Checking for Uppercase Characters in C: A Technical Guide
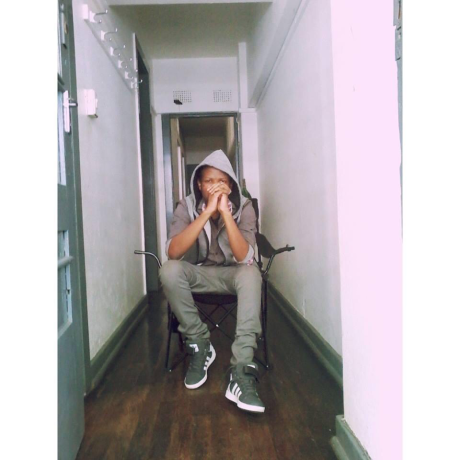
In programming, it is often necessary to perform various operations on characters, including checking if a character is uppercase or lowercase. In this technical guide, we will explore how to write a function in C that checks for uppercase characters. By understanding the ASCII values of characters and leveraging conditional statements, we can easily determine whether a character is uppercase or not.
Function Prototype: Let's start by defining the function prototype for our uppercase character checker:
int _isupper(int c);
The _isupper
function takes an integer c
as input, representing the ASCII value of a character. It returns 1 if the character is uppercase and 0 otherwise.
Implementation: To implement the _isupper
function, we need to compare the ASCII value of the character with the range of uppercase characters. In ASCII, uppercase characters have values ranging from 65 to 90.
int _isupper(int c) {
if (c >= 65 && c <= 90) {
return 1;
} else {
return 0;
}
}
In this implementation, we use an if
statement to check if the ASCII value c
falls within the range of uppercase characters. If it does, we return 1 to indicate that the character is uppercase. Otherwise, we return 0.
Usage: Now that we have our _isupper
function, let's see how we can use it to check if a character is uppercase:
int result = _isupper('A');
if (result == 1) {
printf("The character is uppercase.\n");
} else {
printf("The character is not uppercase.\n");
}
In this example, we call the _isupper
function and pass the ASCII value of the character 'A' as an argument. The function returns 1 because 'A' has an ASCII value of 65, which falls within the range of uppercase characters. As a result, the program outputs "The character is uppercase."
Conclusion: In this technical guide, we have explored how to write a function in C that checks for uppercase characters. By leveraging the ASCII values of characters and conditional statements, we can easily determine whether a character is uppercase or not. This function can be a valuable tool in various programming scenarios where character manipulation is required.
Subscribe to my newsletter
Read articles from Wisdom Ncube directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
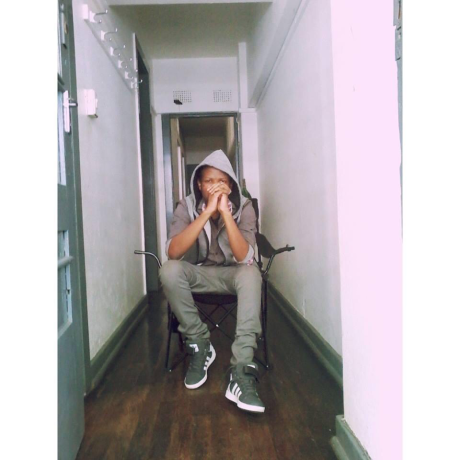
Wisdom Ncube
Wisdom Ncube
I am a Software Engineering learner at ALX documenting my journey.