Beginning Day 7 of my 100 Days DSA Challenge

2 min read
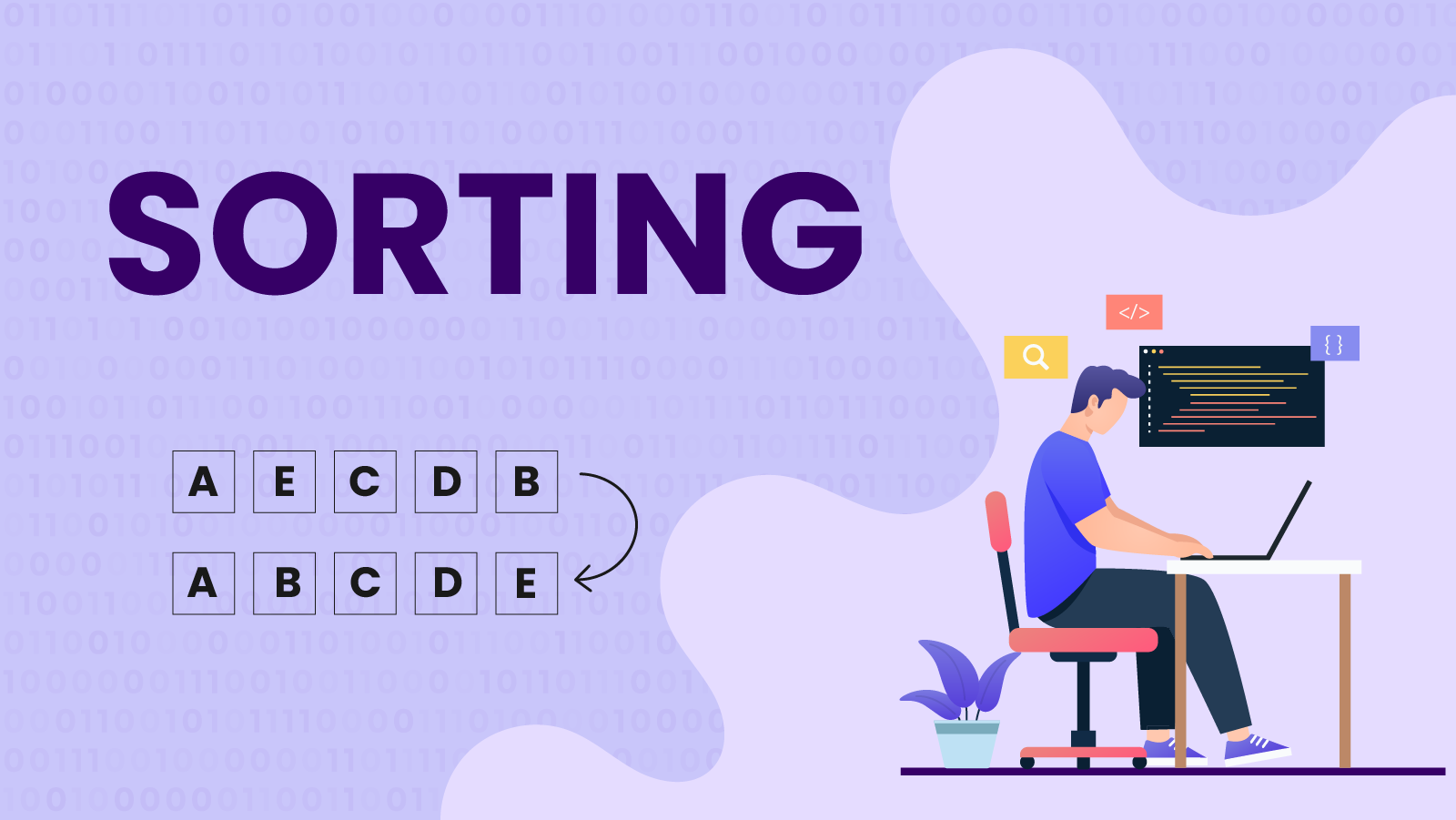
Hello! guys. It's yours truly on this side. I know you guys eagerly waiting for my today's blog, so here I am.
Today we'll be going via the concept of sorting and the simplest type of sorting i.e. bubble sort. So, not delaying any longer and starting with today's topic. Before understanding this topic you should know what is sorting in real life. It's basically just arranging a collection of items in a particular way.
Sorting is the process of arranging the data either in ascending or in descending order.
#include<bits/stdc++.h>
using namespace std;
int main(){
vector <int> arr{10, 70, 8, 1, 9, 50, 100};
sort(arr.begin(), arr.end());
cout << "Array after sorting...\n";
for (auto x : arr)
{
cout << x << " ";
}
return 0;
}
The above code gave you a hint about what's sorting and how we perform it.
- Bubble Sort = It's the way of sorting any data structure by comparing the current element with its next element. The process is done till the end of the data structure. and the result that we obtain is a sorted array.
#include<bits/stdc++.h>
using namespace std;
void BubbleSort(int arr[], int size){
if(size ==0 ||size ==1){
return;
}
for(int i = 0; i < size-1; i++){
if(arr[i] > arr[i+1]){
swap(arr[i], arr[i+1]);
}
}
BubbleSort(arr, size-1);
}
int main(){
int n;
cout << "Enter the size of array : ";
cin >> n;
int arr[n];
cout << "Enter the elements of array : ";
for (int i = 0; i < n; i++)
{
cin >> arr[i];
}
BubbleSort(arr, n);
cout << "Array after sorting...\n";
for (int i = 0; i < n; i++)
{
cout << arr[i] << " ";
}
return 0;
}
11
Subscribe to my newsletter
Read articles from Sawan Badhwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
