Introduction to Node.js
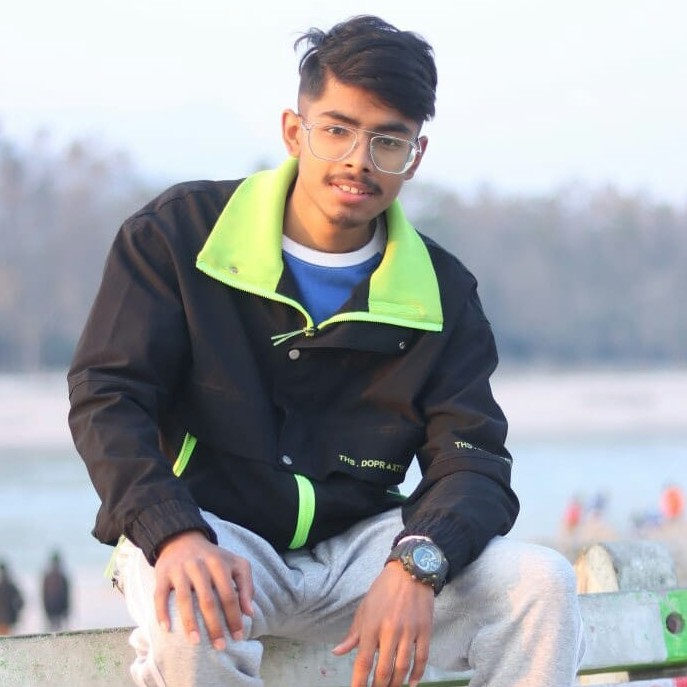
Table of contents
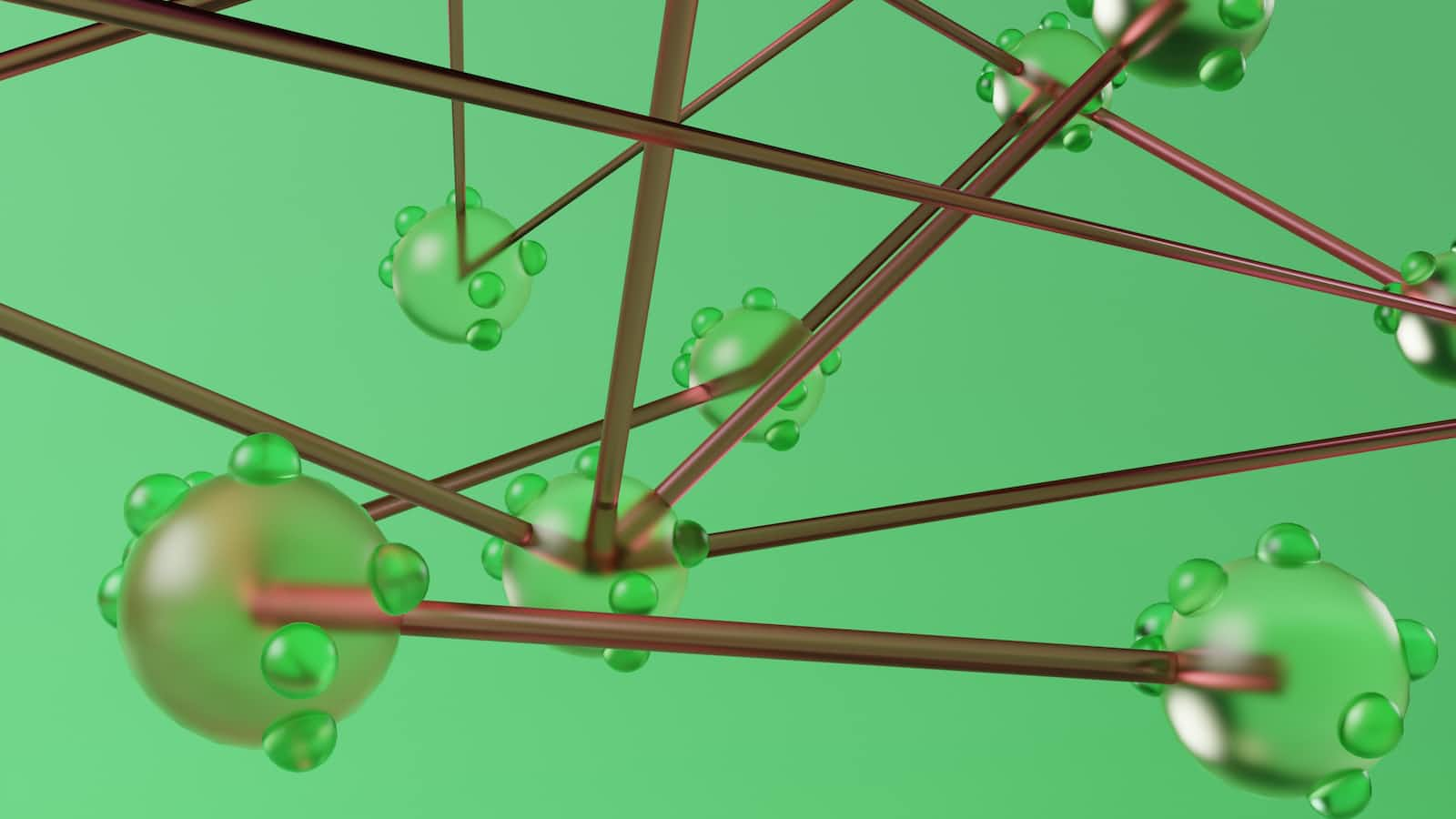
NodeJS
It is a runtime environment to run JavaScript outside the browser. It allows JavaScript to be used on the server side and create awesome web apps. Even though we can still use JS functions like setInterval and setTimeout, we can't use browser functionalities like window/document, Web APIs, etc. To get started with NodeJS, we need to install its latest version from the official NodeJS page.
Creating Node App
Just create a normal app.js file and write your JavaScript code there. Waallah! your first node app is ready.
console.log("Hello World");
To run this app, run the command: node app.js
If you're using VS-Code IDE, then simply press Ctrl + Alt + N to run the code.
Globals
They are the variables and methods which can be accessed throughout the entire application. Some of the important globals are as follows:
Global Name | Description |
__dirname | A variable that stores the path to the current directory |
__filename | A variable that stores the current program filename |
require() | A function to import the module |
module | An object to manage module functionality |
process | An object containing information about the environment in which the app is being executed |
Modules
If your node app becomes complex, it is better to split the code into different modules for easy maintenance, security and clean code. In NodeJS, every file can be considered as a module. Let's see an example of a code without modules:
// Variables
let num1 = 20;
let num2 = 10;
// Function
function adder(n1, n2)
{
console.log(`Sum is: ${n1 + n2}`);
}
// Output: Sum is: 30
adder(num1, num2);
Now, let us assume this is a complex code and break it down into modules of variable, function and main app.
variableModule.js:
let num1 = 20;
let num2 = 10;
module.exports = {num1, num2};
Here, the module is a global object that is being used to export the variables from this value.js similar to a react component being exported.
functionModule.js:
function adder(n1, n2)
{
console.log(`Sum is: ${n1 + n2}`);
}
module.exports = {adder};
app.js:
// Importing the exported modules
let num = require("./variableModule");
let func = require("./functionModule");
// Accessing module variables and functions
func.adder(num.num1, num.num2);
Subscribe to my newsletter
Read articles from Giver Kdk directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
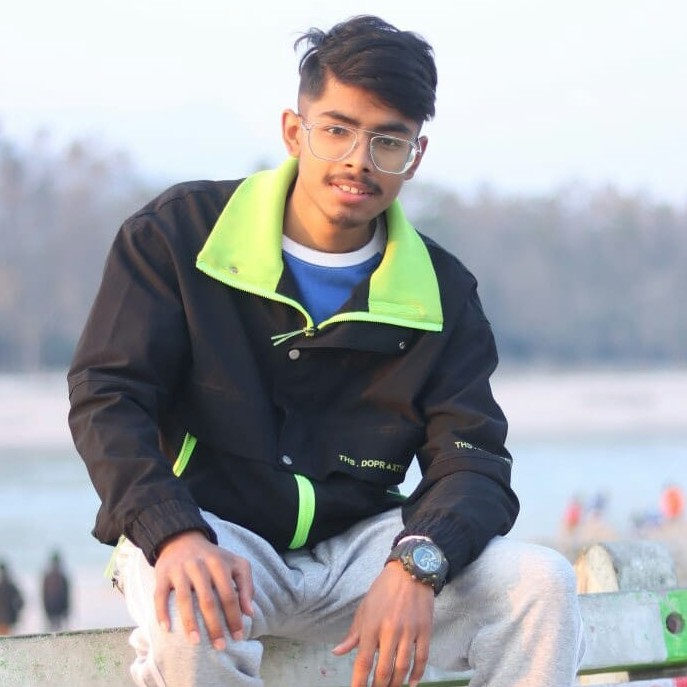
Giver Kdk
Giver Kdk
I am a guy who loves building logic.