Building a BMI Calculator in C: A Personal Project
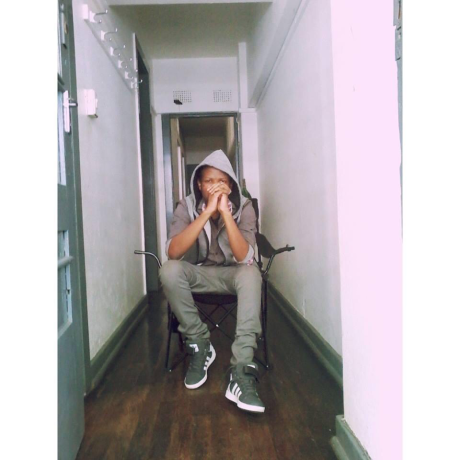
Table of contents
#include <stdio.h>
/**
* main - BMI calculator
* description - Calculates the Body Mass Index (BMI)
* Return: 0
*/
int main(void)
{
/* Declaring variables */
float height;
float weight;
float BMI;
printf("Enter your height in cm: "); /*prompt user to input height in cm*/
scanf("%f", &height);
printf("Enter your weight in kg: "); /*prompt user to input weight in kgs*/
scanf(" %f", &weight);
height = height / 100; /*convert height cm to m*/
BMI = weight / (height * height); /*calculate BMI*/
if (BMI < 18.5)
printf("You are underweight. \n");
else if (BMI >= 18.5 && BMI < 25)
printf("Your weight is normal \n");
else if (BMI >= 25 && BMI < 30)
printf("You are overweight. \n");
else
printf("You are obese \n");
return 0;
}
Hello everyone! Today, I want to share a personal project that I've been working on to enhance my skills in C programming. It's a simple yet practical application: a Body Mass Index (BMI) calculator.
The Body Mass Index (BMI) is a measure that uses your weight and height to calculate if your weight is healthy. The BMI calculation divides an adult's weight in kilograms by their height in meters squared. The resulting number can indicate whether the person has a healthy or unhealthy body weight.
The code starts with the inclusion of the stdio.h library, which is used for input/output operations.
The main function is where our program starts. We declare three float variables: height, weight, and BMI.
We then ask the user to enter their height in centimeters and their weight in kilograms using printf and scanf.
The height is converted from centimeters to meters by dividing it by 100. The BMI is then calculated by dividing the weight by the square of the height.
Finally, we use conditional statements (if and else if) to classify the user's weight status based on their calculated BMI.
This project was a great way to practice C programming. It involved user input, arithmetic operations, and control structures, which are fundamental concepts in programming. I hope you found this walkthrough helpful. If you're learning C or programming in general, I encourage you to try building this BMI calculator yourself!
Subscribe to my newsletter
Read articles from Wisdom Ncube directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
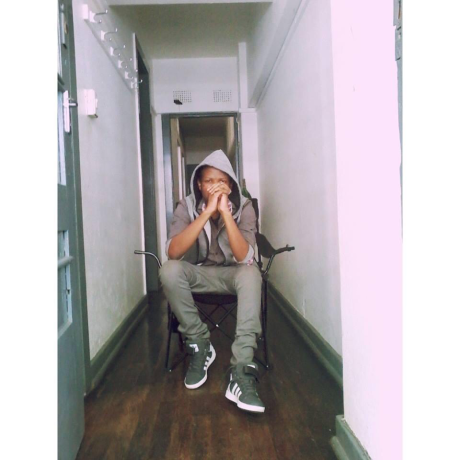
Wisdom Ncube
Wisdom Ncube
I am a Software Engineering learner at ALX documenting my journey.