Day 2 of the Bash Scripting Challenge! ๐
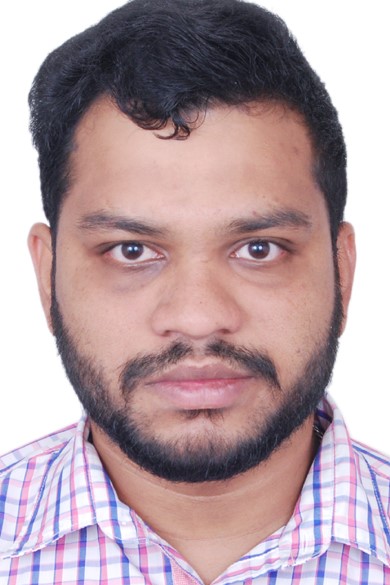
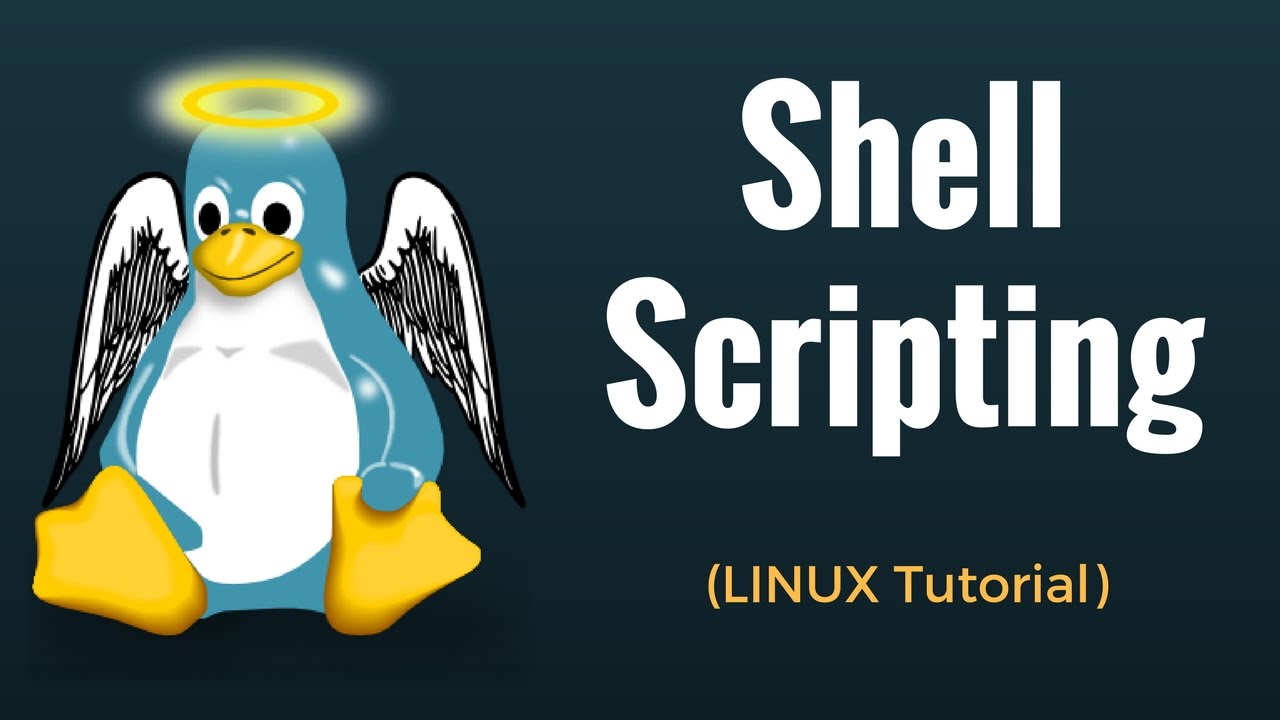
Welcome to Day 2 of the #TWSBashBlaze Challenge. In this challenge, we will create a bash script that serves as an interactive file and directory explorer. We'll create a script that will allow you to explore the files and directories in the current path and also provide a character-counting feature for the user's input.
Part 1: File and Directory Exploration
In this task, we are going to create a bash script explorer.sh which will display a welcome message and list all the files and directories in the current path upon execution.
For each file and directory, the script will print its name and size in human-readable format (e.g., KB, MB, GB). This information will be obtained using the ls command with appropriate options.
The list of files and directories will be displayed in a loop until the user decides to exit the explorer.
Part 2: Character Counting
After displaying the file and directory list, the script will prompt the user to enter a line of text.
The script will read the user's input until an empty string is entered (i.e., the user presses Enter without any text).
For each line of text entered by the user, the script will count the number of characters in that line.
The character count for each line entered by the user will be displayed.
#!/bin/bash
# Part 1: File and Directory Exploration
echo "Welcome to the Interactive File and Directory Explorer!"
while true; do
# List all files and directories in the current path
echo "Files and Directories in the Current Path:"
ls -lh
# Part 2: Character Counting
read -p "Enter a line of text (Press Enter without text to exit): " input
# Exit if the user enters an empty string
if [ -z "$input" ]; then
echo "Exiting the Interactive Explorer. Goodbye!"
break
fi
# Calculate and print the character count for the input line
char_count=$(echo -n "$input" | wc -m)
echo "Character Count: $char_count"
done
Output Screen:
Thank you for reading!
Contact me at :
Linkedin: https://www.linkedin.com/in/sutish-pradhan/
E-mail: psutish@gmail.com
Subscribe to my newsletter
Read articles from sutish pradhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
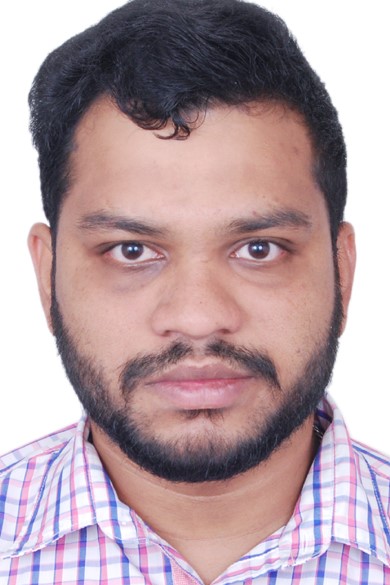
sutish pradhan
sutish pradhan
Hey there! I am Sutish I am a Devops engineer, started writing articles on my DevOps and cloud journey. My purpose is to share the concepts that I learn, the projects that I build, and the tasks that I perform regarding DevOps. Hope you all find it useful.