Understanding the Difference Between "==" and "===" in JavaScript
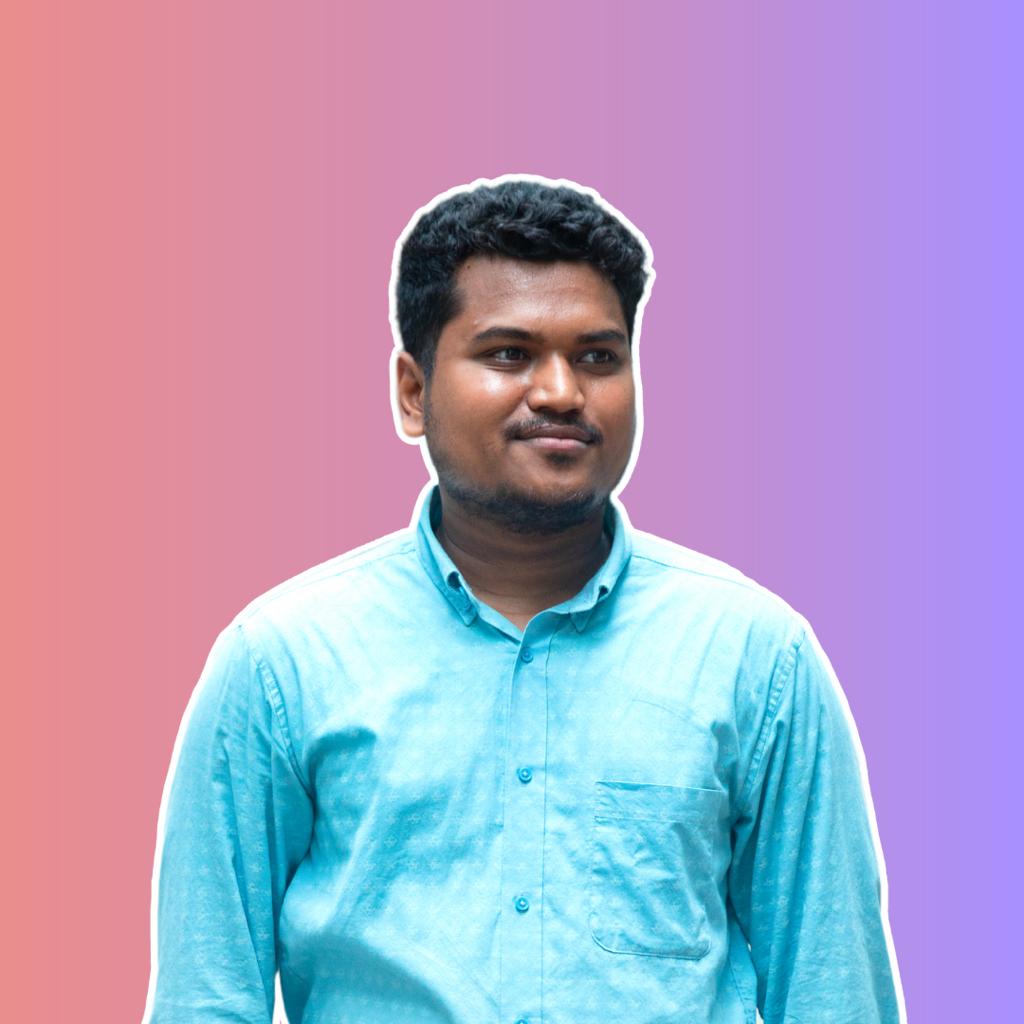
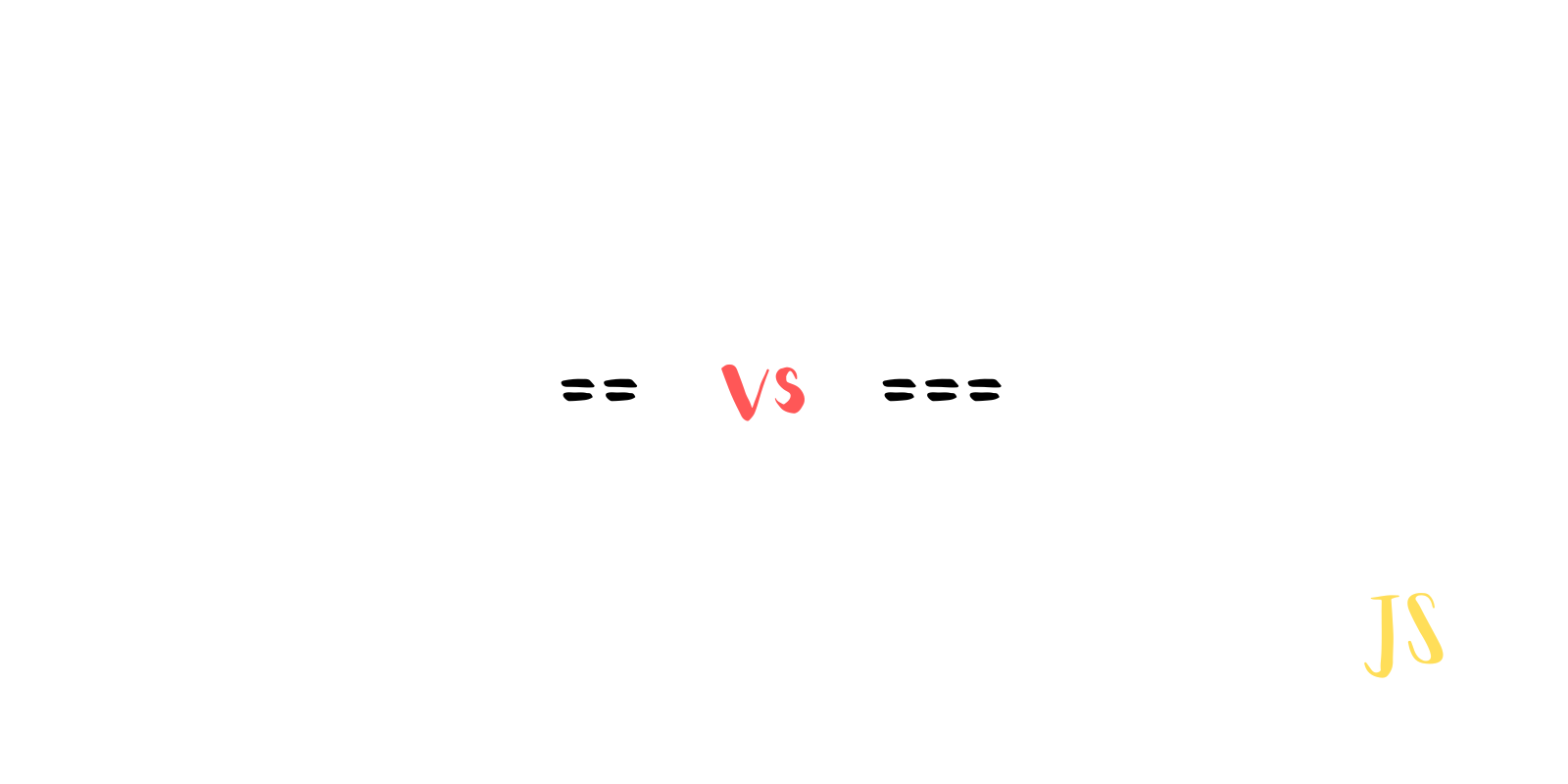
Introduction:
If you're new to JavaScript, you might find the ==
and ===
operators a bit confusing. But don't worry, by the end of this blog post, you'll have a clear idea of what they do and when to use them.
The Basics: ==
vs. ===
Both ==
and ===
are used to compare things in JavaScript. However, they do it in slightly different ways.
==
- The Abstract Equality Operator: This operator doesn't care much about data types. It tries to make things match by converting them if needed. Sometimes, this can lead to surprises when comparing different types of things.===
- The Strict Equality Operator: Unlike==
, this one insists that both things you're comparing have to be exactly the same type and value. It doesn't try to change their type. This makes it more precise and predictable.
Examples:
Let's see how they work with some examples:
Using ==
(loose equality):
console.log(2 == 2);
// Output: true, because both things are the same.
console.log(2 == '2');
// Output: true, because it turns the string '2' into a number before comparing.
console.log(false == false);
// Output: true, because both things are the same.
console.log(false == 0);
// Output: true, because it turns '0' into false before comparing.
Using ===
(strict equality):
console.log(2 === 2);
// Output: true, because both things are the same type and value.
console.log(2 === '2');
// Output: false, because it doesn't change the type, and they're different types.
console.log(false === false);
// Output: true, because both things are the same type and value.
console.log(false === 0);
// Output: false, because it doesn't change the type, and they're different types.
Conclusion:
When in doubt, it's often safer to use ===
(strict equality) because it cares about both the type and the value being the same. This can help avoid unexpected surprises in your JavaScript code, making it more reliable and sturdy.
Now that you understand these operators, you're better equipped to use them wisely in your code. Happy coding! ๐
Subscribe to my newsletter
Read articles from Hasan Rahman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
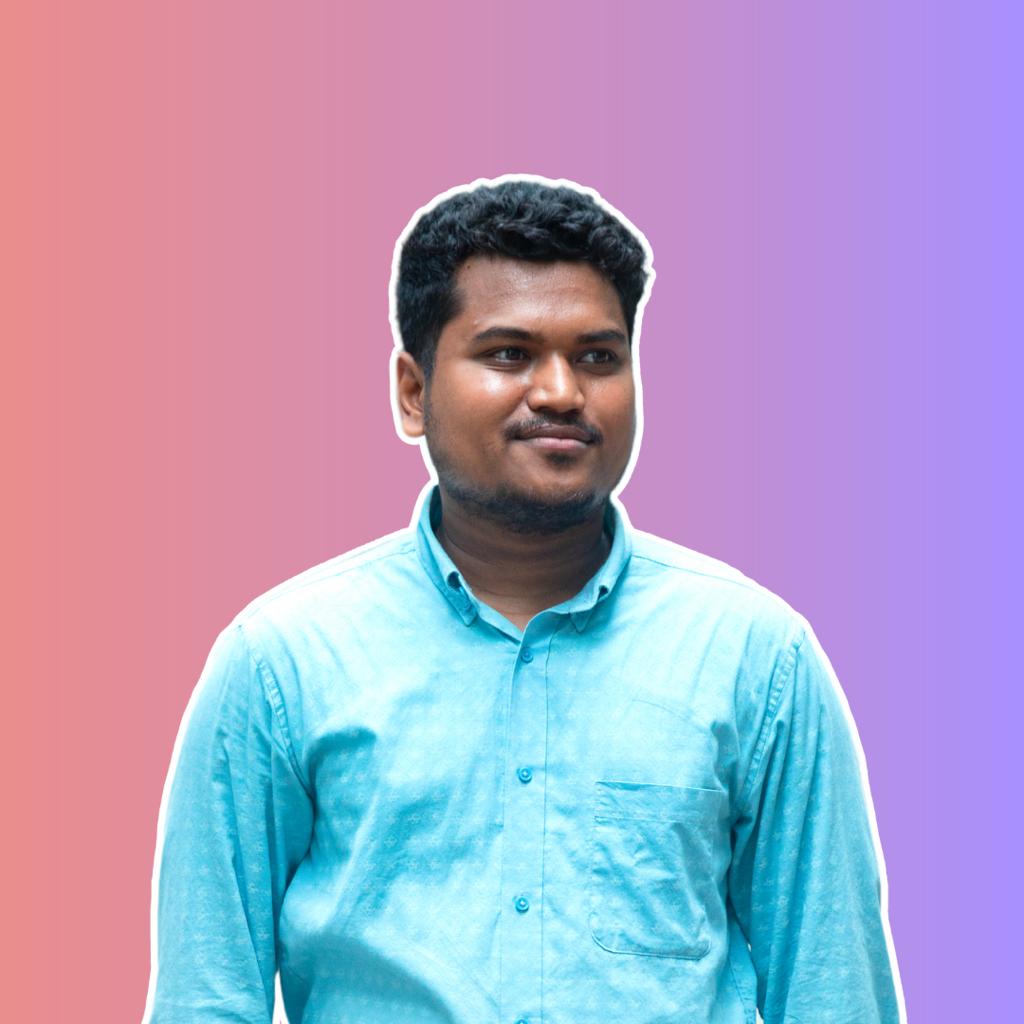