C Structures (also called structs) for absolute beginners
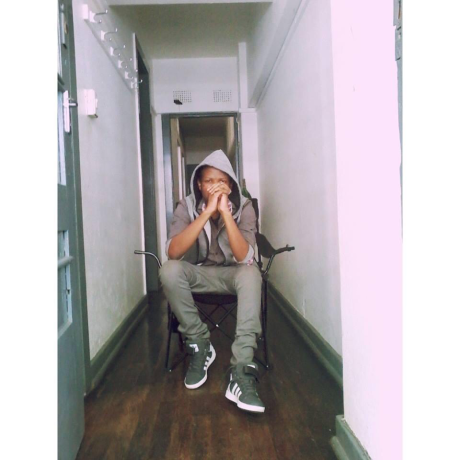
Introduction
Structs are like supercharged variables that allow you to group related information together. They are perfect for representing real-world objects or entities in your code. In this article, we will explore the basics of structs, including their declaration, initialization, and how they relate to variables and arrays. By the end, you'll have a solid understanding of structs and how they can make your code more organized and powerful.
What are Structs?
Imagine you have a bunch of information about a person: their name, age, and address. Instead of storing each piece of information in separate variables, structs let you combine them into a single entity. It's like creating a custom data type just for that person. This custom data type is called a struct.
Declaration of Structs
To create a struct, you need to define its name and the data members it contains. Each data member has a name and a data type, just like variables. Here's an example:
struct Person {
string name;
int age;
string address;
};
In this example, we've defined a struct called "Person" with three data members: "name" (a string), "age" (an integer), and "address" (another string). Think of a struct as a blueprint for creating variables that hold this specific set of information.
Initialization of Structs
Once you've defined a struct, you can create variables of that struct type and give them values. There are two ways to initialize a struct: using the dot notation or using an initializer list.
- Dot Notation: With the dot notation, you assign values to each member individually. Here's an example:
Person john;
john.name = "John Doe";
john.age = 25;
john.address = "123 Main St";
In this example, we've created a variable called "john" of type "Person" and assigned values to its members using the dot notation. Now, "john" holds all the information about a person.
- Initializer List: Alternatively, you can use an initializer list to initialize a struct in one line. Here's an example:
Person jane = {"Jane Smith", 30, "456 Elm St"};
In this example, we've created a variable called "jane" of type "Person" and provided values for its members using the initializer list. It's a more concise way of initializing a struct.
Accessing Struct Members
To access the members of a struct, you use the dot notation. It's similar to accessing variables or elements in an array. For example, to access the name of the person stored in the john
variable, you would use john.name
.
cout << "Name: " << john.name << endl;
cout << "Age: " << john.age << endl;
cout << "Address: " << john.address << endl;
In this example, we use the dot notation to access the name, age, and address members of the john
variable and print their values. It's like accessing specific pieces of information from a struct.
Structs and Variables
Think of a struct as a supercharged variable that can hold multiple pieces of information. Instead of having separate variables for a person's name, age, and address, you can group them together in a struct. It makes your code more organized and easier to work with.
Structs and Arrays
Arrays are great for storing multiple values of the same data type. But what if you want to store different types of information together? That's where structs shine. You can create an array of structs to store a collection of related information. For example:
Person people[3];
people[0] = {"John Doe", 25, "123 Main St"};
people[1] = {"Jane Smith", 30, "456 Elm St"};
people[2] = {"Bob Johnson", 40, "789 Oak Ave"};
In this example, we've created an array called "people" that can hold three "Person" structs. Each element of the array represents a different person with their own name, age, and address.
Conclusion
Structs are a powerful tool for organizing and managing related information in your code. They allow you to create custom data types that can hold multiple pieces of data. By understanding structs and how they relate to variables and arrays, you can take your programming skills to the next level.
I hope this article has provided you with a clear and beginner-friendly understanding of structs. Happy coding!
Subscribe to my newsletter
Read articles from Wisdom Ncube directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
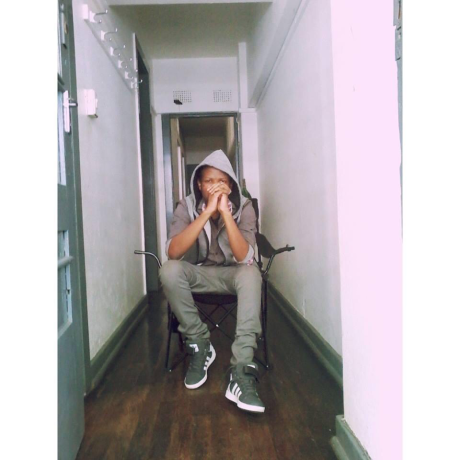
Wisdom Ncube
Wisdom Ncube
I am a Software Engineering learner at ALX documenting my journey.