"Understanding C++ Function Parameters: Pass by Value vs. Pass by Reference Explained"

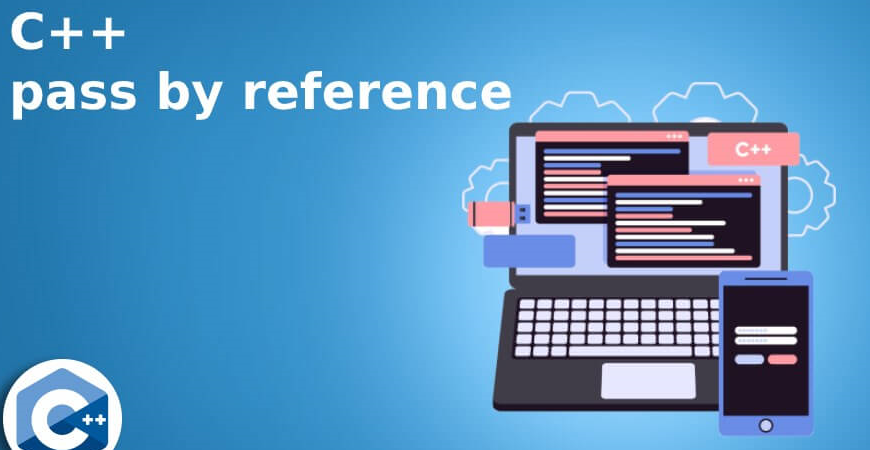
Introduction
When working with functions in C++, understanding how data is passed between functions is essential. Two common methods for passing data are pass by value and pass by reference. In this blog post, we'll explore these concepts in-depth, explaining the differences and helping you understand when to use each approach.
Before getting started let us discuss about reference variable. In an easy way to explain, a boy who has different names. He is called by the name "LOVE" at home but "LOVELY" at school but he is the same boy having 2 different names.
Now the technical definition, a reference variable is an alternative name for an existing variable. It allows you to manipulate the original variable directly, using a different name. Reference variables are declared using the &
symbol.
#include<iostream>
using namespace std;
int main()
{
int i=5;
int& j=i;//reference variable
cout<<i<<endl;//5
cout<<j<<endl;//5
j++;
cout<<i<<endl;//6
cout<<j<<endl;//6
return 0;
}
Explanation:
int& j = i;
creates a reference variablej
that is initialized with the value ofi
. In other words,j
is a reference to the same memory location asi
, so any changes made toj
will affecti
and vice versa.Initially, both
i
andj
have the value5
, so the first twocout
statements print5
for both variables.When
j
is incremented (j++
), it modifies the original variablei
. Therefore, after the increment operation, bothi
andj
have the value6
, so the last twocout
statements print6
for both variables.
Now, let's talk about pass-by value and pass-by reference with functions :
Pass By Value :-
#include <iostream>
using namespace std;
void update(int n)
{
n++;
}
int main()
{
int n = 5;
cout << "before " << n << endl;//5
update2(n);
cout << "After " << n << endl;//5
return 0;
}
EXPLANATION :
The code starts by including the necessary header file
iostream
, which is used for input and output operations in C++.The
using namespace std;
line allows you to use standard C++ names likecout
without needing to prefix them withstd::
.The
update
function is defined, which takes an integern
as a parameter. However, this function does not actually modify the original value ofn
passed to it. It only increments the local parametern
by 1 inside the function. Since the parameter is passed by value, the changes made to it inside the function are local and do not affect the variablen
in themain
function.Inside the
main
function, an integer variablen
is declared and initialized with the value5
.The program prints the value of
n
before calling theupdate
function:"before 5"
. This is done using thecout
statement.The
update
function is called with the variablen
as an argument. However, the function does not modify the originaln
variable declared in themain
function. The localn
inside the theupdate
function is modified, but this change does not affect then
in themain
function because the parameter is passed by value, creating a separate copy of the variable inside the function.After the
update
function call, the program prints the value ofn
again usingcout
. Since theupdate
function did not modify the originaln
, the output will be"After 5"
.
In summary, the code demonstrates the concept of passing parameters by value to a function in C++. Any modifications made to the parameters inside the function do not affect the original variables passed to the function.
Pass By Reference :-
#include <iostream>
using namespace std;
void update2(int &n)
{
n++;
}
int main()
{
int n = 5;
cout << "before " << n << endl;//5
update2(n);
cout << "After " << n << endl;//6
return 0;
}
The
update2
function is defined to take an integer parametern
by reference (&n
). By passingn
by reference, any modifications made ton
inside the function will directly affect the original variable passed from the calling function.In the
main
function, an integer variablen
is declared and initialized with the value5
.The program prints the initial value of
n
using thecout
statement:"before 5"
. This shows the value ofn
before any modifications.The
update2
function is called withn
as an argument. Sincen
is passed by reference, any changes made ton
inside theupdate2
function will directly modify the originaln
variable declared in themain
function.After the function call, the program prints the modified value of
n
using thecout
statement:"After 6"
. This shows the value ofn
after theupdate2
function has incremented it.The
main
function ends by returning 0, indicating successful execution of the program.In summary, this code demonstrates the concept of passing a variable by reference to a function in C++. The
update2
function modifies the original variablen
directly, allowing changes made inside the function to be reflected outside the function's scope. As a result, the output of the program shows the value ofn
before and after the function call, demonstrating how pass by reference works in C++.Choosing the Right Approach
Use pass by value when you want to work with a local copy of the data without modifying the original variable.
Use pass by reference when you need to modify the original data within the function or want to avoid the overhead of copying large data structures.
Conclusion
Understanding these concepts is crucial for writing efficient and maintainable C++ code. By choosing the appropriate parameter passing method, you can optimize your functions for better performance and readability.Conclusion
In C++, the choice between pass by value and pass by reference depends on the specific requirements of your functions. By understanding these concepts, you can design your functions effectively, ensuring that your code is both efficient and functional. Whether you're a beginner or an experienced programmer, mastering these fundamental concepts is a key step towards becoming proficient in C++ development.
Experiment with both methods, and consider the context of your program to determine which approach is best suited for your needs. Happy coding!
Subscribe to my newsletter
Read articles from RuchikaRawani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

RuchikaRawani
RuchikaRawani
Hey there! I'm Ruchika Rawani, a second-year student at LPU Punjab, pursuing BCA. Currently immersed in the world of Data Structures and Algorithms (DSA), I am also exploring the realms of web development. As a fellow beginner, I channel my learning journey into insightful blogs tailored for beginners. Writing not only serves as a means of sharing knowledge but also acts as a powerful tool for solidifying my own understanding of complex topics. Stay tuned as I continue to unravel the mysteries of coding and web development through my engaging and beginner-friendly blogs.