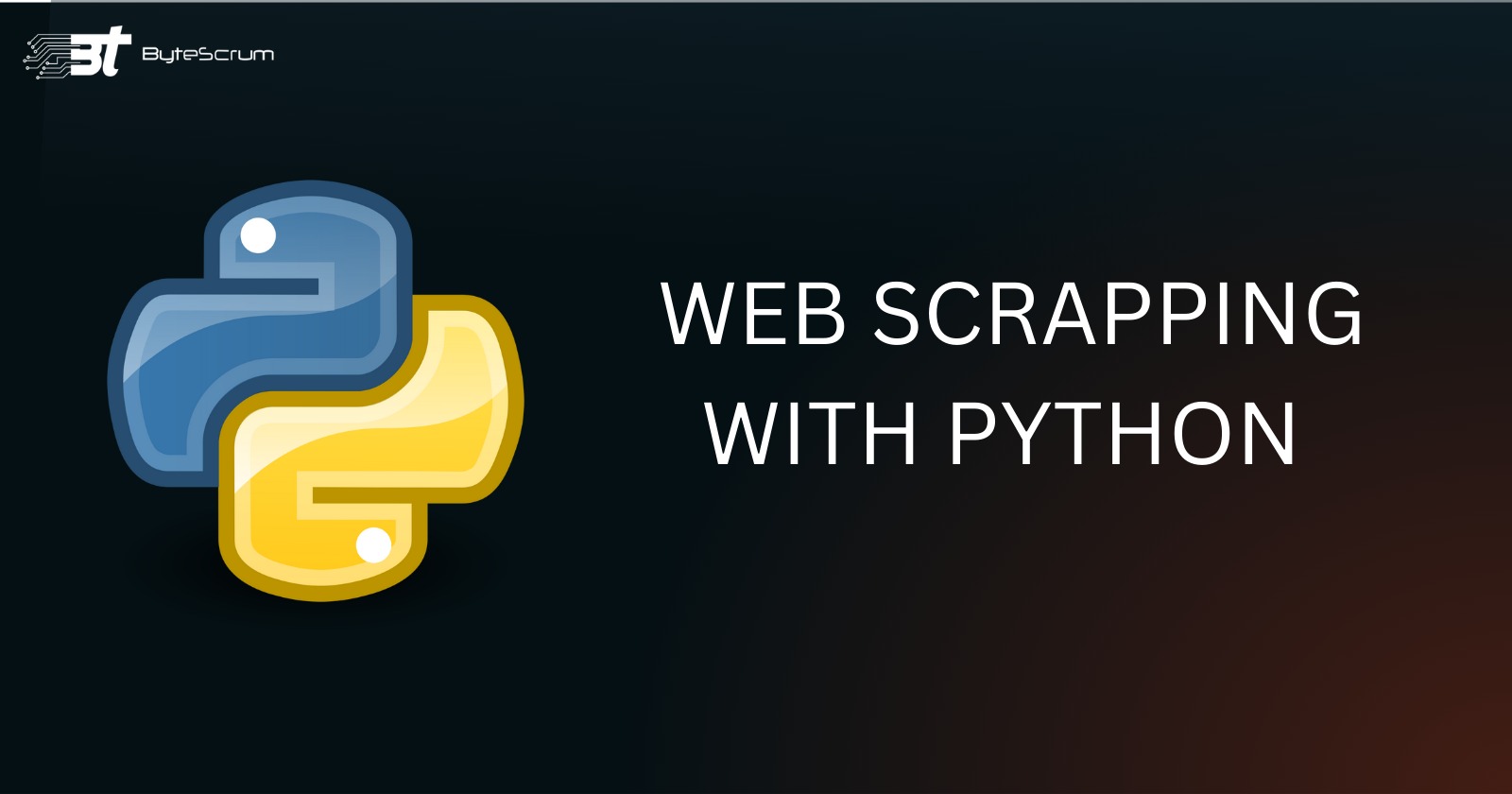
In today's digital age, the web is a treasure trove of information. Websites contain a wealth of data, and sometimes, you might want to extract specific information from them. Python provides a powerful and versatile library called BeautifulSoup for web scraping, and this blog will guide you through the process. We'll use Python to scrape a website and extract email addresses, phone numbers, metadata, and social media links. Let's get started!
Introduction to Web Scraping
Web scraping is the process of extracting data from websites. It's a valuable technique for various purposes, from data analysis to research and automation. In this blog, we'll use Python to scrape a website and extract specific types of information.
Setting Up Your Environment
Before we dive into web scraping, you need to set up your Python environment. Make sure you have Python installed, and install the required libraries using pip:
pip install requests beautifulsoup4
The Python Code
Here's a Python code snippet that scrapes a website and extracts email addresses, phone numbers, metadata, and social media links. You can use this code as a starting point for your web scraping projects.
import requests
from bs4 import BeautifulSoup
import re
# Function to extract emails using regex
def extract_emails(text):
return re.findall(r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,7}\b', text)
# Function to extract phone numbers using regex
def extract_phone_numbers(text):
return re.findall(r'\b(?:\d{3}[-.\s]?)?\d{3}[-.\s]?\d{4}(?:\s?ext\s?\d+)?\b', text)
# Function to extract meta data
def extract_meta_data(soup):
title = soup.find('title').get_text() if soup.find('title') else ""
meta_keywords = soup.find('meta', {'name': 'keywords'})
meta_keywords = meta_keywords["content"] if meta_keywords else ""
meta_description = soup.find('meta', {'name': 'description'})
meta_description = meta_description["content"] if meta_description else ""
return title, meta_keywords, meta_description
# Function to extract social media links
def extract_social_media_links(soup):
social_links = []
social_media_tags = soup.find_all('a', href=re.compile(r"facebook|twitter|linkedin|instagram"))
for tag in social_media_tags:
social_links.append(tag.get('href'))
return social_links
# URL of the website to scrape
url = "https://www.bytescrum.com" # Replace with the URL of the website you want to scrape
# Send an HTTP GET request
response = requests.get(url)
# Check if the request was successful
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
# Extract unique email addresses and phone numbers
email_addresses = list(set(extract_emails(response.text)))
phone_numbers = list(set(extract_phone_numbers(response.text)))
# Extract meta data
title, meta_keywords, meta_description = extract_meta_data(soup)
# Extract social media links
social_media_links = extract_social_media_links(soup)
# Display the extracted data
print("Email Addresses:", email_addresses)
print("Phone Numbers:", phone_numbers)
print("Title:", title)
print("Meta Keywords:", meta_keywords)
print("Meta Description:", meta_description)
print("Social Media Links:", social_media_links)
else:
print(f"Failed to retrieve the web page. Status code: {response.status_code}")
// output
Email Addresses: ['info@bytescrum.com', 'support@bytescrum.com']
Phone Numbers: ['601-4311', '7607815580']
Title: Top IT Company: Web, Mobile & Blockchain Solutions
Meta Keywords: web development, mobile app development, blockchain development, Laravel development, WordPress, React, website security, website recovery
Meta Description: ByteScrum Technologies - Leading IT company in USA, Canada, and the Netherlands for web, mobile, and blockchain solutions
Social Media Links: ['https://www.facebook.com/bytescrum', 'https://twitter.com/bytescrum', 'https://www.linkedin.com/company/bytescrum/', 'https://www.instagram.com/bytescrum/']
Code Breakdown
We start by importing the necessary libraries:
requests
for making HTTP requests andBeautifulSoup
for parsing HTML.The code defines four functions to extract different types of data: email addresses, phone numbers, metadata, and social media links. These functions use regular expressions and BeautifulSoup to locate and extract the data.
You should replace the
url
variable with the URL of the website you want to scrape.The code sends an HTTP GET request to the specified URL and checks if the request was successful (status code 200). If successful, it parses the HTML content using BeautifulSoup.
The extracted data is stored in variables and then displayed on the screen.
Legal and Ethical Considerations
While web scraping is a powerful tool, it's important to be aware of the legal and ethical implications. Always review a website's terms of service and privacy policy to ensure compliance. Avoid aggressive scraping that might overload a server and disrupt a website's normal operation.
Summary
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
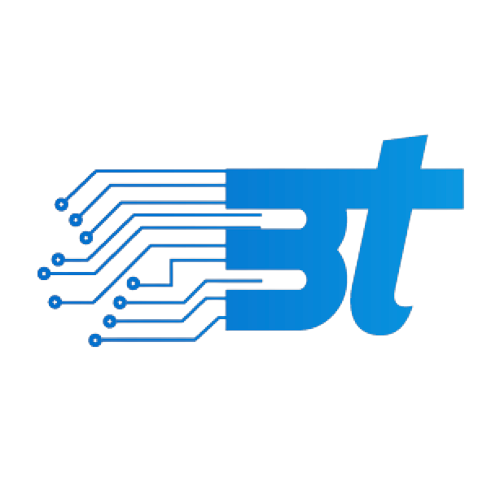
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.