Day 5 - Command Line Args and Environment Variables in Python
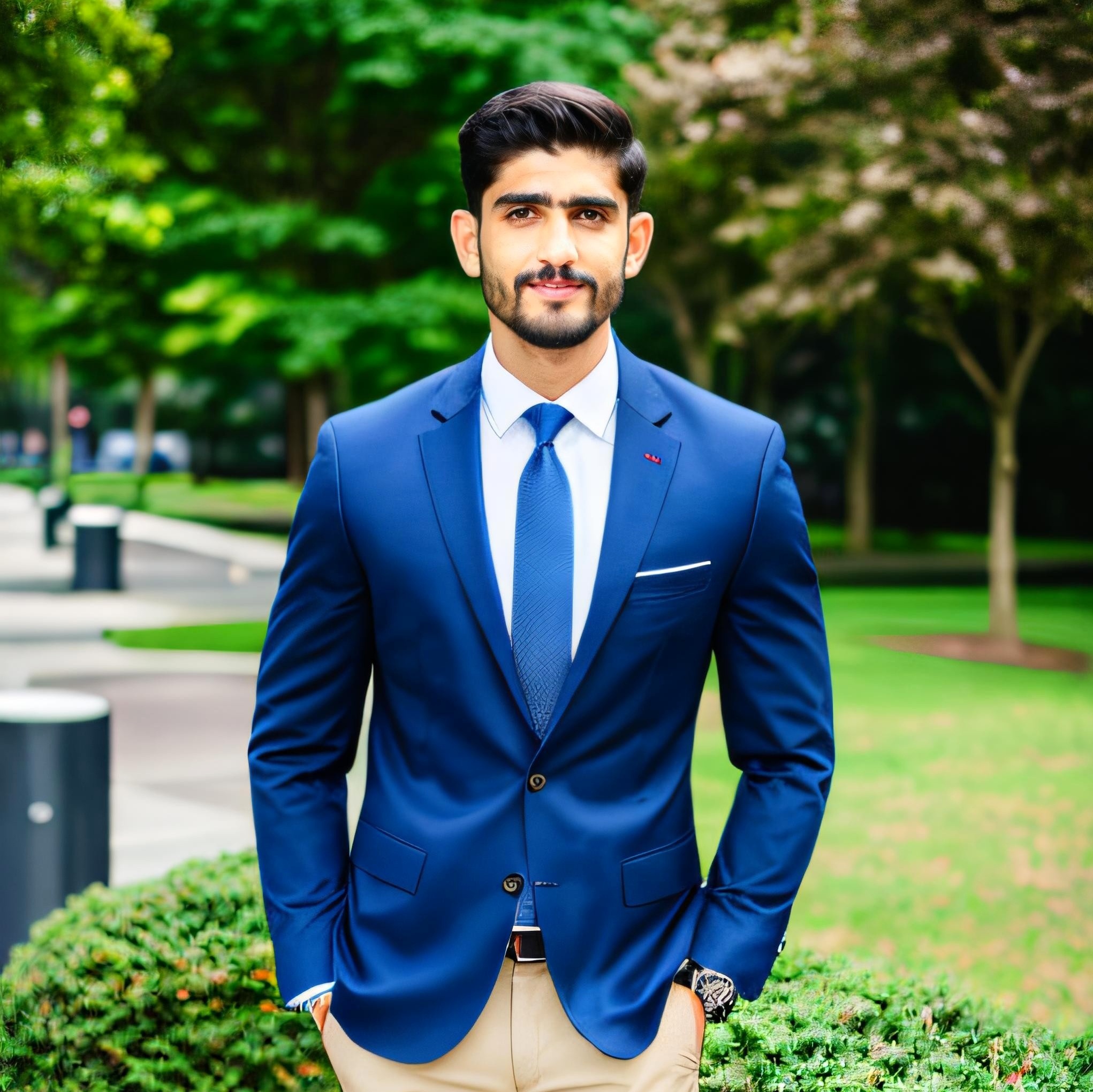
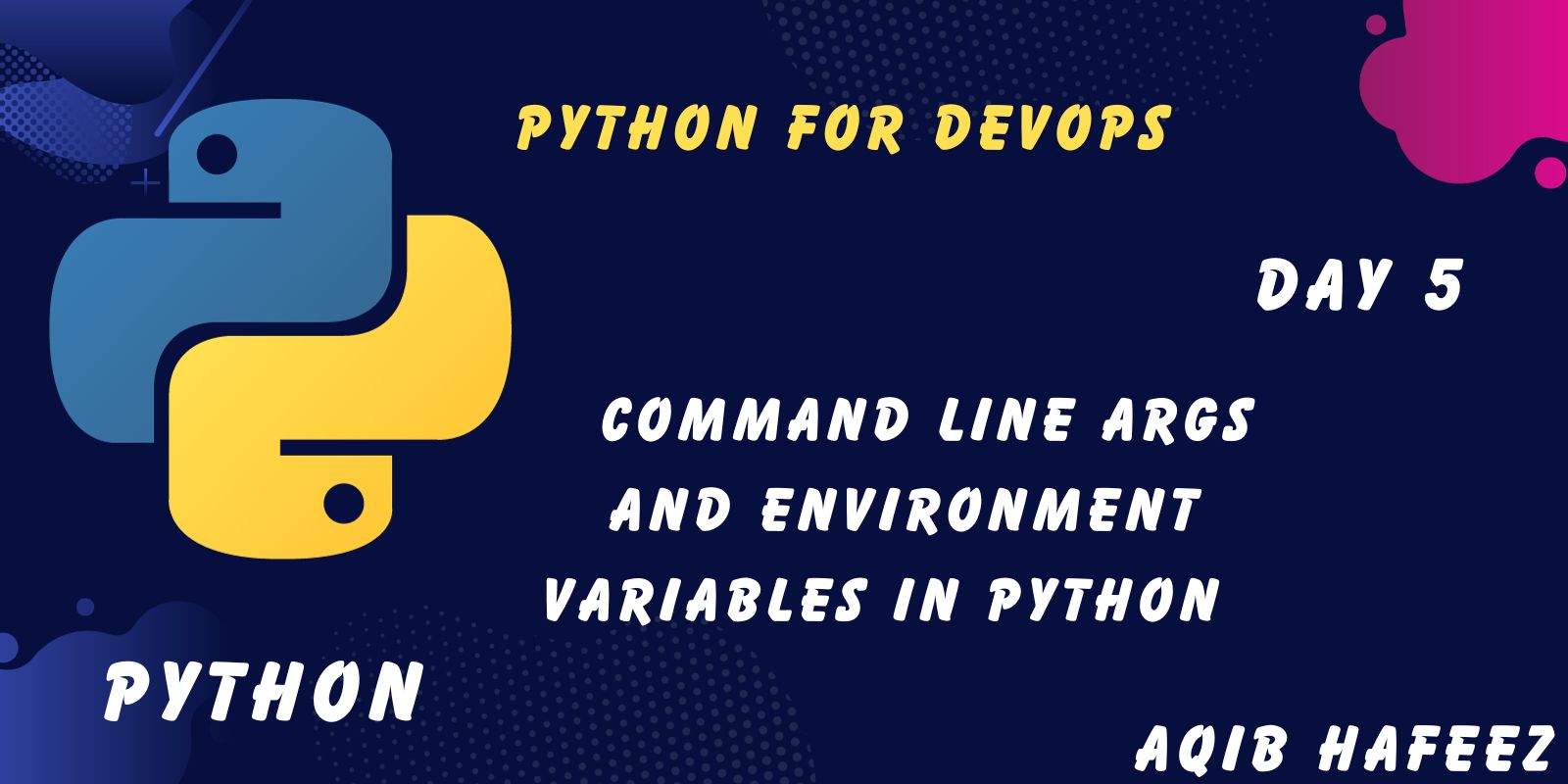
Introduction:
Welcome to Day 5 of our Python journey! In this article, we'll explore a fundamental aspect of Python programming: handling command-line arguments and environment variables. These are essential tools for building versatile and configurable applications. Let's break it down step by step.
Step 1: Setting the Stage
Before diving into command line arguments and environment variables, make sure you have Python installed on your system. You can download Python from the official website (python.org).
Step 2: What Are Command Line Arguments?
Command line arguments are parameters passed to a Python script when it's executed from the terminal. These arguments allow you to customize the behavior of your script without modifying its source code.
Here's how you can access command line arguments:
import sys
# Get the script name
script_name = sys.argv[0]
# Get additional command line arguments
arguments = sys.argv[1:]
Step 3: Parsing Command Line Arguments
To make command-line argument handling more user-friendly, you can use libraries like argparse
. This library simplifies argument parsing, provides help messages, and enforces argument types.
import argparse
parser = argparse.ArgumentParser(description="A simple Python script with command line arguments.")
parser.add_argument("--input", help="Input file")
parser.add_argument("--output", help="Output file")
args = parser.parse_args()
input_file = args.input
output_file = args.output
Step 4: Environment Variables
Environment variables are global variables that can be accessed by all processes running on your system. Python allows you to access and manipulate environment variables easily using the os
module.
To access an environment variable:
import os
my_variable = os.environ.get("MY_VARIABLE")
Step 5: Setting Environment Variables
You can also set environment variables within your Python script:
import os
os.environ["MY_VARIABLE"] = "Hello, World!"
Step 6: Running Your Python Script
Now, let's put it all together. Save your Python script (let's call it script.py
) with the command line argument and environment variable code we discussed.
To run your script with command line arguments:
python script.py --input input.txt --output output.txt
To access an environment variable in your script:
import os
my_variable = os.environ.get("MY_VARIABLE")
print(my_variable)
Step 7: Conclusion
In this article, we've covered the basics of working with command-line arguments and environment variables in Python. These concepts are crucial for building versatile and configurable applications that can adapt to different scenarios.
As you continue your Python journey, you'll find many use cases for command-line arguments and environment variables, from creating user-friendly command-line tools to configuring application settings.
Keep exploring and experimenting, and in no time, you'll become a Python pro. Happy coding!
Subscribe to my newsletter
Read articles from AQIB HAFEEZ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
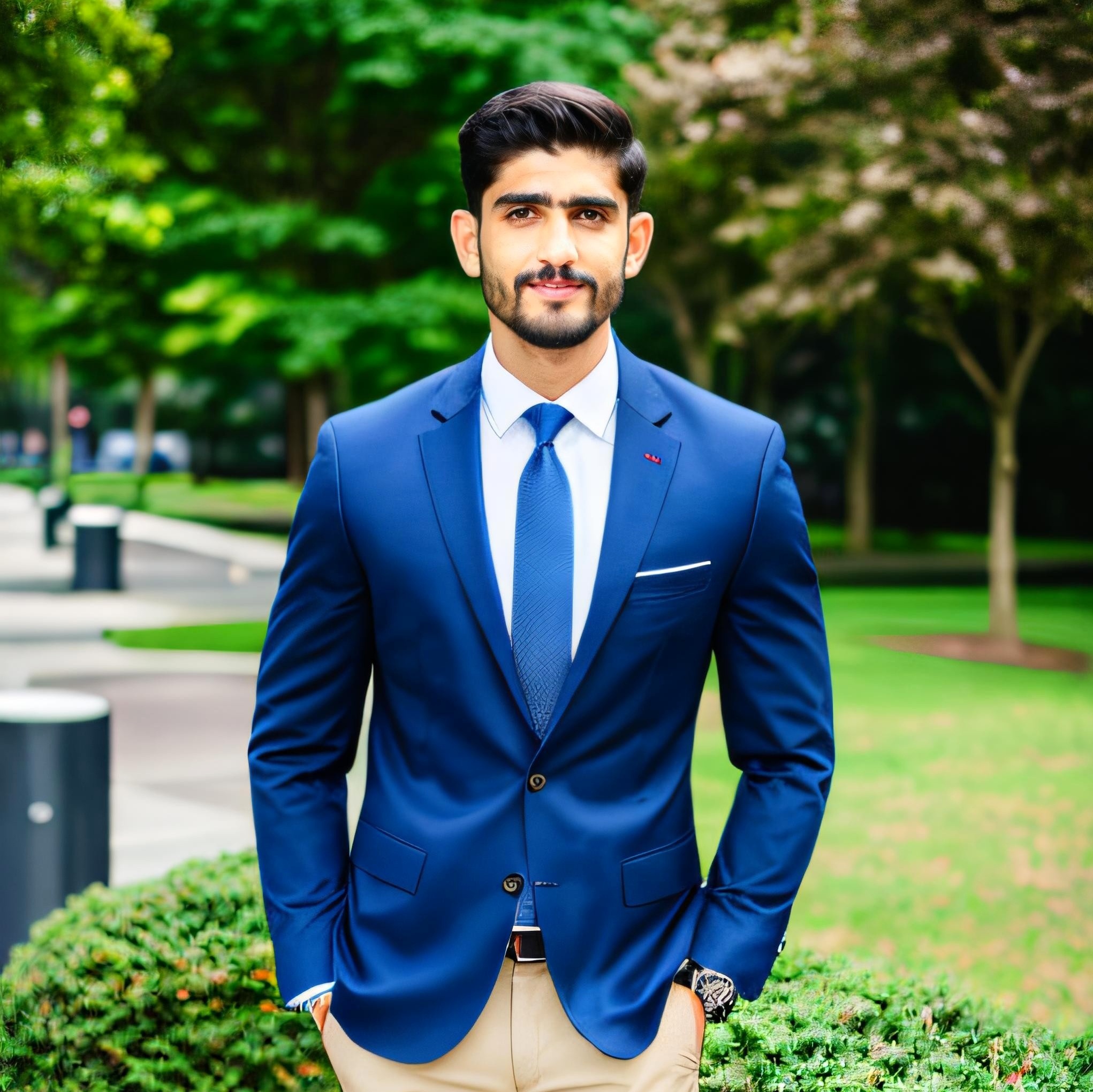
AQIB HAFEEZ
AQIB HAFEEZ
DevOps Engineer, Linux, Git, GitHub, GitLab, CI/CD pipelines, Jenkins, Docker, Kubernetes, Ansible & AWS. Practical experience in these DevOps tools enhances my passion for streamlined workflows, automated processes, and holistic development solutions. Proficient in digital and Facebook marketing, aiming to merge technical acumen with marketing finesse.