Three.js Day- 1
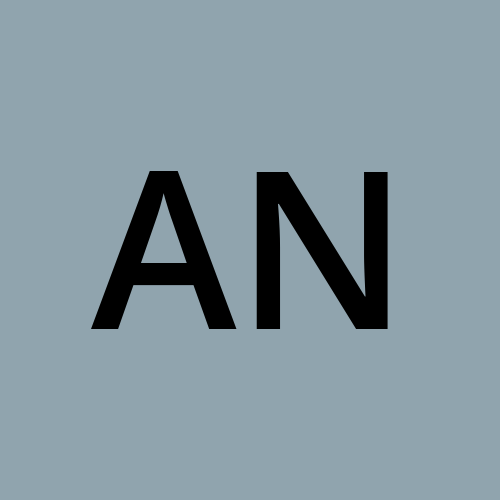
On my first day I didn't learn anything complicated about three.js. Today I learned some basics about three.js, like how to include it in your project and creating a Red Cube.
First of all, create a basic html file. Then link your js with it using the script tag and src attribute. To check that your js file is linked and working perfectly open the Developer Tools.
To do that, you can right-click anywhere on the page that pops up when you click on open in live server and choose Inspect
or you can press F12
on Windows and CMD + OPTION + I
on MacOS.
Then navigate to the Console tab on top of the Developer Tools.
You should keep the Console open at all time to see potential errors and warnings.
How to load three.js
Download the three.js file from the link https://threejs.org/ and click on the download button to download a zip file and unzip it. It will take some time to download as the file is heavy but don't worry we only need one file from that.\
After unzipping the file Go to the build/
folder and copy the three.min.js
file to your project.
Make sure to add three.min.js above the js link so that it loads before.
First Scene (We are going to create a Red Cube)
To produce something on the screen we need 4 elements to get started
A scene that will contain objects -- (We can say that a scene is like a container : We can put objects, particles, lights etc. in it. We can render that scene by using three.js when we need it. (A scene is like a movie set)
Some objects -- (Mesh : It is like the visible object
To create that red cube, we need to create a type of object named Mesh. A Mesh is the combination of a geometry (the shape) and a material (how it looks).
A camera
A renderer
To create a scene we use the we use the Scene class:
// Scene
const scene = new THREE.Scene()
Objects (Mesh)
Objects can be many things like one can have primitive geometries, imported models, particles, lights, and so on. For today we are just starting only with a small cube.
To create a desired cube, we need to create a type of object named Mesh. Mesh is the visible object combination of geometry(the shape) and material (how it looks/color).
There are many geometries and many materials, but we will keep things simple for now and create a BoxGeometry and a MeshBasicMaterial.
To create the geometry, we use the BoxGeometry class with the first 3 parameters that correspond to the box's size.
// Object
const geomtery = new THREE.BoxGeomtry(1,1,1)
As we have created the first part of mesh: geometry, it's time to create the second part of it: material.
To create the material, we use the MeshBasicMaterial class with one paramter: an object {} containing all the options. (MeshBasicMaterial--->A class to provide parmeters to a material u have to provide an object bcoz there can be a lot of variables). We need to provide color property.
// Object
const geometry = new THREE.BoxGeomtry(1,1,1)
const material = new THREE.MeshBasicMaterial({color: 0xff0000})
To create the final mesh, we use the Mesh class and send the geometry and material as parameters.
// Object
const geometry = new THREE.BoxGeometry(1,1,1)
const material = new THREE.MeshBasicMaterial({color: 0xff0000})
const mesh = new THREE.Mesh(geometry, material)
You may now think that the work is completed but it's not completed yet. You have created the mesh but not have added it to the scene. So let's add your mesh to scene . To add the mesh to your scene use the add(...) method:
// Object
const geometry = new THREE.BoxGeometry(1,1,1)
const material = new THREE.MeshBasicMaterial({color: 0xff0000})
const mesh = new THREE.Mesh(geometry, material)
scene.add(mesh)
It's important to add your object(mesh) to the scene to be able to see it. If you don't add your object to the scene then you won't be able to see it.
Camera(Point Of View)
The camera which we are going to discuss is not visible. We provide a camera for like having a theoretical point of view. Every time we render some scene it's from that camera's point of view. One can have multiple cameras i.e. multiple point of view just like on a movie set, and we can switch between those cameras based on our needs but when u do render it's from one camera. Usually, we use only one camera. There are many different types of cameras but for now we simply need a camera that handles perspective(making close objects look more prominent than far objects).
then provide a camera it's for having a point of view
To create the camera, we use the PerspectiveCamera class. We have to provide two essential parameters to the PerspectiveCamera class:
The Field of View ---> Vertical field of view---If u provide a small view it's like viewing from a scope.
The Aspect Ratio
The field of view is how large your vision angle is. If you use a very large angle, you'll be able to see in every direction at once but there will be much distortion because the result will be drawn on a small rectangle. If you use a small angle, things will look zoomed in. The field of view (or fov
) is expressed in degrees and corresponds to the vertical vision angle.
The Aspect ratio is the width of the canvas divided by it's height.
Now let's add camera to our scene. It's very important to add camera in our scene, so always remember it.
// Sizes
const sizes = {
width = 800,
height = 600
}
// Camera
const camera = new THREE.PerspectiveCamera(75, sizes.width / sizes.height)
scene.add(camera)
Provide a Renderer
It will do a render of your scene but seen through the Camera point of view, and will draw it into the canvas.For now, we will add the canvas to the html and send it to the renderer.
Create a canvas in HTML before you load the scripts and provide a class named webgl.
<canvas class="webgl"></canvas>
To create the renderer, we use the WebGLRenderer class with one parameter: an object {}
containing all the options. We need to specify the canvas
property corresponding to the <canvas>
we added to the page.
We need to update(provide) size to the renderer with the setSize(...) method using the sizes object we created above. The setSize(...) method will automatically resize our canvas accordingly.[When u resize the renederer it resizes the canvas].
// Canvas
const canvas = document.querySelector('.webgl')
console.log(canvas);
const renderer = new THREE.WebGLRenderer({
canvas
})
renderer.setSize(sizes.width, sizes.height)
Right now, you won’t be able to see anything, but your canvas is there and has been resized accordingly. You can use the Developer Tools to inspect the <canvas>
if you are curious.
First Render
Just almost there everything is set It's time to work on our first render.[ Do renderer.render() u have to provide a scene and the camera] Call the render(...)
method on the renderer and send it the scene
and the camera
as parameters:
renderer.render(scene, camera)
Can't see anything here's the issue: we haven't specified our object's position, nor our camera's. Both are in the default position, which is the center of the scene and we can't see an object from its inside (by default) i.e. everything is in the center of the scene --- camera is inside the cube---- we need to move the camera backward
By default, u can only see one side of triangle.
To transform/move the camera/objects (it can be anything) a little bit we can use the following properties
Position
Rotation
Scale
For now, we will use the position property to move the camera backward.
const camera = new THREE.PerspectiveCamera(75, sizes.width / sizes.height)
camera.position.z = 3
scene.add(camera)
Thus, by following all these steps you will be able to create and see your first render.
Subscribe to my newsletter
Read articles from Anurag directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
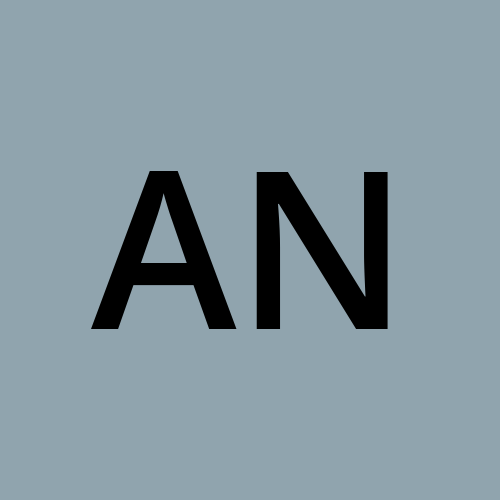