Mastering Go's Control Flow and Functions: Your Path to Gopher Greatness
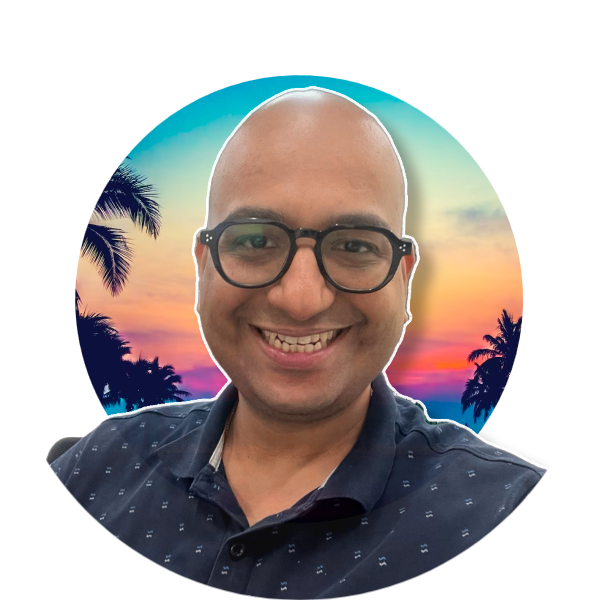
Table of contents
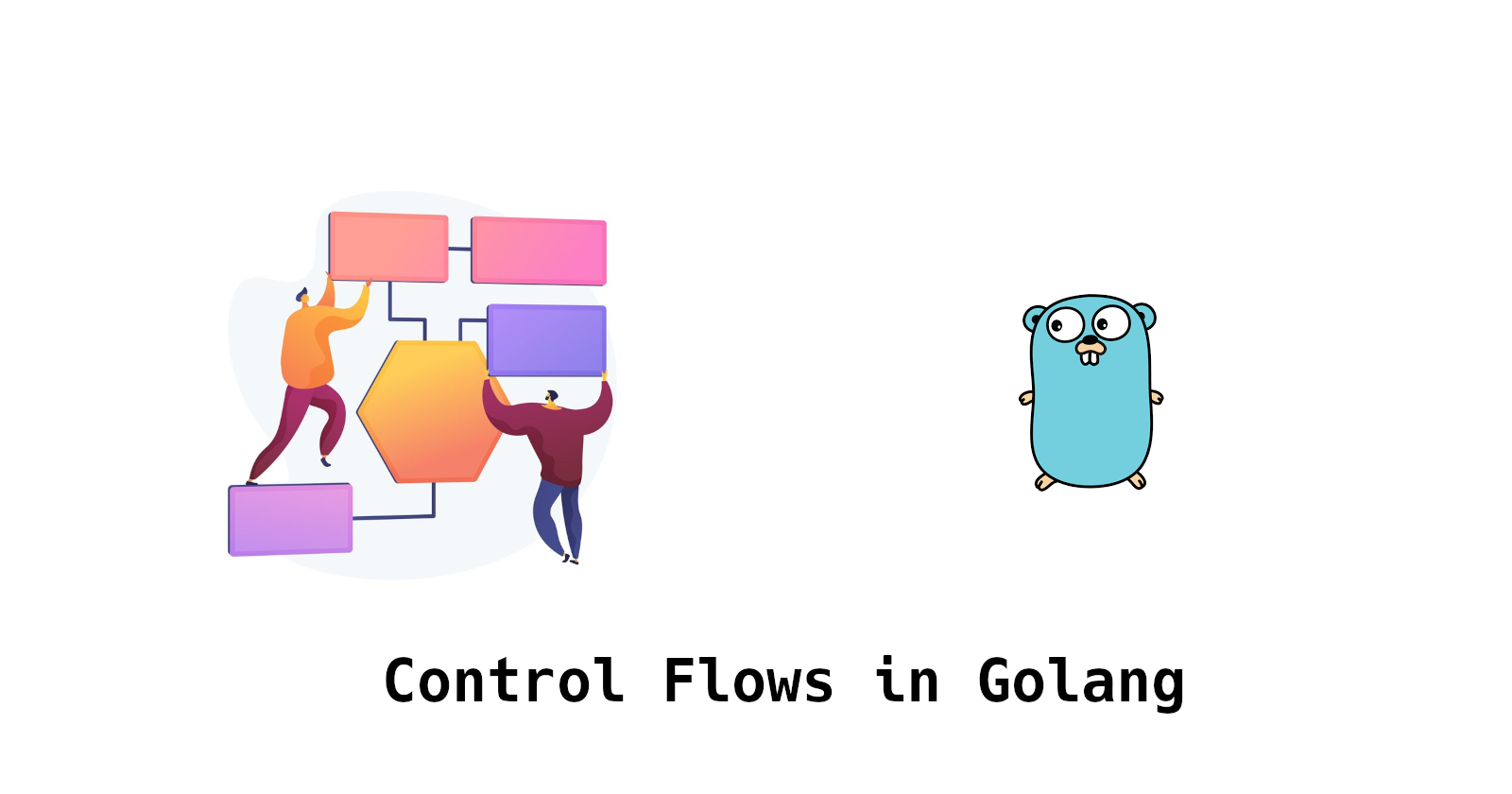
Introduction
Welcome to the world of Go's control structures and functions! In this guide, we'll dive deep into the essential aspects of programming with Go, including control flow structures (if, for, switch) and the versatility of functions. These are the tools that empower developers to create dynamic and responsive Go applications. Whether you're a seasoned Python developer or new to coding, Go's elegant approach will captivate you.
Simpler explanation
Control structures in Go are like traffic signals for your code, guiding it through decision-making and looping. Functions are like magic spells you can cast to perform specific tasks whenever you need them.
Sample Code:
Control Structures
package main
import "fmt"
func main() {
// If-Else control structure
num := 10
if num%2 == 0 {
fmt.Println("Even")
} else {
fmt.Println("Odd")
}
// For loop
for i := 1; i <= 5; i++ {
fmt.Println(i)
}
// Switch control structure
fruit := "apple"
switch fruit {
case "apple":
fmt.Println("It's an apple.")
case "banana":
fmt.Println("It's a banana.")
default:
fmt.Println("It's something else.")
}
}
Functions in Action
package main
import "fmt"
// Define a simple function
func greet(name string) string {
return "Hello, " + name + "!"
}
func main() {
// Call the greet function
message := greet("Gopher")
fmt.Println(message)
}
We demonstrate if-else for decision-making, a for loop for iteration, and a switch statement for multi-way branching.
In the function example, we define a
greet
function that takes a name and returns a greeting message.
Reference Links
Conclusion
You've now embarked on a journey to master Go's control structures and functions, crucial elements in building dynamic and efficient applications. Control structures help you make decisions and repeat actions, while functions enable you to encapsulate logic for reuse. Armed with these tools, you're well-prepared to tackle complex programming tasks and create elegant Go code like a true Gopher! Keep exploring and experimenting to hone your skills further.
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
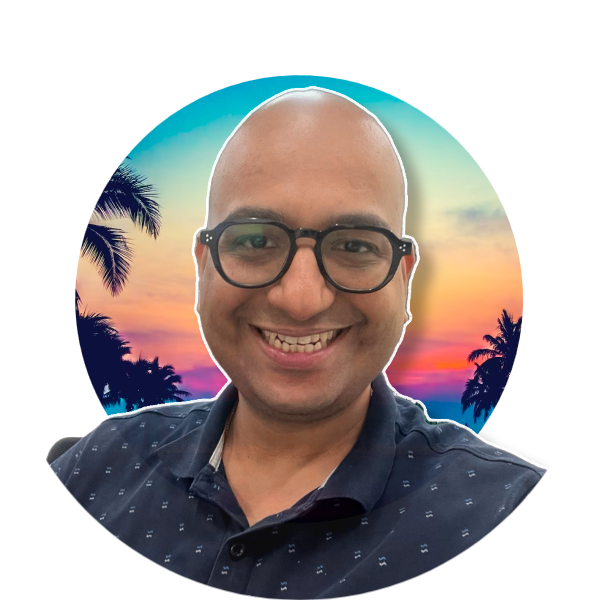
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.