Day 6 but Day 4 of Recursion

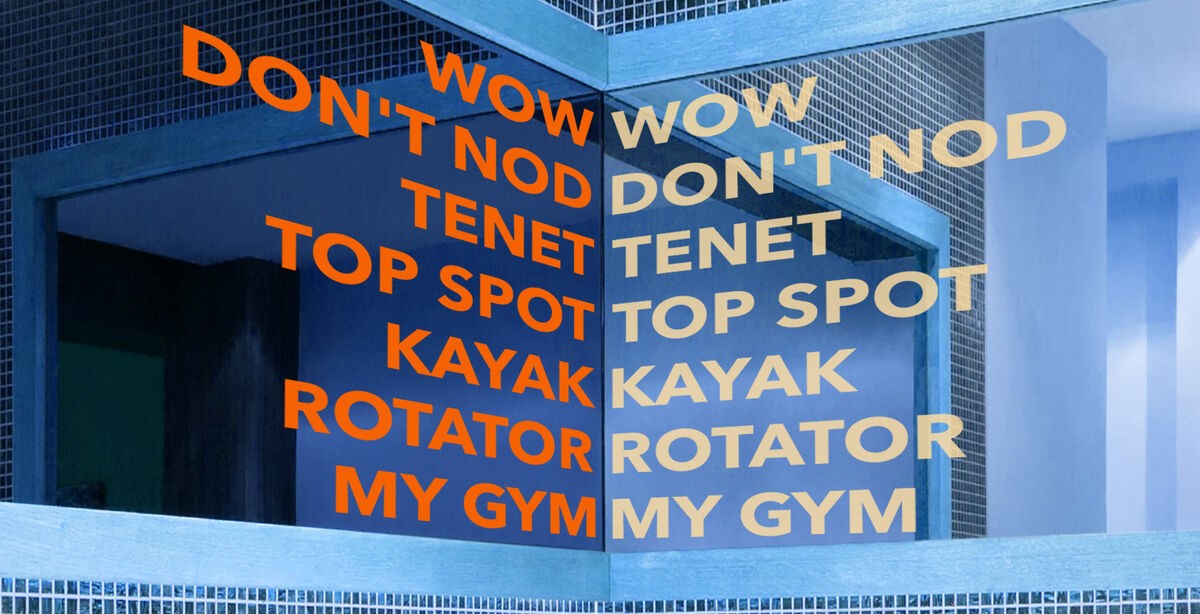
Hello! everyone, it's nice to interact and share my experiences with you. I hope you like my blogs. As we were going through the topics of recursion, we also tried to solve a few of the questions with the help of recursion. However, solving only a few problems won't help us fully grasp the concept of recursion. So again today we'll be going via the questions of recursions.
Reversing a string = String isn't a datatype, as many may confuse it. The string is a collection of characters, however, all the string characters are accessed using the concept of indexing just like arrays.
#include<bits/stdc++.h>
using namespace std;
void reverseString(string& str, int i, int j){
//base case
if (i >= j)
{
return;
}
swap(str[i], str[j]);
i++;
j--;
reverseString(str, i, j);
}
int main(){
string s;
cout << "Enter string : ";
cin >> s;
reverseString(s, 0, s.length()-1);
cout << "Reversed String = " << s;
return 0;
}
Palindrome = When a string id equal to the reverse of itself, then this case of string is known as Palindrome. Given below is the code you can used to check if a string is Palindrome or not.
#include<bits/stdc++.h>
using namespace std;
bool checkPalindrome(string str, int i, int j){
//base case
if(i >= j){
return true;
}
if(str[i] != str[j]){
return false;
}
else{
return checkPalindrome(str, i+1, j-1);
}
}
int main(){
string s;
cout << "Enter string : ";
cin >> s;
bool isPalindrome = checkPalindrome(s, 0, s.length()-1);
if(isPalindrome){
cout << "It's a Palindrome";
}
else{
cout << "It's not a Palindrome";
}
return 0;
}
Power of number = In mathematics, we're sometimes asked to calculate the power of a number like 2^3, 3^11, 5^1/2. Follow the code to understand it properly: -
#include<bits/stdc++.h> using namespace std; int power(int a, int b){ if (b == 0) { return 1; } if(b == 1){ return a; } int ans = power(a, b/2); if(b%2 == 0){ return ans*ans; } else{ return a*ans*ans; } } int main(){ int a, b; cout << "Enter a & b: "; cin >> a >> b; int ans = power(a, b); cout << "Answer = " << ans << endl; return 0; }
Subscribe to my newsletter
Read articles from Sawan Badhwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
