internship journey- Learning
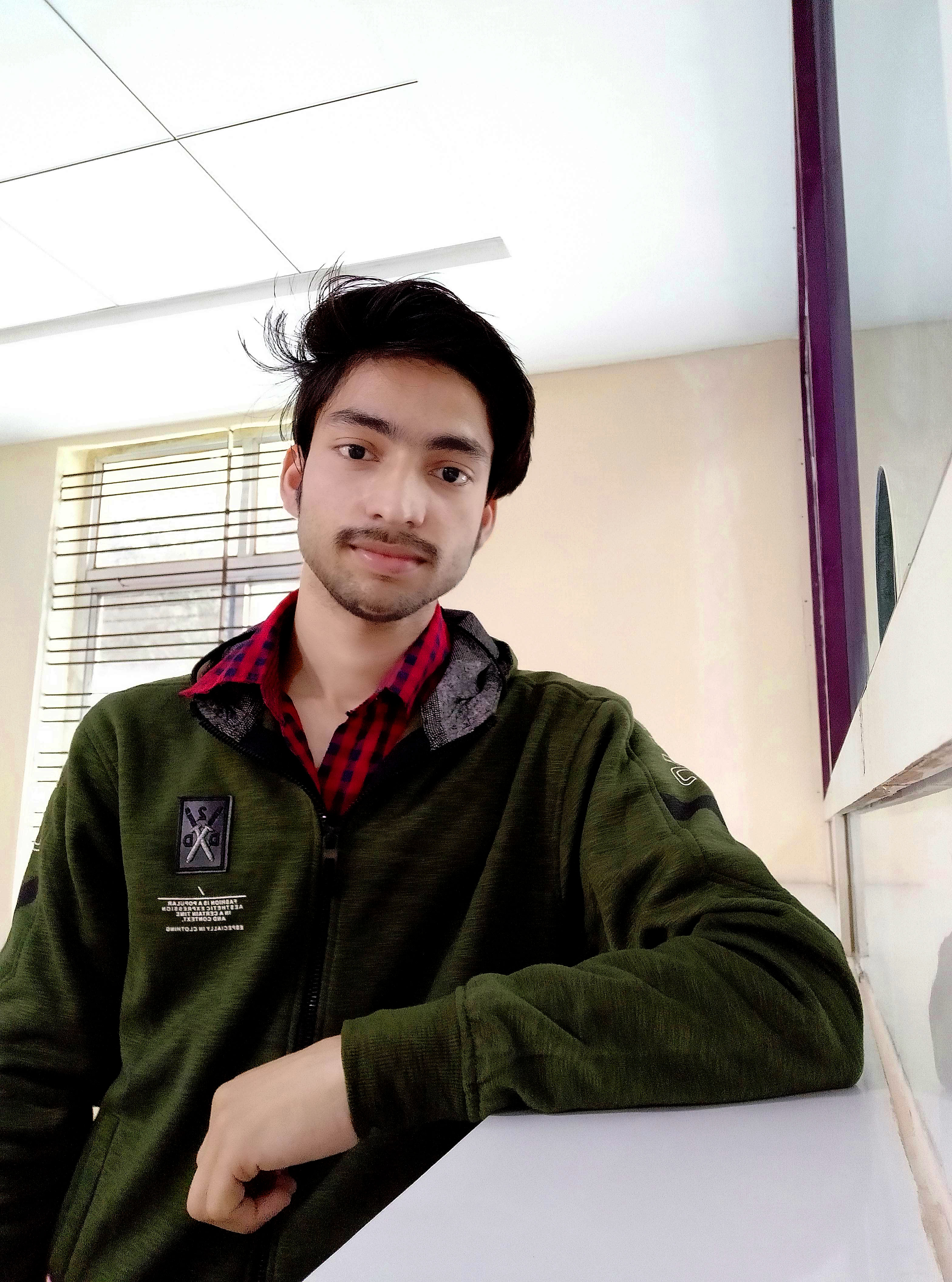
Table of contents
- [Notes 1 : Apna College π]
- Export and Import
- definition
- Code to Run in Command Prompt
- VS Code Terminal
- Solve Error while adding < p > tag
- To Create Subcomponents in navbar.js like file
- (React Functional Component) rfc Code Snippets in Product_List.js ()
- Loop in JSX
- using the State Hook
- Important Points to keep in mind π€―π€― ββ
- Make Custom changes in jsx :
- Export and Import
- definition
- Code to Run in Command Prompt
- VS Code Terminal
- Solve Error while adding < p > tag
- To Create Subcomponents in navbar.js like file
- (React Functional Component) rfc Code Snippets in Product_List.js ()
- Loop in JSX
- using the State Hook
- Important Points to keep in mind π€―π€― ββ
- Make Custom changes in jsx :
[Notes 1 : Apna College π]
Notes 3 : Form Validation using JS
Export and Import
Named Exports :
Named exports are useful to export several values. During the import, it is mandatory to use the same name of the corresponding object.
export class Sample {
constructor(name) {
this.name = name;
}
import { Sample } from "./computer.js";
Default Exports :
Default exports are useful to export only a single object, function, variable. During the import, we can use any name to import.
export default class Computer {
constructor(name) {
this.name = name;
}
run() {
console.log("The Computer is now Running");
}
}
import Computer from "./computer.js";
definition
Module :
In Node.js, Modules are the blocks of encapsulated code that communicates with an external application on the basis of their functionality.
Modules can be a single file or a collection of multiples files/folders.
The reason programmers are heavily reliant on modules is because of their re-usability as well as the ability to break down a complex piece of code into manageable chunks.
Code to Run in Command Prompt
npm : Node Package Manager
npx : Node Package Execute
Run These Commands in CMD π
node --version
npm --version
npx -v
#
#
VS Code Terminal
Create React App
React JS Documentation Link π
Create React App is a comfortable environment for learning React, and is the best way to start building a new single-page application in React.
It sets up your development environment so that you can use the latest JavaScript features, provides a nice developer experience, and optimizes your app for the production.
To create a project, run :
npx create-react-app my-app-name
npm start β
This π command will not run because you have to move inside that folder where node is created
ls // This will list all the folder
cd project_name
npm start β
You can view namaste-world in the browser
Local : http://localhost:3000
React JS has hot reloading features. (Live Server Features)
Solve Error while adding < p > tag
/Project_name/src/App.js
function App() {
return (
<> β
<React.Fragment> β
<p>{1 + 1}</p>
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React <br></br>
Namaste React
</a>
</header>
</div>
</React.Fragment> β
</> β
);
}
Go one folder back π
cd ../
Important Extension in VS Code for React JS :
ES7+ React/Redux/React-Native snippets
Prettier - Code formatter
Simple React Snippets
Material Icon Theme by Philipp Kief
getbootstrap.com
Copy some links like π
jsDelivr
When you only need to include Bootstrapβs compiled CSS or JS, you can use jsDelivr.
and PASTE it to the index.html
project_name/public/index.html
Note : β Where π€·ββοΈ to put Bootstrap JS Link π
β Before the END of the Body Tag.
<!-- β¨ JavaScript Bundle with Popper β¨ -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/js/bootstrap.bundle.min.js" integrity="sha384-ka7Sk0Gln4gmtz2MlQnikT1wXgYsOg+OMhuP+IlRH9sENBO0LRn5q+8nbTov4+1p" crossorigin="anonymous"></script>
</body>
</html>
To Create Subcomponents in navbar.js like file
To write React JS Snippets.
Install Extension :
- Simple React Snippets
You will get default Output as
class extends Component {
state = { }
render() {
return ( ... );
}
}
export default ;
Make Some Change Then Only it will Work by Adding π π
- Add ClassName at two positions.
- Add keyword React.Folder_Name i.e, React.Component
- ππππ
Now Updated Code will look like :β¬
class Navbar extends React.Component {
state = { }
render() {
return ( ... );
}
}
export default Navbar;
If you directly write the HTML code inside the return ( ... )
As Shown above π
You will Get Error! ββ
To Solve This Error
β You can use HTML to JSX Online Compiler π
Then only Paste the JSX Code inside the three dot
return ( ... );
Newly Added Navbar.js will work when React is import : π
In Navbar.js file, on the top of code Add :
Shortcut (type) : " imr " in vs code
import React from 'react';
...
Also import β these all component .js files in App.js
import logo from './logo.svg';
import './App.css';
import React from 'react';
import Navbar from './components/Navbar'; β
β¨
function App() {
return (
<>
<React.Fragment>
<Navbar/> β¨ β
<ProductList/>
<Footer/>
{/* If any of the above π three listed files are not Available then it will show Nothing on Screen π΅π΅ */}
</React.Fragment>
</>
);
}
export default App;
Create array object in App.js & Passing it to the Components β
function App() {
// Create Array Object for Products before return function β
β
const product = [
{
price : 99999,
name : "Iphone 10S Max",
quantity: 0,
},
{
price: 10000,
name : "Redmi Note 8",
quantity : 0,
}
]
return (
<>
<Navbar />
{/* Passing data of Product Array in the Components π β
*/}
<ProductList product={product}/>
{/* <Footer/> */}
{/* If any of the above π three listed files are not Available then it will show Nothing on Screen π΅π΅ */}
</>
);
}
(React Functional Component) rfc Code Snippets in Product_List.js ()
type rfc for React Functional Component
import React from "react";
export default function ProductList() {
return <div></div>;
}
Extracting the Passed value/object as Parameter Using :
export default function ProductList(y) {
console.log(y);
...
}
OR
/* Using props
π· It is an object which stores the value of attributes of a tag and work similar to the HTML attributes.
π· It gives a way to pass data from one component to other components.
π· It is similar to function arguments.
β¨ Note : Jitne bhi component hote hai React ke andar wo Pure Function hote hai
Koi bhi Input pass krne pe Output Same hi rhega
*/
export default function ProductList(props) {
console.log(props);
...
}
Class Component Code Snippets π
type cc to get class component code snippets
class className extends Component {
state = { }
render() {
return ();
}
}
export default className;
Loop in JSX
π΄ For loop don't work in JSX.
π’ It will support Map Function, ForEach
using the State Hook
Refer to React Hooks Documentation;
Whatβs a Hook?
Our new example starts by importing the useState Hook from React:
import React, { useState } from "react";
function Example() {
// ...
}
- What does calling useState do?
π’ To make change effective we can use document.get.ElementById('');
But agar javascript hi use krna tha Then what's the use of react
To do so, we can use useSate to make changes effective.
π’ It declares a "state variable".
- What does the useState return ?
π· Normally, variables "disappear" when the function exits but state variables are preserved by React.
import React, { useState } from 'react';
function Example() {
// Declare a new state variable, which we'll call "count"
const [count, setCount] = useState(0);
π Here count is our variable.
π· We can declare a state variable called count, and set it to 0. React will remember its current value between re-renders, and provide the most recent one to our function.
π· If we want to update the current count, we can call setCount
β Need of useState ?
// for editing title creating new variable named title
let title = props.title;
// π Effective changes is not visisble by creating varible. β
// So Let's use useState in place of variable name
useState("");
useState("Hello School");
// π here props.title is passed to the useState as a value
const [title, setTitle] = useState(props.title);
// here setTitle is the function name using which new value will be stored
JavaScript Spread Operator (...)
The JavaScript spread operator (...) allows us to quickly copy all or part of an existing array or object into another array or object.
Important Points to keep in mind π€―π€― ββ
If you are using state inside the file then must create state earlier in it. Eg. this.state
If you are using class component then create state as an OBJECT. Eg. this.props
If you are using functional components then use HOOKS.
Make Custom changes in jsx :
|class className
for= htmlFor=
- Those tags which don't have closing tags add / at the pre-end
Subscribe to my newsletter
Read articles from Ayush Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
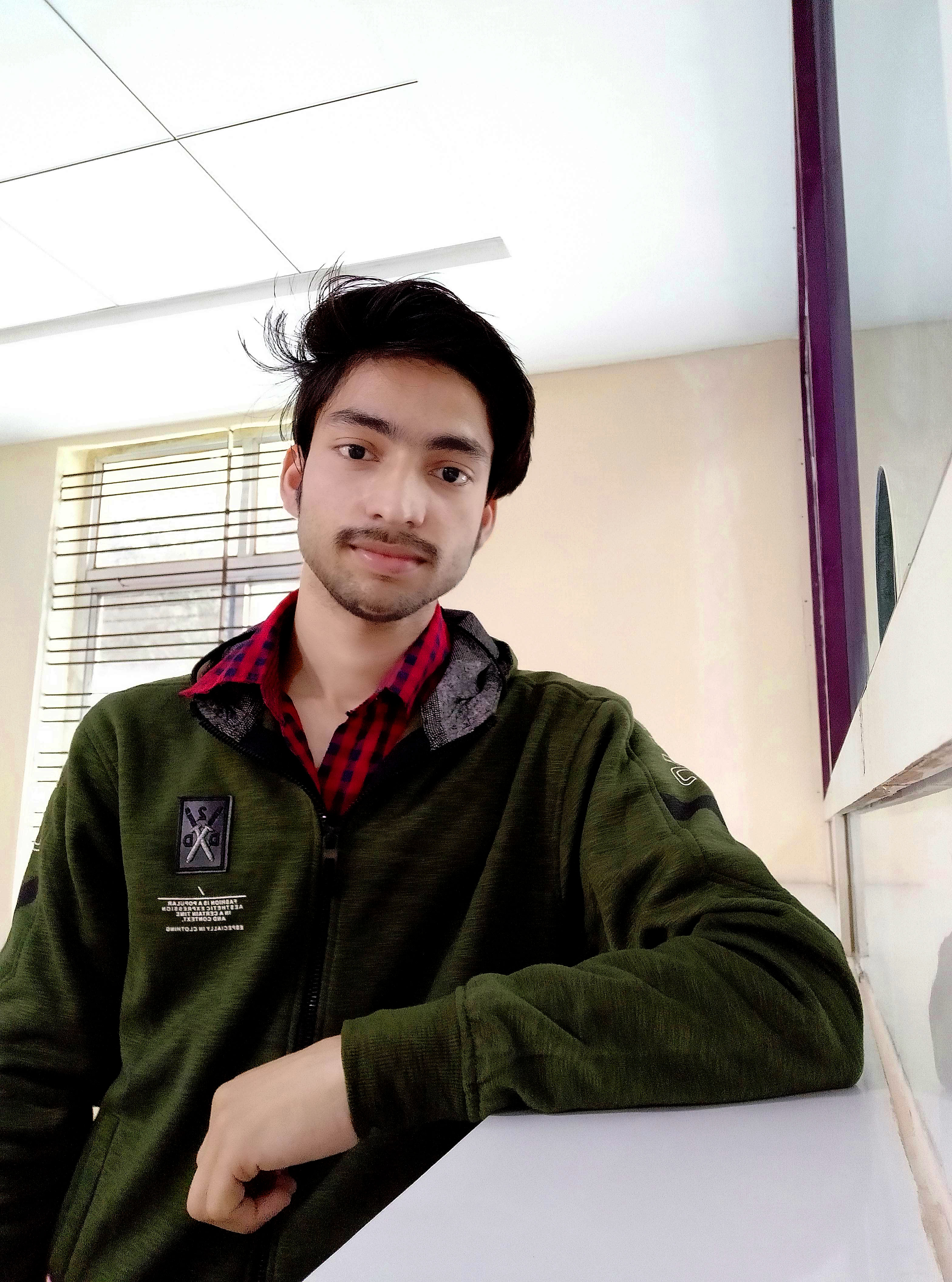
Ayush Kumar
Ayush Kumar
A passionate MERN Stack Developer from India I am a full stack web developer with experience in building responsive websites and applications using JavaScript frameworks like ReactJS, NodeJs, Express etc.,