Day 4 Task 1 - Process Monitoring with Bash #TWSBashBlazeChallenge🚀
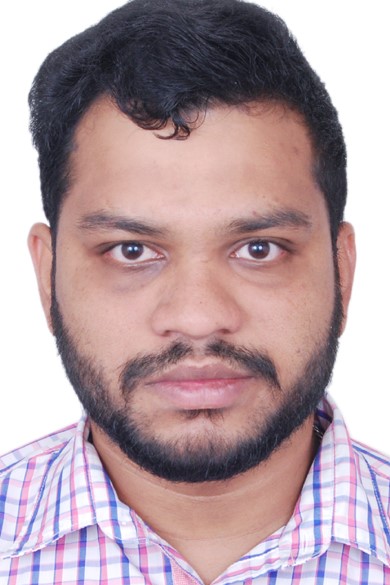
Table of contents
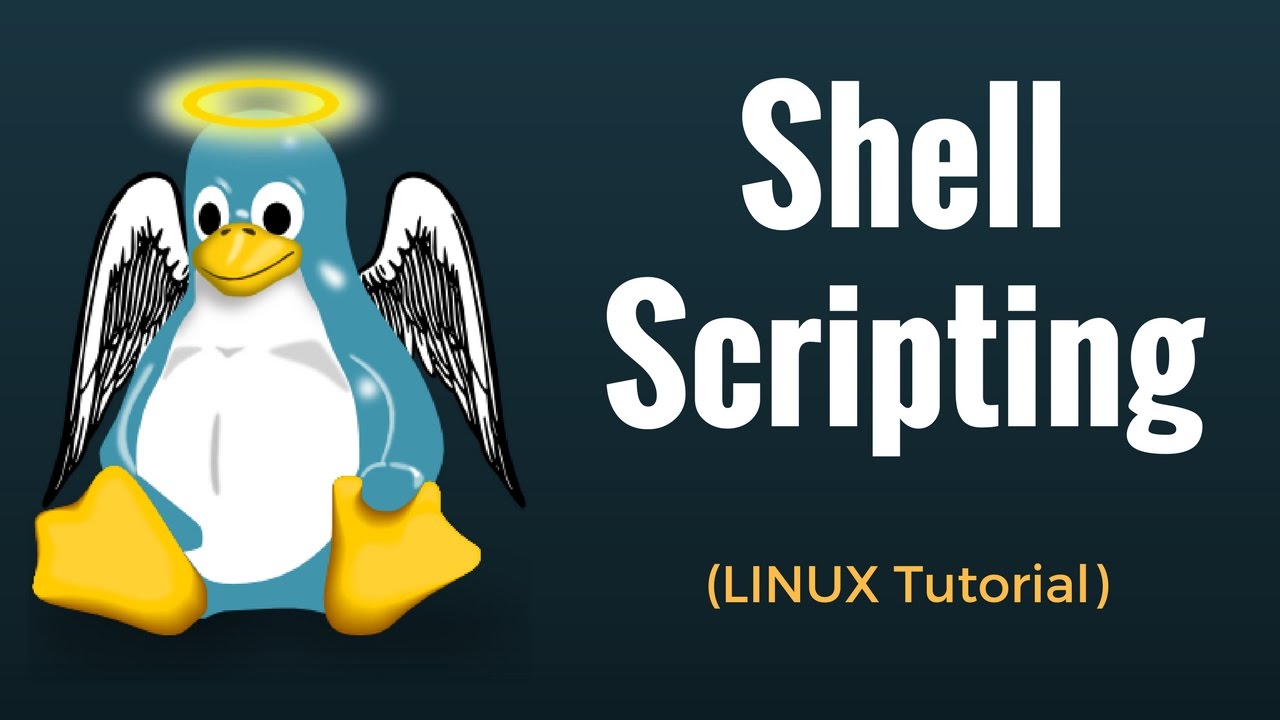
Introduction:
Welcome to the world of Linux process monitoring! In this blog, we will write a Bash script that efficiently monitors a specific process on a Linux system. The script's primary goal is to ensure the process is always running, and if it unexpectedly stops, it should be restarted automatically.
📋How to Use the Script
#!/bin/bash
# Function to check if the process is running
check_process() {
process_name=$1
pid=$(pgrep -x "$process_name")
if [ -n "$pid" ]; then
echo "Process $process_name is running with PID $pid."
return 0
else
echo "Process $process_name is not running."
return 1
fi
}
# Function to restart the process
restart_process() {
process_name=$1
attempts=$2
for ((i=1; i<=$attempts; i++)); do
echo "Attempt $i to restart process $process_name."
# Add the command to start the process here, e.g., "command_to_start_process"
# Replace the above line with the appropriate command to start the process.
sleep 1 # Wait for a moment before checking if the process is running.
if check_process "$process_name"; then
echo "Process $process_name restarted successfully."
return 0
fi
done
echo "Unable to restart process $process_name after $attempts attempts."
return 1
}
# Main script
if [ $# -eq 0 ]; then
echo "Usage: $0 <process_name>"
exit 1
fi
process_name=$1
max_attempts=3
if check_process "$process_name"; then
exit 0
else
restart_process "$process_name" "$max_attempts"
fi
Save the above script in a file named
monitor_process.sh.
Make the script executable using
chmod 744 monitor_process.sh.
To monitor a specific process, run the script with the process name as an argument:
./monitor_process.sh <process_name>.
E.g.:
./monitor_process.sh nignx
🖥️ Output Screens
📍 Conclusion
Our Bash monitoring script, monitor_process.sh
, is a valuable tool for DevOps professionals to keep critical processes running smoothly without constant manual intervention. It helps you to ensure high availability and also reduce downtime!
Hope you found this blog useful! 😊
Thank you for reading!
Contact me at :
Linkedin: https://www.linkedin.com/in/sutish-pradhan/
E-mail: psutish@gmail.com
Subscribe to my newsletter
Read articles from sutish pradhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
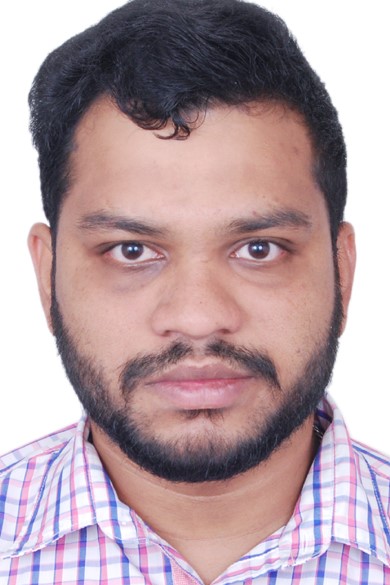
sutish pradhan
sutish pradhan
Hey there! I am Sutish I am a Devops engineer, started writing articles on my DevOps and cloud journey. My purpose is to share the concepts that I learn, the projects that I build, and the tasks that I perform regarding DevOps. Hope you all find it useful.