Socket Programming with Python

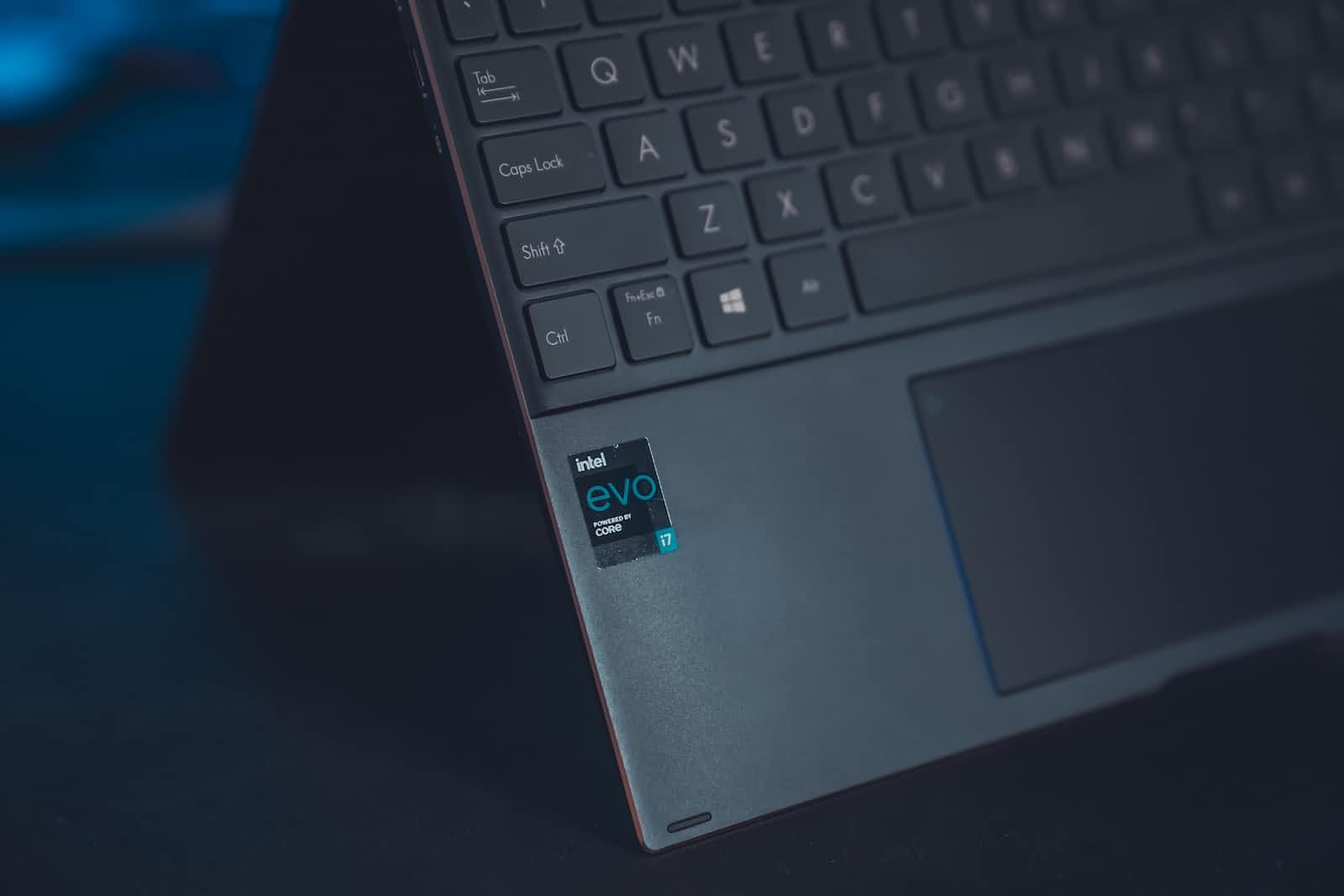
A detailed guide to learning the fundamentals of Socket programming with Python. Explore the power of creating network connections, sending and receiving data, and building robust network applications to harness the potential of sockets for seamless communication between devices.
Introduction
A socket is an endpoint for sending and receiving data across a computer network, enabling seamless data transfer. Socket programming is a fundamental concept in computer networking that enables applications to communicate over a network. Sockets are characterized by their type (TCP or UDP) and their address family (IPv4 or IPv6).
Python provides a powerful and straightforward way to implement socket programming, making it an essential skill for developers working on networked applications. In this article, we delve into the intricacies of Socket Programming with Python, discussing how to create both server and client applications, along with some common use cases.
Socket programming forms the backbone of network applications and has extensive use cases in various domains, from web development to Internet of Things (IoT) devices.
Sockets Functionality
Sockets operate through client-server architecture, where one device acts as a server, waiting for incoming connections, and others act as clients, initiating connections to the server. This two-way communication allows for smooth data flow between the devices.
There are two types of sockets; TCP and UDP sockets. TCP sockets offer reliable, ordered, and error-checked data transmission, making them ideal for applications that require data integrity, like web browsing and email. UDP sockets, on the other hand, provide faster but less reliable communication, suitable for applications like video streaming and online gaming.
Building Socket Connections
Creating a socket with Python
Socket programming involves two primary roles: building the server and the client. A server socket waits for incoming connections from clients, while a client socket initiates a connection to a server. Servers often listen on a specific IP address and port, allowing clients to connect and exchange data. Python's built-in socket
library simplifies socket programming. By creating a socket object, you can specify the socket family, socket type, and protocol, tailoring the socket to your application's needs.
To begin socket programming in Python, you first need to import the socket
module and then create a socket using the socket()
function, specifying the address family and socket type.
For instance:
import socket
# Create a TCP/IP socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Every socket has an associated address and port number, allowing applications to identify and connect. This addressing scheme ensures data reaches the intended recipient. Using Python's socket
library, you can establish connections by binding the server socket to an address and port. Clients can then connect to this address to initiate communication and once a connection is established, Python's socket functions allow for a bidirectional flow of data.
A simple TCP server
To create a basic server, follow the following steps:
Import the socket module
Create a socket object using the
socket.socket()
method.Bind the socket to a specific address and port using the
bind()
method.Listen for incoming connections with the
listen()
method.Accept incoming connections using the
accept()
method.Communicate with the client using the socket connection
Let us consider a simple TCP server that echoes the messages from the client.
import socket
# create a socket object
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# bind the socket to a specific address and port
server_address = ('localhost', 12345)
server_socket.bind(server_address)
# listen for incoming connections
server_socket.listen(5)
print("Server is listening...")
while True:
# accept connections from outside
client_socket, address = server_socket.accept()
# communicate with the client
data = client_socket.recv(1024)
client_socket.sendall(b'Server received: ' + data)
client_socket.close()
A TCP client
Building the TCP client involves:
Importing socket module.
Creating a socket object using
socket.socket()
method.Connecting to the server using
connect()
method.Sending and receiving data through the established connection.
Here's a basic client implementation:
import socket
# Create a TCP/IP socket
client_sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Connect to the server's address and port
server_address = ('localhost', 12345)
client_sock.connect(server_address)
# Send data to the server
client_sock.sendall(b"Hello, server!")
# Receive data from the server
data = client_sock.recv(1024)
print("Received:", data.decode())
# Close the socket
client_sock.close()
Handling Multiple clients
In scenarios where a server needs to handle multiple clients simultaneously, the select
module can be utilized. This module allows for efficient I/O multiplexing, enabling a single process to manage multiple sockets concurrently.
The select
module works by monitoring multiple sockets and notifying the program when one or more sockets are ready for reading, or writing, or have an error condition. It prevents the program from blocking on a socket that is not ready, enabling it to handle multiple connections concurrently.
Here is an example of using select
module to handle several clients:
import socket
import select
# Create a socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 8080))
server_socket.listen(5)
# List to keep track of socket objects
sockets_list = [server_socket]
while True:
# Get the list of sockets that are ready to be read through select
read_sockets, _, _ = select.select(sockets_list, [], [])
for sock in read_sockets:
# If a new connection is requested
if sock == server_socket:
client_socket, client_address = server_socket.accept()
sockets_list.append(client_socket)
print(f"New connection from {client_address}")
# Handle messages from existing clients
else:
try:
data = sock.recv(1024)
if data:
# Broadcast the received data to all clients
for socket_item in sockets_list:
if socket_item != server_socket and socket_item != sock:
try:
socket_item.send(data)
except:
# Handle socket errors
socket_item.close()
sockets_list.remove(socket_item)
except:
continue
# Close the server socket
server_socket.close()
The code above enters an infinite loop to continuously handle incoming connections and messages. The select.select
function is called with sockets_list
as the first argument to monitor the sockets. The second and third arguments are empty lists indicating that we are only interested in sockets that are ready for reading.
If the socket being processed is the server socket, a new connection has been requested, and the accept
method is called on the server socket to accept the connection and obtain a new client socket.
However, if the socket being processed is not the server socket, it means a message has been received from an existing client. The code attempts to receive data from the client using the recv
method. If data is received, it is broadcast to all other clients in the sockets_list
excluding the server socket and the original client socket from which the message originated.
In the case of a socket error, while sending data to a client, the code closes the client socket and removes it from the sockets_list
Leveraging the power of socket programming
Socket Programming empowers developers to create a wide array of network applications, some prominent use cases include:
Web servers and clients: Socket programming is used in web applications to establish connections between web servers and clients. It enables the transfer of data between the server and the client browser, enabling real-time communication and dynamic content delivery.
Real-time Messaging applications: Socket programming is often used in the development of messaging applications where users can send and receive real-time messages. Instant messaging platforms and chat applications rely on socket programming to enable real-time communication between users
Online Gaming: Multiplayer games that involve real-time interaction between players rely on socket programming to facilitate communication between the game server and multiple clients. This enables seamless real-time gaming experiences and allows players to interact with each other in the game environment.
IoT communication: Socket programming is crucial in IoT applications, where devices communicate and exchange data over the internet. IoT devices use sockets to connect to servers or other devices, enabling data transmission, monitoring, and control over the network.
Distributed systems: Socket programming plays a significant role in the development of distributed systems, where multiple nodes of a system need to communicate with each other. It allows different parts of a distributed system to exchange information and coordinate activities, facilitating the seamless functioning of the overall system.
Subscribe to my newsletter
Read articles from Xam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Xam
Xam
A software engineer with an inclination towards security. I like to simplify the things I know so you can understand them better, the gods must be crazy!! Top Tier!!