Node.js: npm & package.json
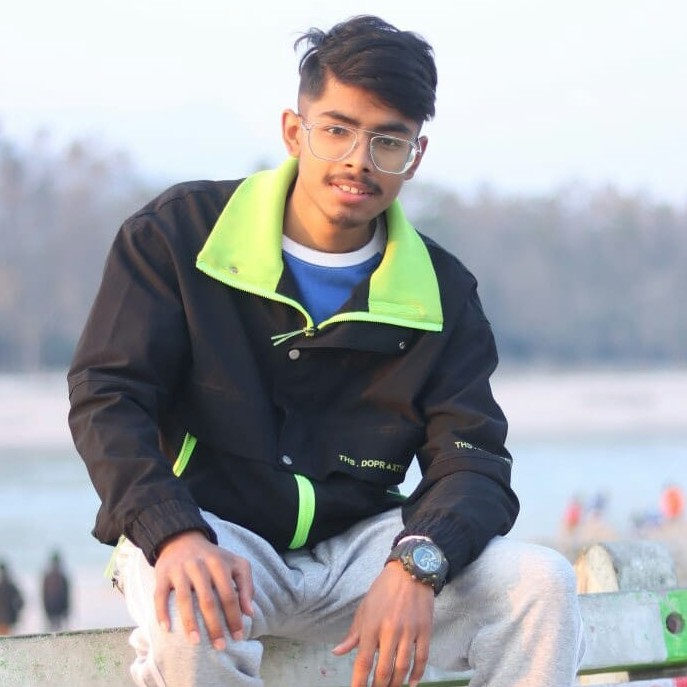
Table of contents
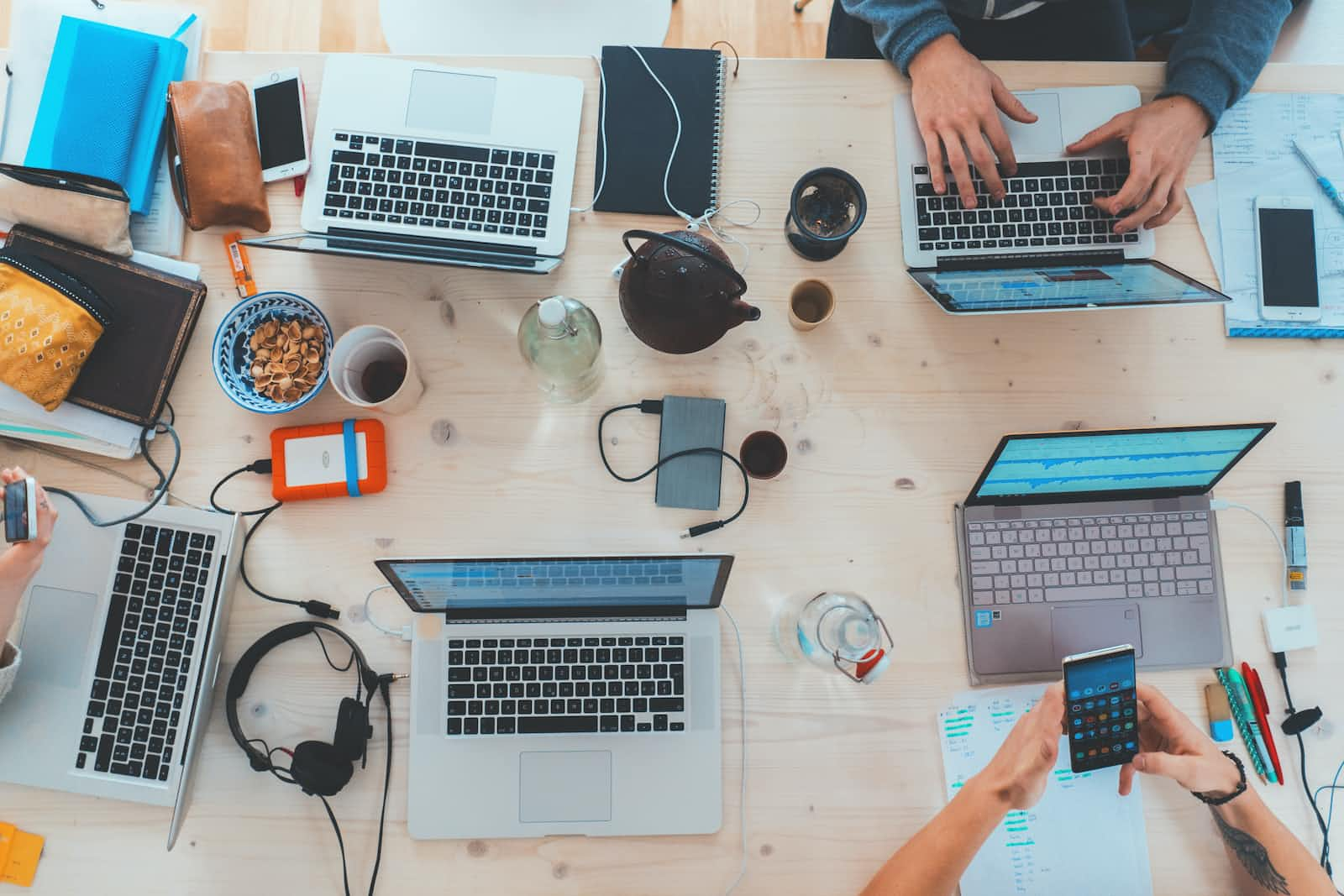
What is NPM?
It is the world's largest JavaScript package library. It has command-line tools to install and manage the packages. Once, you install Node.js on your computer, 'npm' comes with it. NPM commands are as follows:
npm install
[Installs packages from package.json]
npm install <package_name>
[Installs package locally]
npm install -g <package_name>
[Installs package globally]
npm install <package_name> --save-dev
[Installs package as dev dependency]
npm uninstall <package_name>
[Uninstalls package locally]
npm uninstall -g <package_name>
[Uninstalls package globally]
npm uninstall --save-dev <package_name>
[Uninstalls dev dependency packages]
npm outdated
[Lists out local outdated packages]
npm outdated -g
[Lists out global outdated packages]
npm update <package_name>
[Updates specific local package]
npm update -g <package_name>
[Updates specific global package]
npm update
[Updates all local packages]
npm update -g
[Updates all global packages]
Say hi to package.json!
'package.json' is a special kind of file with keys and values that is used to manage the packages that we are using. It is needed to keep track of project dependencies. To create this file, we use the following command:
npm init -y
[Creates package.json file with default values]
npm init
[Creates package.json file with custom values]
According to your project folder, the default 'package.json' looks somewhat like this:
{
"name": "my_project",
"version": "1.0.0",
"description": "This is the project/package description",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Now, you can see information about your project(or package if you're making one).
"name" specifies the name of the project/package
"description" gives a short description of your project/package
"main" is the entry point to your project/package
"scripts" include commands to run or build your project/package
Likewise, 'package.json' stores information about the packages that we install. For example:
npm install bootstrap
npm install lodash
After this, you should see a folder named 'node_modules' which contains your installed packages. Now, the 'package.json' would get updated as follows:
{
"name": "my_project",
"version": "1.0.0",
"description": "This is the project/package description",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"bootstrap": "^5.3.2",
"lodash": "^4.17.21"
}
}
We can see we have an extra key named "dependencies" that has information about the version of the packages that we just installed.
npm install
which is said to install packages from package.json file? What do we mean by that?That means whenever we run npm install
, it reads the 'package.json' and installs the listed packages in it. But, we have already installed those packages, right? So, what is the use of it?
Okay, I agree that we have already installed those packages, but what if we need to share our project with other developers? How are they gonna know which packages we used for the project?
We don't normally upload the 'node_modules' folder(which contains all our packages) on GitHub because it can become too large.
Instead, we just upload the 'package.json' file along with our project files.
Whenever other developers clone our project, 'package.json' is included in it.
Now, they can simply install the required packages by running
npm install
.
But wait, did you notice something fishy in the above diagram? The package versions of developers are different. It is because when Developer-2 installed the packages through 'package.json', npm installed the most latest version of the package possible. To know how package versions are managed, see this QnA.
Now, how do we match the package versions? This is where 'package-lock.json' comes to the rescue.
package-lock.json
'package-lock.json' locks the exact version of the packages and sub-packages that were used when creating the project. So, it is larger than 'package.json' in size.
So, do we have to create this 'package-lock.json' file by ourselves? No, you don't have to worry about it.
'package-lock.json' is created automatically when Developer-1 installs the packages for creating the project.
It also gets pushed along with the project file in Github.
When Developer-2 installs the packages, npm uses both 'package.json' and 'package-lock.json' to install the exact version of the packages.
Congratulations, you made it to the end!
Subscribe to my newsletter
Read articles from Giver Kdk directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
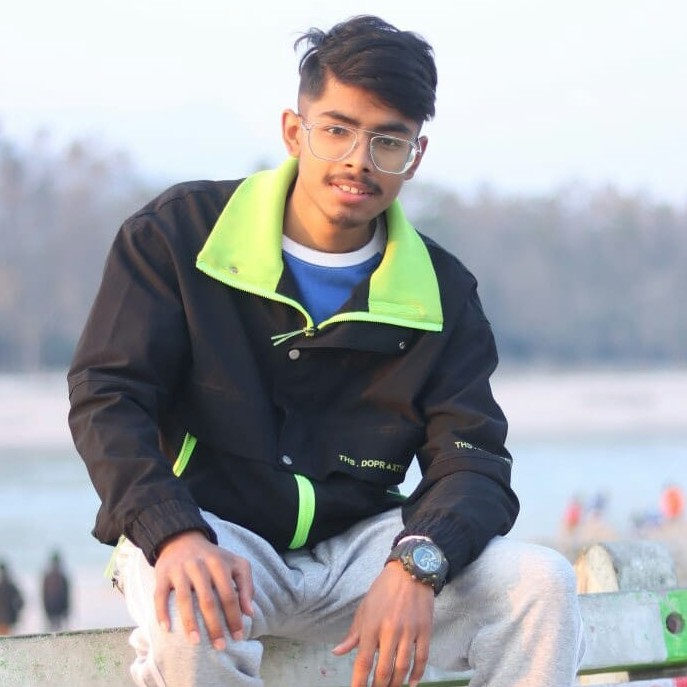
Giver Kdk
Giver Kdk
I am a guy who loves building logic.