C# Loops
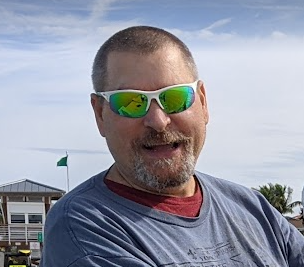
Table of contents
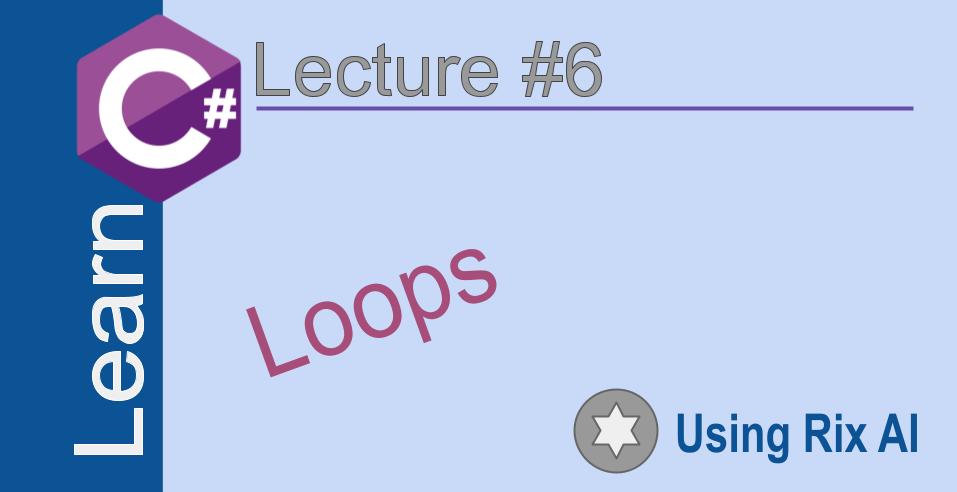
A repetitive statement in computer science refers to a statement or block of code that is executed repeatedly for a fixed number of times or as long as a certain condition is met.
Some examples of repetitive statements are:
• For loops - Execute a block of code for a fixed number of iterations. The basic structure is:
for (initialization; condition; increment)
{
// code to be executed
}
• While loops - Execute a block of code as long as a condition is true. The basic structure is:
while (condition)
{
// code to be executed
}
• Do-while loops - Similar to while loops, but the block of code is executed at least once before checking the condition. The basic structure is:
do
{ // code to be executed
}
while (condition);
Repetitive statements are useful for performing the same set of operations on multiple elements of an array or collection, iterating through a range of numbers, etc. They allow us to avoid writing the same code multiple times.
The main components of a repetitive statement are:
An initialization step
A condition that determines whether the loop continues or terminates
An increment or decrement step
Example
Here is a code example of a loop that prints out the days of the week to the console:
string[] days = { "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday" };
for (int i = 0; i < days.Length; i++)
{
Console.WriteLine(days[i]);
}
How it works:
We define an array
days
that contains the days of the week as string values.We use a for loop, initialized as follows:
i = 0
- Start at index 0i < days.Length
- Continue until i is less than the length of the arrayi++
- Increment i by 1 after each iteration
On each iteration, we print the day at the current array index
days[i]
to the console usingConsole.WriteLine()
.The loop continues, incrementing i until i reaches days.Length (7 in this case), then exits.
The output will be:
Monday
Tuesday
Wednesday
Thursday
Friday
Saturday
Sunday
Break & Continue
In C# loops, break and continue have the following functions:
Break:
The break statement immediately terminates the execution of the loop (for, while, do while, foreach). Control of the program flows to the next statement after the loop.
Syntax:
break;
Example:
for (int i = 0; i < 10; i++)
{
if (i == 5)
{
break;
}
Console.WriteLine(i);
}
// Prints 0 1 2 3 4
Here, the loop breaks when i becomes 5, so only numbers 0 to 4 are printed.
Continue:
The continue statement skips the current iteration of the loop and continues with the next iteration.
Syntax:
continue;
Example:
for (int i = 0; i < 10; i++)
{
if (i % 2 == 0)
{
continue;
}
Console.WriteLine(i);
}
// Prints 1 3 5 7 9
Here, when i is even, we continue to the next iteration, so only odd numbers from 1 to 9 are printed.
So in summary, the break exits the entire loop, while continuing is used to skip the current iteration and continue with the next one.
Nested loops
Nested loops in C# are loops that contain other loops within them. This allows us to iterate over multiple data structures in an inner-outer fashion.
Some examples of nested loops are:
- A for loop inside a for loop:
for (int i = 0; i < 10; i++)
{
for (int j = 0; j < 10; j++)
{
Console.WriteLine("i = {0}, j = {1}", i, j);
}
}
- A while loop inside a for loop:
for (int i = 0; i < 10; i++)
{
int j = 0;
while (j < 10)
{
Console.WriteLine("i = {0}, j = {1}", i, j);
j++;
}
}
- A foreach loop inside a while loop:
int[] array = {1, 2, 3, 4};
int i = 0;
while (i < array.Length)
{
foreach (int num in array)
{
Console.WriteLine(num);
}
i++;
}
The inner loop will be executed completely for each iteration of the outer loop.
Nested loops allow us to perform more complex iterations. For example, the first example above will print all combinations of i and j from 0 to 9.
The name of the loop variables does not matter - i and j are just used as examples here. You can name them anything meaningful for your code.
Best practice
Here are some best practices for loops in C#:
Name your loop variables meaningfully - i, j, k are not very descriptive. Use names like index, row, column etc. based on what the loop is iterating over.
Add spaces around operators for readability.
Use meaningful conditional expressions in the loop.
Avoid magic numbers. Store constants in variables with meaningful names.
Avoid deeply nested loops. Refactor the code if nesting goes beyond 3 levels.
Add comments to explain what the loop is doing.
Avoid infinite loops by:
Having a condition that will eventually become false.
Checking for that condition on each iteration.
Exiting the loop using break when needed.
Example of avoiding infinite loop:
int input;
while (true)
{
Console.Write("Enter a number: ");
input = int.Parse(Console.ReadLine());
if (input == 0)
{
break; // Exit loop
}
Console.WriteLine(input);
}
// Program exits loop when 0 is entered
Here we have a condition to exit the loop when 0 is entered. We check that condition on each iteration and break out of the loop when needed.
So in summary, give meaningful names, add spaces and comments, avoid nesting too deep and most importantly have a condition to exit the loop to avoid infiniteness.
Disclaim: We will learn more about loops later. This article is created with AI for beginners to understand the fundamentals. Ask any questions in comments.
Learn and prosper! 🖖
Subscribe to my newsletter
Read articles from Elucian Moise directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
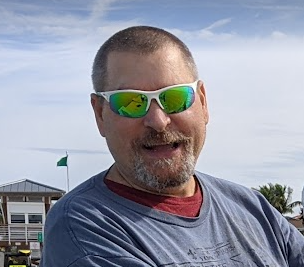
Elucian Moise
Elucian Moise
Software engineer instructor, software developer and community leader. Book author. Computer enthusiast and experienced programmer. Born in Romania, living in US.