Python with a Command Line Interface
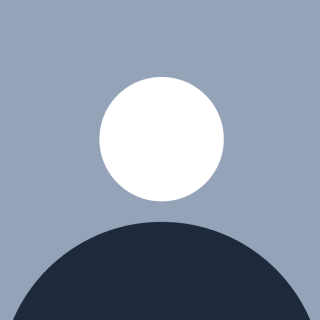
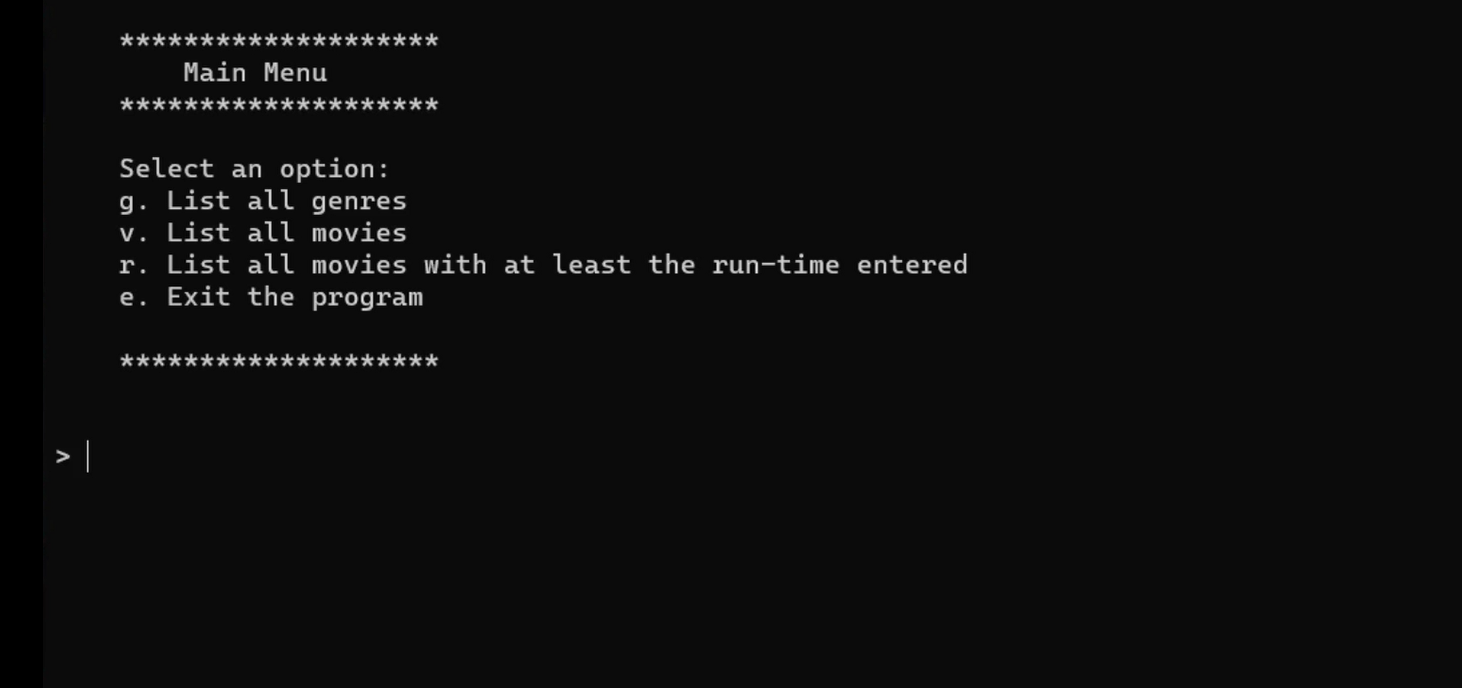
Main Menu
To start the cli.py file, we will add the main menu.
#cli.py
def main_menu():
print('''
********************
Main Menu
********************
Select an option:
g. List all genres
v. List all movies
r. List all movies with at least the run-time entered
e. Exit the program
********************
''')
if __name__ == "__main__":
main()
There are four options. We will start with the last one: the exit function.
#cli.py
def main():
choice = "0"
while choice != "e":
main_menu()
choice = input("> ")
if choice == "g":
pass
elif choice == "v" or choice == "r":
pass
elif choice == "e":
exit_program()
else:
print("Invalid choice")
You will notice that we added some placeholders for now. This will set us up for the rest of the menu. There is also a print at the end in case an invalid choice is entered.
The exit_program() function will be in a different file called helpers.py. This will need to be imported in the cli.py file as follows.
#cli.py
from helpers import(
exit_program
)
We will import more helper functions as we go along.
#helpers.py
def exit_program():
print("Goodbye!")
exit()
exit_program() will be used in each menu that is created.
Genre Menu
Choosing option "g" in the main menu will bring us to the genre menu.
There are two genres already called Romantic comedy and Action. Underneath the genres, there is a new menu. This includes options to look at more details for a genre, create a new genre, go back to the main menu, or exit. This menu is called all_menu() because it will be used for the all genres option from the main menu as well as the all movies and run time options.
#cli.py
def all_menu():
print('''
----------------------
Select an option:
i. Enter id for more details
n. Enter name for more details
c. Create new
m. Back to Main Menu
e. Exit the program
----------------------
''')
In order to see all the genres and the all_menu(), we need to work on the 'if choice == "g"' option from the code we wrote earlier. I am using "..." to represent that there is missing code that we wrote before and needs to be included.
The first thing we will need is a new choice variable. We will use all_genre_choice and set it to "0". Then, there will be a while loop to keep us seeing the all menu until we choose to go back to the main menu. That is what "m" represents.
#cli.py
...
if choice == "g":
all_genre_choice = "0"
while all_genre_choice != "m":
print('''
~~~~~~~~~~~~~~~~~~~~
Genres
~~~~~~~~~~~~~~~~~~~~
ID: Genre''')
list_genres()
print('''
~~~~~~~~~~~~~~~~~~~~
''')
all_menu()
...
Most of the above code is styling to make the menus and displays easier to see when a new menu pops up on the screen. list_genres() is a new function that we see here. This is imported from helpers.py.
#helpers.py
from models.genre import Genre
def list_genres():
genres = Genre.get_all()
for genre in genres:
print(f"{genre.id}: {genre.name}")
In this post, we will not be covering the Genre class. Just know that this class has a method called get_all() that gets all the genres we need to display in our genres menu. Also, that each genre has an id and name.
In our cli.py file after we display the all_menu(), we need to come up with some code that will deal with the options from all_menu(). Again, to save space, I am using "..." to say that there is other information there.
#cli.py
from helpers import(
create_genre,
exit_program
)
...
if choice == "g":
...
all_menu()
all_genre_choice = input("> ")
if all_genre_choice == "i" or all_genre_choice == "n":
pass
elif all_genre_choice == "c":
create_genre()
elif all_genre_choice == "m":
print("Back to Main Menu")
elif all_genre_choice == "e":
exit_program()
else:
print("Invalid choice")
...
I have included a "pass" for choosing "i" or "n" since that will take us to the next menu and more details for a genre.
- To choose "c" means to create a new genre. create_genre() is a function in helpers.py. This needs to be imported to cli.py.
#helpers.py
def create_genre():
name = input("Enter the genre's name: ")
try:
genre = Genre.create(name)
print(f"Success: {genre}")
except Exception as exc:
print("Error creating genre: ", exc)
To choose "m" means to go back to the main menu. We only include a print here. This helps the program understand that all_genre_choice = "m" and we are only allowed to see the all_menu() if all_genre_choice != "m". This means that the CLI will push us back to the previous menu which is the main menu.
To choose "e" means to exit the program. We have already looked at this exit function.
The next thing to do would be to work on choosing one of the genres. Since we have already looked at two menus and how to go back to the previous menu, we will now stop here.
Full Code
https://github.com/nlisterfelt/python-p3-v2-final-project-template.
Walk-through Video
Subscribe to my newsletter
Read articles from Nicole Listerfelt directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by