Getting Started with ngx-translate: Installation and Usage
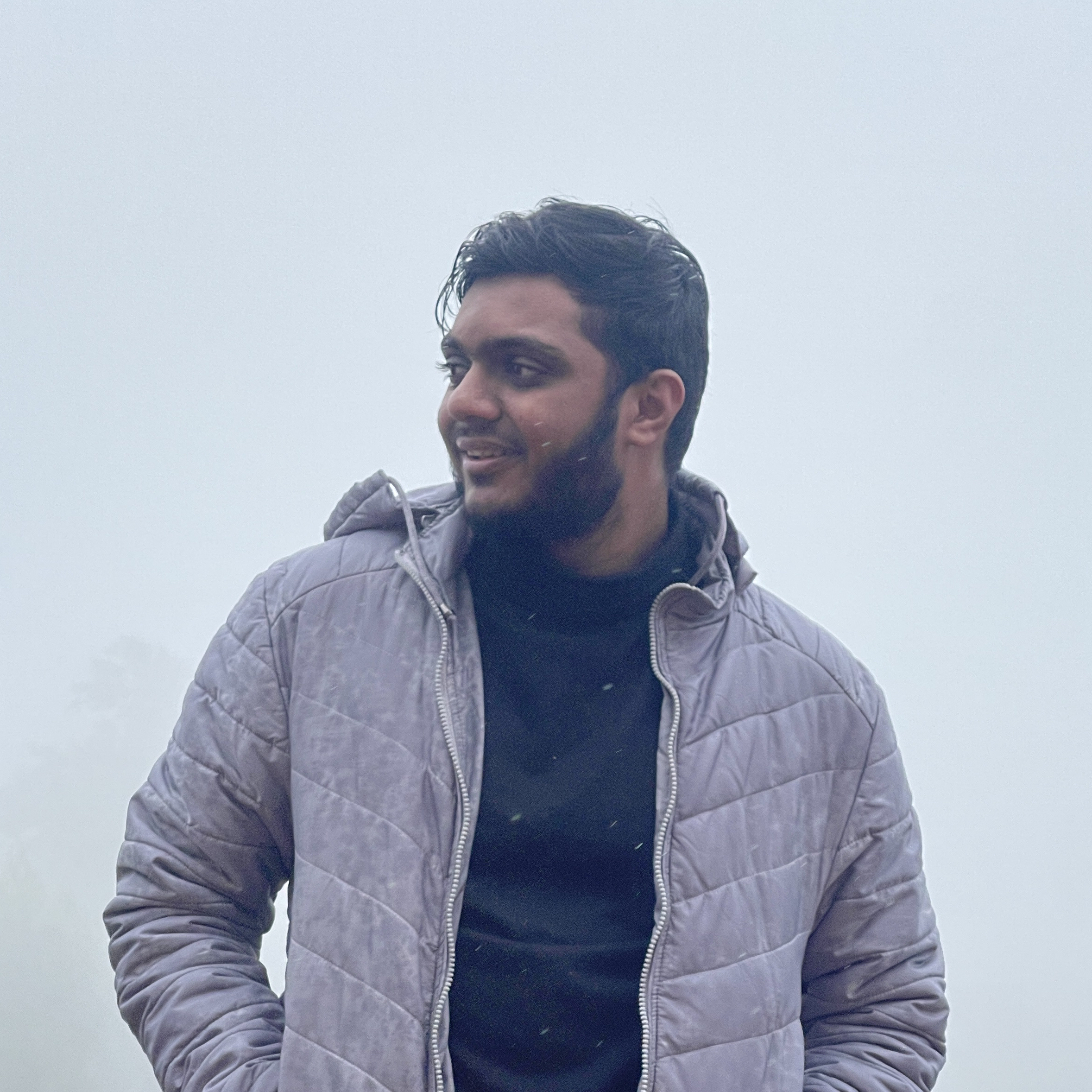
Table of contents
- What is ngx-translate?
- Why is ngx-translate essential for our app?
- Step 1: Create your Angular project
- Step 2:Install the ngx-translate library
- Step 3:Integration of the translation module in our code
- Step 4: Setting Up Components and Language Handling in app.component.ts
- Step 5: Creating the JSON translation files.

What is ngx-translate?
Ngx-translate is a library used for internationalization (i18n) and localization (i10n) in Angular applications.
This allows users to change the language of the applications at run time without the need to reload the whole app.
Ngx-translate makes it easier for the developers to allow their app to support multiple languages.
Why is ngx-translate essential for our app?
Global Accessibility: ngx-translate is crucial to ensure our app is accessible to a global audience, irrespective of language barriers.
Enhanced User Experience: It provides a seamless user experience by allowing users to interact with the app in their preferred language.
Wider Reach: By offering content in multiple languages, we expand our app's reach to diverse demographics, potentially increasing user engagement and adoption.
Localization: ngx-translate simplifies the localization process, making it easy to adapt the app for various regions and cultures.
Flexibility: It grants users the flexibility to switch between languages, fostering inclusivity and user satisfaction.
We will now look into each step that you require to make your Angular app a multi-lingual app.
Step 1: Create your Angular project
- Run this command to install Angular :npm install -g @angular/cli
- Now run this to create your angular project :ng new my-first-project
Step 2:Install the ngx-translate library
- Run the command below to install the ngx-translate/core library:npm install @ngx-translate/core
- We will need to install the loader that will help us to load the translation files:npm install @ngx-translate/http-loader
Once we are done with the installation of all the required packages we need to register the translation module
Step 3:Integration of the translation module in our code
Now open the app.module.ts file in update the code as follows:
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { TranslateModule, TranslateLoader } from '@ngx-translate/core';
import { HttpClient } from '@angular/common/http';
export function HttpLoaderFactory(http: HttpClient) {
return new TranslateHttpLoader(http, './assets/i18n/', '.json');
}
@NgModule({
declarations: [
AppComponent
],
imports: [
// Initializing TranslateModule with loader
TranslateModule.forRoot({
loader: {
provide: TranslateLoader, // Main provider for loader
deps: [HttpClient], // Dependencies which helps serving loader
}
}),
],
providers: [],
bootstrap: [AppComponent]
});
export class AppModule { }
Now that the registration process is complete, the next step involves setting up the components. In our app.component.ts
file, we'll focus on initializing and setting the default language, as well as implementing language switching functionality.
Step 4: Setting Up Components and Language Handling in app.component.ts
Initialization:
The first crucial step is to initialize ngx-translate and set the default language. This ensures that your application displays content in the desired language from the start.
Additionally, we should include an option that enables users to freely choose their preferred language.
So we will add a function to switch languages in our app.component.ts
// Import necessary ngx-translate modules
import { TranslateService } from '@ngx-translate/core';
// ...
constructor(private translate: TranslateService) {
// Set the default language (e.g., English)
translate.setDefaultLang('en');
}
// Create a function to switch the language dynamically
switchLanguage(language: string) {
// Use ngx-translate to change the current language
this.translate.use(language);
}
We will implement a dropdown menu for selecting the language in our app.component.html
<select (change)="switchLanguage($event.target.value)">
<option value="en">English</option>
<option value="fr">Français</option>
<option value="es">Español</option>
<!-- Add more language options as needed -->
</select>
Step 5: Creating the JSON translation files.
We should place our translation files for various languages in the following directory path, where we'll create and store the translations.
src/
├── app/
├── assets/
│ i18n/
├── en.json
└── fr.json
└── es.json
Here's an example of what the contents of a translation file (en.json
) might look like:
{
"HELLO": "Hello",
"WELCOME": "Welcome to our application"
}
And similarly for fr.json
{
"HELLO": "Bonjour",
"WELCOME": "Bienvenue dans notre application"
}
This approach allows you to generate translation files for any desired language.
In a nutshell, ngx-translate is your gateway to a globally accessible app. By creating translations for different languages, you enhance user engagement. Now, equipped with ngx-translate, you're all set to offer a multilingual experience that welcomes a broader audience. Happy translating!
Give me a follow on LinkedIn!
Subscribe to my newsletter
Read articles from Inaamul hasan Shaikh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
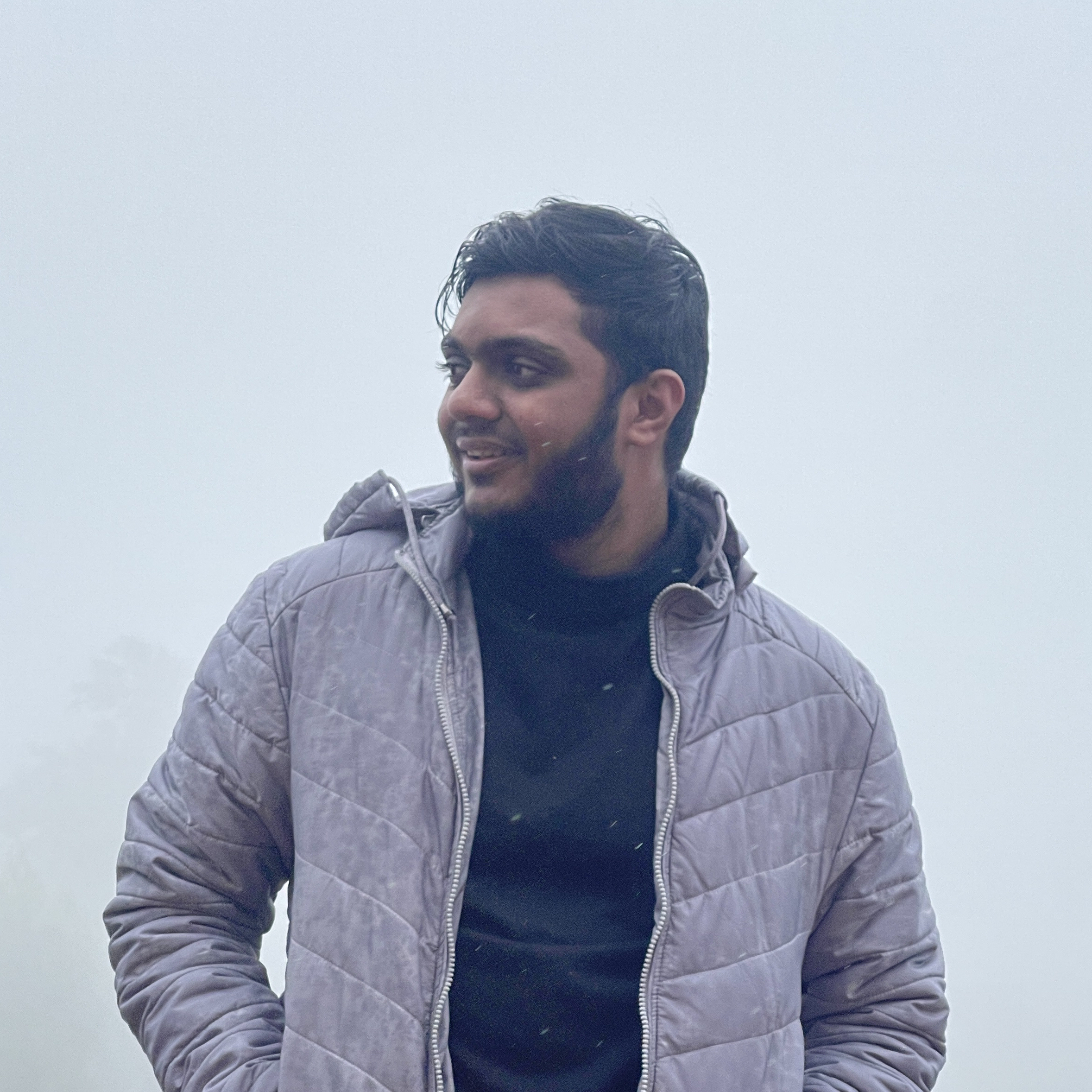