Day4: Task 2 Monitoring Metrics Script of the Bash Script! ๐
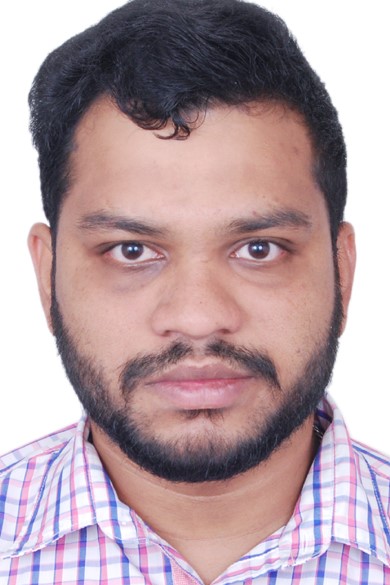
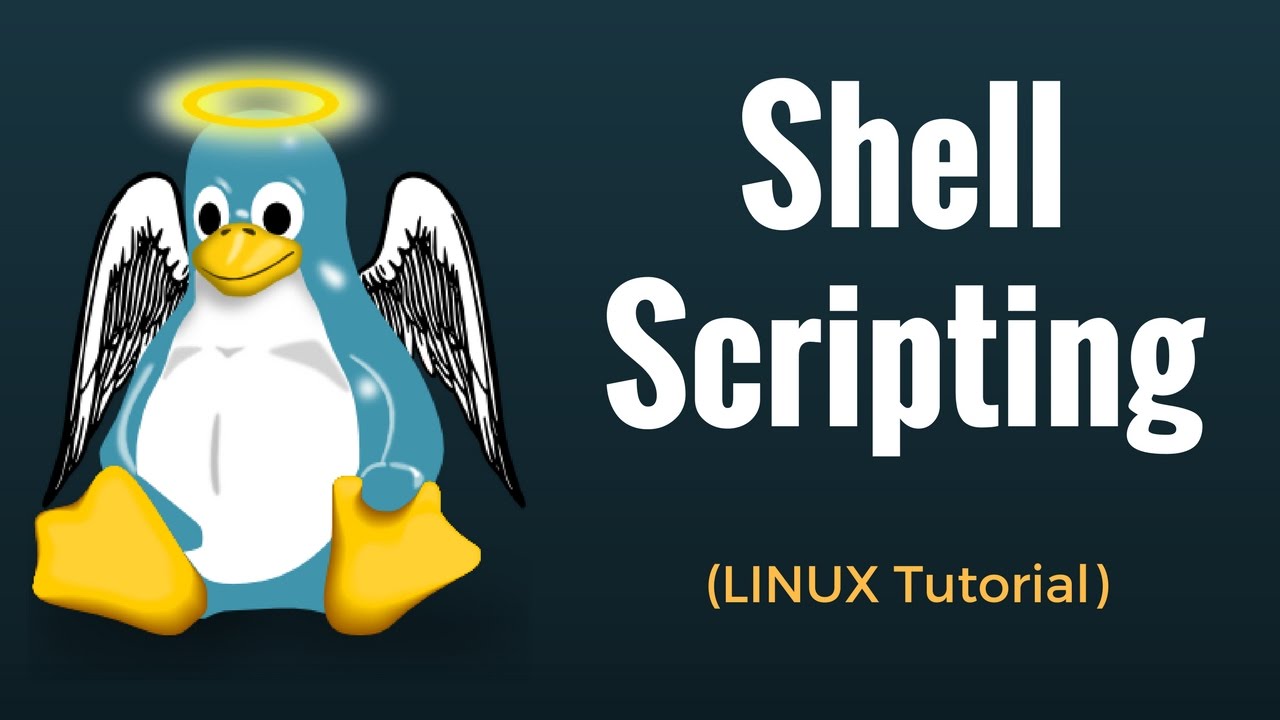
๐Introduction:
Welcome to the world of Linux process monitoring! In this blog, we will write a Bash script that monitors system metrics like CPU usage, memory usage, and disk space usage. The script will provide a user-friendly interface, allow continuous monitoring with a specified sleep interval, and extend its capabilities to monitor specific services like Nginx.
๐The Tasks: Implementing the Perfect Monitoring Script
Enhance the script by providing a user-friendly interface that allows users to interact with the script through the terminal. Display a simple menu with options to view the metrics and an option to exit the script.
Implementing Basic Metrics Monitoring ๐ we will write a Bash script that monitors the CPU usage, memory usage, and disk space usage of the system using the
top
,free
, anddf
commands. These commands provide real-time data on CPU usage, memory usage, and disk space, respectively.User-Friendly Interface ๐ค we will enhance the script by providing a user-friendly interface that allows users to interact with the script through the terminal. Display a simple menu with options to view the metrics and an option to exit the script.
Continuous Monitoring with Sleep โฐ Continuous monitoring is a key feature. We'll introduce a loop to keep fetching metrics until the user decides to exit. We'll also add a "sleep" mechanism to pause monitoring at a specified interval.
Monitoring Nginx Service ๐ Extending the script's capabilities, we'll check if the Nginx service is running and display its status. If it's not running, the user can start it using the script.
Monitoring a Different Service ๐ ๏ธ We'll take it a step further by allowing users to monitor any service of their choice. The script will prompt the user to enter the service name and display its status accordingly.
Error Handling โ To make the script robust, we'll implement error handling. Meaningful error messages will guide users on troubleshooting and fixing issues.
๐ Let's Dive into the Script!
#!/bin/bash
# Function to display system metrics (CPU, memory, disk space)
function view_system_metrics() {
echo "---- System Metrics ----"
# Fetch CPU usage using `top` command and extract the value using awk
cpu_usage=$(top -bn 1 | grep '%Cpu' | awk '{print $2}')
# Fetch memory usage using `free` command and extract the value using awk
mem_usage=$(free | grep Mem | awk '{printf("%.1f", $3/$2 * 100)}')
# Fetch disk space usage using `df` command and extract the value using awk
disk_usage=$(df -h / | tail -1 | awk '{print $5}')
echo "CPU Usage: $cpu_usage% Mem Usage: $mem_usage% Disk Space: $disk_usage"
}
# Function to monitor a specific service
function monitor_service() {
echo "---- Monitor a Specific Service ----"
read -p "Enter the name of the service to monitor: " service_name
# Check if the service is running using `systemctl` command
if systemctl is-active --quiet $service_name; then
echo "$service_name is running."
else
echo "$service_name is not running."
read -p "Do you want to start $service_name? (Y/N): " choice
if [ "$choice" = "Y" ] || [ "$choice" = "y" ]; then
# Start the service using `systemctl` command
systemctl start $service_name
echo "$service_name started successfully."
fi
fi
}
# Main loop for continuous monitoring
while true; do
echo "---- Monitoring Metrics Script ----"
echo "1. View System Metrics"
echo "2. Monitor a Specific Service"
echo "3. Exit"
read -p "Enter your choice (1, 2, or 3): " choice
case $choice in
1)
view_system_metrics
;;
2)
monitor_service
;;
3)
echo "Exiting the script. Goodbye!"
exit 0
;;
*)
echo "Error: Invalid option. Please choose a valid option (1, 2, or 3)."
;;
esac
# Sleep for 5 seconds before displaying the menu again
sleep 5
done
๐ Running the Script
Now that we are ready with the script, we're ready to make it executable.
To make your script executable use the command:
chmod 744 monitoring_script.sh
After making it executable we're ready to run it using the command below
./monitoring_script.sh
๐ฅ๏ธ Output Screens
๐ Conclusion
With this powerful and user-friendly Bash script, you can now effortlessly monitor your system's performance and crucial services.
Hope you found this blog useful! ๐
Thank you for reading!
Contact me at :
linkedin: https://www.linkedin.com/in/sutish-pradhan/
E-mail: psutish@gmail.com
Subscribe to my newsletter
Read articles from sutish pradhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
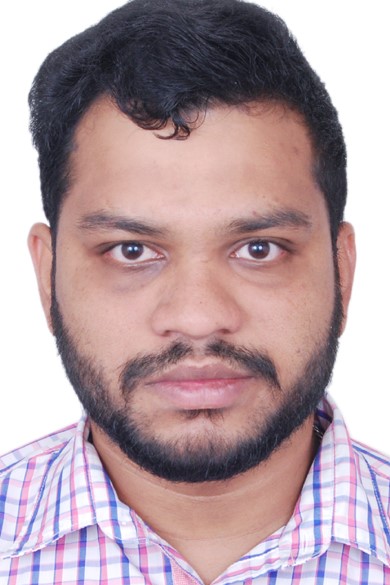
sutish pradhan
sutish pradhan
Hey there! I am Sutish I am a Devops engineer, started writing articles on my DevOps and cloud journey. My purpose is to share the concepts that I learn, the projects that I build, and the tasks that I perform regarding DevOps. Hope you all find it useful.