Pointers in C Programming: Explained Simply
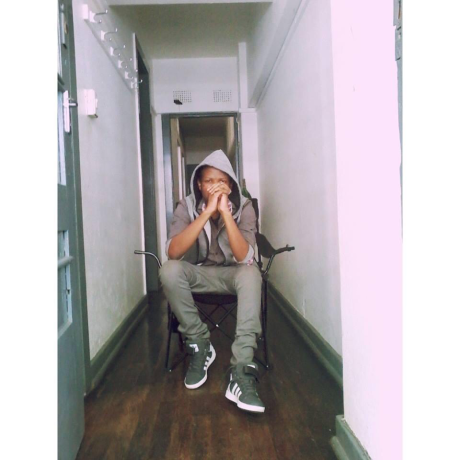
Introduction
Pointers are a fundamental concept in C programming that allow us to work with memory addresses instead of values directly. They provide powerful capabilities to manipulate data and optimize memory usage. In this article, we will explore the simplest use of pointers in C and understand their significance.
What are Pointers?
Pointers are special variables in C that store memory addresses instead of values[^0^]. Just like a treasure map points to the location of hidden treasure, a pointer points to the memory location where a value is stored[^1^]. It doesn't hold the actual value, but it allows us to access and manipulate that value indirectly.
A Simple Use Case
Let's consider a simple example to understand the use of pointers. Imagine we have a variable called "num" that stores the value 5. We can declare a pointer variable, "ptr", which will point to the memory address of "num". Here's how we can do it:
int num = 5; // Declare and initialize a variable
int *ptr = # // Declare and initialize a pointer, pointing to the address of "num"
In the above code, the "&" operator is used to get the memory address of "num" and assign it to the pointer variable "ptr"[^7^]. Now, "ptr" holds the memory address of "num" rather than the value itself.
Why is this useful?
Using pointers allows us to perform various operations, such as:
Modifying the Value: By dereferencing the pointer using the "*" operator, we can access and modify the value stored in the memory location it points to[^6^]. For example,
*ptr = 10;
would change the value of "num" to 10.Passing by Reference: Pointers are commonly used to pass variables by reference to functions. Instead of passing a copy of the variable, we pass its memory address. This allows the function to directly modify the original value[^3^]. Here's an example:
void increment(int *ptr) {
(*ptr)++; // Increment the value pointed by the pointer
}
int main() {
int num = 5;
increment(&num); // Pass the address of "num" to the function
printf("%d", num); // Output: 6
return 0;
}
- Dynamic Memory Allocation: Pointers enable us to allocate memory dynamically at runtime, which is useful when dealing with data structures like arrays and linked lists[^2^]. We can allocate memory using functions like
malloc()
andcalloc()
, and the pointer will store the address of the allocated memory.
Conclusion
Pointers in C are powerful tools that allow us to work with memory addresses and manipulate data indirectly. By using pointers, we can optimize memory usage, modify values directly, and pass variables by reference. However, it's important to handle pointers carefully to avoid errors like accessing invalid memory addresses. Understanding pointers is crucial for mastering C programming and unlocking its full potential.
Subscribe to my newsletter
Read articles from Wisdom Ncube directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
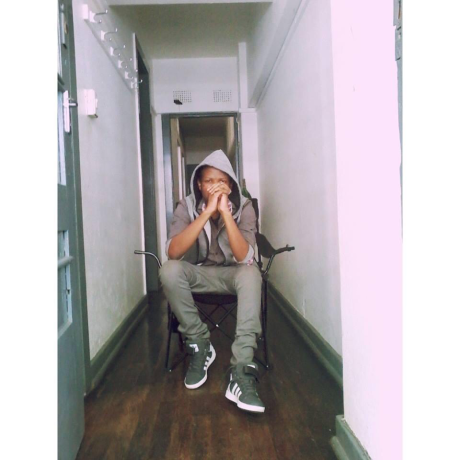
Wisdom Ncube
Wisdom Ncube
I am a Software Engineering learner at ALX documenting my journey.