Software Engineer Top 10 Coding Principles
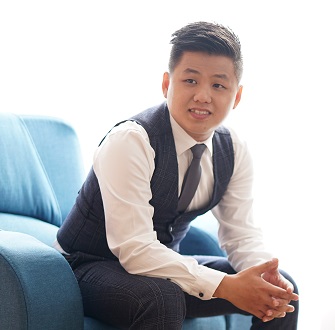
Table of contents
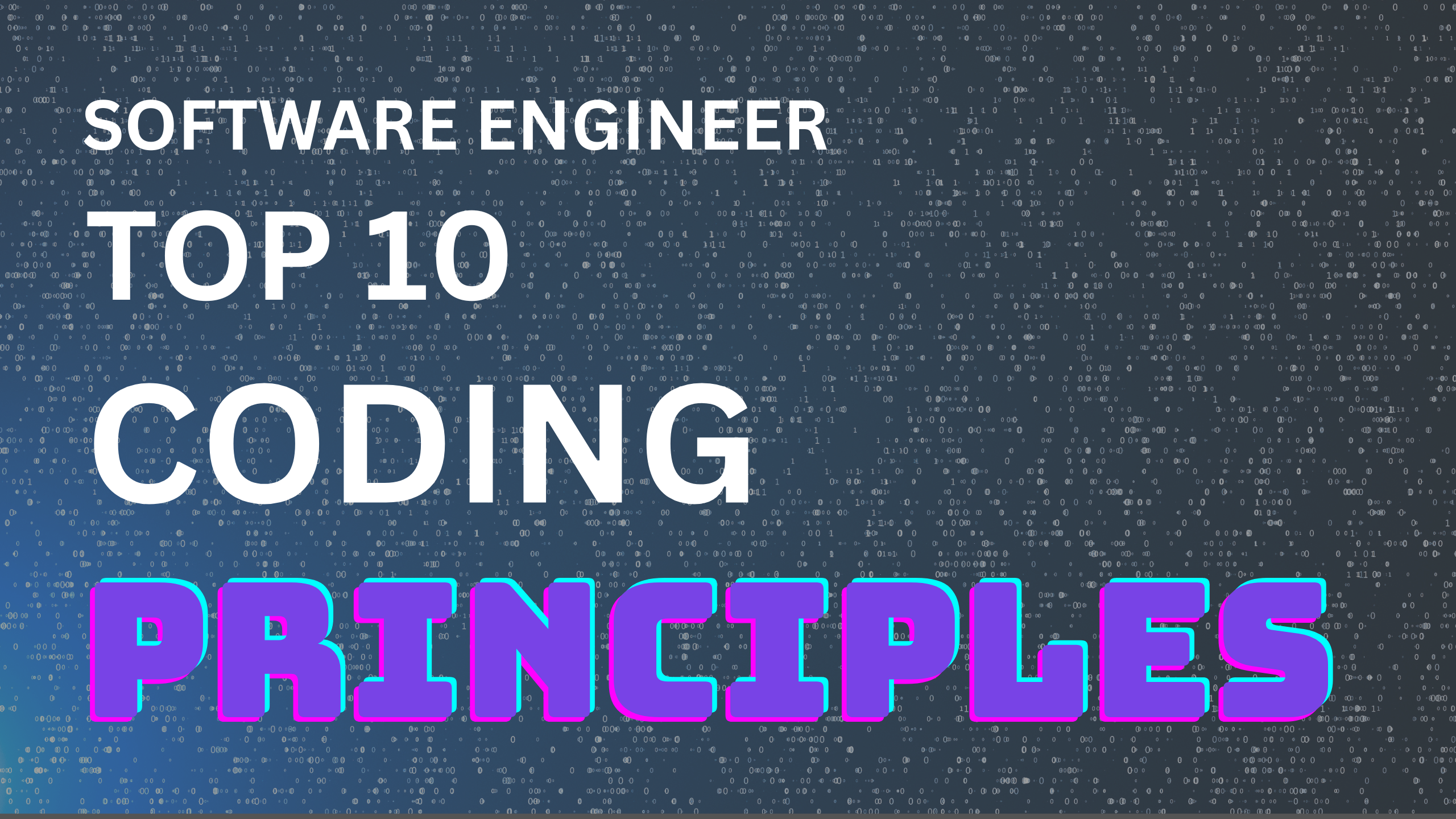
Many junior software engineers tend to dive headfirst into coding without considering any coding principles. This often results in unstandardized, messy code or leads to the proliferation of redundant code. It's a common experience, one that I've personally gone through, and it's shared by many in the software development community.
But what are coding principles, and why are they important? According to Wikipedia, "Coding best practices are a set of informal rules that the software development community employs to help improve software quality." While it's described as informal, I believe it should be a formal rule within any software development organization. These best practices serve as a guideline for code reviews, particularly for junior software engineers, ensuring that the code adheres to high standards.
Initially, it might seem like a waste of time to apply coding principles, especially when deadlines are looming. However, the long-term benefits are clear: clean, maintainable, and scalable code. In this article, we'll explore the top 10 coding principles, dividing them into two parts. The first part, covering principles 1 to 5, focuses on general usage principles. The second part, principles 6 to 10, delves into the famous Object-Oriented Programming (OOP) SOLID principles by Robert C. Martin, also known as Uncle Bob.
General Usage Principles:
1. KISS (Keep it Simple, Stupid)
The KISS principle may have a somewhat silly acronym, but it carries a profound message. As engineers, we sometimes overcomplicate our code, perhaps to showcase our skills or due to a lack of pre-coding planning. Simplicity in code is key. The simpler your code is, the more accessible it is for others to understand and maintain in the future, sparing you from blame for creating overly complex code.
2. DRY (Don't Repeat Yourself)
As mentioned in the introduction, redundant code is a common problem in software development. We often copy code to speed up work, leading to duplicated code scattered throughout the codebase. The DRY principle advocates for encapsulation and reusability through functions, methods, or components. However, be cautious not to overuse this principle to the extent that your code becomes overly fragmented, violating the KISS principle.
3. YAGNI (You Aren't Gonna Need It)
YAGNI reminds us not to clutter the codebase with unused or unnecessary code. Avoid the temptation to include code you think might be needed in the future. If it's essential, store it separately. Eliminate any used but non-essential code to keep your codebase straightforward.
4. BDUF (Big Design Up Front)
BDUF has a controversial reputation as it may appear contrary to agile principles. However, it doesn't advocate for creating a final, unchangeable design upfront. Instead, it emphasizes having a clear design, such as a diagram or a list of components, to prevent poorly structured code and improve time and resource estimation. While agile development encourages incremental shipping and iterative improvement, some upfront design can save time and effort in the long run.
5. SoC (Separation Of Concern)
Separating your code into clear sections or domains, where each section handles a specific area, reduces coupling and enhances cohesion. This principle aligns with design patterns like model-view-controller (MVC) and microservices, emphasizing the separation of concerns between data (Model), UI (View), and logic (Controller). It's a fundamental principle that underpins two of the SOLID principles, Single Responsibility and Interface Segregation, which we'll discuss later.
SOLID Principles by Robert C. Martin:
6. Single Responsibility Principle (SRP)
A class should have only one reason to change, meaning it should have a single responsibility. Avoid creating "God Classes" that try to do everything. Adhering to SRP results in better software, making it easier to unit test, reducing coupling, and facilitating class organization.
7. Open/Closed Principle
Software entities should be open for extension but closed for modification. Instead of modifying existing classes, extend them to accommodate new requirements. This approach minimizes potential bugs arising from class modifications.
8. Liskov Substitution Principle (LSP)
The Liskov Substitution Principle (LSP) is the most complex of the SOLID principles. It dictates that when extending a class, the child class should be able to function as the parent class without breaking its behavior. In other words, the child class should not introduce unexpected changes or issues, ensuring a consistent design.
9. Interface Segregation
Avoid creating general-purpose interfaces and opt for smaller, client-specific interfaces tailored to their needs. This prevents clients from being burdened with unnecessary methods, adhering to the "many client-specific interfaces" principle.
10. Dependency Inversion (DI)
The Dependency Inversion Principle consists of two parts: high-level modules should not depend on low-level modules, and abstractions should not depend on details. Instead, both should depend on abstractions, improving code flexibility and maintainability.
In conclusion, implementing these principles may initially seem challenging, with their value not immediately apparent in small codebases. However, as your application grows, you'll find that adhering to these principles results in cleaner, more scalable, and more maintainable code.
Thank you for reading, and I hope you've found this article valuable. If you like it please subscribe as in the future I am planning to create more articles that explain all the top 10 principles. Cheers!
Subscribe to my newsletter
Read articles from Then directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
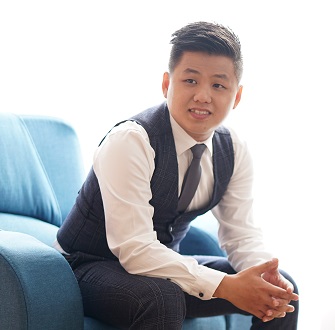
Then
Then
I am a software engineer with experience as a tech lead.