Class Based Component & React Life Cycle
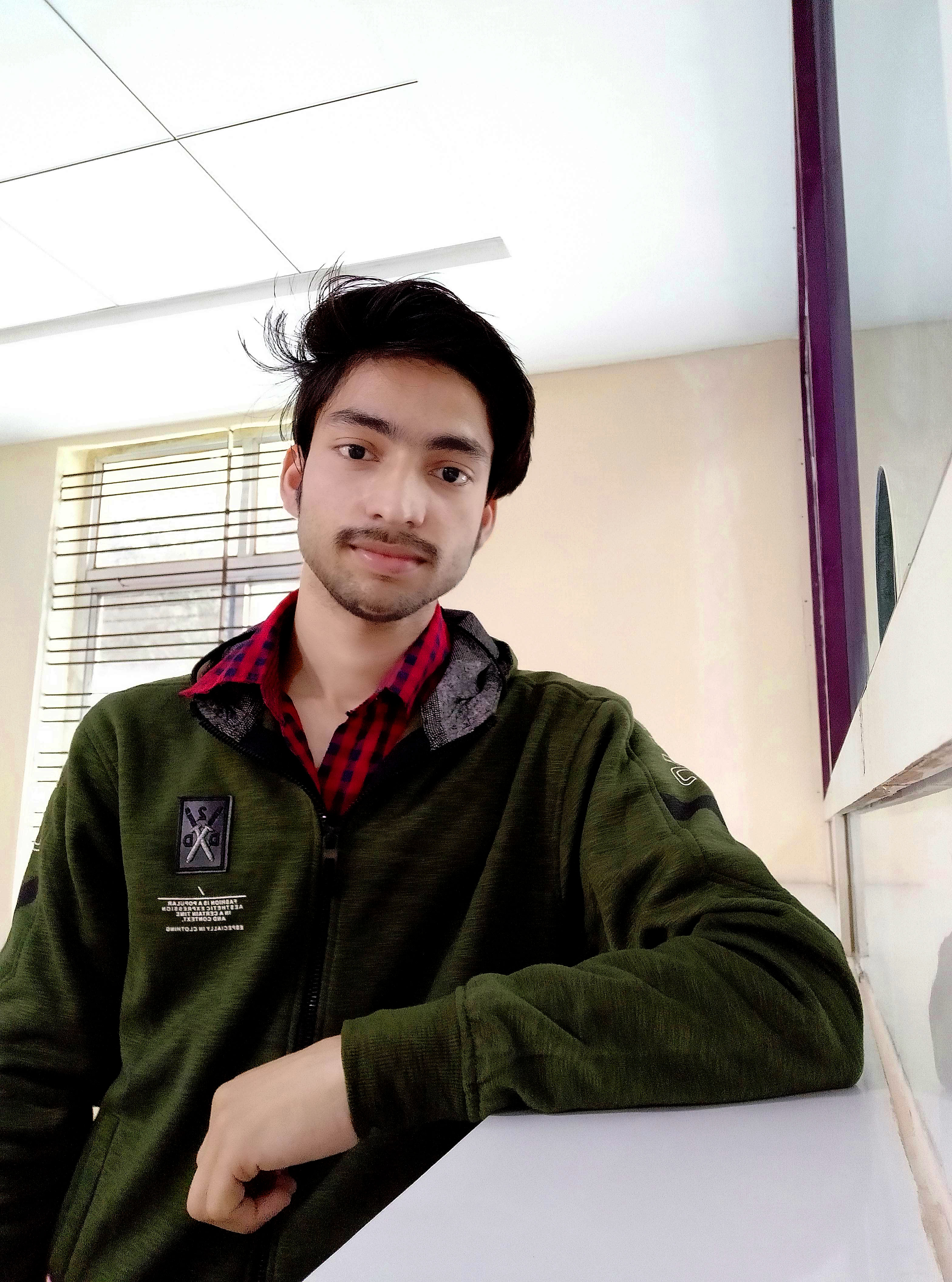
4 min read
Class Based Components :
1. Syntax :
import React from "react";
function App() {
return (
<div>App</div>
);
}
class App extends React.Component {
render() {
return <div>React Class Based Component - App</div>
}
}
export default App;
1.2 Advance
class App extends React.Component {
constructor(props) {
super(props); // parent class ko props send ho jaayega
this.state = {
'myName': 'Er. Ayush Kumar',
'skill': 'Web Developer',
};
this.state = { count: 0 };
// To use this out of this class in any function, we require π
this.incrementFun = this.incrementFun.bind(this)
this.decrementFun = this.decrementFun.bind(this)
this.handleChange = this.handleChange.bind(this)
}
incrementFun() {
console.log('Working +');
// π―β Different ways to update value inside the function
this.setState({ count: this.state.count + 1 })
// this.setState((prev) => {
// return {count : prev.count+1}
// })
// this.setState({
// 'count': 20
// })
}
// βββπ― Different ways to handle change in the input tag
handleChange(e) {
this.setState({
// myName: 'Ayush' // not working
myName: e.target.value
})
}
render() {
return (
<>
<h1 >{this.state.myName}</h1>
<h3>{this.state.skill}</h3>
<button onClick={this.decrementFun} className="">
Decrement
</button>
<button onClick={this.incrementFun} className="bg-green-500 hover:bg-green-700 text-white font-bold py-2 px-4 rounded">
Increment
</button>
<hr />
{/* Input Tag me value pass -------------------------------- */}
<div className="mt-5 flex justify-center">
<input value={this.state.myName} onChange={this.handleChange} type="text" className="w-2/6 px-4 py-2 leading-tight text-gray-700 bg-gray-200 border rounded appearance-none focus:outline-none focus:bg-white focus:border-blue-500" placeholder="Enter text" />
</div>
</>
)
}
2. How to pass value through props πβ‘οΈπ?
// FileName: πApp.js
class App extends React.Component {
render() {
return (
<>
<NameComp name={this.state.myName} />
<NameComp name={46} />
{/* <NameComp name={[46]} /> */}
</>
)
}
}
export default App;
// FileName: NameComp.jsx
import React from 'react'
import ProtoType from 'prop-types'
class NameComp extends React.Component {
constructor(props) {
super(props)
}
render() {
return (
<div>NameComp : <p className='text-teal-600 font-bold text-2xl' >
{ this.props.name }</p>
</div>
)
}
}
NameComp.defaultProps = {
name: "No Name Added yet"
}
NameComp.propTypes = {
// name: ProtoType.string,
name: ProtoType.string.isRequired,
}
export default NameComp
II. React Life Cycle
/*
React Component Lifecycle:
1. componentDidMount
2. shouldComponentUpdate
3. componentDidUpdate
4. componentWillUnmount
*/
import React from 'react';
import NameComp from './NameComp';
class App extends React.Component {
constructor(props) {
super(props)
this.state = {
count: 0,
}
console.log('1. constructor');
this.increment = this.increment.bind(this)
}
increment() {
this.setState({
count: this.state.count + 1,
})
// Agar should shouldComponentUpdate() me return false hai, then UI me value update nhi hogi, but console me value change hota rahega.
console.log("Count Value : ", this.state.count)
}
componentDidMount() {
console.log('4.1 component mounted Successfully');
setTimeout(() => {
console.log('API Fetched (using setTimeout)');
}, 2000)
}
componentDidUpdate() {
console.log('4.2 component DID UPDATE -> YES');
setTimeout(() => {
console.log('4.2 component DID UPDATE - API Fetched (using setTimeout)');
}, 2000)
}
shouldComponentUpdate() {
let bool = true;
console.log('shouldComponentUpdate : ', bool);
return bool;
}
componentWillUnmount() {
console.log("Unmounting");
return false;
}
render() {
console.log('2. In b/w render() - return() Method');
return (
<>
{console.log('3. Inside the return method')}
React Life Cycle
<span className='my-custom block my-4' >count: {this.state.count} </span>
<button onClick={this.increment} className="my-4 mx-4 bg-blue-500 text-white hover:bg-blue-700 font-semibold py-2 px-4 rounded">
Increment
</button>
</>
)
}
}
export default App;
II.(a) Another Example using an event Listener
// FileName: πHome.js
import React, { useEffect } from 'react'
// ------- π― Home Element using Functional component ---------------------
// const HomeUsingFunctionalComponent = () => {
// const mouseHoverFunc = (e) => {
// // console.log(e.clientX, " ", e.clientY);
// console.log(e.clientX);
// console.log(e.clientY);
// }
// useEffect(() => {
// document.addEventListener('mousemove', mouseHoverFunc)
// return () => {
// document.removeEventListener('mousemove', mouseHoverFunc)
// }
// }, [])
// File Name: π Home.jsx
// return (
// <div>Welcome to Home Page</div>
// )
// }
// export default HomeUsingFunctionalComponent
// ------- π― Home Element using Functional component ---------------------
// ------- π’ Home Element using Class Based component ---------------------
const moveOverFunc = (e) => {
console.log(e.clientX)
}
export default class Home extends React.Component {
constructor(props) {
super(props);
}
componentDidMount() {
document.addEventListener("mousemove", moveOverFunc);
}
componentDidUpdate() {
document.addEventListener("mousemove", moveOverFunc);
return true;
}
shouldComponentUpdate() {
return true
}
componentWillUnmount() {
document.removeEventListener("mousemove", moveOverFunc);
}
render() {
return (
<div>Home Using Class Based Component</div>
)
}
}
// ------- π’ Home Element using Class Based component ---------------------
// FileName: πApp.js
/*
React Component Lifecycle:
1. componentDidMount
2. shouldComponentUpdate
3. componentDidUpdate
4. componentWillUnmount
*/
import React from 'react';
import NameComp from './NameComp';
import { BrowserRouter as Router, Routes, Route, Link } from 'react-router-dom'
import Home from './components/Home';
import About from './components/About';
import Navbar from './components/Navbar';
class App extends React.Component {
constructor(props) {
super(props)
this.state = {
count: 0,
}
console.log('1. constructor');
this.increment = this.increment.bind(this)
}
increment() {
this.setState({
count: this.state.count + 1,
})
// Agar should shouldComponentUpdate() me return false hai, then UI me value update nhi hogi, but console me value change hota rahega.
console.log("Count Value : ", this.state.count)
}
render() {
return (
<>
<Router>
<Navbar />
<Routes>
<Route path='/' element={<Home />} />
<Route path='/about' element={<About />} />
</Routes>
</Router>
</>
)
}
}
export default App;
0
Subscribe to my newsletter
Read articles from Ayush Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
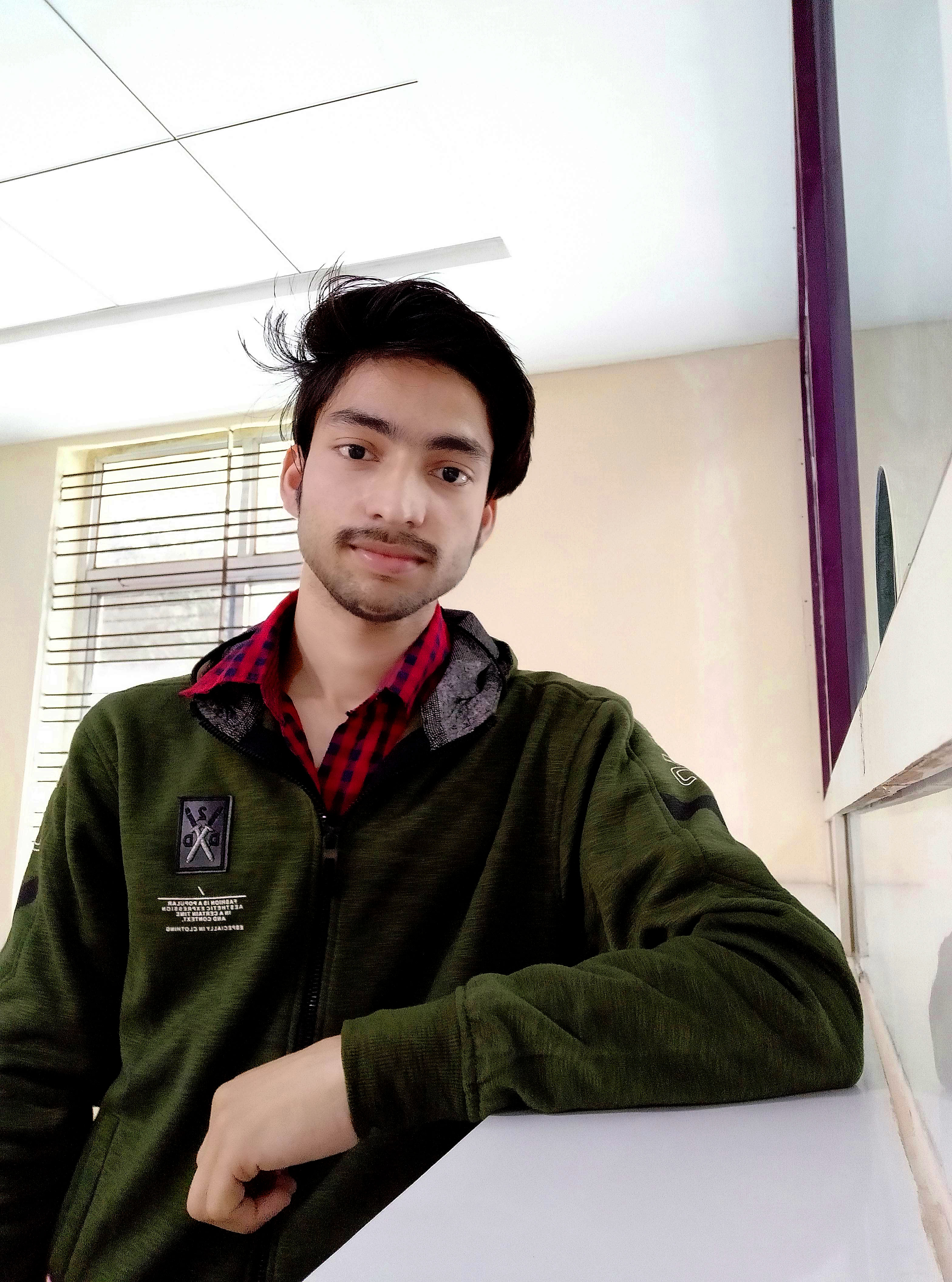
Ayush Kumar
Ayush Kumar
A passionate MERN Stack Developer from India I am a full stack web developer with experience in building responsive websites and applications using JavaScript frameworks like ReactJS, NodeJs, Express etc.,